Arduino Nano 33 IoT - TM1637 4-Digit 7-Segment Display
This guide shows you how to use the Arduino Nano 33 IoT with the TM1637 4-digit 7-segment display module. It explains these topics:
- Wiring the 4-digit 7-segment display to the Arduino Nano 33 IoT board.
- Writing a program for the Arduino Nano 33 IoT to show information on the 4-digit 7-segment display.
In this guide, we will use a 4-digit 7-segment display module with a colon in the middle. If you want to show numbers with decimals, please check the 74HC595 4-digit 7-segment Display Module tutorial.
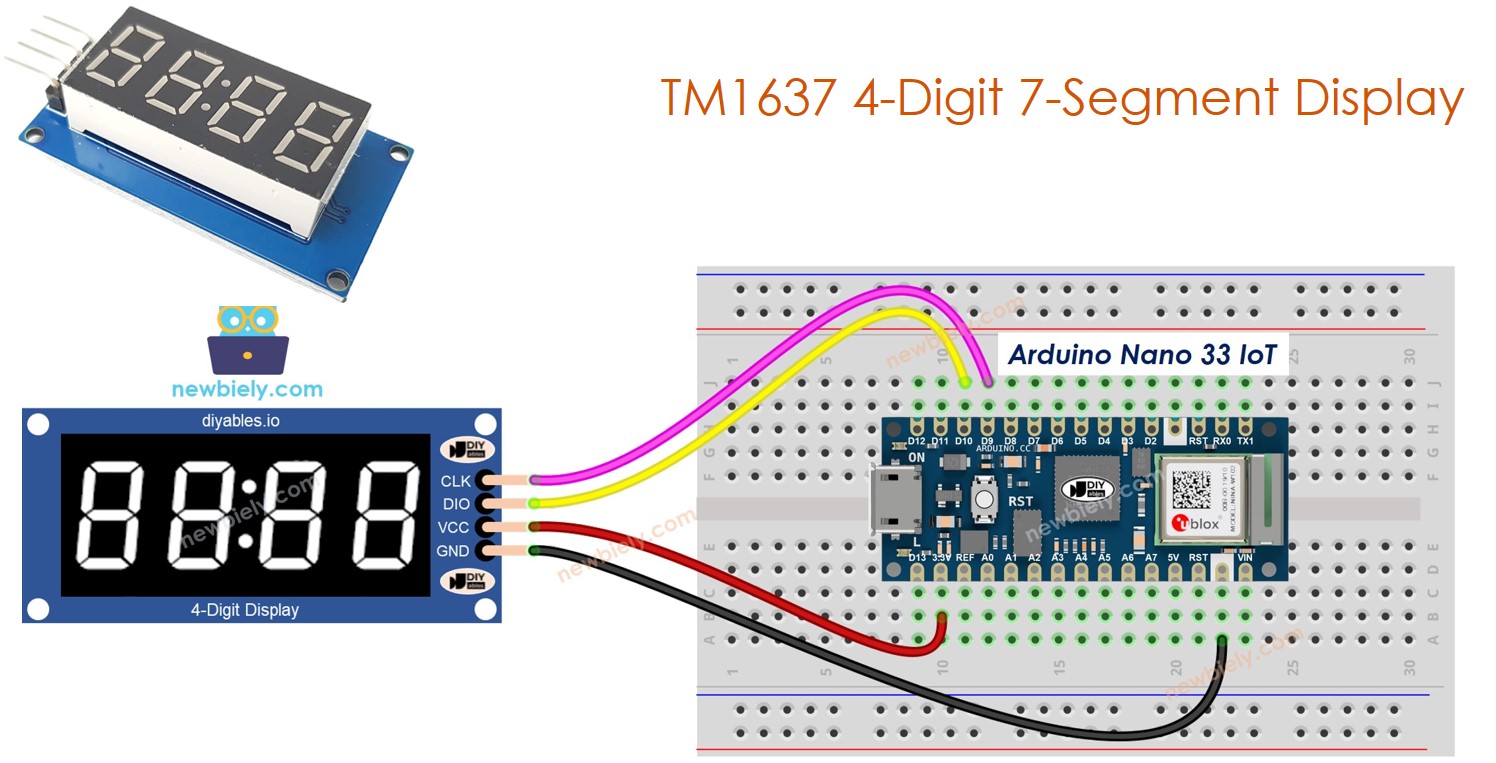
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of TM1637 4-digit 7-segment Display
A 4-digit 7-segment display is often used in clocks, timers, counters, and even to show temperature. Normally, you need 12 wires to connect it. The TM1637 module makes things simpler by only requiring 4 wires: 2 for power and 2 for controlling the display.
A TM1637 module usually has four seven-segment LED displays and one of these options:
- A colon-shaped LED in the center: This is great for showing time in hours and minutes, minutes and seconds, or the scores of two teams.
- Four dot-shaped LEDs for each number: These work well for showing temperatures or any number with decimal points.
The TM1637 4-Digit 7-Segment Display Pinout
The TM1637 module with a display that shows four digits has four pins:
- CLK pin: This is a clock input pin. Connect it to any digital pin on the Arduino Nano 33 IoT.
- DIO pin: This is a data input/output pin. Connect it to any digital pin on the Arduino Nano 33 IoT.
- VCC pin: This pin supplies power to the module. Connect it to a 3.3V to 5V power source.
- GND pin: This is a ground pin. Connect it to the ground on the Arduino Nano 33 IoT.
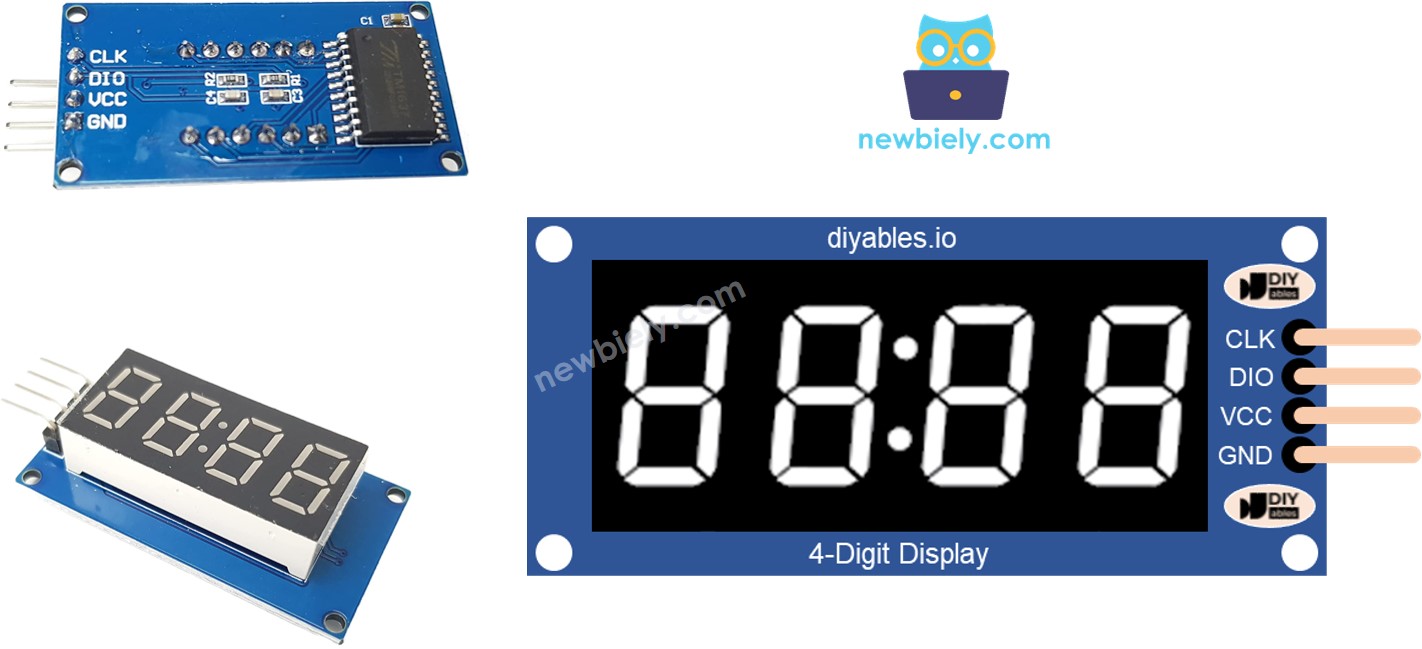
Wiring Diagram
To connect a TM1637 to an Arduino Nano 33 IoT, you need four wires—two for power and two to control the display. You can power the module using the Arduino's 5V output. Connect the CLK and DIO pins to any digital pins on the Arduino, for example, pins 2 and 3. If you use different pins, change the pin numbers in your code.
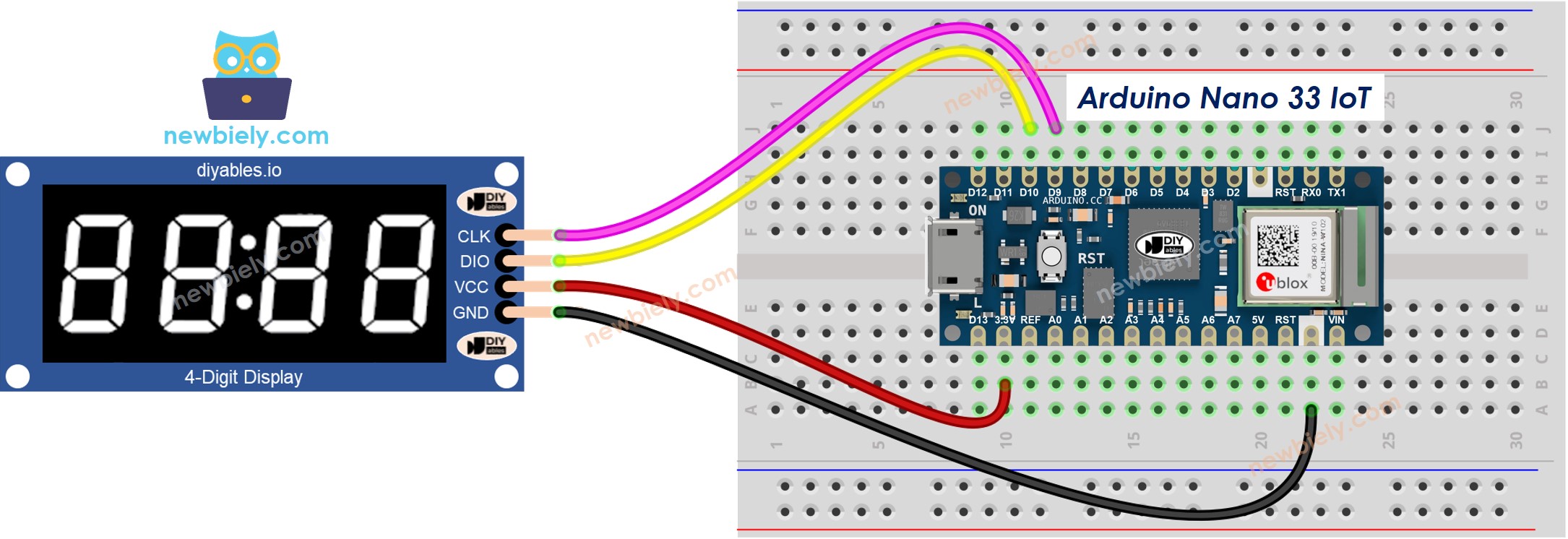
This image is created using Fritzing. Click to enlarge image
Library Installation
To easily program a TM1637 4-digit 7-segment Display, you need to install the TM1637 Display library by Avishay Orpaz. Follow the steps below to install the library.
- Open the Library Manager by clicking the Library Manager icon on the left side of the Arduino IDE.
- Type TM1637 in the search box, then select the TM1637Display library by Avishay Orpaz.
- Click the Install button.
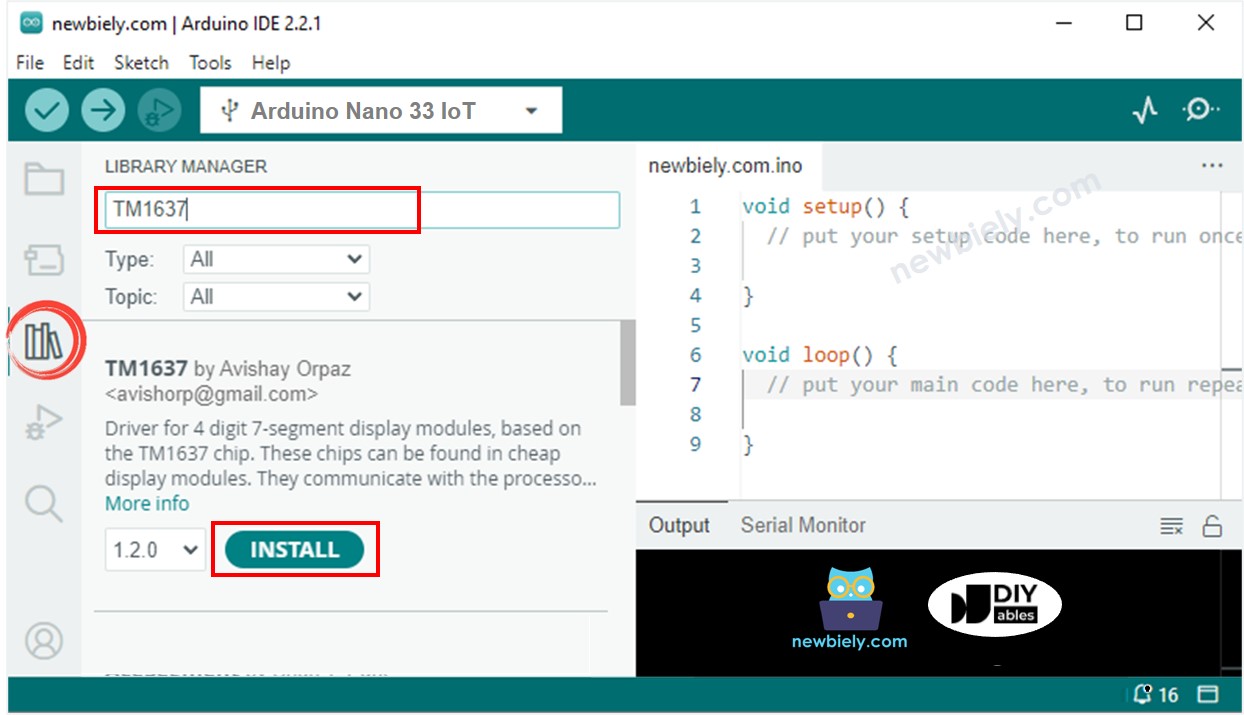
How To Program For TM1637 4-digit 7-segment using Arduino Nano 33 IoT
- Add the library
- Choose the Arduino Nano 33 IoT pins that connect to the display module’s CLK and DIO. For example, you might use pins D9 and D10.
- Make a new TM1637Display device.
- Then you can show numbers, numbers with decimals, numbers with minus signs, or letters. For letters, you must say which style of letters to use. Let's look at each type one by one.
- Showing numbers: look at the examples below. In the description, "_" represents a digit that is not actually shown.
- Display the number using a colon or a dot:
At the end of this guide, you'll find more details about the functions.
Arduino Nano 33 IoT Code
Detailed Instructions
If you are new to the Arduino Nano 33 IoT, be sure to check out our Getting Started with Arduino Nano 33 IoT tutorial. Then, follow these steps:
- Connect the components to the Arduino Nano 33 IoT board as depicted in the diagram.
- Use a USB cable to connect the Arduino Nano 33 IoT board to your computer.
- Launch the Arduino IDE on your computer.
- Select the Arduino Nano 33 IoT board and choose its corresponding COM port.
- Copy the code above and paste it into the Arduino IDE.
- Compile and send the code to your Arduino Nano 33 IoT board by clicking the Upload button in the Arduino IDE.
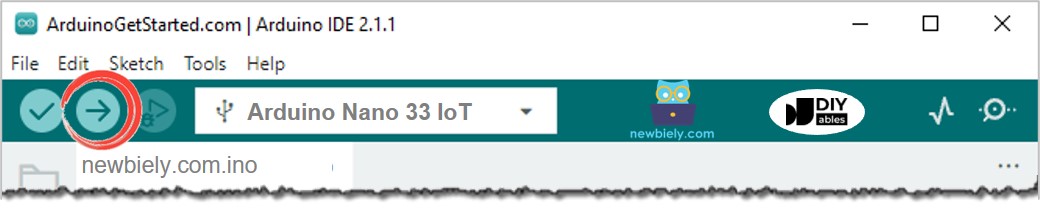
- Take a look at the different ways the 7-segment display lights up.
Video Tutorial
Function References
Here are the sources for:
- Display.clear(): Clear the display.
- Display.showNumberDec(): Show a decimal number on the display.
- Display.showNumberDecEx(): Show a decimal number on the display with extra options.
- Display.setSegments(): Change the segments on the display.
- Display.setBrightness(): Adjust the brightness of the display.
display.clear()
Description
This function clears the screen. It turns off all the LED lights.
display.showNumberDec()
Description
The 7-segment display shows a number in base ten. This function does that job.
Syntax
Parameter
- Num is the number that appears on the 7-segment display, and it can be any value between -9999 and 9999.
- Leading_zero is an optional setting (it is false by default) that decides whether extra zeros are shown at the beginning of the number.
- Length is another optional setting with a default of 4, which decides how many digits are displayed.
- Pos is also an optional setting (default is 0) that determines where the most important digit (the leftmost digit) is placed.
Please note that the function won't show anything if the number is not in the allowed range or if the length is more than 4.
showNumberDecEx()
Description
This function is a more advanced version of showNumberDec() that gives you extra control when showing a decimal number on a 7-segment display. It lets you manage the dot or colon parts of each digit separately.
Syntax
Parameter
- Num1: This is the number that will show on the 7-segment display. It must be a value between -9999 and 9999.
- Dots: This setting tells which dot parts of the display should light up. Each bit in the value represents one digit on the display. Possible values are:
- 0b10000000 for the first dot (0.000)
- 0b01000000 for the second dot (00.00) or the colon (00:00), based on the display type.
- 0b00100000 for the third dot (000.0)
- Leading_zero: This is an extra setting that is false by default. If you set it to true, zeros at the beginning will be shown.
- Length: This is an extra setting that is 4 by default. It tells how many digits are shown on the 7-segment display.
- Pos: This is an extra setting which is 0 by default. It sets the position of the most important digit in the number.
For instance, if you call display.showNumberDecEx(1530, 0b01000000), it will display:
- If the module has a colon LED, the 7-segment display shows 15:30.
- If the module has dot LEDs, the 7-segment display shows 15.30.
Please note that the function will not show anything if the number is not within the allowed range or if the length is more than 4.
setSegments()
Description
This function lets you choose directly which parts of a 7-segment display light up. You can use it to show letters, symbols, or turn off all the LED segments.
Syntax
Parameter
- Segments: This parameter sets the parts of the 7-segment display. It is an array of bytes, where each byte represents one digit and each bit in that byte shows a specific segment.
- Length: This optional parameter has a default value of 4. It tells how many digits to show on the 7-segment display.
- Pos: This optional parameter has a default value of 0. It tells where the most important digit (the one on the left) is located.
This function is useful when you want to display letters or symbols that are not shown on a regular 7-segment display. You can create any design you want by controlling the segments directly.
Please note that the function won’t show anything if the number is not in the allowed range or if the length is more than 4.
setBrightness()
Description
You can change how bright the seven-segment display is using this function.
Syntax
Parameter
- Brightness: This setting changes how bright the 7-segment display is. The number must be between 0 and 7, and a larger number makes the display brighter.
- On: This option, which is true by default, turns the display on or off. If it is set to false, the display will be turned off.