Arduino Nano 33 IoT - WS2812B LED Strip
In this guide, we will learn how to use the Arduino Nano 33 IoT to control a WS2812B RGB LED strip. We will use just one pin on the Arduino Nano 33 IoT:
- Arduino Nano 33 IoT lets you change the color of each LED on the strip one by one.
- Arduino Nano 33 IoT lets you adjust the brightness of each LED on the strip one by one.
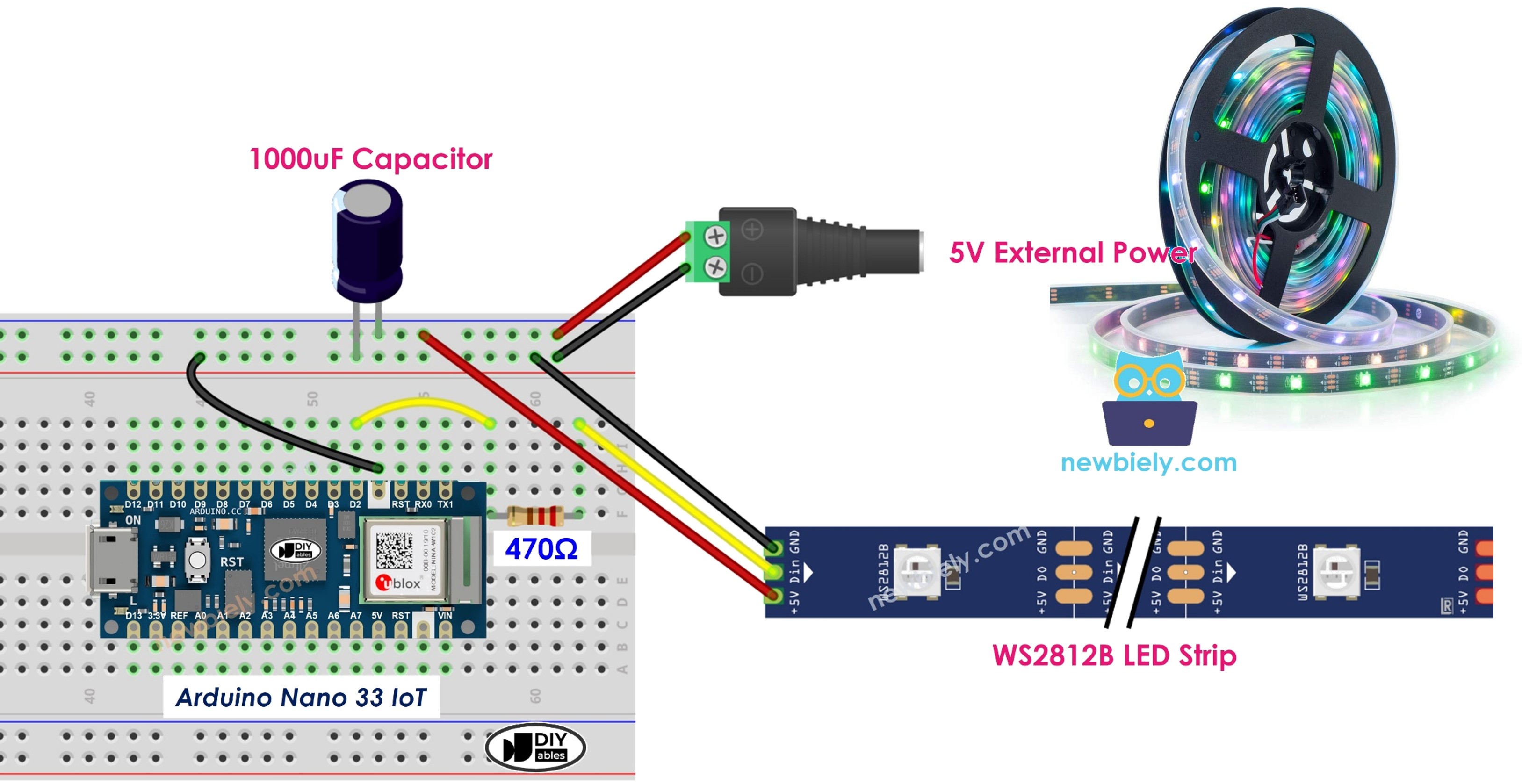
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of WS2812B RGB LED Strip
Pinout
The WS2812B LED strip has three connection points:
- GND pin: Connect this pin to ground (0V).
- VCC pin: Connect this pin to the 5V power supply.
- Din pin: This pin gets the control signal. Connect it to a pin on the Arduino Nano 33 IoT.
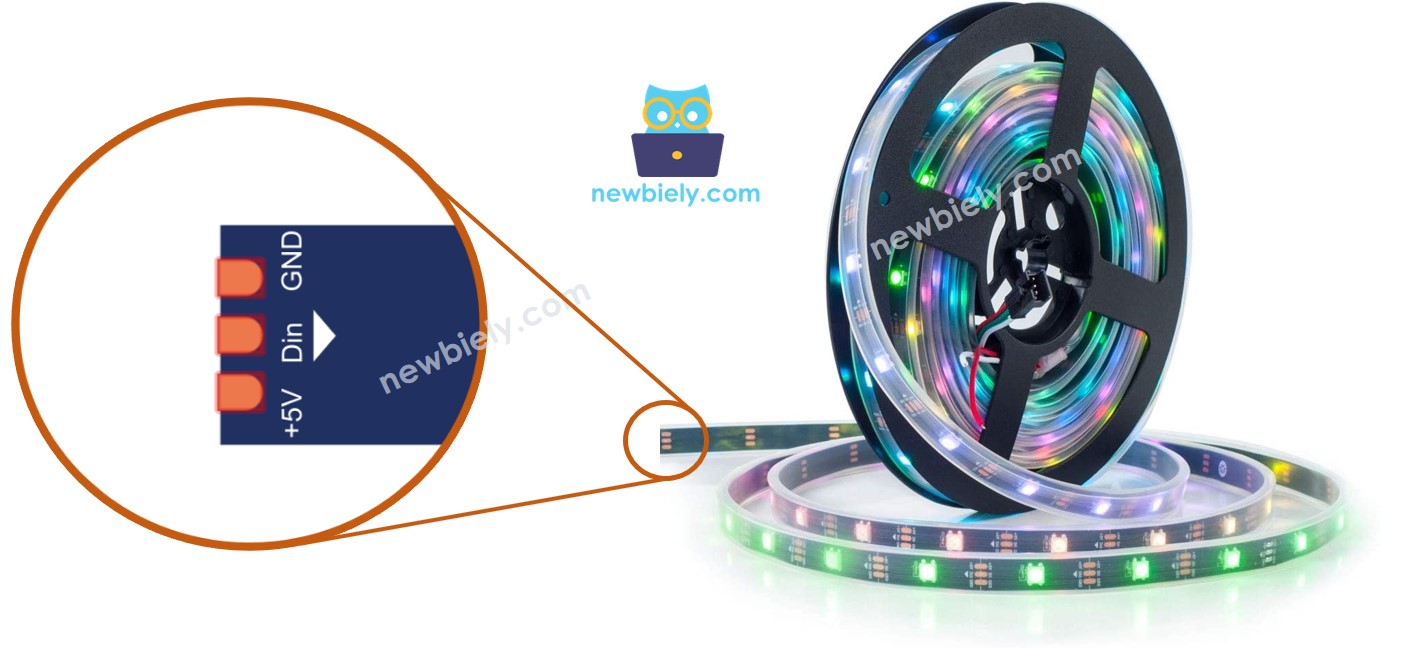
※ NOTE THAT:
Different manufacturers may arrange the pins in different orders. Always follow the labels printed on the LED strip.
Wiring Diagram
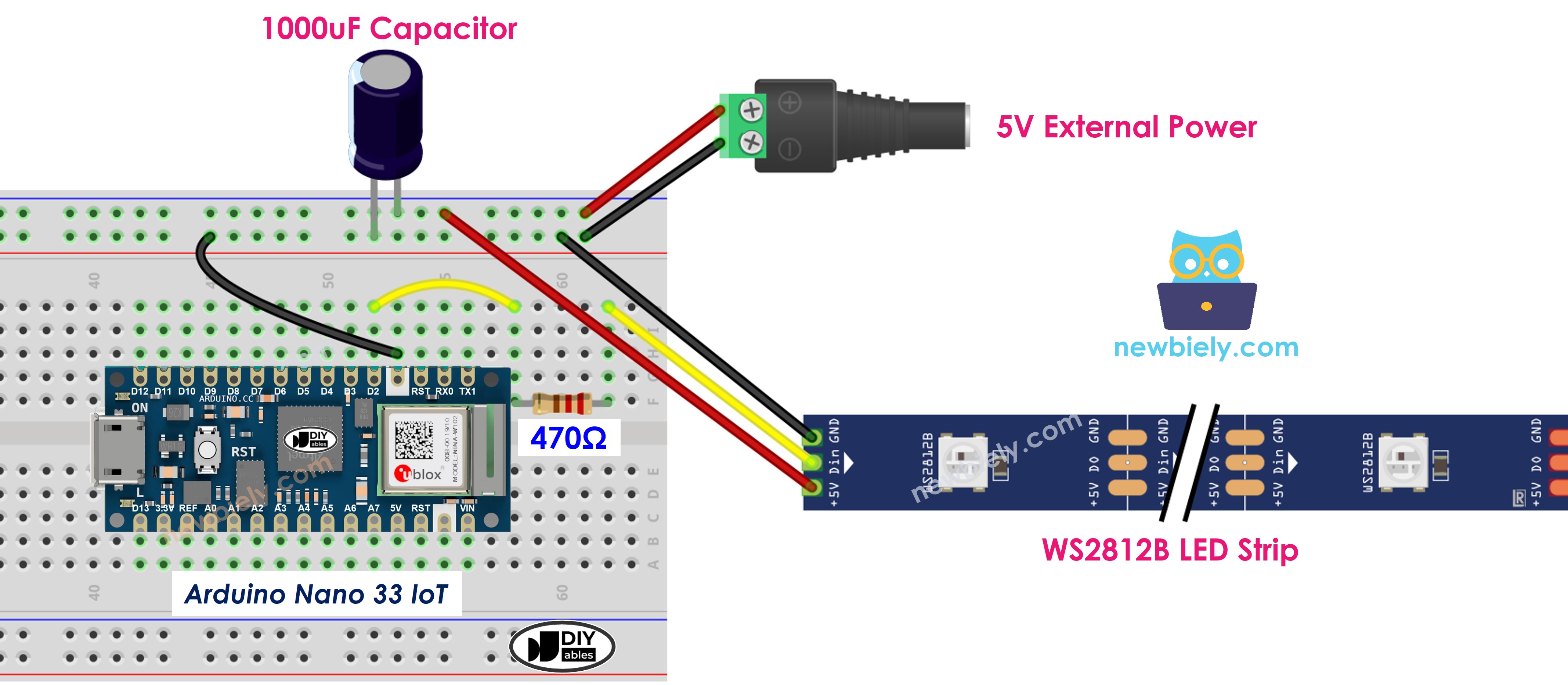
This image is created using Fritzing. Click to enlarge image
How To Program For WS2812B RGB LED Strip
You can use two different libraries to control a WS2812B LED strip:
- Adafruit NeoPixel toolkit for controlling colorful LED lights.
- FastLED toolkit for managing LED strips.
This lesson will use the Adafruit NeoPixel library.
- Create a WS2812B object.
- Starts the WS2812B
- Change the color of each LED light (they are called pixels).
- Change the brightness for the whole strip.
※ NOTE THAT:
- WS2812B.setBrightness() controls the brightness for every light on the LED strip. If you want to change the brightness for each light one by one, you need to adjust its color value.
- The changes made with WS2812B.setBrightness() and WS2812B.setPixelColor() will only be visible after calling WS2812B.show().
Arduino Nano 33 IoT Code
Here's what the code does:
- Change each pixel to green, one at a time, waiting a little bit between each change.
- Switch off all pixels for two seconds.
- Change all pixels to red at the same time for two seconds.
- Keep doing these steps forever.
Detailed Instructions
If you are new to the Arduino Nano 33 IoT, be sure to check out our Getting Started with Arduino Nano 33 IoT tutorial. Then, follow these steps:
- Connect the components to the Arduino Nano 33 IoT board as depicted in the diagram.
- Use a USB cable to connect the Arduino Nano 33 IoT board to your computer.
- Launch the Arduino IDE on your computer.
- Select the Arduino Nano 33 IoT board and choose its corresponding COM port.
- Click the Library Manager icon on the left side of the Arduino IDE.
- In the search box, type Adafruit NeoPixel and look for the WS2812B library by Adafruit.
- Press the Install button to add the NeoPixel library.
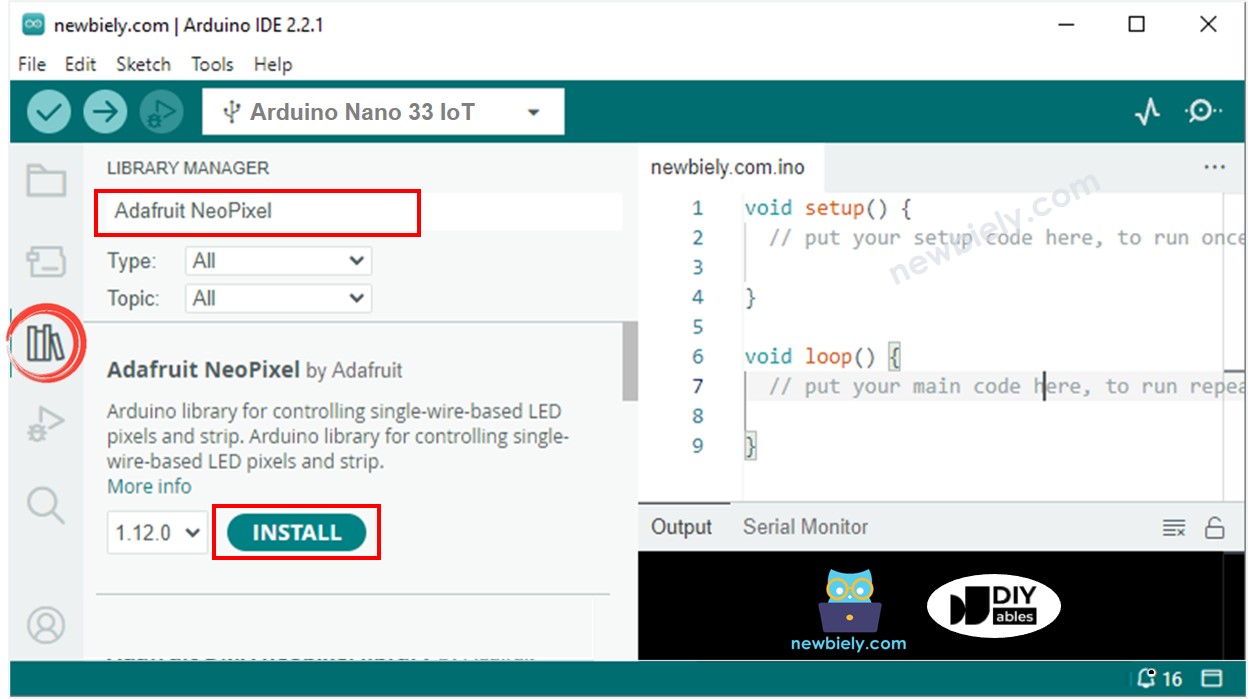
- Copy the code above and open it in the Arduino IDE.
- Click the Upload button in the Arduino IDE to send the code to your Arduino Nano 33 IoT board.
- Watch the LED light show.
※ NOTE THAT:
If you want a complex LED light effect, try our paid programming service.