Arduino Nano 33 IoT - Potentiometer Servo Motor
This guide shows you how to use the Arduino Nano 33 IoT to set the position of a servo motor using a value from a knob. In this lesson, we will learn:
- How to hook up a potentiometer (a type of knob) and a servo motor (a small motor) to your Arduino Nano 33 IoT.
- How to write a simple program for the Arduino Nano 33 IoT that reads the value from the potentiometer and controls the servo motor based on that value.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Servo Motor and Potentiometer
If you're new to using the Servo Motor, Potentiometer, and Arduino Nano 33 IoT, please check out these tutorials:
These tutorials explain how Servo Motor and Potentiometer work, their pinouts, how to connect them to the Arduino Nano 33 IoT, and how to program Arduino Nano 33 IoT to work with the Servo Motor and Potentiometer.
Wiring Diagram
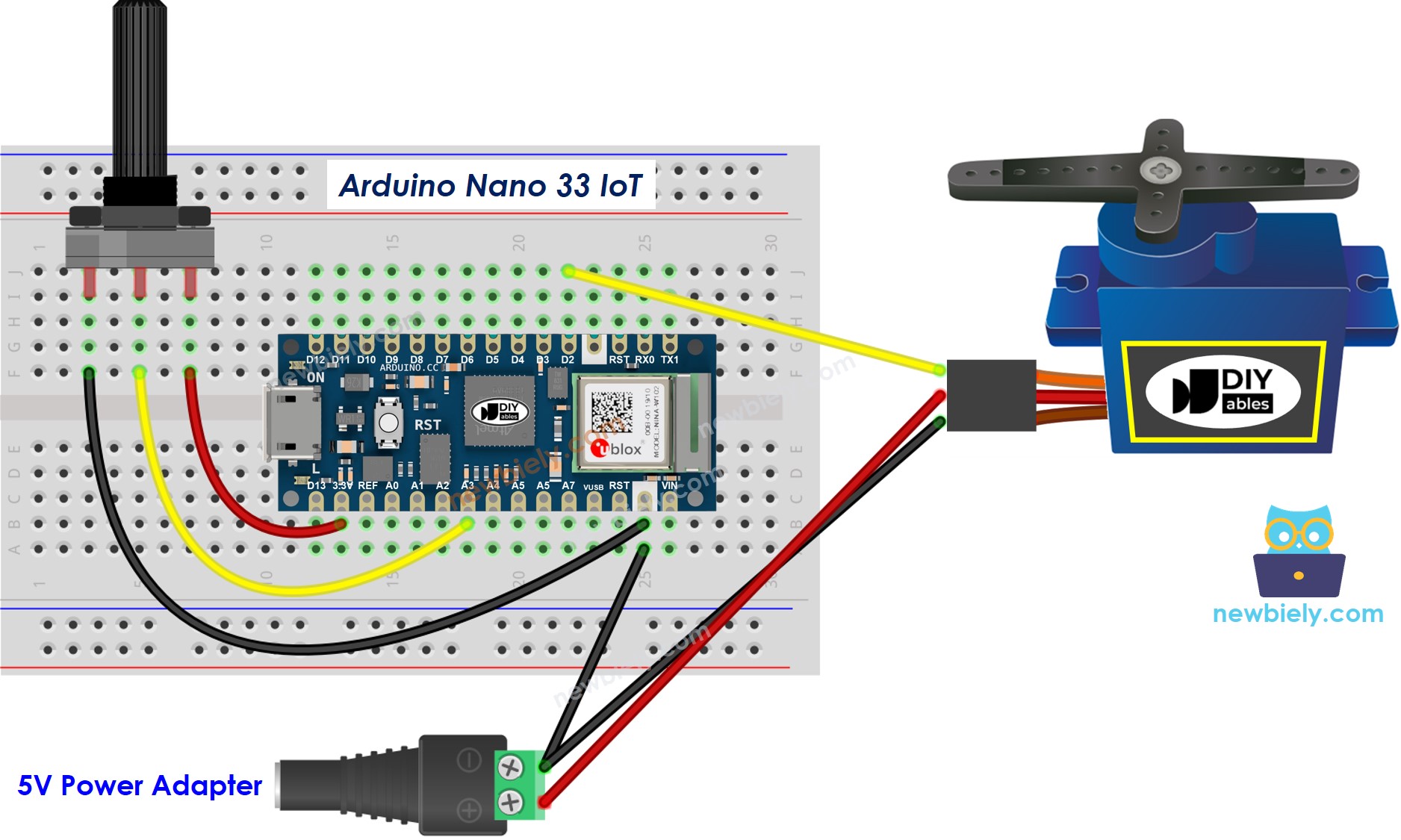
This image is created using Fritzing. Click to enlarge image
※ NOTE THAT:
Please note that the Arduino Nano 33 IoT pins A4 and A5 have built-in pull-up resistors for I2C communication. This can affect analog readings, so it is recommended to avoid using these pins with any devices/sensors that relies on ADC.
How To Program
- Read the value from the potentiometer, which can be any number between 0 and 1023.
- Change it into an angle between 0 and 180.
- Set the servo to the given angle.
Arduino Nano 33 IoT Code
Detailed Instructions
If you are new to the Arduino Nano 33 IoT, be sure to check out our Getting Started with Arduino Nano 33 IoT tutorial. Then, follow these steps:
- Connect the components to the Arduino Nano 33 IoT board as depicted in the diagram.
- Use a USB cable to connect the Arduino Nano 33 IoT board to your computer.
- Launch the Arduino IDE on your computer.
- Select the Arduino Nano 33 IoT board and choose its corresponding COM port.
- Copy the code above and open it in the Arduino IDE.
- Click the Upload button in the Arduino IDE to send the code to the Arduino Nano 33 IoT.
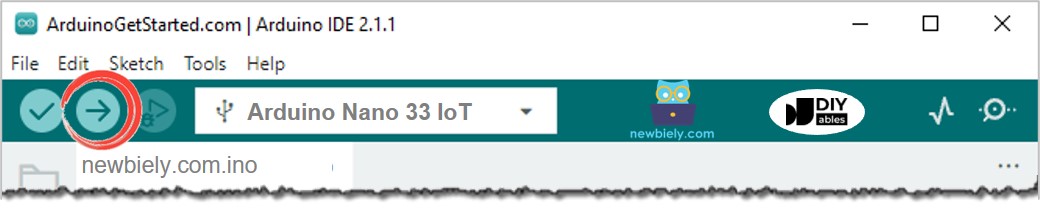
- Open the Serial Monitor
- Rotate the knob on the potentiometer
- Watch the servo motor move
- See the result in the Serial Monitor
Code Explanation
Take a look at the step-by-step explanation in the code comments!