Arduino Nano 33 IoT - Touch Sensor
This guide shows you how to use the Arduino Nano 33 IoT with a touch sensor (which is also called a touch switch or touch button).
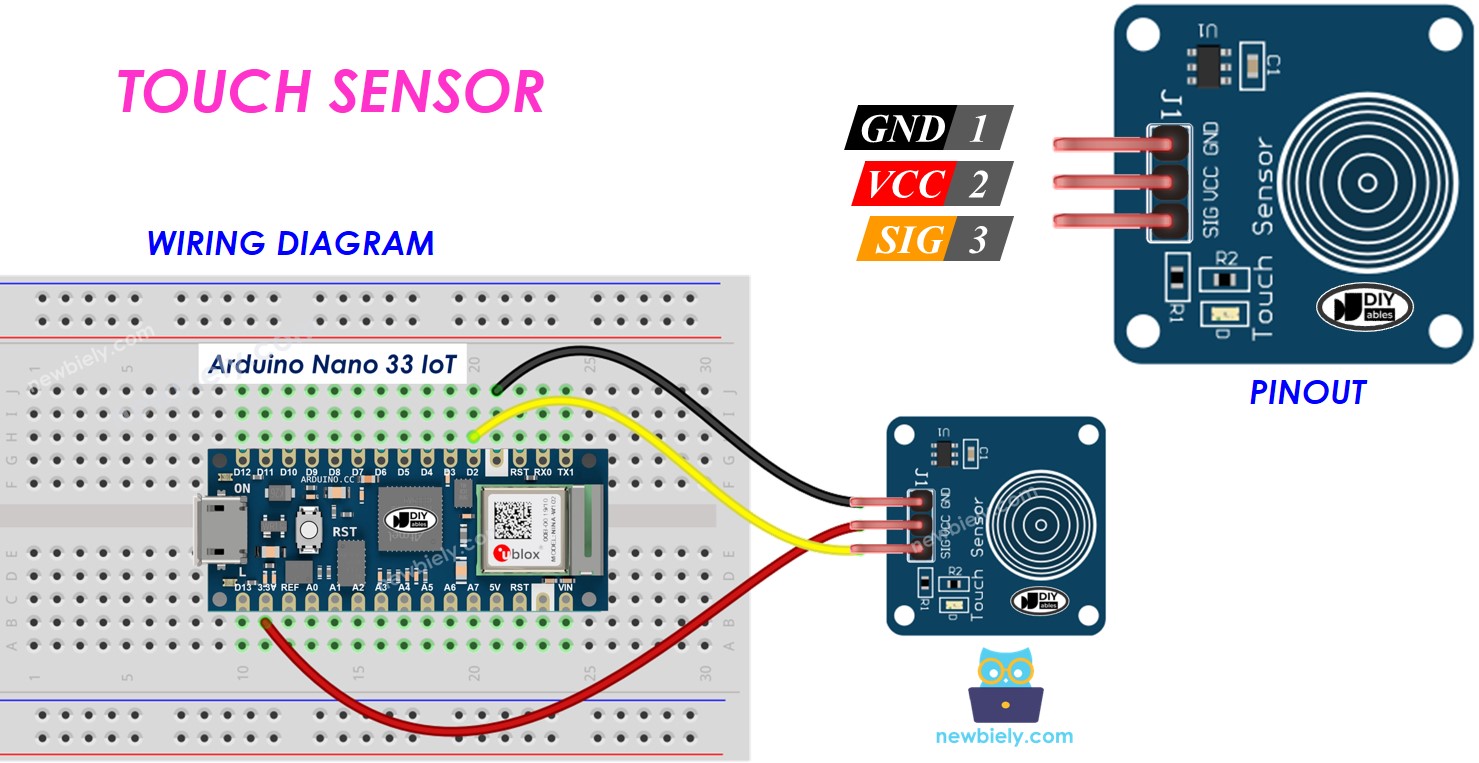
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Touch Sensor
Touch Sensor Pinout
A touch sensor has three pins.
- GND pin: Connect this pin to ground (0V).
- VCC pin: Connect this pin to the power supply (5V or 3.3V).
- SIGNAL pin: This pin sends out a signal. It is LOW when not touched and HIGH when touched. Connect this pin to one of the input pins on the Arduino Nano 33 IoT.
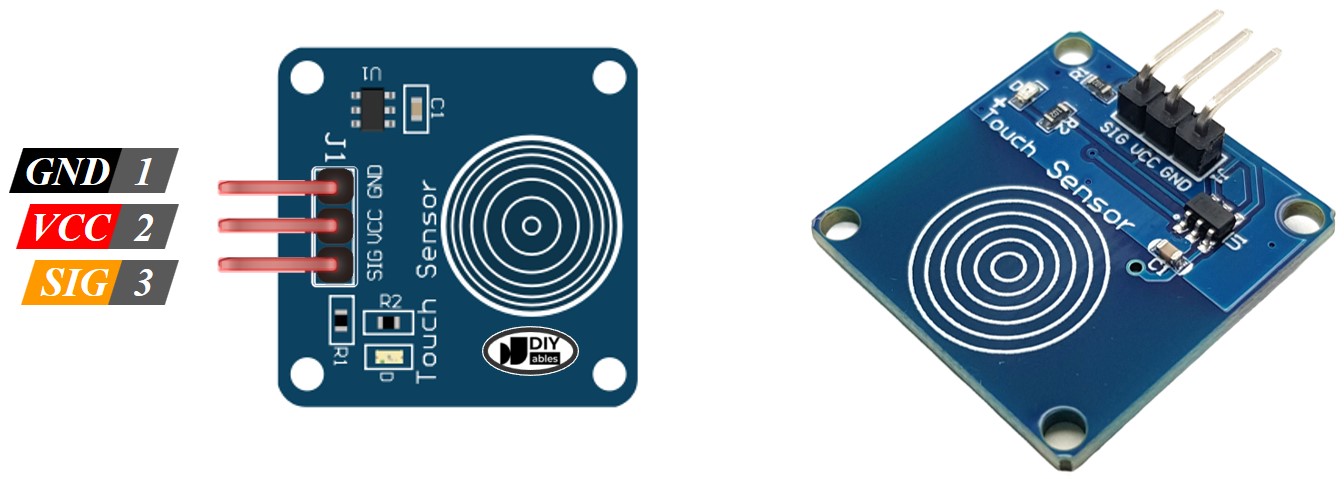
How Touch Sensor Works
- When you do not touch the sensor, the SIGNAL pin is low.
- When you touch the sensor, the SIGNAL pin is high.
Arduino Nano 33 IoT - Touch Sensor
We can hook the touch sensor's signal pin to one of the Arduino Nano 33 IoT's input pins. Then, we use the board's program to check if the touch sensor is active.
Wiring Diagram between Touch Sensor and Arduino Nano 33 IoT
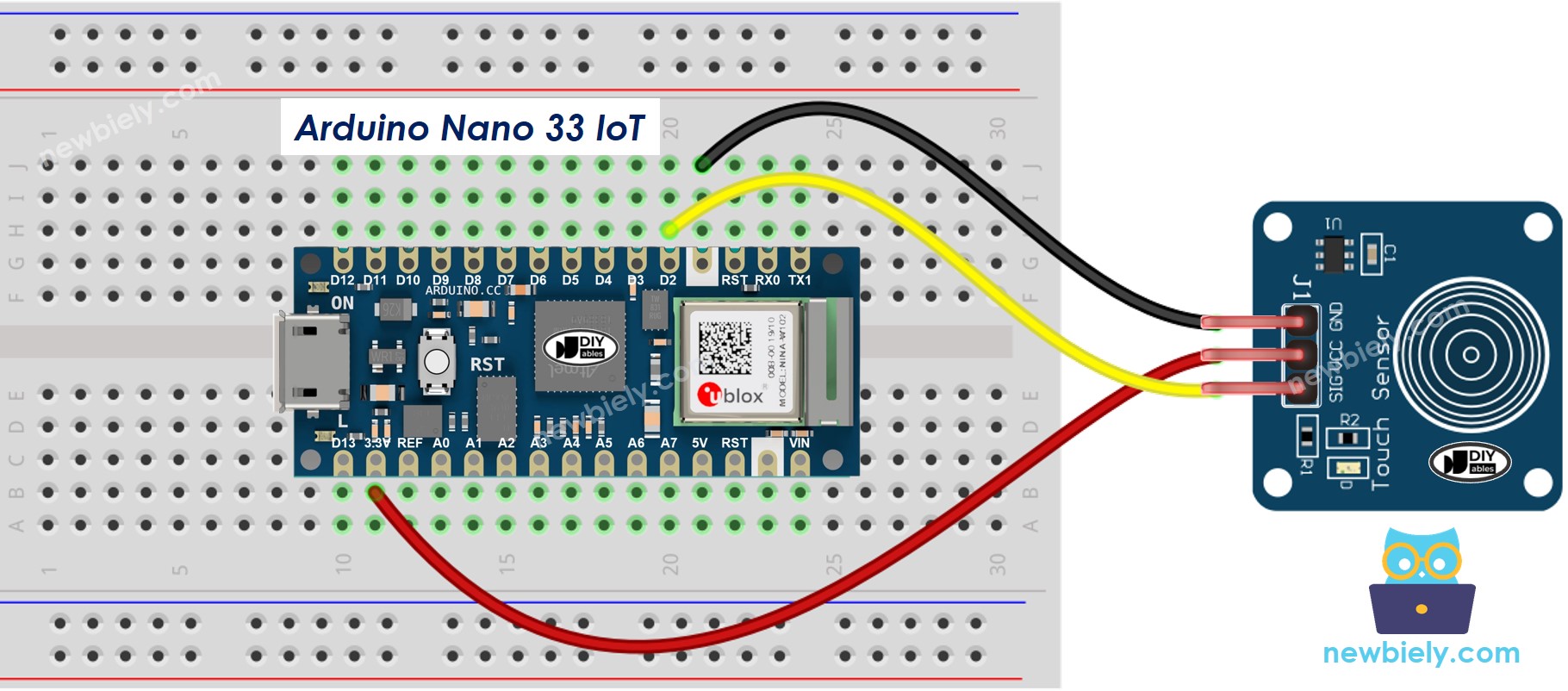
This image is created using Fritzing. Click to enlarge image
※ NOTE THAT:
Please note that the Arduino Nano 33 IoT pins A4 and A5 have built-in pull-up resistors for I2C communication. Although these pins can be used as digital input pins, it is recommended to avoid using them for digital input. If you must use them, do NOT use internal or external pull-down resistors for these pins
How To Program Touch Sensor
- This code sets a pin on the Arduino Nano 33 IoT (like pin D2) as a digital input using the pinMode() function.
- Checks the current condition of an Arduino Nano 33 IoT pin using the digitalRead() function.
Touch Sensor - Arduino Nano 33 IoT Code
This code checks the touch sensor and shows its status on the Serial Monitor.
Detailed Instructions
If you are new to the Arduino Nano 33 IoT, be sure to check out our Getting Started with Arduino Nano 33 IoT tutorial. Then, follow these steps:
- Connect the components to the Arduino Nano 33 IoT board as depicted in the diagram.
- Use a USB cable to connect the Arduino Nano 33 IoT board to your computer.
- Launch the Arduino IDE on your computer.
- Select the Arduino Nano 33 IoT board and choose its corresponding COM port.
- Copy the code above and paste it into the Arduino IDE.
- Click the Upload button in the Arduino IDE to compile and send the code to your Arduino Nano 33 IoT board.
- Touch the sensor with your finger, then lift your finger off.
- Open the Serial Monitor to see the result, which should look like the image below.