Arduino Nano 33 IoT - SD Card
This guide will show you how to use a Micro SD Card with the Arduino Nano 33 IoT. We will look at these topics:
- How to open a file on the Micro SD Card with the Arduino Nano 33 IoT, and also create a new file if it does not exist.
- How to write data into a file on the Micro SD Card using the Arduino Nano 33 IoT.
- How to read a file from the Micro SD Card one character at a time with the Arduino Nano 33 IoT.
- How to read a file from the Micro SD Card one line at a time with the Arduino Nano 33 IoT.
- How to add more content to an already existing file on the Micro SD Card using the Arduino Nano 33 IoT.
- How to replace the content inside a file on the Micro SD Card with new content using the Arduino Nano 33 IoT.
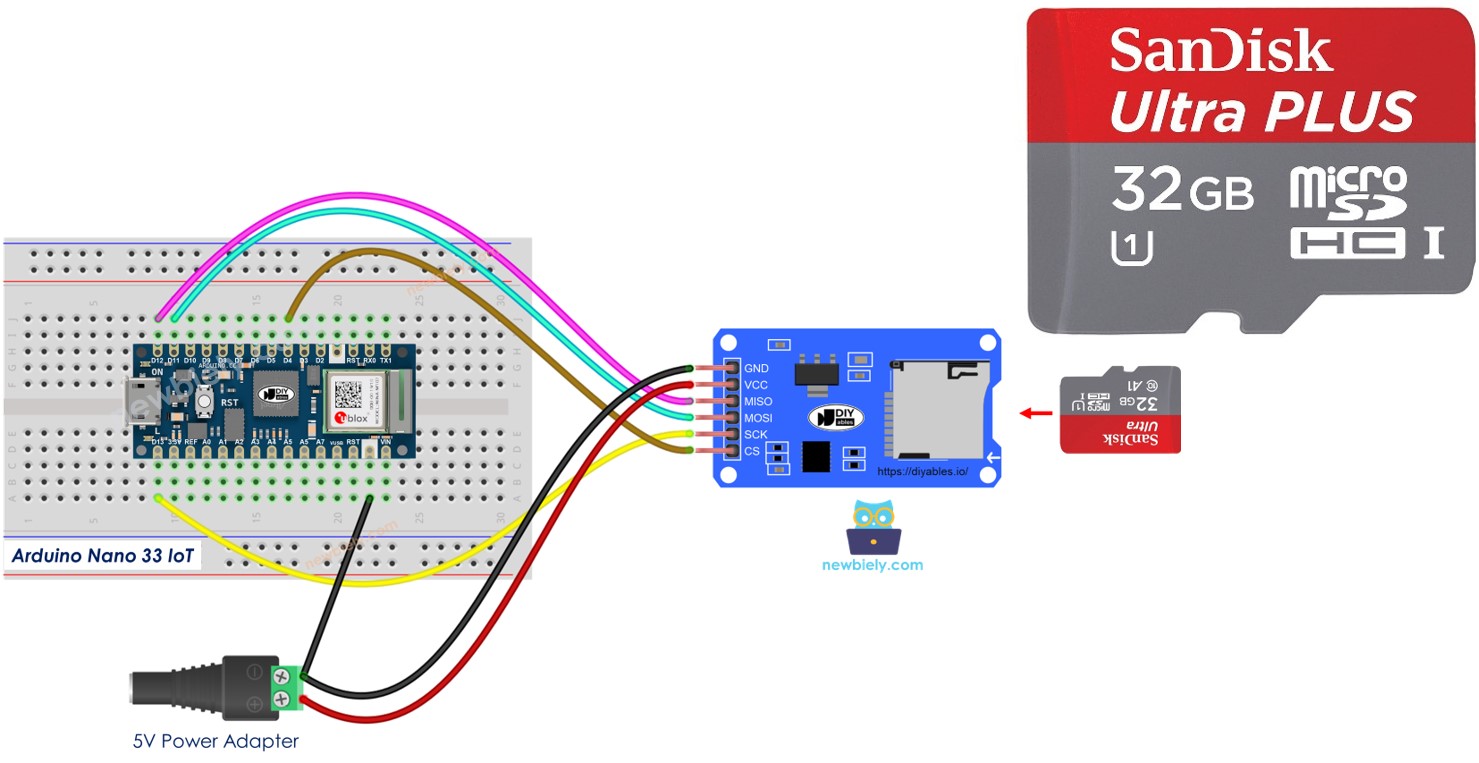
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Micro SD Card Module
The Micro SD Card Module works with the Arduino Nano 33 IoT and is designed to hold a Micro SD Card. Simply put, the module connects the Arduino Nano 33 IoT to the Micro SD Card.
Pinout
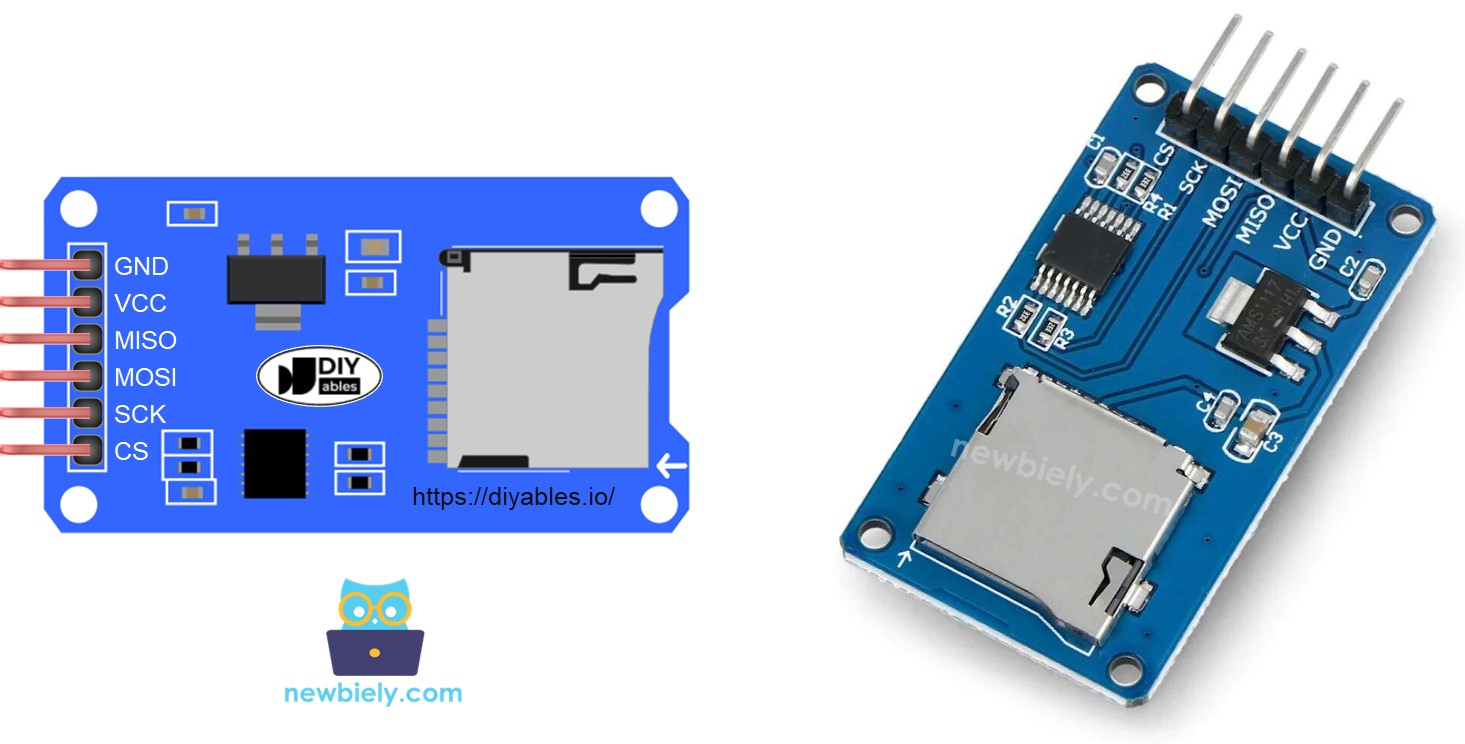
The Micro SD card module comes with six pins.
- VCC pin: connect to the Arduino Nano 33 IoT's 5V pin.
- GND pin: connect to the Arduino Nano 33 IoT's ground (GND) pin.
- MISO pin (Master In Slave Out): connect to the Arduino Nano 33 IoT's MISO pin.
- MOSI pin (Master Out Slave In): connect to the Arduino Nano 33 IoT's MOSI pin.
- SCK pin: connect to the Arduino Nano 33 IoT's SCK pin.
- SS pin (Slave Select): connect to the pin set as SS in the Arduino Nano 33 IoT code.
Preparation
- Plug the micro SD card into your computer using a USB 3.0 SD card reader.
- Make sure the micro SD card is set to FAT16 or FAT32 format (look it up online if you need help).
Wiring Diagram
- This diagram shows how to connect the wires when using the USB port to power the Arduino Nano 33 IoT board.
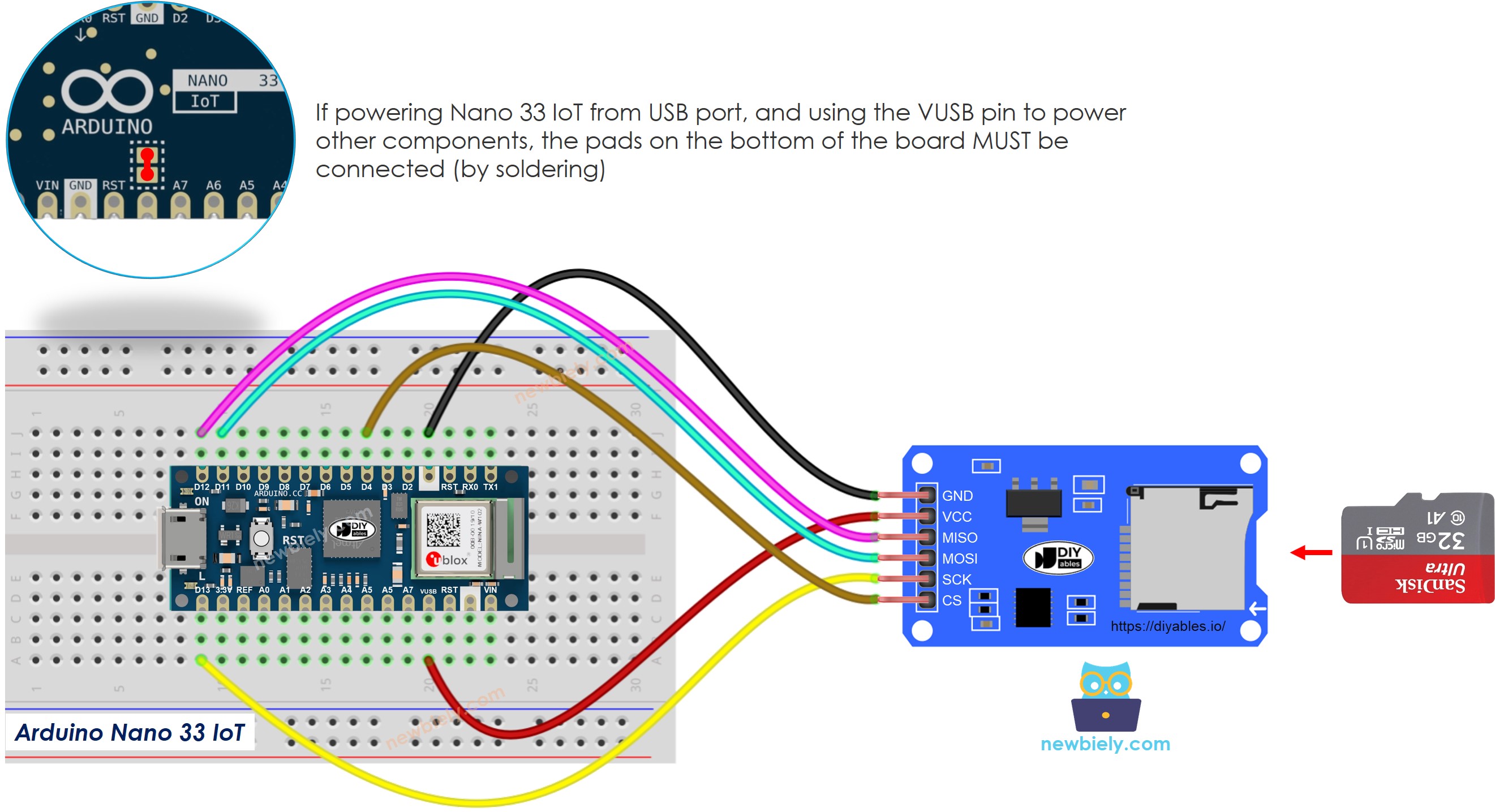
This image is created using Fritzing. Click to enlarge image
- Wiring diagram for powering the Arduino Nano 33 IoT board using the Vin pin.
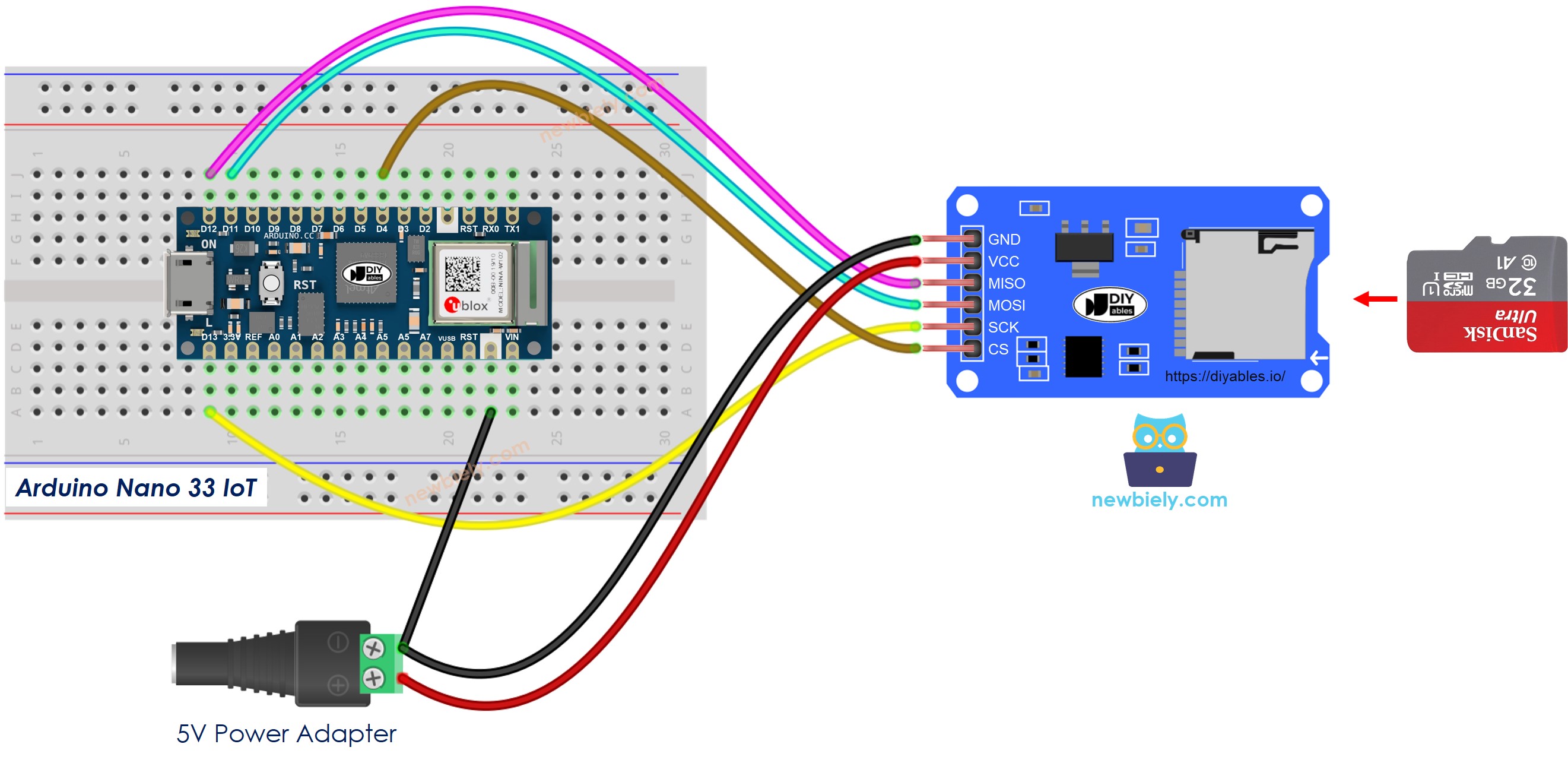
This image is created using Fritzing. Click to enlarge image
Arduino Nano 33 IoT - How to open a file on Micro SD Card and create if not existed
Arduino Nano 33 IoT Code
Detailed Instructions
If you are new to the Arduino Nano 33 IoT, be sure to check out our Getting Started with Arduino Nano 33 IoT tutorial. Then, follow these steps:
- Insert the Micro SD Card into the Micro SD Card module.
- Connect the components to the Arduino Nano 33 IoT board as depicted in the diagram.
- Use a USB cable to connect the Arduino Nano 33 IoT board to your computer.
- Launch the Arduino IDE on your computer.
- Select the Arduino Nano 33 IoT board and choose its corresponding COM port.
- Open the Serial Monitor in the Arduino IDE.
- Copy the code from above and paste it into the Arduino IDE.
- Click the Upload button in the Arduino IDE to send the code to the Arduino Nano 33 IoT.
- Check the Serial Monitor to see the results from the first run.
- The Serial Monitor will display the results in the next tests.
※ NOTE THAT:
You might not see any output on the Serial Monitor the first time because you uploaded your code before opening it.
- Remove the Micro SD Card from the device
- Put the Micro SD Card into a USB SD Card reader
- Plug the USB SD Card reader into your computer
- See if the file is there or not
Arduino Nano 33 IoT - How to write/read data to/from a file on Micro SD Card
Here's what the code does:
- Save information into a file
- Open the file, read it one letter at a time, and show it on the Serial Monitor
- The Serial Monitor shows what is inside the file.
※ NOTE THAT:
By default, data is added to the end of the file. If you restart the Arduino Nano 33 IoT with the code above, the text will be added again, and the Serial Monitor will show extra lines like this:
You can also remove the Micro SD Card from the module and open it on your computer to see the files (you need a USB SD Card reader).
Arduino Nano 33 IoT - How to read a file on Micro SD Card line-by-line
- The answer is shown on the Serial Monitor.
※ NOTE THAT:
If you don't erase the file content first, you might see extra lines on the Serial Monitor.
Arduino Nano 33 IoT - How to overwrite a file on Micro SD Card
Normally, new data is added to the end of the file. The easiest way to replace a file is to remove the old file and create a new one with the same name.
- The result is shown on the Serial Monitor.
- Restart the Arduino Nano 33 IoT.
- Make sure the file's data is added to the Serial Monitor.
You can remove the micro SD card from the module and view it on your computer. You'll need a USB card reader for that.