Arduino Nano 33 IoT - Door Lock System using Password
This guide explains how to use an Arduino Nano 33 IoT, a keypad, and an electromagnetic lock to build a door lock system. When you enter a password on the keypad, the system checks its correctness. If the password is right, the Arduino Nano 33 IoT sends a signal to the electromagnetic lock that opens the door. The guide also shows you how to add an LCD for extra convenience. The code for the Arduino Nano 33 IoT supports multiple passwords.
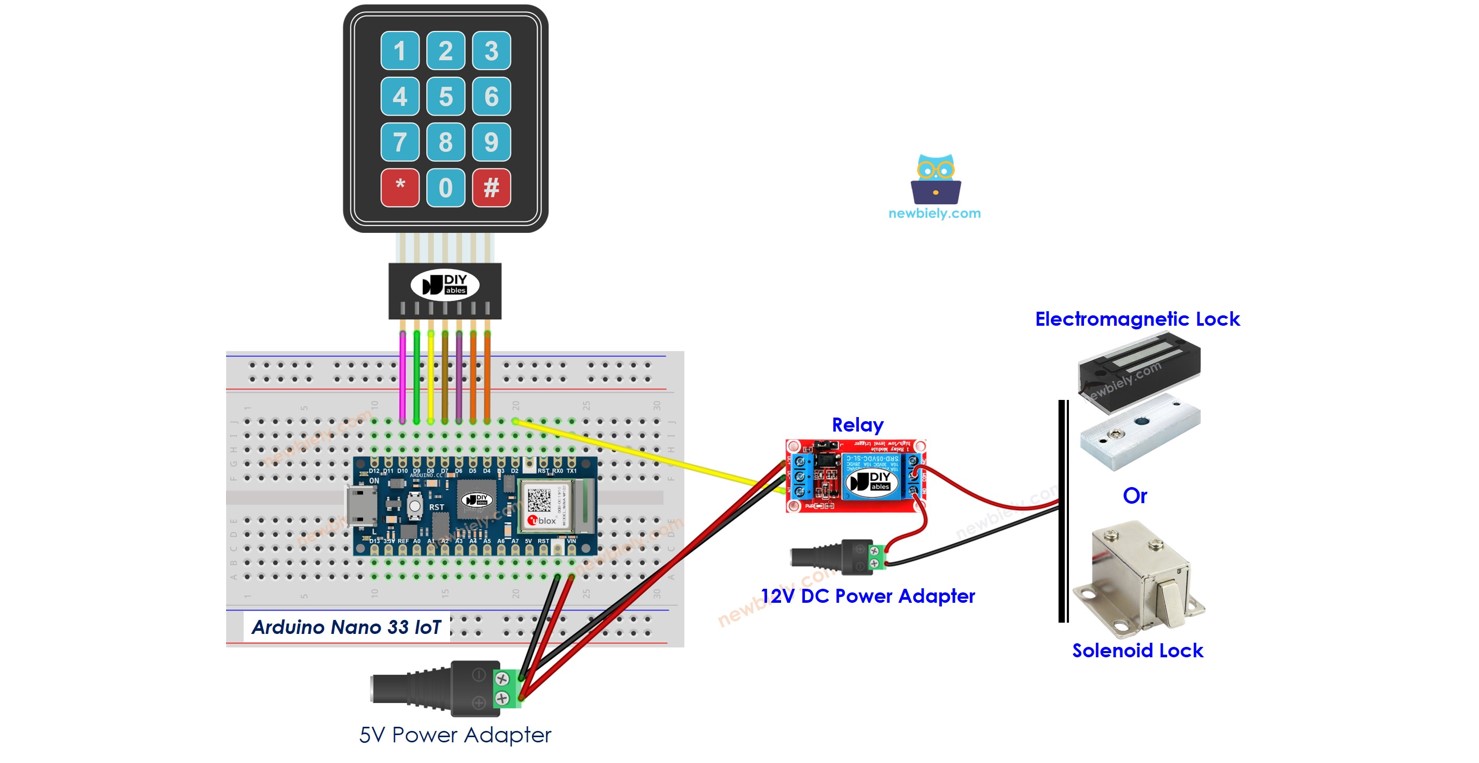
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Keypad, Electromagnetic Lock and LCD
If you're new to using the Keypad, Electromagnetic Lock, LCD, and Arduino Nano 33 IoT, please check out these tutorials:
These tutorials explain how Keypad, Electromagnetic Lock and LCD work, their pinouts, how to connect them to the Arduino Nano 33 IoT, and how to program Arduino Nano 33 IoT to work with the Keypad and Electromagnetic Lock and LCD.
How check the password
- The special key * is used to start over the password.
- The special key # is used to finish entering the password.
- Every key you press, except for * and #, is added to a word.
- When the # key is pressed, the password entry is done. The Arduino Nano 33 IoT then checks the word against a list of passwords saved before.
- If the entered password is correct, the door will open.
Wiring Diagram
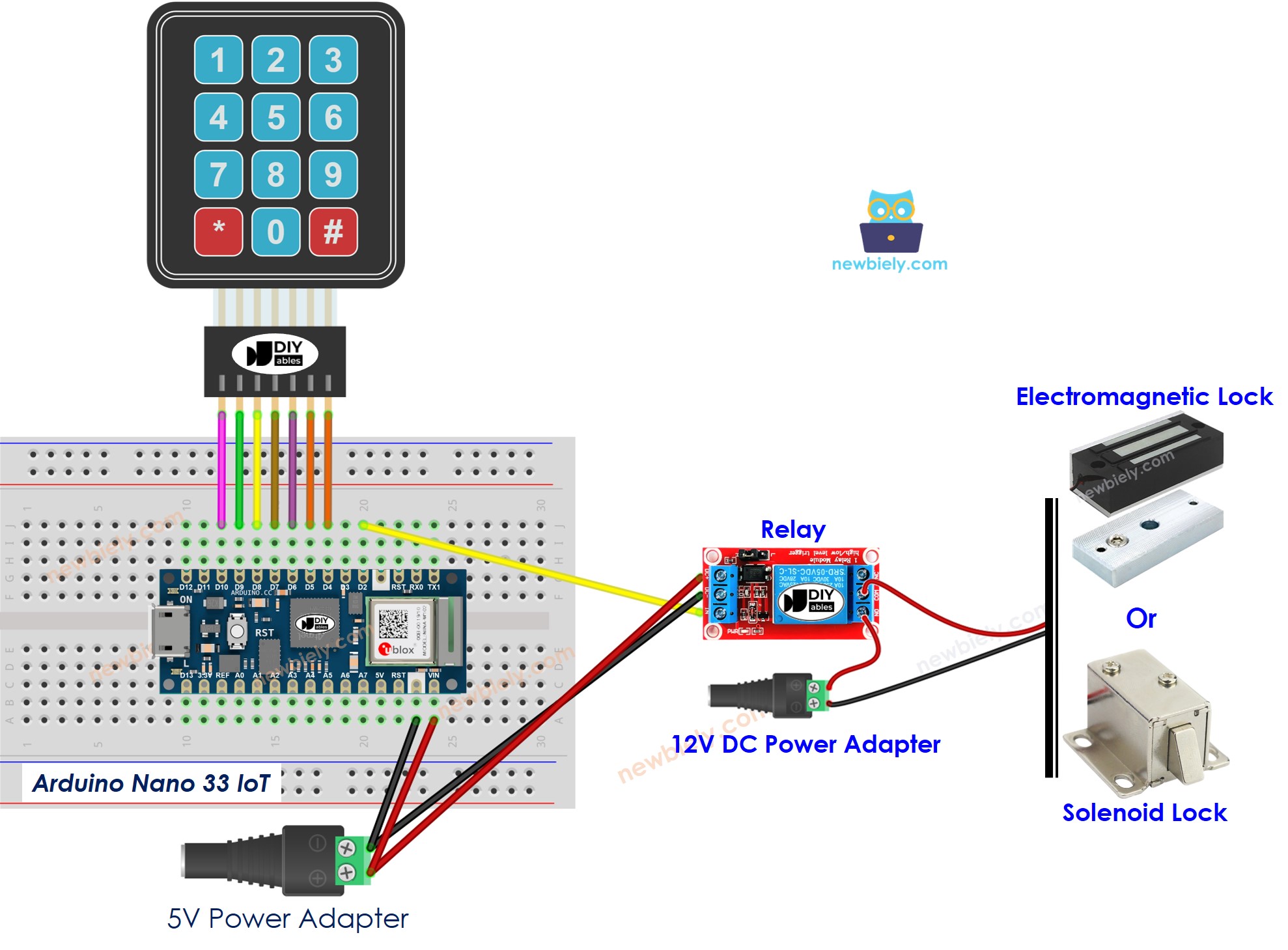
This image is created using Fritzing. Click to enlarge image
Arduino Nano 33 IoT Code - Door lock system with password using keypad, electromagnetic lock
Detailed Instructions
If you are new to the Arduino Nano 33 IoT, be sure to check out our Getting Started with Arduino Nano 33 IoT tutorial. Then, follow these steps:
- Connect the components to the Arduino Nano 33 IoT board as depicted in the diagram.
- Use a USB cable to connect the Arduino Nano 33 IoT board to your computer.
- Launch the Arduino IDE on your computer.
- Select the Arduino Nano 33 IoT board and choose its corresponding COM port.
- Click the Libraries icon on the left side of the Arduino program.
- Type DIYables_Keypad in the search box and find the keypad library by DIYables.io.
- Click the Install button to add the keypad library.
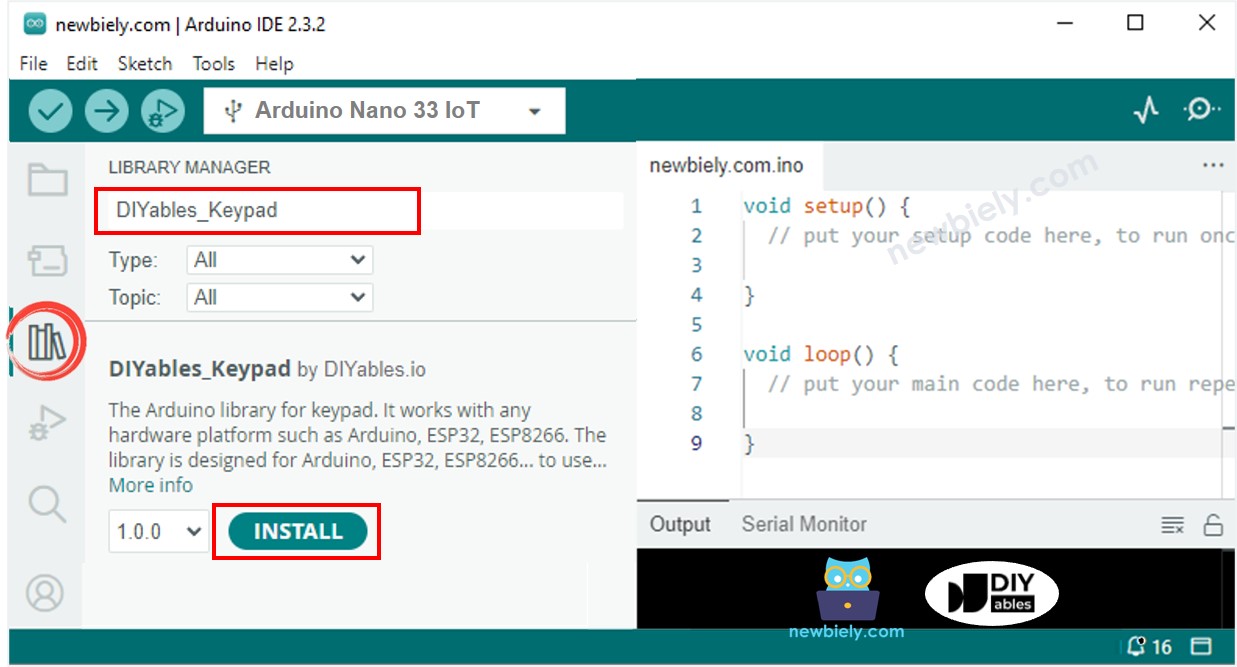
- Copy the code above and paste it into the Arduino IDE.
- Click the Upload button in the Arduino IDE to compile and send the code to the Arduino Nano 33 IoT board.
- To test the electromagnet door lock, put the electromagnet close to the metal plate.
- Press the keys "1111" and then press the "#" key.
- Observe the force between the electromagnet and the metal plate.
- Press the keys "1234" and then press the "#" key.
- Watch the force between the electromagnet and the metal plate again.
- View the results on the Serial Monitor.
Arduino Nano 33 IoT Code - Door lock system with password using keypad, electromagnetic lock and LCD
The code below adds an LCD I2C screen to show the access status. To learn how to wire the LCD, please check this tutorial: Arduino Nano 33 IoT - LCD I2C tutorial
※ NOTE THAT:
The LCD I2C address might be different for each maker. In our code, we used the address 0x27, which is given by the DIYables maker.