Arduino Nano 33 IoT - Button - Debounce
When you press or release a button, or flip a switch between ON and OFF, its state changes from LOW to HIGH (or HIGH to LOW) just one time. Is this correct?
No, it's not. In the real world, when you press a button once, its state (LOW and HIGH) can change back and forth several times very quickly instead of just one time. This is because of the physical properties of the button, and it's known as chattering. Chattering can make devices like the Arduino Nano 33 IoT detect several button presses when you only pressed it once. This causes problems. The method used to fix this issue is called debouncing. This tutorial explains how to do that.
This guide gives:
How to remove false signals from a single button in Arduino Nano 33 IoT code
How to remove false signals from one button using a library in Arduino Nano 33 IoT code
How to remove false signals from several buttons using a library in Arduino Nano 33 IoT code
Or you can buy the following kits:
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand,
DIYables .
We have detailed guides about the button. These guides include clear information and simple step-by-step instructions on the hardware pin layout, how it works, how to wire it to the Arduino Nano 33 IoT, and the Arduino Nano 33 IoT code. Find out more at the links below.
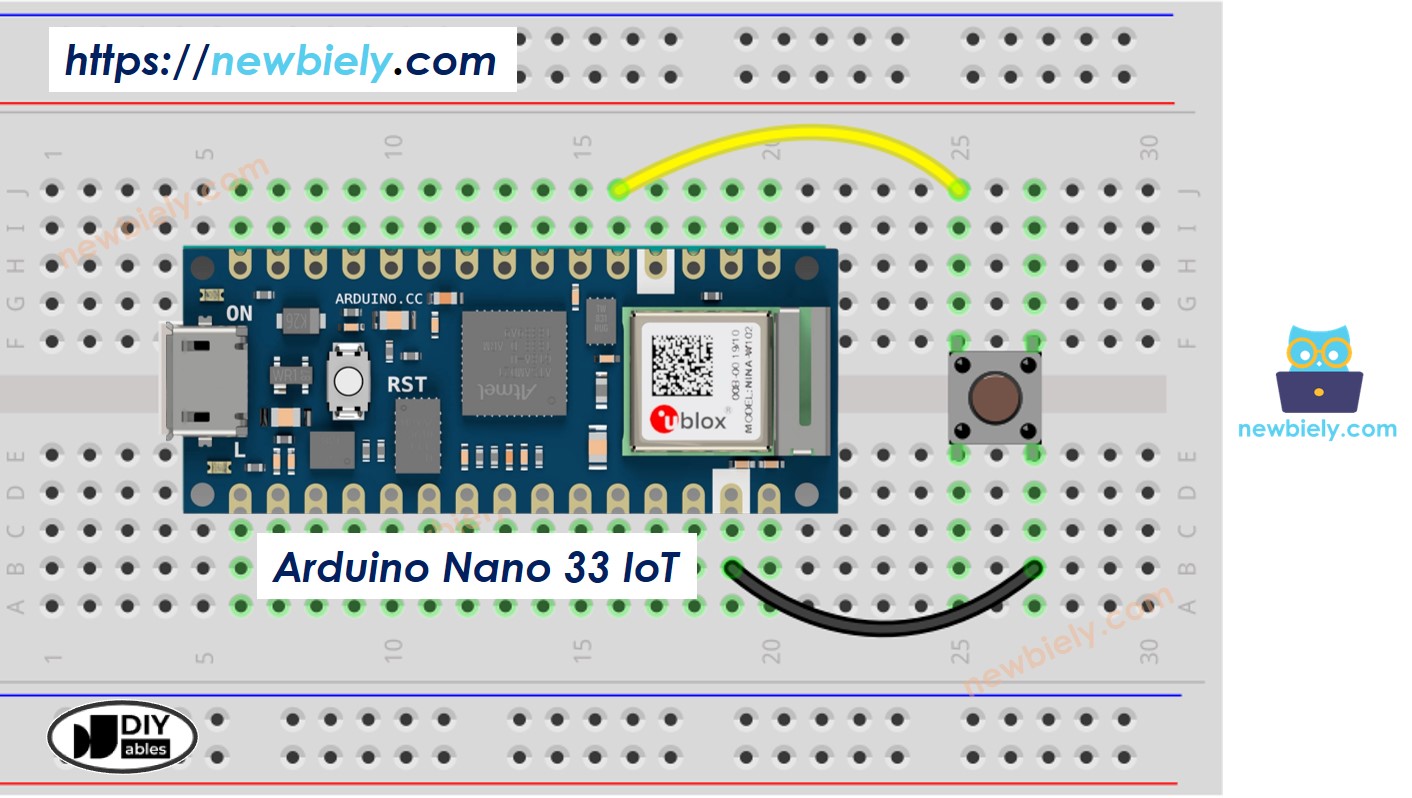
This image is created using Fritzing. Click to enlarge image
To explain clearly, let's run the Arduino Nano 33 IoT code once without debounce and once with debounce, and then compare what happens.
If you are new to the Arduino Nano 33 IoT, be sure to check out our Getting Started with Arduino Nano 33 IoT tutorial. Then, follow these steps:
Connect the components to the Arduino Nano 33 IoT board as depicted in the diagram.
Use a USB cable to connect the Arduino Nano 33 IoT board to your computer.
Launch the Arduino IDE on your computer.
Select the Arduino Nano 33 IoT board and choose its corresponding COM port.
Copy the code below and paste it into the Arduino IDE.
#define BUTTON_PIN 2
int prev_state = LOW;
int button_state;
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP);
}
void loop() {
button_state = digitalRead(BUTTON_PIN);
if (prev_state == HIGH && button_state == LOW)
Serial.println("The button is pressed");
else if (prev_state == LOW && button_state == HIGH)
Serial.println("The button is released");
prev_state = button_state;
}
Press the button and hold it for a few seconds, then let it go.
Look at the Serial Monitor to see the result. It should look like the image below.
The button is pressed
The button is pressed
The button is pressed
The button is released
The button is released
You pressed and released the button one time, but the Arduino Nano 33 IoT detected several presses and releases.
※ NOTE THAT:
Chattering doesn't happen every time. If you don't see it, please try the test a few times.
#define BUTTON_PIN 2
#define DEBOUNCE_TIME 50
int prev_state_steady = LOW;
int prev_state_flick = LOW;
int button_state;
unsigned long last_debounce_time = 0;
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP);
}
void loop() {
button_state = digitalRead(BUTTON_PIN);
if (button_state != prev_state_flick) {
last_debounce_time = millis();
prev_state_flick = button_state;
}
if ((millis() - last_debounce_time) > DEBOUNCE_TIME) {
if(prev_state_steady == HIGH && button_state == LOW)
Serial.println("The button is pressed");
else if(prev_state_steady == LOW && button_state == HIGH)
Serial.println("The button is released");
prev_state_steady = button_state;
}
}
Click the Upload button in the Arduino IDE to compile and send the code to the Arduino Nano 33 IoT board.
Open the Serial Monitor in the Arduino IDE.
Press and hold the button for a few seconds, then let it go.
Look at the result in the Serial Monitor. It will show something similar to the image below.
The button is pressed
The button is released
You can see that you pressed and released the button only once, and the Arduino Nano 33 IoT detected just that one press. The extra signals have been removed.
To help beginners, especially when working with several buttons that might send extra signals, we created a simple library called ezButton. You can learn more about the ezButton library here: https://arduinogetstarted.com/tutorials/arduino-button-library
#include <ezButton.h>
#define DEBOUNCE_TIME 50
ezButton button(D2);
void setup() {
Serial.begin(9600);
button.setDebounceTime(DEBOUNCE_TIME);
}
void loop() {
button.loop();
if (button.isPressed())
Serial.println("The button is pressed");
if (button.isReleased())
Serial.println("The button is released");
}
Let's write code that stops three buttons from being clicked too many times in a row.
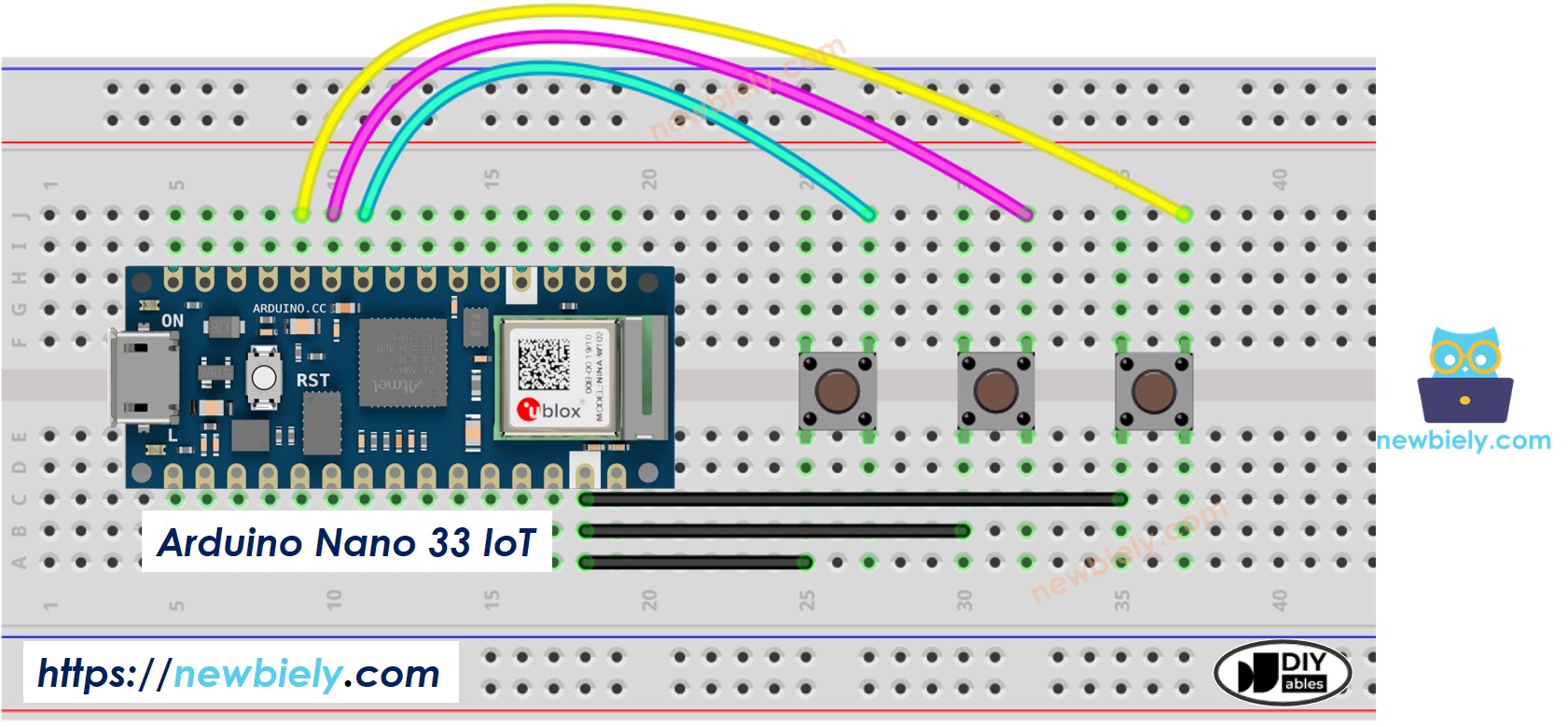
This image is created using Fritzing. Click to enlarge image
#include <ezButton.h>
#define DEBOUNCE_TIME 50
ezButton button1(D6);
ezButton button2(D7);
ezButton button3(D8);
void setup() {
Serial.begin(9600);
button1.setDebounceTime(DEBOUNCE_TIME);
button2.setDebounceTime(DEBOUNCE_TIME);
button3.setDebounceTime(DEBOUNCE_TIME);
}
void loop() {
button1.loop();
button2.loop();
button3.loop();
if (button1.isPressed())
Serial.println("The button 1 is pressed");
if (button1.isReleased())
Serial.println("The button 1 is released");
if (button2.isPressed())
Serial.println("The button 2 is pressed");
if (button2.isReleased())
Serial.println("The button 2 is released");
if (button3.isPressed())
Serial.println("The button 3 is pressed");
if (button3.isReleased())
Serial.println("The button 3 is released");
}
※ NOTE THAT:
Please note that the Arduino Nano 33 IoT pins A4 and A5 have built-in pull-up resistors for I2C communication. Although these pins can be used as digital input pins, it is recommended to avoid using them for digital input. If you must use them, do NOT use internal or external pull-down resistors for these pins
The DEBOUNCE_TIME depends on the device. Different devices might need different values.
Debounce should also work for switches like on/off, limit, reed, and touch sensors.