Arduino Nano 33 IoT - Soil Moisture Sensor
This guide shows you how to use the Arduino Nano 33 IoT board to measure soil moisture with a sensor. We will cover:
- Comparing a Capacitive Moisture Sensor and a Resistive Moisture Sensor
- Using the Arduino Nano 33 IoT to read numbers from a capacitive moisture sensor
- Steps to adjust a capacitive moisture sensor for accurate readings
- How the Arduino Nano 33 IoT checks if the soil is wet or dry
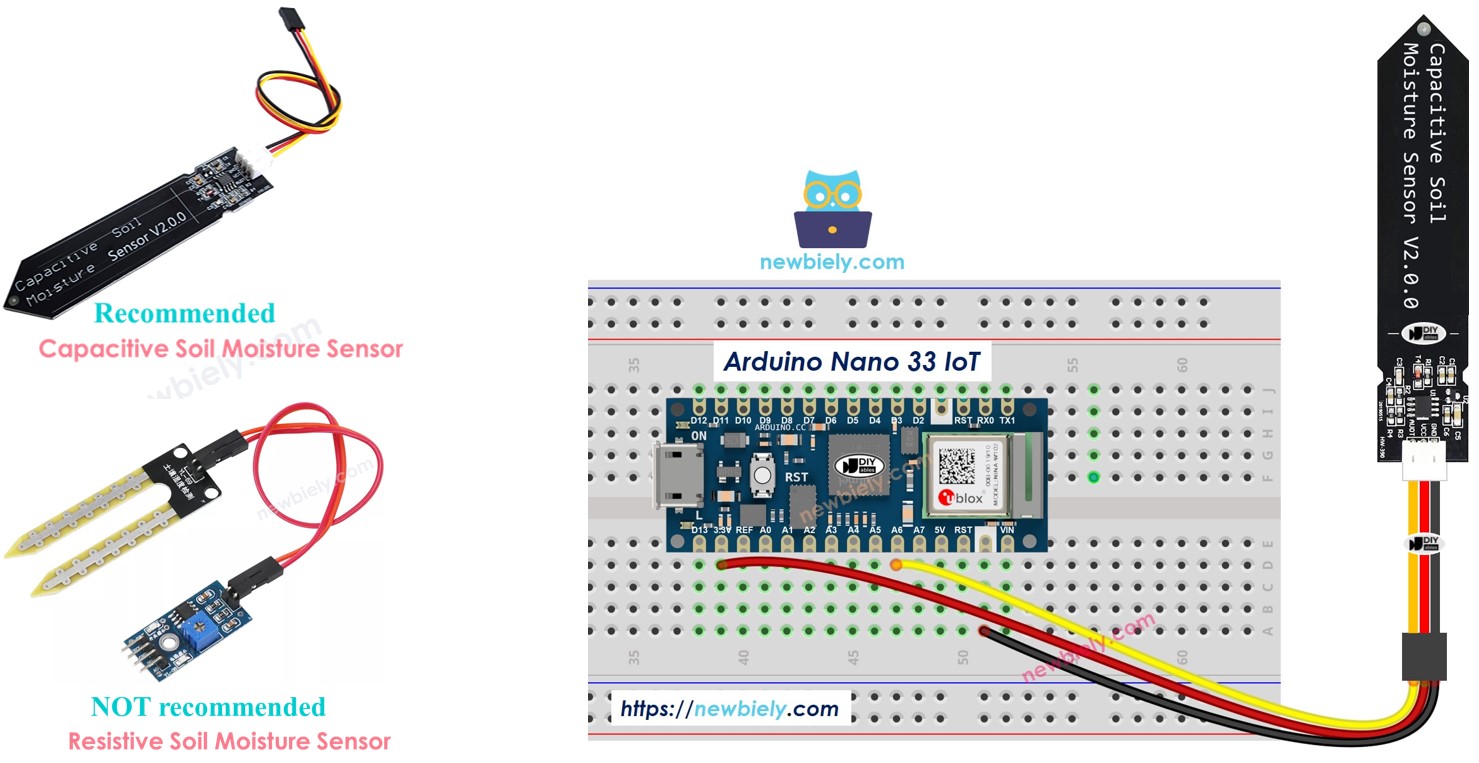
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Buy Note: Many soil moisture sensors available in the market are unreliable, regardless of their version. We strongly recommend buying the sensor with TLC555I Chip from the DIYables brand using the link provided above. We tested it, and it worked reliably.
Overview of Soil Moisture Sensor Sensor
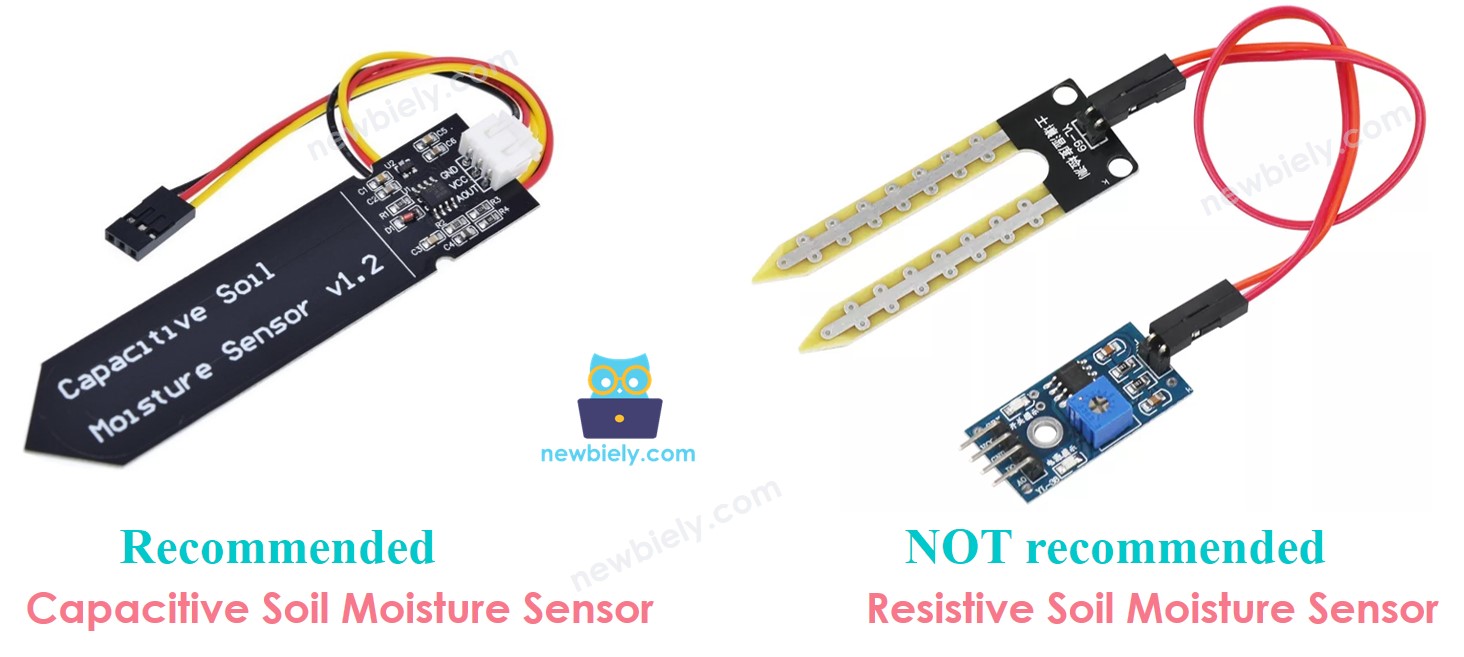
Moisture sensors come in two types:
- Resistive moisture sensor – a water sensor that measures moisture by checking how well electricity flows through a material.
- Capacitive moisture sensor – a water sensor that detects moisture by measuring changes in a material’s ability to hold an electrical charge.
Both sensors show the soil moisture level. But they work in different ways. We strongly suggest using the capacitive moisture sensor because of these reasons:
- The resistive soil moisture sensor gets damaged over time. The electrical current between its metal parts causes it to wear away.
- The capacitive soil moisture sensor does not get damaged over time. Its metal parts are hidden, so no electrical current passes between them.
The picture below shows rust on a sensor that checks how wet the soil is using electrical resistance.
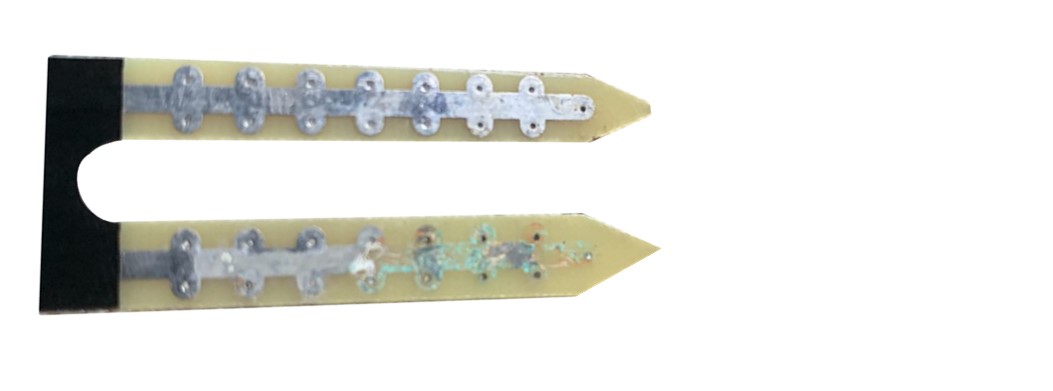
For the rest of this guide, we will use the capacitive soil moisture sensor.
Capacitive Soil Moisture Sensor Pinout
A capacitive sensor for soil moisture comes with three connection pins.
- GND pin: Connect this pin to ground (0 volts).
- VCC pin: Connect this pin to the power supply (5V or 3.3V).
- AOUT pin: This pin sends an analog signal. The voltage it outputs goes down when the soil is more moist. Connect this pin to an analog input on the Arduino Nano 33 IoT.
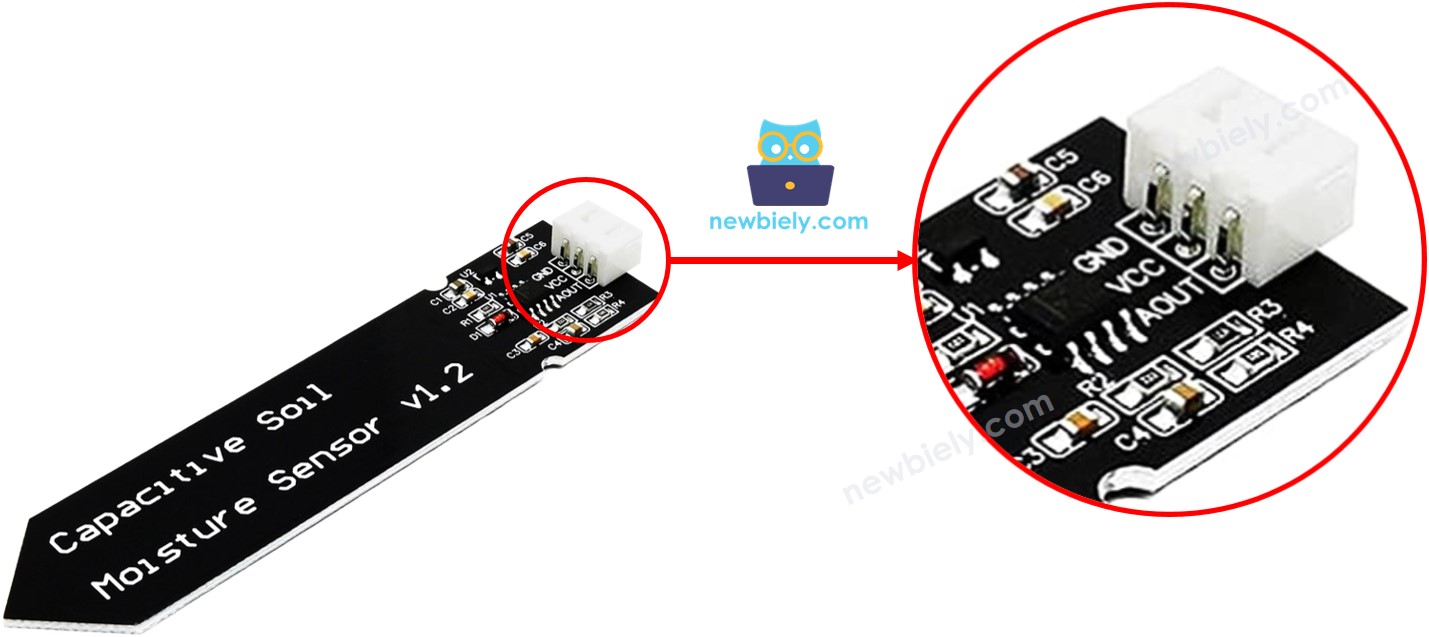
How It Works
When there is more water in the soil, the AOUT pin's voltage is lower.
Wiring Diagram
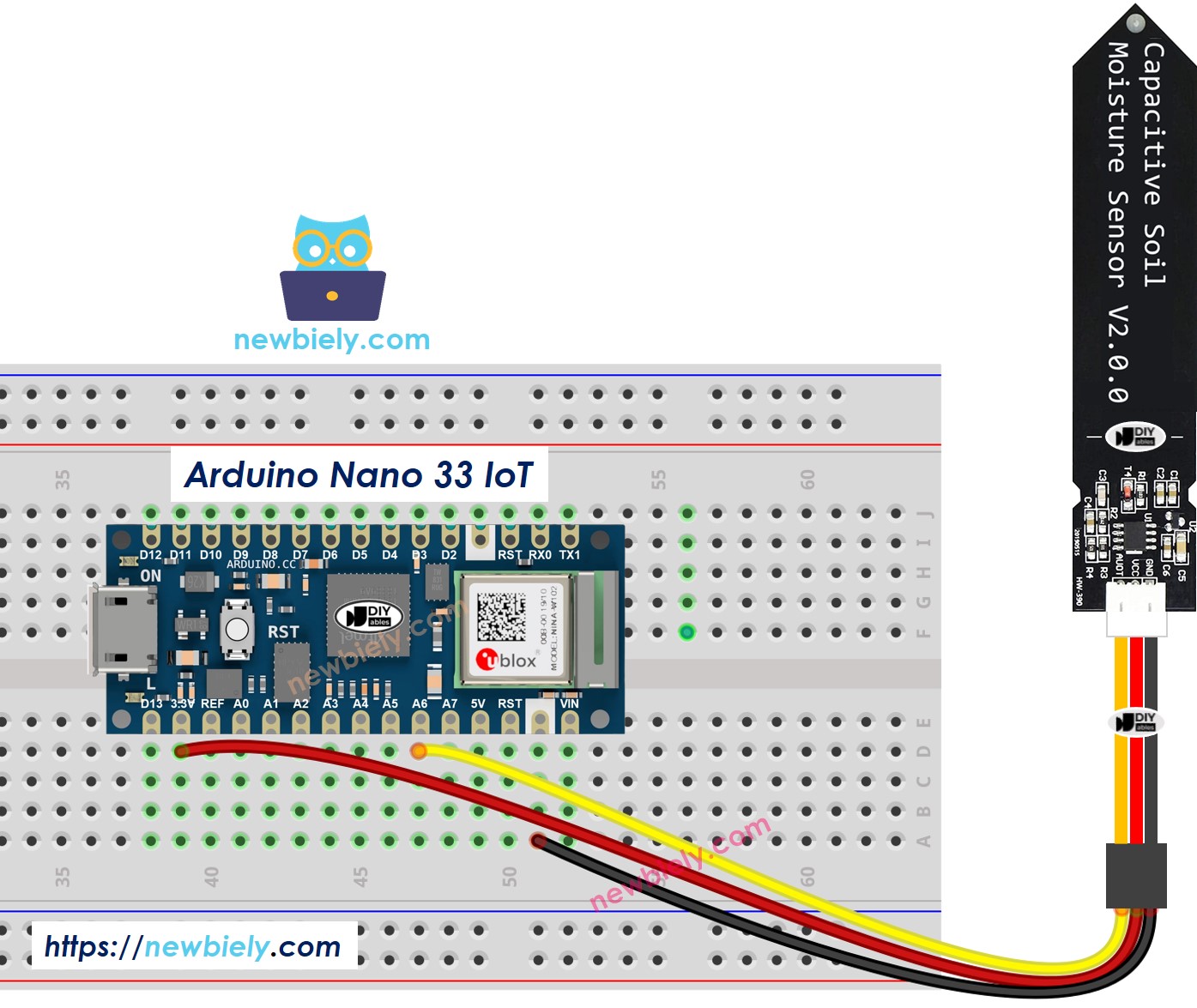
This image is created using Fritzing. Click to enlarge image
※ NOTE THAT:
Please note that the Arduino Nano 33 IoT pins A4 and A5 have built-in pull-up resistors for I2C communication. This can affect analog readings, so it is recommended to avoid using these pins with any devices/sensors that relies on ADC.
Arduino Nano 33 IoT Code
Detailed Instructions
If you are new to the Arduino Nano 33 IoT, be sure to check out our Getting Started with Arduino Nano 33 IoT tutorial. Then, follow these steps:
- Connect the components to the Arduino Nano 33 IoT board as depicted in the diagram.
- Use a USB cable to connect the Arduino Nano 33 IoT board to your computer.
- Launch the Arduino IDE on your computer.
- Select the Arduino Nano 33 IoT board and choose its corresponding COM port.
- Copy the code shown above and paste it into the Arduino IDE.
- Click the Upload button in the Arduino IDE to send the code to your Arduino Nano 33 IoT board.
- Bury the sensor in the soil, then pour some water onto the soil. Alternatively, slowly lower the sensor into a cup of salt water.
- Open the Serial Monitor to see the result. It will look like this:
※ NOTE THAT:
- Don't use pure water for testing because it doesn't let electricity flow, so it won't change the sensor's numbers.
- The sensor will never show a zero reading. It is normal for the sensor's numbers to be between 3100 and 2600, although this might change depending on how deep the sensor is placed, what the soil or water is made of, and the power supply voltage.
- Don't cover the circuit part (which is on top of the sensor) with soil or water, because this might damage the sensor.
Calibration for Capacitive Soil Moisture Sensor
The reading from the moisture sensor is not absolute. It changes based on what the soil is made of and how much water it has. In real use, we must calibrate the sensor to find the point where the soil is considered wet or dry.
How to calibrate:
- Upload the code to the Arduino Nano 33 IoT.
- Insert the moisture sensor into the soil.
- Insert the moisture sensor into the soil.
- Slowly water the soil.
- Open the Serial Monitor.
- Write down the number you see when the soil changes from dry to wet. This number is called THRESHOLD.
Determine if the soil is wet or dry
After calibrating, change the THRESHOLD value you recorded in the code below. This code checks if the soil is wet or dry.
The result is shown on the serial screen.