Arduino Nano 33 IoT - Serial Monitor
This guide shows you how to use the Serial Monitor in the Arduino IDE with the Arduino Nano 33 IoT.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Serial Monitor
When programming an Arduino Nano 33 IoT, you need a tool to see how your code is working. Use the Serial Monitor in the Arduino IDE. This tool is made for two things:
- Arduino Nano 33 IoT to PC: Your Arduino Nano 33 IoT sends information using a Serial connection. The PC's Serial Monitor gets this information and shows it on the screen. This feature is very helpful for checking and watching your project.
- PC to Arduino Nano 33 IoT: You can type a message on your PC and send it to the Arduino Nano 33 IoT. This makes it easy to control the Arduino Nano 33 IoT from your computer.
You need a USB cable to connect your computer to the Arduino Nano 33 IoT. This same cable is used to send programs to the Arduino Nano 33 IoT.
How To Use Serial Monitor
Open Serial Monitor
Simply click on the icon in the Arduino IDE shown in the image below:
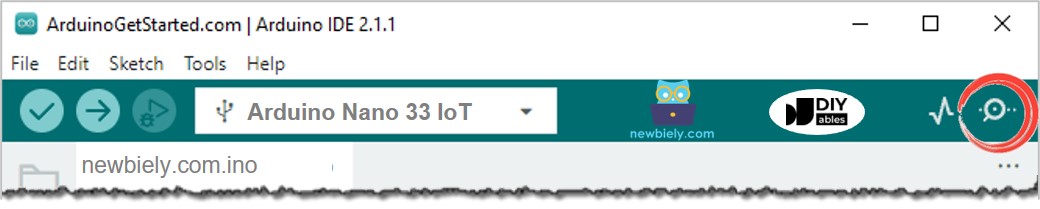
Components on Serial Monitor
The Serial Momitor is made up of 8 parts.
Output console: This part shows the data coming from Arduino Nano 33 IoT.
i. Autoscroll checkbox: This option lets you turn automatic scrolling for the output console on or off.
Timestamp checkbox: This tool lets you add the time before your data.
Clear output button: When you click this button, all the text on the output screen is removed.
Baud rate selection: This part lets you pick the communication speed between your PC and the Arduino Nano 33 IoT. This number must match what is set in the Arduino Nano 33 IoT code (inside the Serial.begin() function).
Textbox: This part lets you type characters. When you click the Send button, those characters will be sent to the Arduino Nano 33 IoT board.
- Ending Selection: This part lets you choose what ending characters to add to the data you send to the Arduino Nano 33 IoT. The options are:
- No ending: doesn’t add anything
- Newline: adds a newline (LF, or '\n')
- Carriage Return: adds a carriage return (CR, or '\r')
- Both NL and CR: adds both a newline and a carriage return
Send button: When you click this button, the Serial Monitor sends the text from the Textbox along with the ending characters to the Arduino Nano 33 IoT.
Arduino Nano 33 IoT To PC
How to send information from the Arduino Nano 33 IoT board to a computer:
- Set the communication speed and start the Serial port with the Serial.begin() function.
- To send info to the Serial Monitor, use one of these functions:
- Serial.print() – https://arduinogetstarted.com/reference/serial-print
- Serial.println() – https://arduinogetstarted.com/reference/serial-println
- Serial.write() – https://arduinogetstarted.com/reference/serial-write
For example, type "Hello World!" into the Serial Monitor.
Example Use
Below is a sample code that sends the text "newbiely.com" from an Arduino Nano 33 IoT to the Serial Monitor every second.
Detailed Instructions
If you are new to the Arduino Nano 33 IoT, be sure to check out our Getting Started with Arduino Nano 33 IoT tutorial. Then, follow these steps:
- Connect the components to the Arduino Nano 33 IoT board as depicted in the diagram.
- Use a USB cable to connect the Arduino Nano 33 IoT board to your computer.
- Launch the Arduino IDE on your computer.
- Select the Arduino Nano 33 IoT board and choose its corresponding COM port.
- Copy the code above and paste it into the Arduino IDE.
- Click the Upload button in the Arduino IDE to compile and send the code to the Arduino Nano 33 IoT board.
- Open the Serial Monitor tool in the Arduino IDE.
- Choose the 9600 baud rate.
- Watch the output appear in the Serial Monitor.
- Try using Serial.print() instead of Serial.println().
PC To Arduino Nano 33 IoT
How to send data from PC to Arduino Nano 33 IoT
- On your computer:
- Write your message in the Serial Monitor.
- Click the "Send" button.
Next, you write the Arduino Nano 33 IoT code to collect and process the data.
- Set the communication speed and start the serial connection.
- See if the new data is there.
- Get information from the Serial port by using one of these functions:
- Serial.read()
- Serial.readBytes()
- Serial.readBytesUntil()
- Serial.readString()
- Serial.readStringUntil()
For instance:
Example Use
This Arduino Nano 33 IoT sample code listens to commands from the Serial port to turn the built-in LED on or off.
- If the text command is "ON", light up the LED.
- If the text command is "OFF", turn off the LED.
How does Arduino Nano 33 IoT know which command it received? For example, if we send the word "OFF," how can it tell if the command is "O," "OF," or "OFF"?
We need to add an ending character when sending a command. You can attach a newline character ('\n') to your message. To do this, choose the "newline" option on the Serial Monitor before you send your data. The Arduino Nano 33 IoT will keep reading until it finds the newline, which acts as the ending signal or separator.
Detailed Instructions
- Copy the code shown above and paste it into the Arduino IDE.
- Click the Upload button in the Arduino IDE to compile and send the code to the Arduino Nano 33 IoT board.
- Open the Serial Monitor in the Arduino IDE.
- Choose a baud rate of 9600 and select the newline option.
- Type "ON" or "OFF" and click the Send button.
- Check the built-in light on the Arduino Nano 33 IoT board. It will show if the light is ON or OFF.
- You can also see the light's status on the Serial Monitor.
- Type the command ON or OFF several times.