Arduino Nano 33 IoT - OLED 128x32 Display
This guide shows you how to use the Arduino Nano 33 IoT with an OLED display. We will learn these things:
- How to hook up an OLED 128x32 display with the Arduino Nano 33 IoT.
- How to program the Arduino Nano 33 IoT to show text and numbers on the OLED 128x32 display.
- How to program the Arduino Nano 33 IoT to center text and numbers in the middle of the OLED 128x32 display.
- How to program the Arduino Nano 33 IoT to draw on the OLED 128x32 display.
- How to program the Arduino Nano 33 IoT to display an image on the OLED 128x32 display.
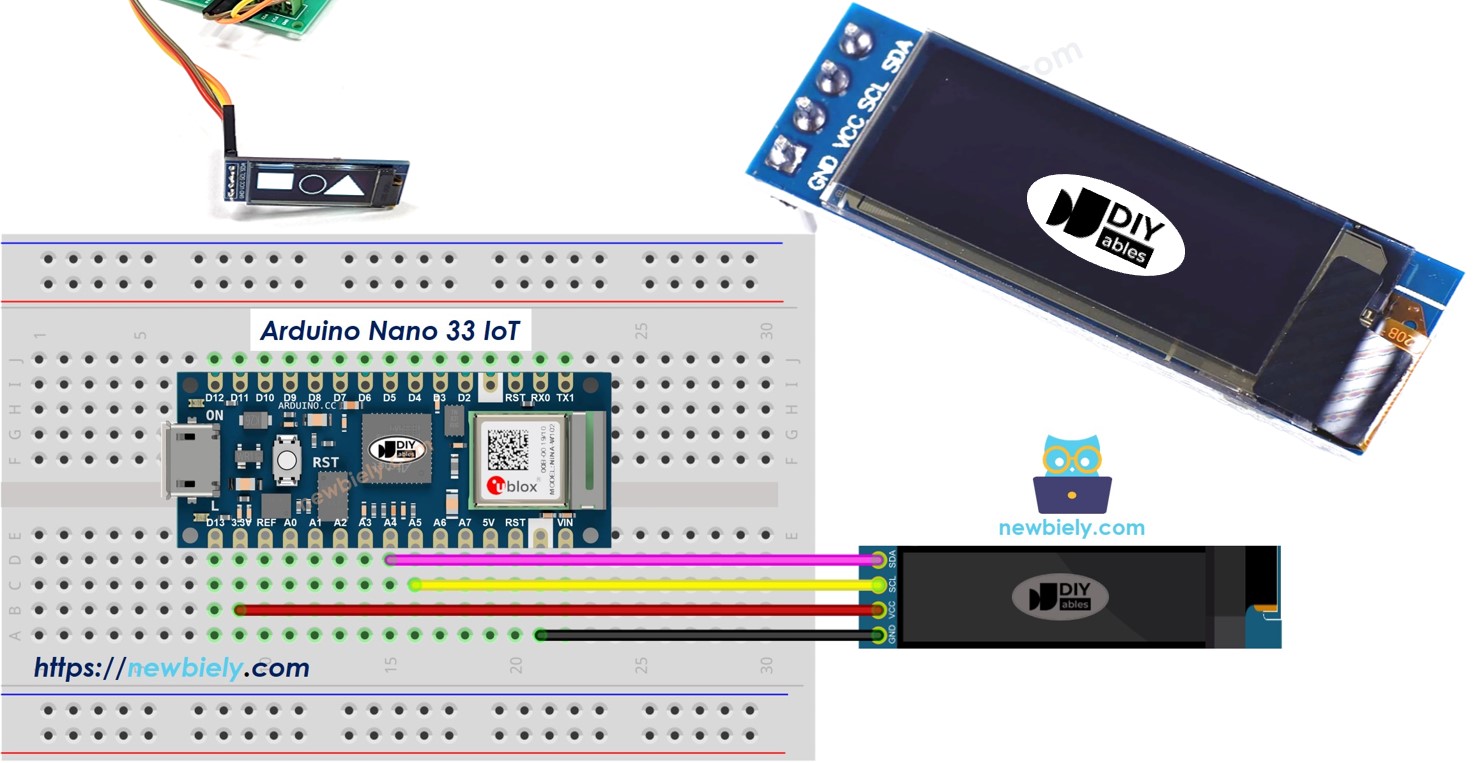
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of OLED Display
There are many types of OLED displays. The most common one used with Arduino Nano 33 IoT is the SSD1306 I2C OLED, available in sizes 128x64 and 128x32.
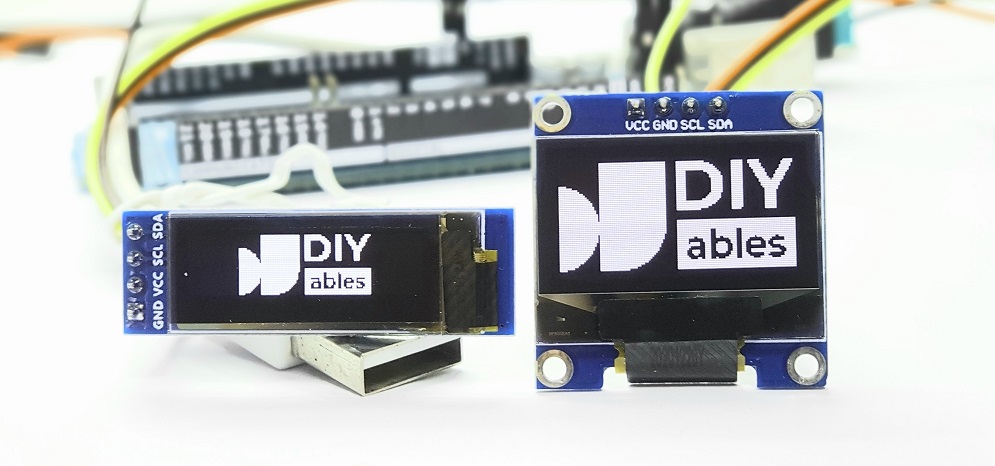
I2C OLED Display Pinout
- GND pin: Connect this pin to the ground (GND) of the Arduino Nano 33 IoT.
- VCC pin: This pin gives power to the display; connect it to the 3.3V or 5V supply.
- SCL pin: This is the clock pin used for I2C communication.
- SDA pin: This is the data pin used for I2C communication.
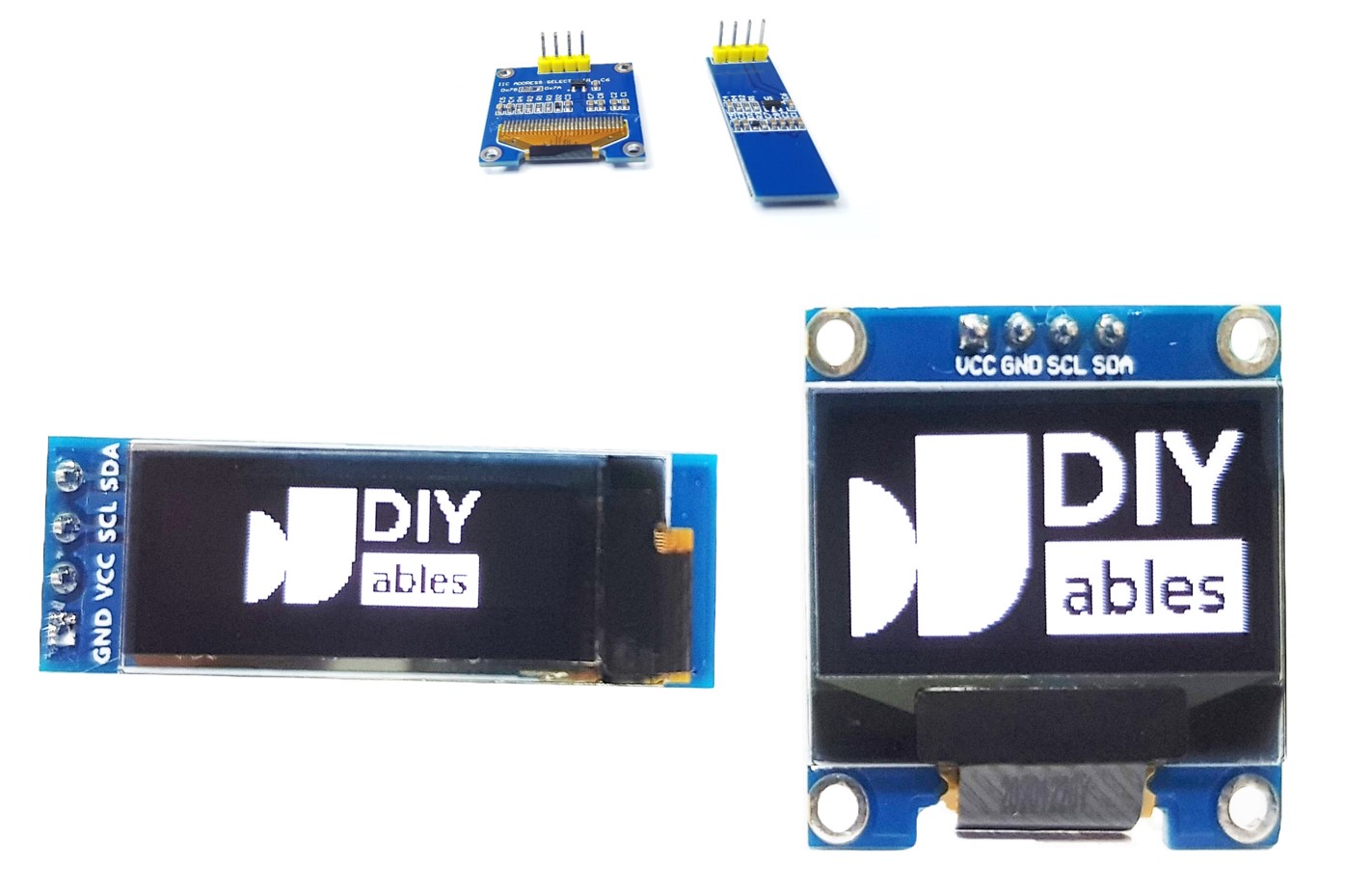
※ NOTE THAT:
- The connections on the OLED module might be different depending on who made it and its type. Please use the labels shown on your OLED module. Look carefully!
- This guide uses an OLED screen that works with the SSD1306 I2C driver. We tested it with the OLED display from DIYables and it works great.
Wiring Diagram
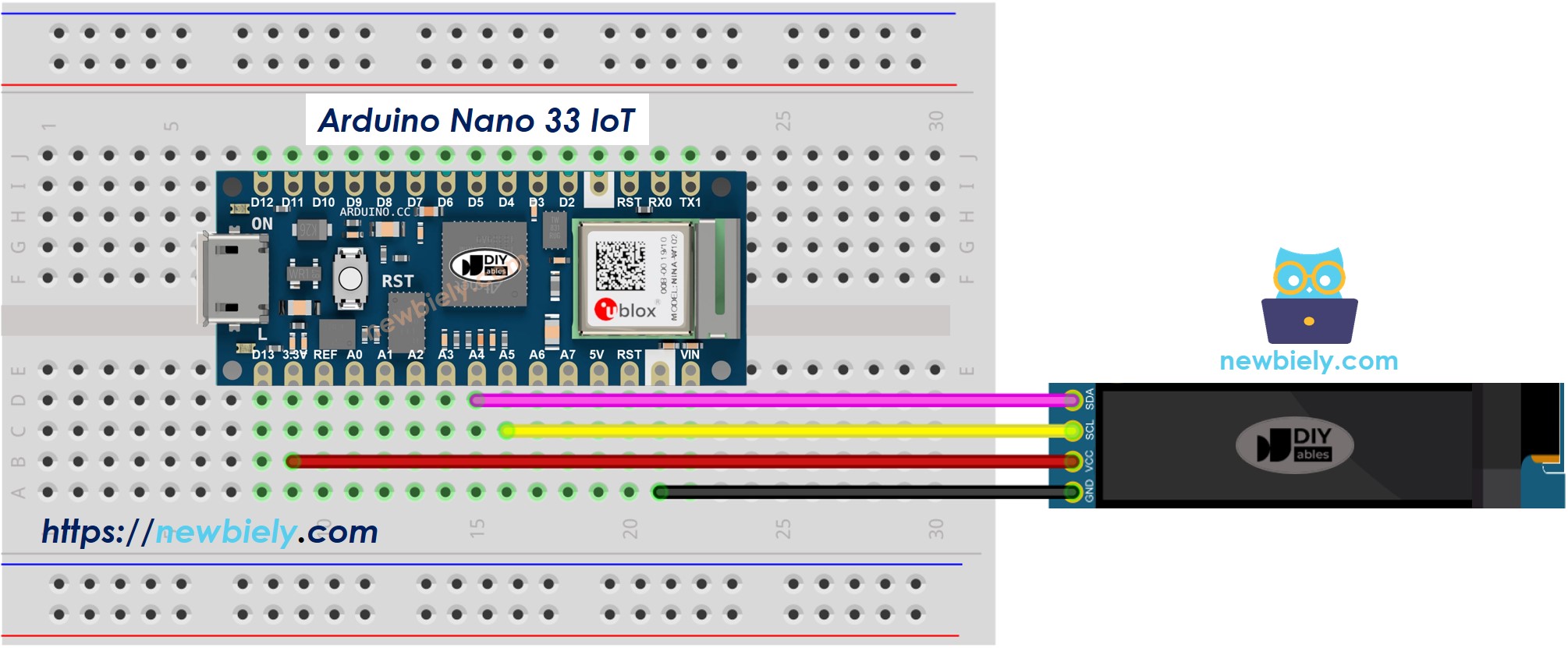
This image is created using Fritzing. Click to enlarge image
Wiring guide for connecting the Arduino Nano 33 IoT to the OLED display:
OLED Module | Arduino Nano 33 IoT |
---|---|
Vin | 3.3V |
GND | GND |
SDA | A4 |
SCL | A5 |
How To Use OLED with Arduino Nano 33 IoT
Install SSD1306 OLED library
- Click on the Libraries icon on the left side of the Arduino IDE.
- Search for SSD1306 and find the SSD1306 library made by Adafruit.
- Then, click the Install button to finish installing.
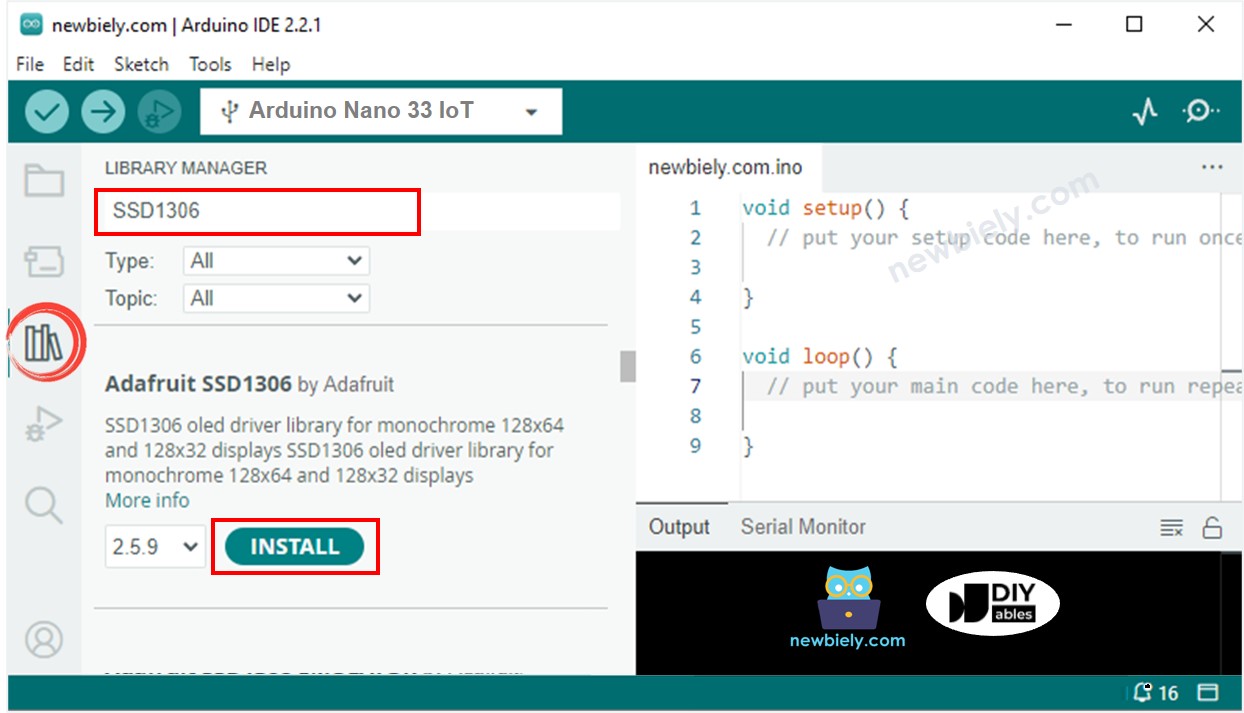
- You might see a message asking you to install extra library files.
- To add all of them, click the Install All button.
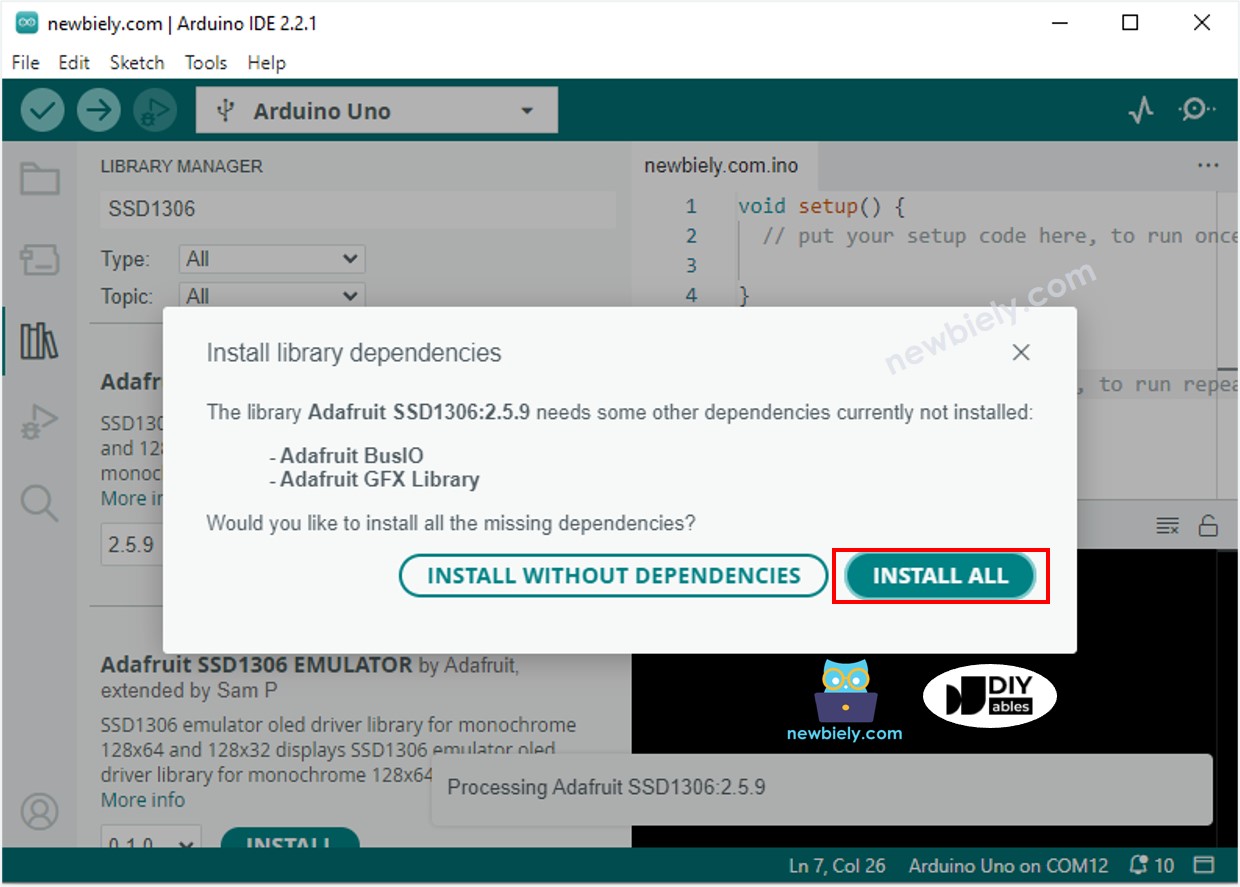
How to program for OLED
- Add the library
- Set the OLED screen size to 128 by 32.
- Make a new SSD1306 OLED item.
- In the setup() function, start the OLED display.
- Then you can show text, images, and draw a line.
Arduino Nano 33 IoT Code - Display Text on OLED
Here are some functions that can show text on the OLED:
- Oled.clearDisplay() – Turns off every pixel on the screen.
- Oled.drawPixel(x, y, color) – Draws a pixel at the specified x and y position.
- Oled.setTextSize(n) – Sets how big the text will be; you can choose a size between 1 and 8.
- Oled.setCursor(x, y) – Moves the starting point for writing text to the x and y coordinates.
- Oled.setTextColor(WHITE) – Sets the text color to white.
- Oled.setTextColor(BLACK, WHITE) – Sets the text color to black and the background color to white.
- Oled.println("message") – Writes a text message on the screen.
- Oled.println(number) – Writes a number on the screen.
- Oled.println(number, HEX) – Writes a number in hexadecimal (base 16) format.
- Oled.display() – Updates the screen so that your changes appear.
- Oled.startscrollright(start, stop) – Scrolls the text from left to right between the chosen start and stop points.
- Oled.startscrollleft(start, stop) – Scrolls the text from right to left between the chosen start and stop points.
- Oled.startscrolldiagright(start, stop) – Scrolls the text from the bottom left to the top right.
- Oled.startscrolldiagleft(start, stop) – Scrolls the text from the bottom right to the top left.
- Oled.stopscroll() – Stops the scrolling text.
How to vertical and horizontal center align text/number on OLED
If you want to learn how to center text and numbers on an OLED display both vertically and horizontally, please check out this guide: How to vertical/horizontal center on OLED.
Arduino Nano 33 IoT Code - Drawing on OLED
Arduino Nano 33 IoT Code – Display Image
To show a picture on an OLED, you need to first change the image (in any format) into a bitmap array. You can do this using an online tool. The picture below shows how to convert an image into a bitmap array; I used the Arduino Nano 33 IoT icon as an example.
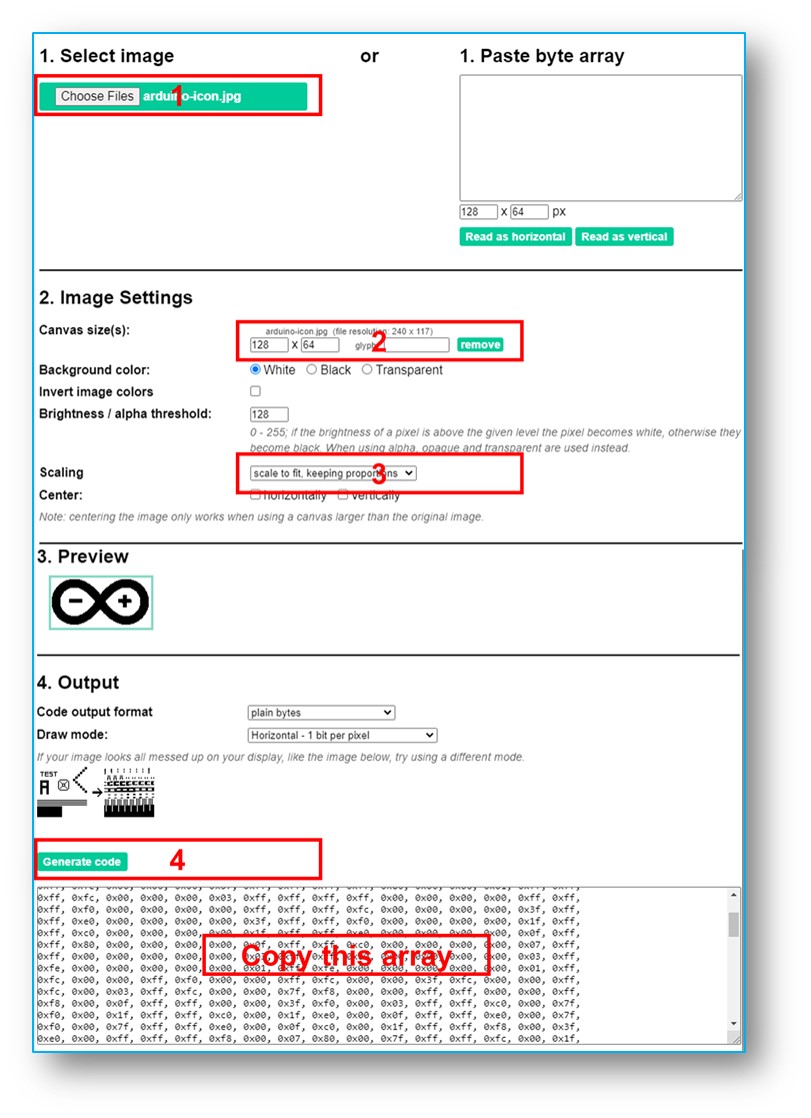
After converting, copy the new array code and replace the ArduinoIcon array in the code below.
※ NOTE THAT:
The image should not be bigger than the screen. You need to make the image smaller and change the width and height settings in the oled.drawBitmap() function.
OLED Troubleshooting
Make sure the OLED works correctly by doing these steps:
- Make sure your wires are connected correctly.
- Check that your OLED has the SSD1306 driver.
- Run the I2C Address Scanner code on your Arduino Nano 33 IoT to find the OLED's I2C address.
The result shown on the Serial Monitor is as follows: