Arduino Nano 33 IoT - GPS
This guide will show you how to get GPS location details like longitude, latitude, and altitude, as well as speed in kilometers per hour, from the NEO-6M GPS module. We will also explain how to calculate the distance between your current location and a set GPS point, such as the location of London.
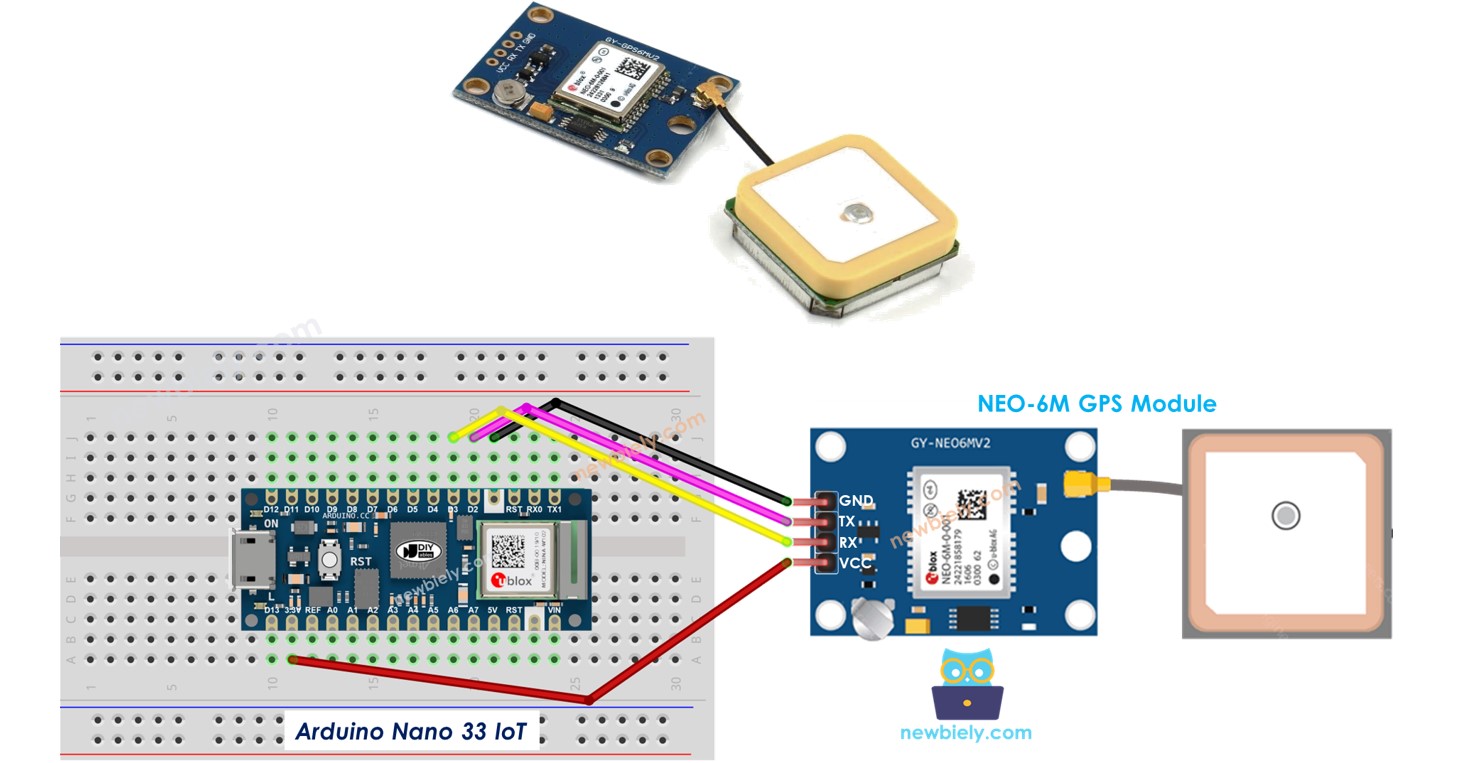
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of NEO-6M GPS module
Pinout
The NEO-6M GPS module comes with four pins.
- VCC pin: This pin must be connected to the power supply (either 3.3V or 5V).
- GND pin: This pin must be connected to ground (0V).
- TX pin: This pin sends data from the GPS module to the Arduino Nano 33 IoT. It must be connected to the Serial RX pin on the Arduino Nano 33 IoT.
- RX pin: This pin receives data from the Arduino Nano 33 IoT to the GPS module. It must be connected to the Serial TX pin on the Arduino Nano 33 IoT.
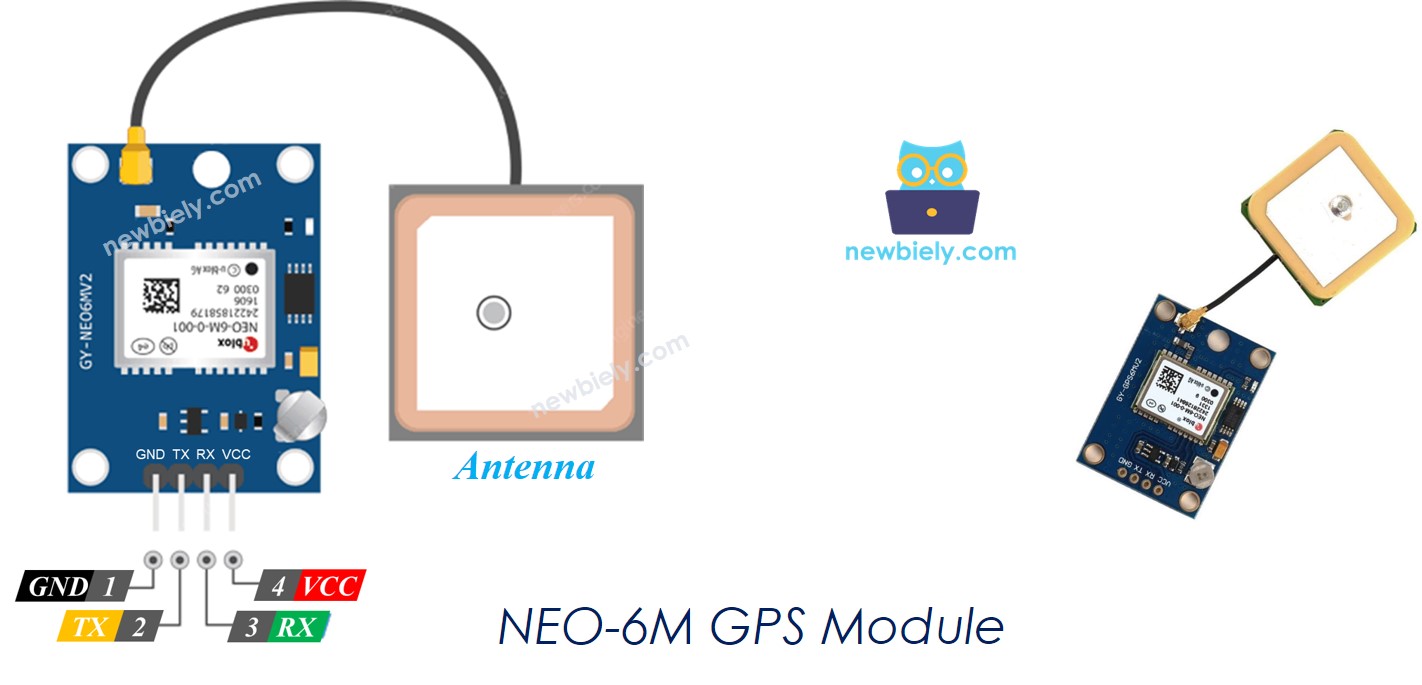
Wiring Diagram
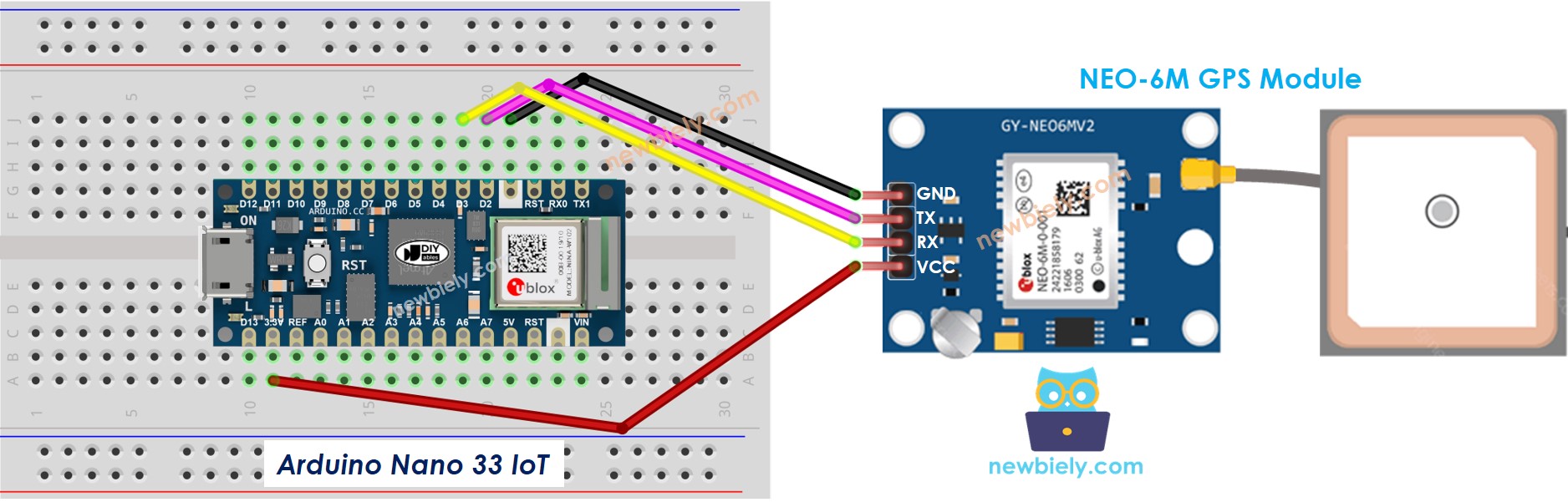
This image is created using Fritzing. Click to enlarge image
Arduino Nano 33 IoT Code
Reading GPS coordinates, speed (km/h), and date time
Detailed Instructions
If you are new to the Arduino Nano 33 IoT, be sure to check out our Getting Started with Arduino Nano 33 IoT tutorial. Then, follow these steps:
- Connect the components to the Arduino Nano 33 IoT board as depicted in the diagram.
- Use a USB cable to connect the Arduino Nano 33 IoT board to your computer.
- Launch the Arduino IDE on your computer.
- Select the Arduino Nano 33 IoT board and choose its corresponding COM port.
- Click the Libraries icon on the left side of the Arduino IDE.
- Type TinyGPSPlus in the search box and then choose the TinyGPSPlus library by Mikal Hart.
- Click the Install button to add the TinyGPSPlus library.
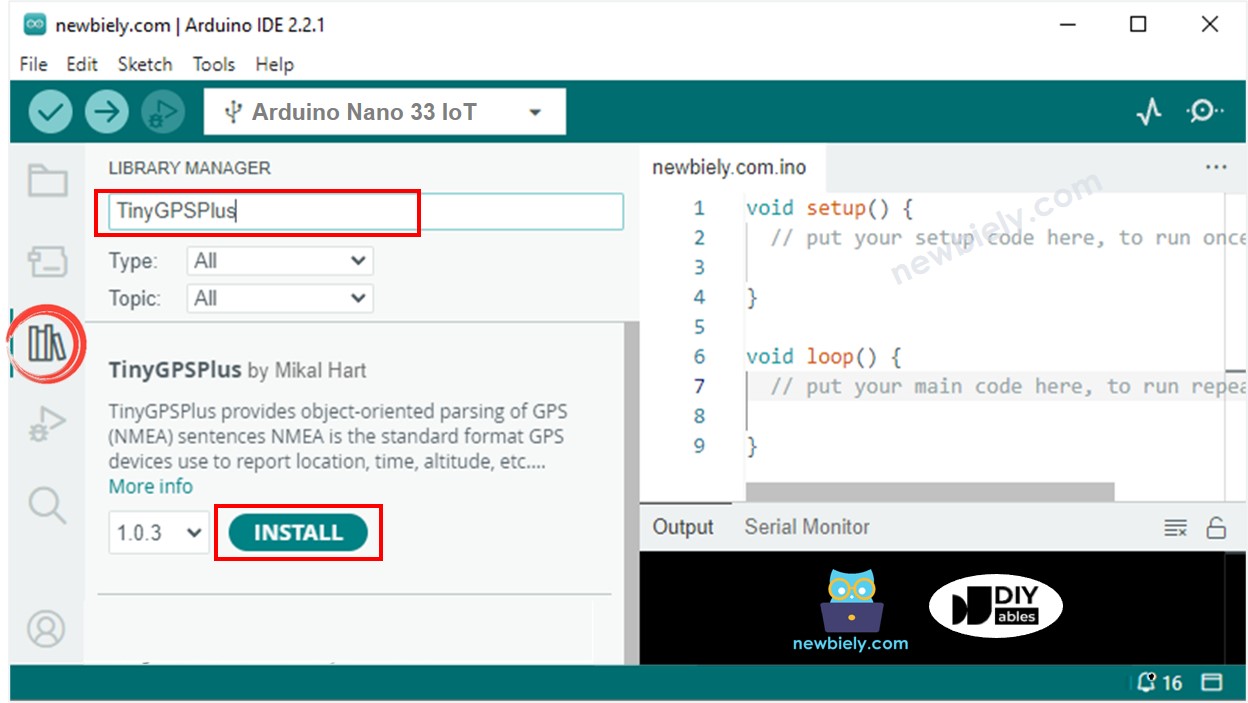
- Copy the code above and open it in the Arduino IDE. Press the Upload button to send the code to your Arduino Nano 33 IoT. Then, check the Serial Monitor to see the result.
Calculating the distance from current location to a predefined location
The code below calculates the distance from your current location to London (latitude: 51.508131, longitude: -0.128002).
Detailed Instructions
- Copy the code above and open it in the Arduino IDE.
- Click the Upload button in the Arduino IDE to send the code to the Arduino Nano 33 IoT.
- Check the results on the Serial Monitor.