Arduino Nano 33 IoT - LED - Blink Without Delay
One of the first programs beginners try is to make an LED blink. The easiest way to blink an LED is to use the delay() function. This function stops the Arduino Nano 33 IoT from doing other tasks. This is fine if you just want to blink one LED. But if you want to blink more LEDs or do other things at the same time, you cannot use the delay() function. We need another solution. This guide will show you how to handle multiple tasks without using delay(). Specifically, we will learn how to blink an LED and check a button’s state.
We will go through three examples below and see how they differ.
- Arduino Nano 33 IoT turns the LED on and off using the delay() function.
- Arduino Nano 33 IoT turns the LED on and off using the millis() function.
- Arduino Nano 33 IoT turns the LED on and off using the ezLED library.
This approach allows the Arduino Nano 33 IoT to handle many tasks at the same time. Turning an LED on and off is just one example.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Wiring Diagram
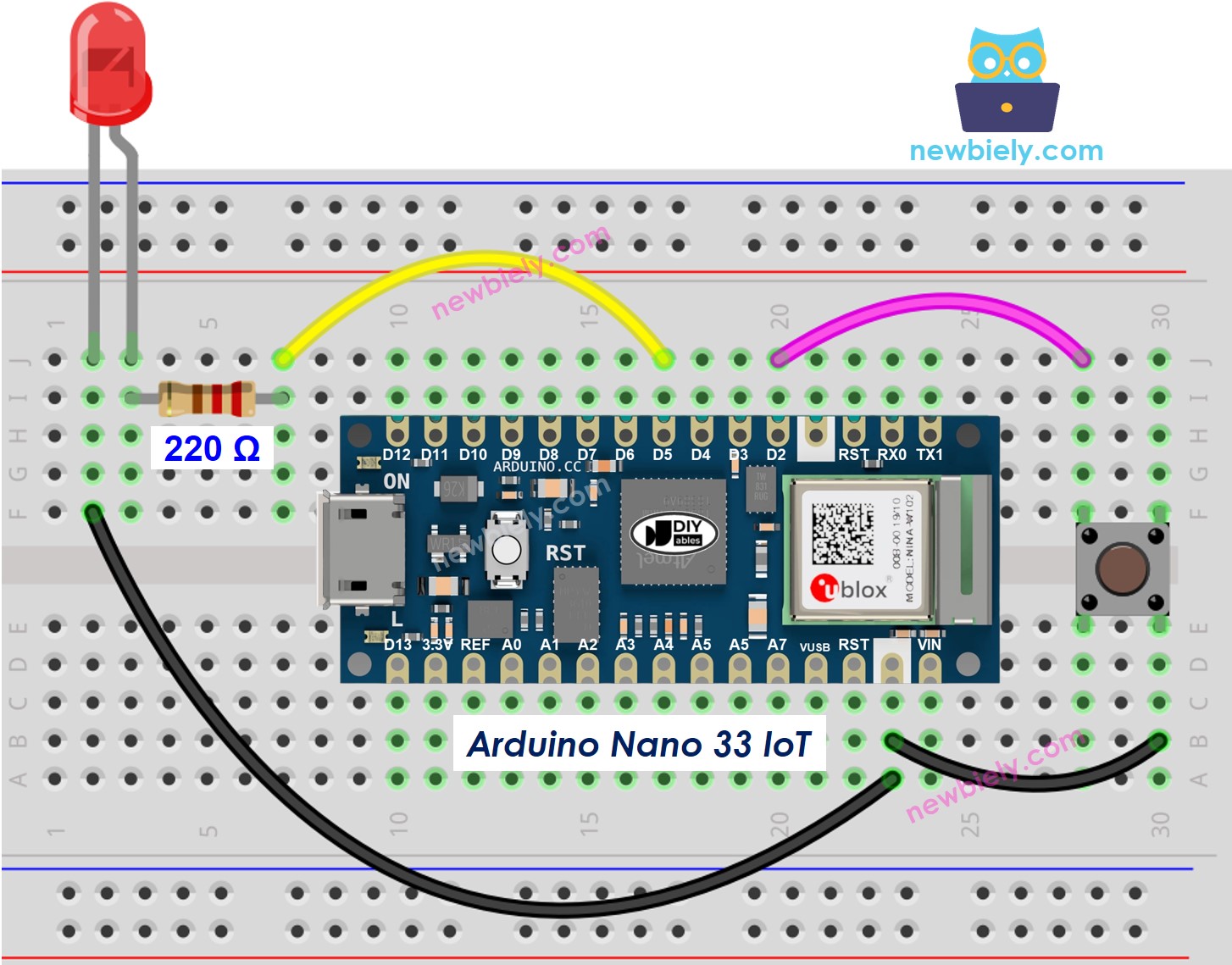
This image is created using Fritzing. Click to enlarge image
Let's compare two versions of the Arduino Nano 33 IoT code that makes an LED blink: one version uses the delay() function and the other version does not.
※ NOTE THAT:
Please note that the Arduino Nano 33 IoT pins A4 and A5 have built-in pull-up resistors for I2C communication. Although these pins can be used as digital input pins, it is recommended to avoid using them for digital input. If you must use them, do NOT use internal or external pull-down resistors for these pins
Arduino Nano 33 IoT Code - With Delay
Detailed Instructions
If you are new to the Arduino Nano 33 IoT, be sure to check out our Getting Started with Arduino Nano 33 IoT tutorial. Then, follow these steps:
- Connect the components to the Arduino Nano 33 IoT board as depicted in the diagram.
- Use a USB cable to connect the Arduino Nano 33 IoT board to your computer.
- Launch the Arduino IDE on your computer.
- Select the Arduino Nano 33 IoT board and choose its corresponding COM port.
- Copy the code from above and paste it into the Arduino IDE.
- Click the Upload button in the Arduino IDE to compile the code and send it to the Arduino Nano 33 IoT board.
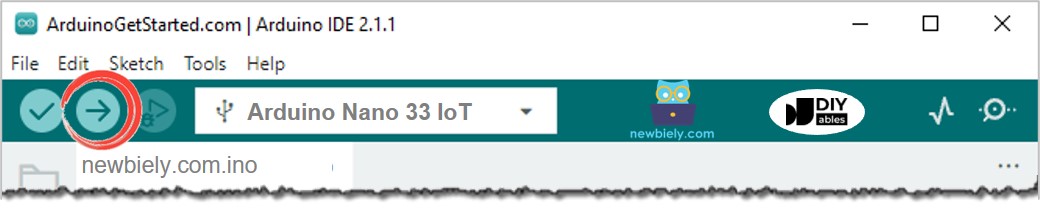
- Open the Serial Monitor in the Arduino software.
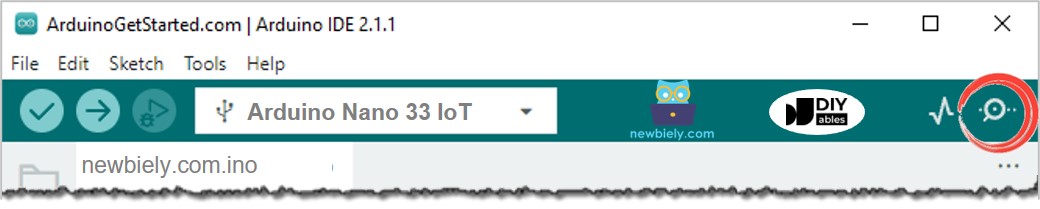
- Press the button 4 times. Watch the LED as it changes between on and off every second. Look at the output in the Serial Monitor.
- On the Serial Monitor, you won't see the state change to 0 four times (after 4 presses). This happens because, during the delay period, the Arduino Nano 33 IoT cannot notice the change.
Arduino Nano 33 IoT Code - Without Delay
Detailed Instructions
- Run the code above and press the button 4 times.
- Watch the LED light: It turns on and off every second.
- Look at the results in the Serial Monitor.
- Every press was noticed.
Line-by-line Code Explanation
The Arduino Nano 33 IoT code above has an explanation for every line. Please check the comments in the code!
Adding More Tasks
This code makes two lights blink at different speeds and checks if the button is pressed.