Arduino Nano 33 IoT - DIP Switch
In electronics, DIP (Dual In-line Package) switches are often used for setting up things like device addresses, communication options, and security codes. This guide will show you how to use a DIP switch with the Arduino Nano 33 IoT. We will cover:
- Understanding how a DIP switch works
- Connecting the DIP switch to an Arduino Nano 33 IoT
- Writing code on the Arduino Nano 33 IoT to check if the DIP switch is ON or OFF
- Writing code on the Arduino Nano 33 IoT to find out the number set by the DIP switch
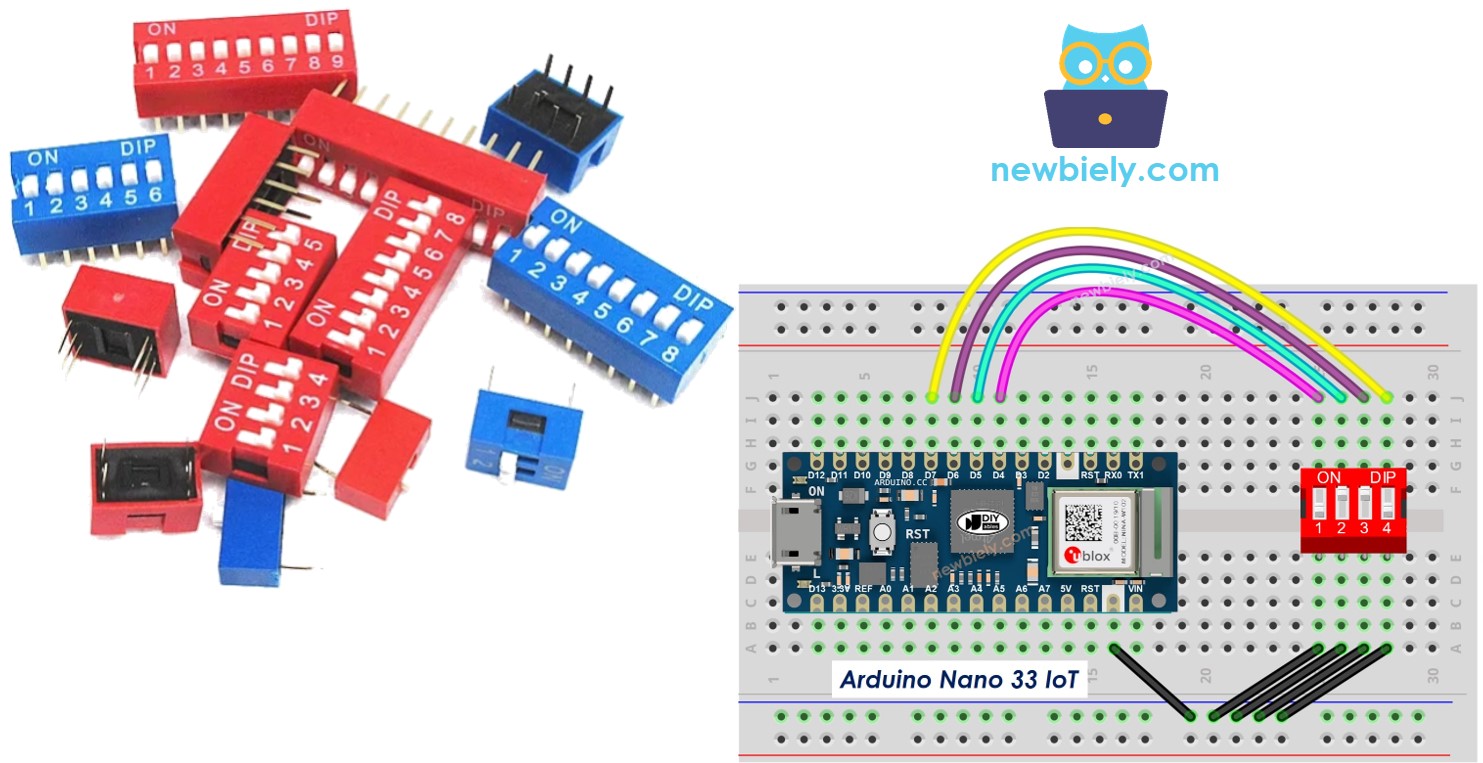
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of DIP Switch
DIP switches are important tools for setting up devices. They let users change things like the device address, communication options, security codes, working modes, and other system settings for different industries and uses.
A DIP switch is a group of small sliding switches that are put together. Each switch is called a "position." There are different kinds of DIP switches depending on how many positions they have. For example, you can have DIP switches with 2, 4, 5, 6, 8, or 10 positions.
Each position on a DIP switch is like one part of a number. By turning these positions on and off, you can set the number you want to use.
Pinout
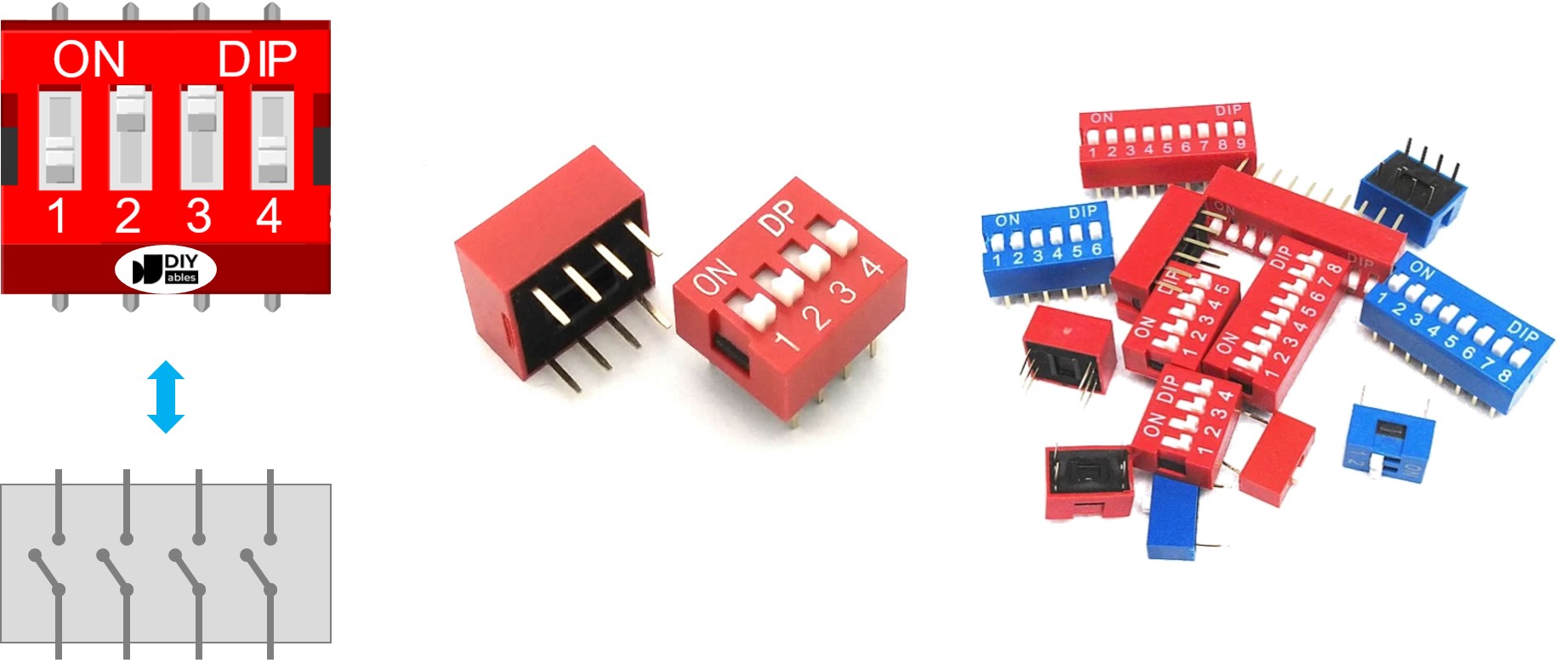
A DIP switch has two rows of pins, with each row having as many pins as there are switch positions. For example, a 4-position DIP switch has 8 pins in total, with 4 pins on each side. In the DIP switch, each pair of opposite pins makes one slide switch. You don't need to worry about the difference between the two sides because the pins work the same on either side.
How It Works
In DIP switches, when a switch is turned on, it means that the switch is active or closed. This means an electrical connection is made, so the current can flow through the switch.
When a switch is off, it means it is not connected or closed. In this condition, the electrical connection is broken, so the current cannot pass through the switch.
Just to be clear:
- ON position: Connects the circuit so electricity can flow.
- OFF position: Disconnects the circuit so electricity stops flowing.
If you connect one side of a switch to ground (GND) and the other side to a pin on the Arduino Nano 33 IoT (set as a pull-up digital input), the table below shows how the switch's position matches the values that the Arduino reads.
DIP switch position | Binary representation | Circuit state | Arduino Nano 33 IoT pin state |
---|---|---|---|
ON | 1 | CLOSED | LOW |
OFF | 0 | OPEN | HIGH |
In the next sections, we'll use a 4-position DIP switch as an example. You can easily switch to using DIP switches with 2, 3, 5, 6, 8, or 10 positions.
Wiring Diagram
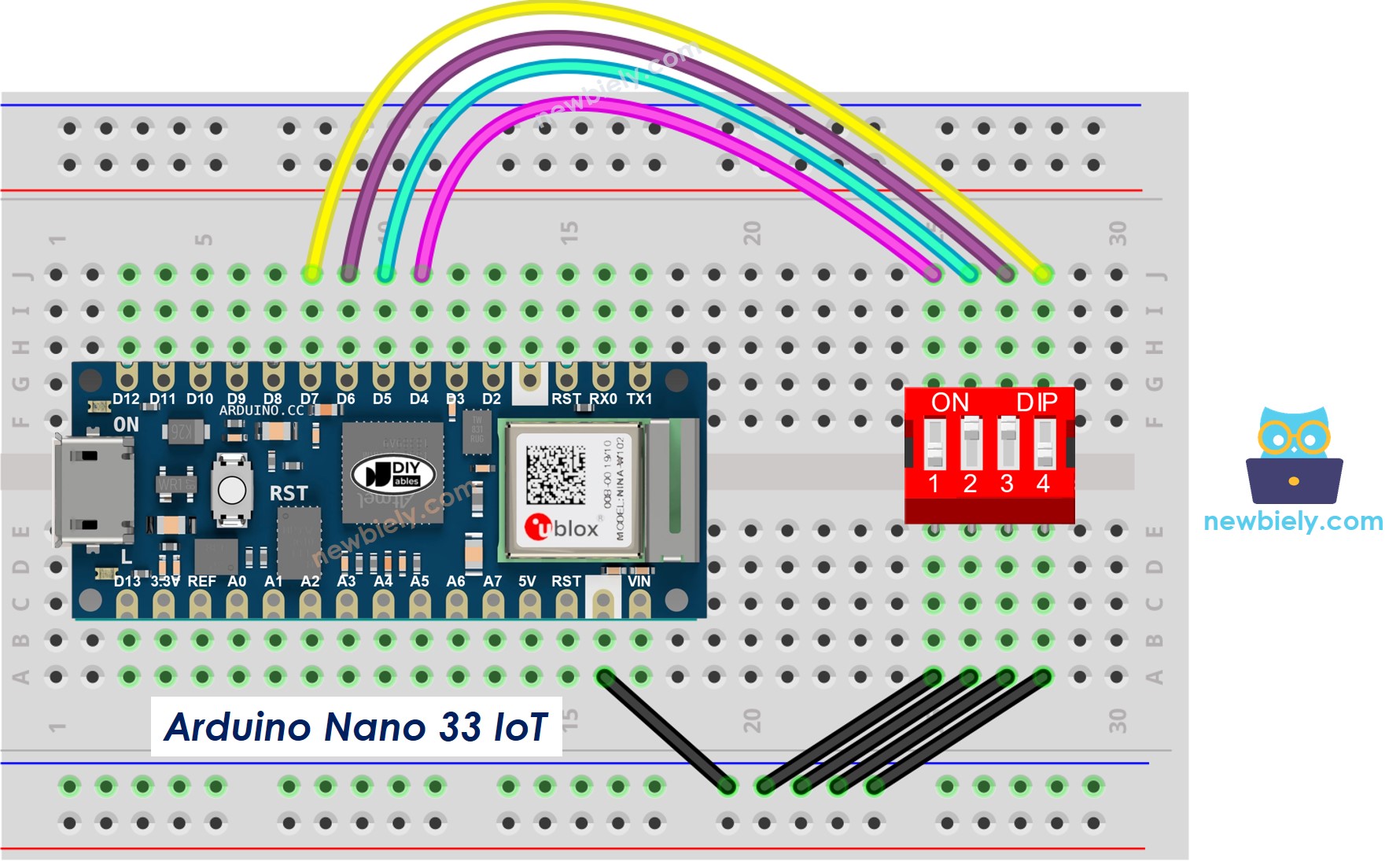
This image is created using Fritzing. Click to enlarge image
※ NOTE THAT:
Please note that the Arduino Nano 33 IoT pins A4 and A5 have built-in pull-up resistors for I2C communication. Although these pins can be used as digital input pins, it is recommended to avoid using them for digital input. If you must use them, do NOT use internal or external pull-down resistors for these pins
Arduino Nano 33 IoT Code - DIP Switch
We will learn with two code examples:
- Checking if each DIP switch is turned on or off.
- Changing the switch positions into a number.
Arduino Nano 33 IoT code - Reading the ON/OFF state of the DIP switch
Detailed Instructions
If you are new to the Arduino Nano 33 IoT, be sure to check out our Getting Started with Arduino Nano 33 IoT tutorial. Then, follow these steps:
- Connect the components to the Arduino Nano 33 IoT board as depicted in the diagram.
- Use a USB cable to connect the Arduino Nano 33 IoT board to your computer.
- Launch the Arduino IDE on your computer.
- Select the Arduino Nano 33 IoT board and choose its corresponding COM port.
- Copy the code above and open it in the Arduino IDE
- Click the Upload button in the Arduino IDE to send the code to your Arduino Nano 33 IoT.
- Turn each switch on the DIP Switch to the ON position one at a time.
- Check the Serial Monitor to see the result.
Arduino Nano 33 IoT code - Encoding the states of DIP switch into a number
Detailed Instructions
- Load the code onto the Arduino Nano 33 IoT.
- Turn on each switch on the DIP switch one at a time.
- Look at the Serial Monitor to see the output, which should look like the example below.
Please note that the value changes based on the position of each slide switch. The table below shows how the ON and OFF positions are linked to a number for a 4-position DIP switch.
Position-1 | Position-2 | Position-3 | Position-4 | Binary Value | Decimal Value |
---|---|---|---|---|---|
OFF | OFF | OFF | OFF | 0000 | 0 |
OFF | OFF | OFF | ON | 0001 | 1 |
OFF | OFF | ON | OFF | 0010 | 2 |
OFF | OFF | ON | ON | 0011 | 3 |
OFF | ON | OFF | OFF | 0100 | 4 |
OFF | ON | OFF | ON | 0101 | 5 |
OFF | ON | ON | OFF | 0110 | 6 |
OFF | ON | ON | ON | 0111 | 7 |
ON | OFF | OFF | OFF | 1000 | 8 |
ON | OFF | OFF | ON | 1001 | 9 |
ON | OFF | ON | OFF | 1010 | 10 |
ON | OFF | ON | ON | 1011 | 11 |
ON | ON | OFF | OFF | 1100 | 12 |
ON | ON | OFF | ON | 1101 | 13 |
ON | ON | ON | OFF | 1110 | 14 |
ON | ON | ON | ON | 1111 | 15 |