Arduino Nano 33 IoT - HTTP Request
In this tutorial, you'll learn how to make HTTP and HTTPS requests with the Arduino Nano 33 IoT board. We cover:
- Making a basic HTTP GET request to retrieve data from a server.
- Making secure HTTPS requests using SSL/TLS on port 443.
- Modifying the GET request to include the sensor data in the query string (e.g., temperature=26&humidity=70).
- Switching to an HTTP POST request to send data in the request body.
- Sending data in various formats like URL-encoded, JSON, or CSV.
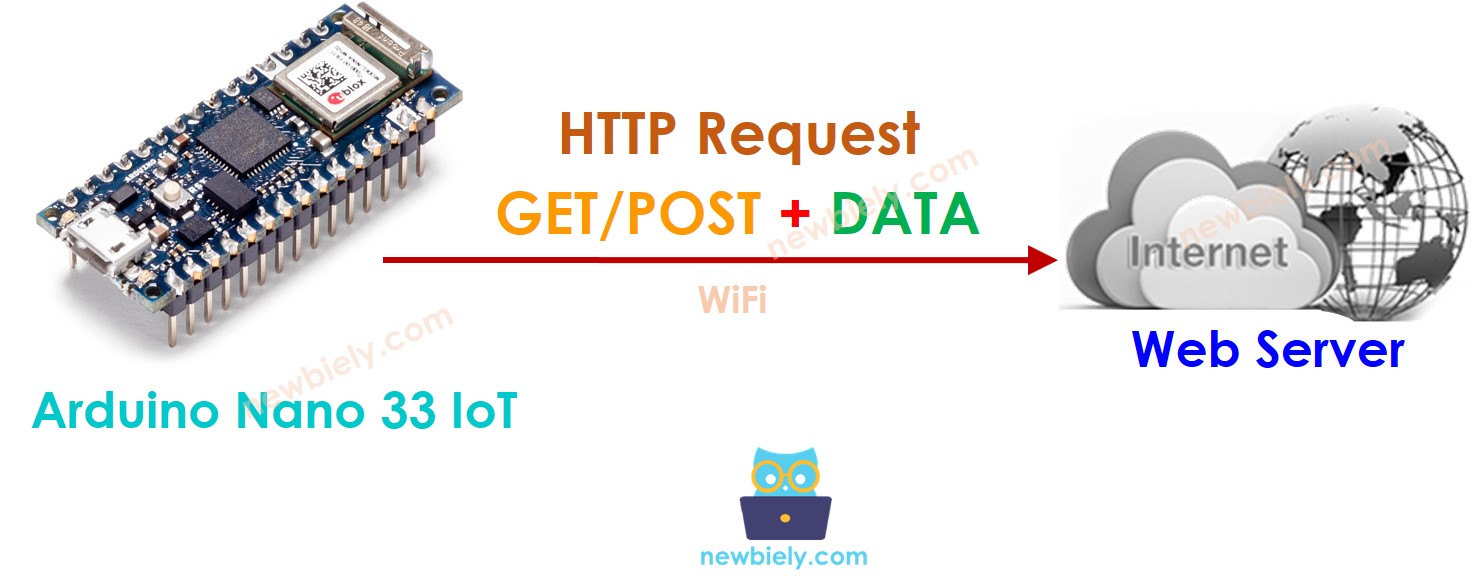
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Basic Concepts of Web Client and Web Server
The web has some basic concepts such as a website address (URL), a hostname, a pathname, a query string, and HTTP requests. You can learn more about these in the HTTP tutorial.
How to Program Arduino Nano 33 IoT to Make an HTTP Request
- Add libraries
- Enter your WiFi network name and password.
- Define the website name, the page path, and the query string details.
- Create an HTTP client object.
- Connect Arduino Nano 33 IoT to the WiFi network.
- Make HTTP GET request
- Get the data that comes back from the web server.
How to include data into HTTP request
We can send information to a web server by adding it to an HTTP request. The way the information is set up depends on the HTTP request method used.
- For a GET request:
- Data can only be sent in the query string of the URL.
- For a POST request:
- Data can be sent not only in the query string but also in other formats like JSON, XML, or image.
- Data is placed in the body of the HTTP request.
Let's learn how to send data as a query string using both HTTP GET and POST methods.
- Make a query string
- HTTP GET: Attach the part that contains extra information (query string) to the main URL (pathname).
- HTTP POST: Place the query string inside the body of the HTTP message.
- For both GET and POST, get the data sent back from the web server.
Arduino Nano 33 IoT Code for Making HTTP Request
Below is the full Arduino Nano 33 IoT code that shows how to send HTTP GET and POST requests.
Detailed Instructions
If you are new to the Arduino Nano 33 IoT, be sure to check out our Getting Started with Arduino Nano 33 IoT tutorial. Then, follow these steps:
- Use a USB cable to connect the Arduino Nano 33 IoT board to your computer.
- Launch the Arduino IDE on your computer.
- Select the Arduino Nano 33 IoT board and choose its corresponding COM port.
- Open the Library Manager by clicking on the Library Manager icon on the left side of the Arduino software.
- Type WiFiNINA in the search box and find the WiFiNINA sensor library made by Arduino.
- Click on the Install button to add the library.
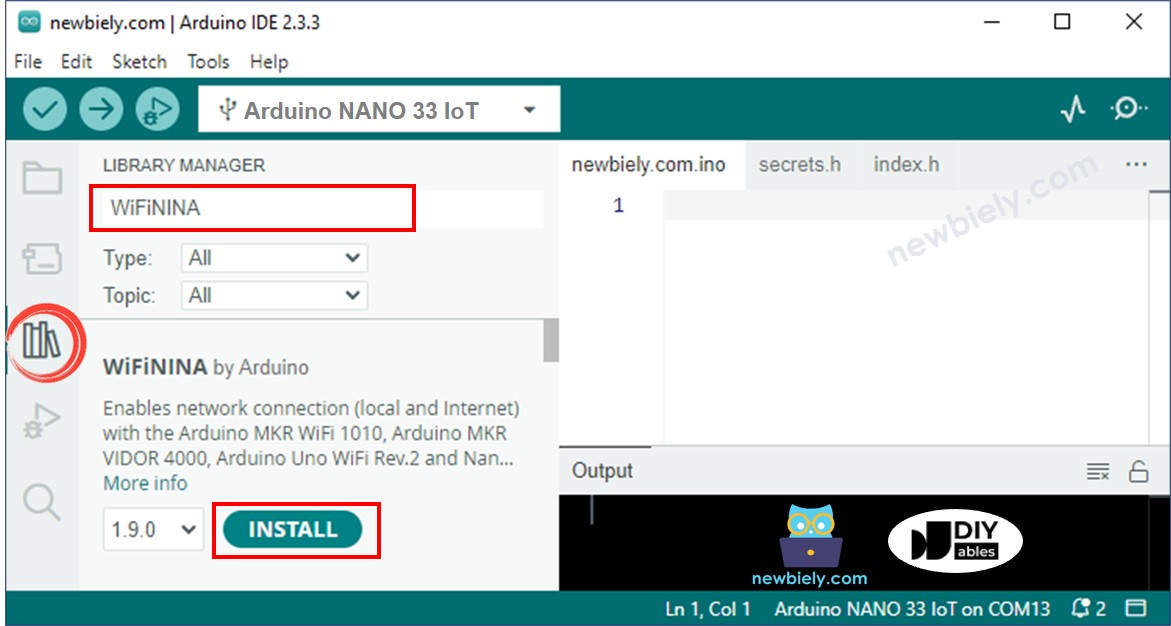
- Type ArduinoHttpClient in the search box and find the ArduinoHttpClient sensor library made by Arduino.
- Click on the Install button to add the library.
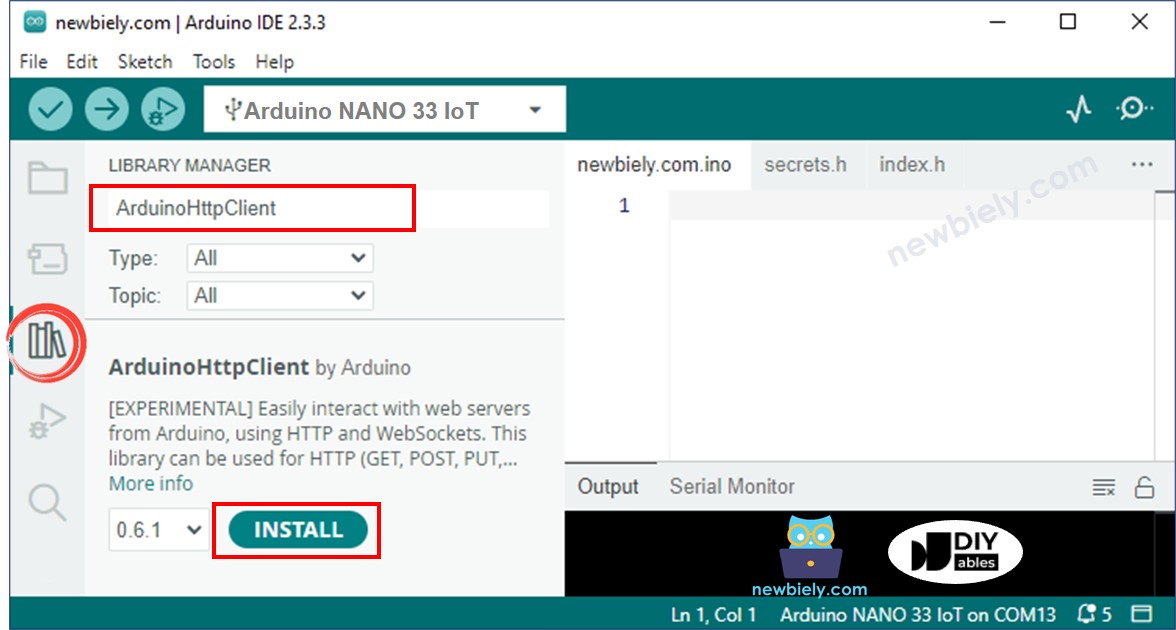
- Copy the above code and open with Arduino IDE
- Change the WiFi details (WIFI_SSID and WIFI_PASSWORD) in the code to yours.
- Optionally, change the HOST_NAME and PATH_NAME
- Click Upload button on Arduino IDE to upload code to Arduino Nano
- Open the Serial Monitor
- Check out the result on Serial Monitor. If everything goes right, you will see the text looks like the below:
Please note that the above code demonstrates how to make an HTTP request. You need to replace the URL and queryString with your own values to make it work.
Arduino Nano 33 IoT Code for Making HTTPS Request
Instructions for Using HTTPS on Arduino Nano 33 IoT
To use HTTPS with the Arduino Nano 33 IoT, follow the same steps as in the previous instructions for HTTP. If you've completed the previous steps, you should already have the necessary libraries installed and your Arduino IDE set up.
Additional Steps:
- Copy and paste the provided HTTPS code into your Arduino IDE.
- Make sure to update the WiFi credentials (WIFI_SSID and WIFI_PASSWORD) in the code.
- Optionally, adjust the HOST_NAME and PATH_NAME to match your server.
- Upload the code to your Arduino Nano 33 IoT.
- Open the Serial Monitor to see the output.
Since the target website supports both HTTP and HTTPS, the output on the Serial Monitor should look similar to what you saw in the HTTP example.
Arduino Nano 33 IoT HTTP vs HTTPS Code Differences
If you compare the HTTP code and HTTPS code, you will see just three lines of difference (excluding the printing statements).
Aspect | HTTP Code | HTTPS Code |
---|---|---|
Port Number | int HTTP_PORT = 80; | int HTTP_PORT = 443; |
WiFi Client Type | WiFiClient wifiClient; | WiFiSSLClient wifiSSLClient; |
HttpClient Initialization | HttpClient client(wifiClient, HOST_NAME, HTTP_PORT); | HttpClient client(wifiSSLClient, HOST_NAME, HTTP_PORT); |
Summary:
- Port Number: HTTP uses 80, while HTTPS uses 443 for secure communication.
- WiFi Client Type: HTTPS requires WiFiSSLClient for SSL/TLS encryption.
- HttpClient Initialization: The SSL client replaces the standard client in HTTPS.
These small changes enable secure, encrypted communication when switching from HTTP to HTTPS.
The rest of this tutorial provides HTTP code, but you can easily modify it for HTTPS by changing the port to 443, using WiFiSSLClient, and updating HttpClient accordingly.
Arduino Nano 33 IoT Code for Making HTTP GET Request with data
In the previous code, the HTTP GET request was made without including any data. However, in practice, you may want to send data, such as sensor readings or peripheral values, as part of the request.
To do this, you can append the data as a query string to the request URL. The code below demonstrates how to modify the existing HTTP GET request to include data:
Arduino Nano 33 IoT Code for Making HTTP POST Request with data
In the previous code, data was included in the URL as a query string for a GET request. However, in many cases, such as sending larger data or sensitive information, it's better to use a POST request, which sends data in the request body instead of the URL.
The code below demonstrates how to modify the GET request to a POST request, ensuring data is properly sent in the request body. 🚀
This example shows how to include data in the URL-encoded format, but you can easily modify the code to send other formats such as JSON, CSV, or any custom format depending on the requirements of your server.