Arduino Nano 33 IoT - LED Matrix
A LED matrix display, also called LED display or dot matrix display, is used a lot. In this tutorial, you will learn:
- How to hook up the Arduino Nano 33 IoT to an 8×8 LED display grid
- How to hook up the Arduino Nano 33 IoT to a 32×8 LED display grid
- How to program Arduino Nano 33 IoT to show letters, numbers, and simple animations on the LED display grid using the Arduino Nano 33 IoT
After that, you can easily change the code for other LED displays like a 16x8 LED display or a 64x8 LED display.
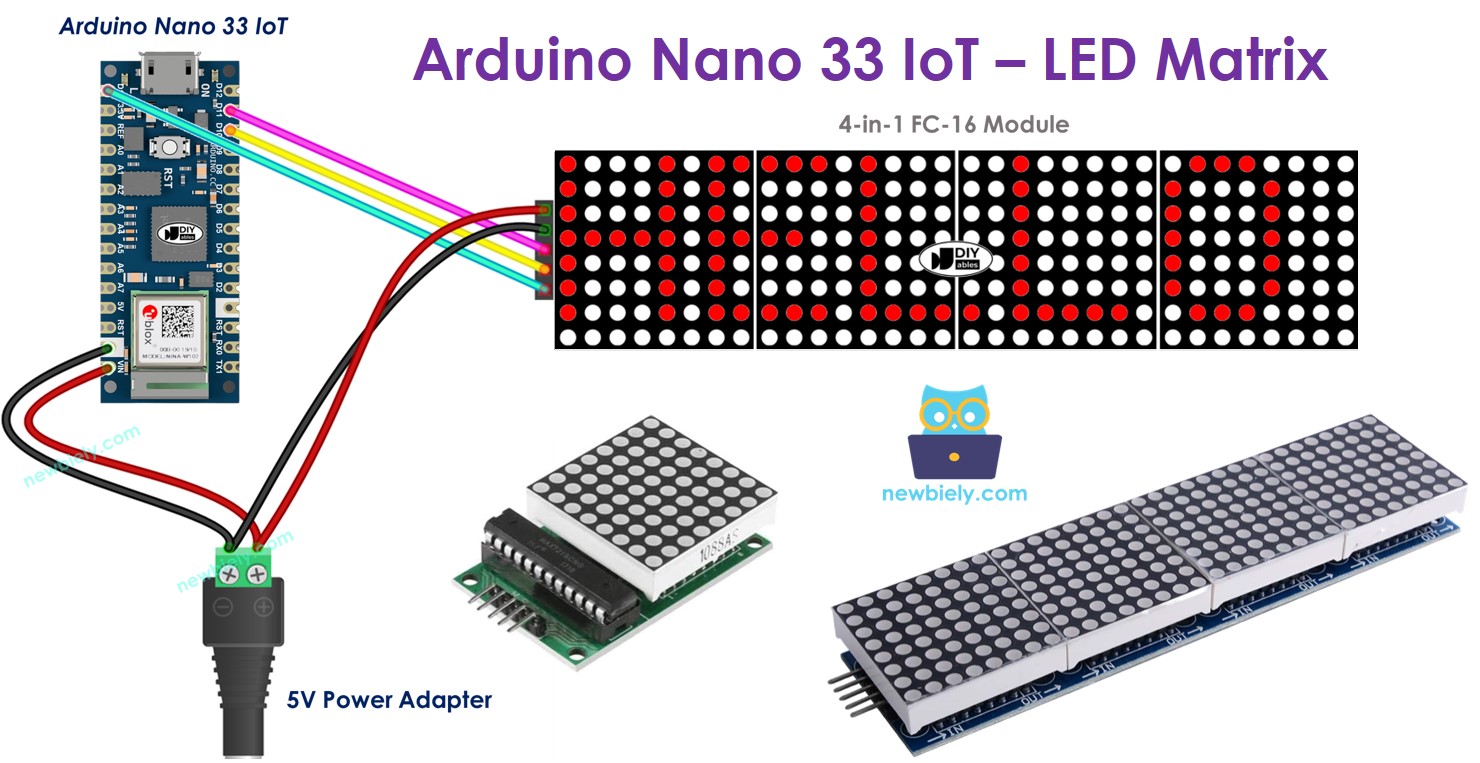
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of LED Matrix
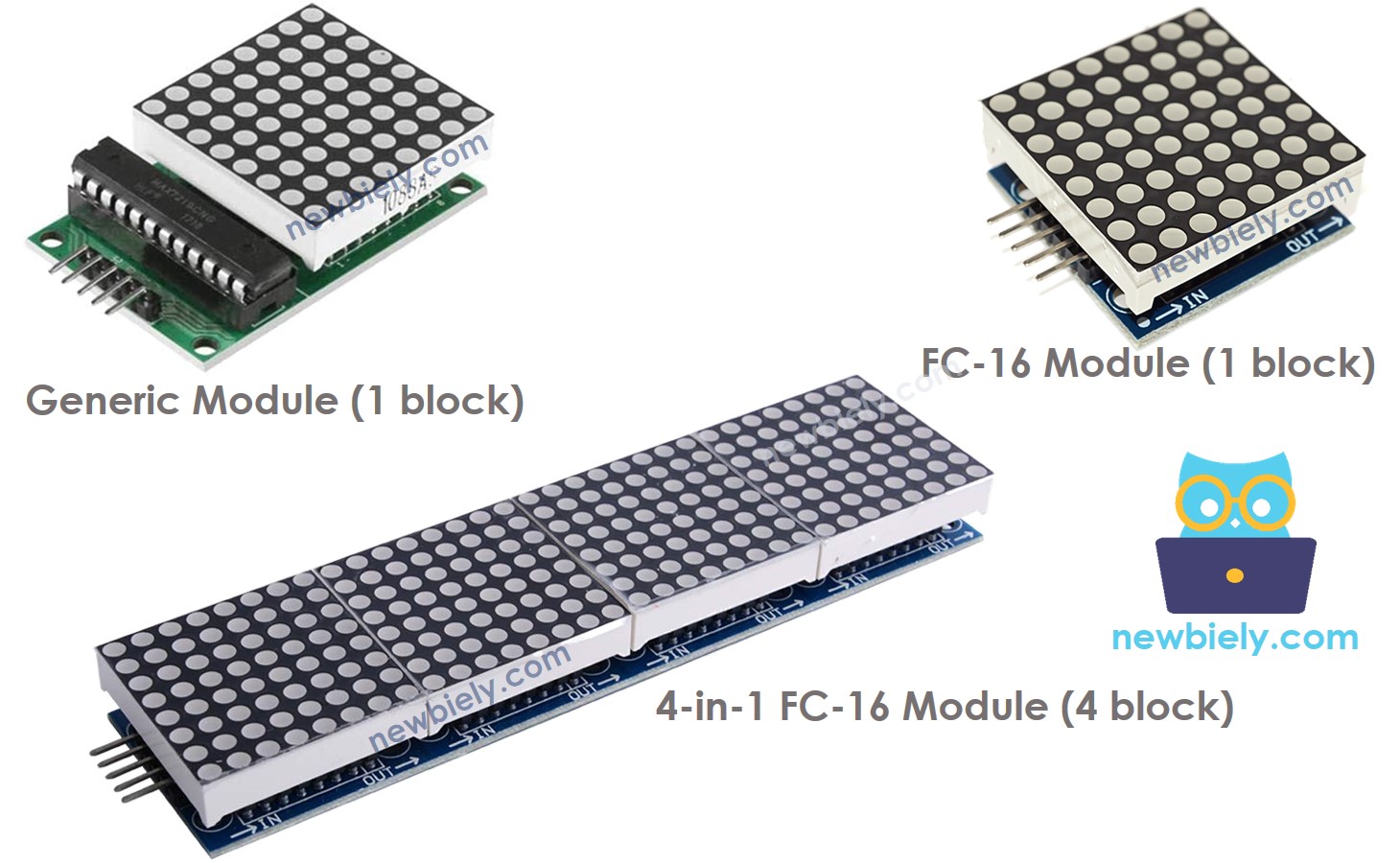
There are many types of LED matrix displays. When using the Arduino Nano 33 IoT, the LED matrix that uses the MAX7219 chip is very popular. This MAX7219 LED matrix has these features:
- The basic part of an LED matrix is called a block.
- Each block has an 8x8 LED grid (64 LEDs) and a MAX7219 chip that controls them.
- There are two common types of blocks: the generic module and the FC-16 module.
- An LED matrix can be made of one block or many blocks connected together.
- You can buy a ready-made LED matrix that has many blocks (for example, a 4-in-1 or an 8-in-1).
- You can also buy individual blocks and connect them yourself to make an LED matrix of the size you want.
- You must set the size of the LED matrix in the Arduino Nano 33 IoT code.
Pinout
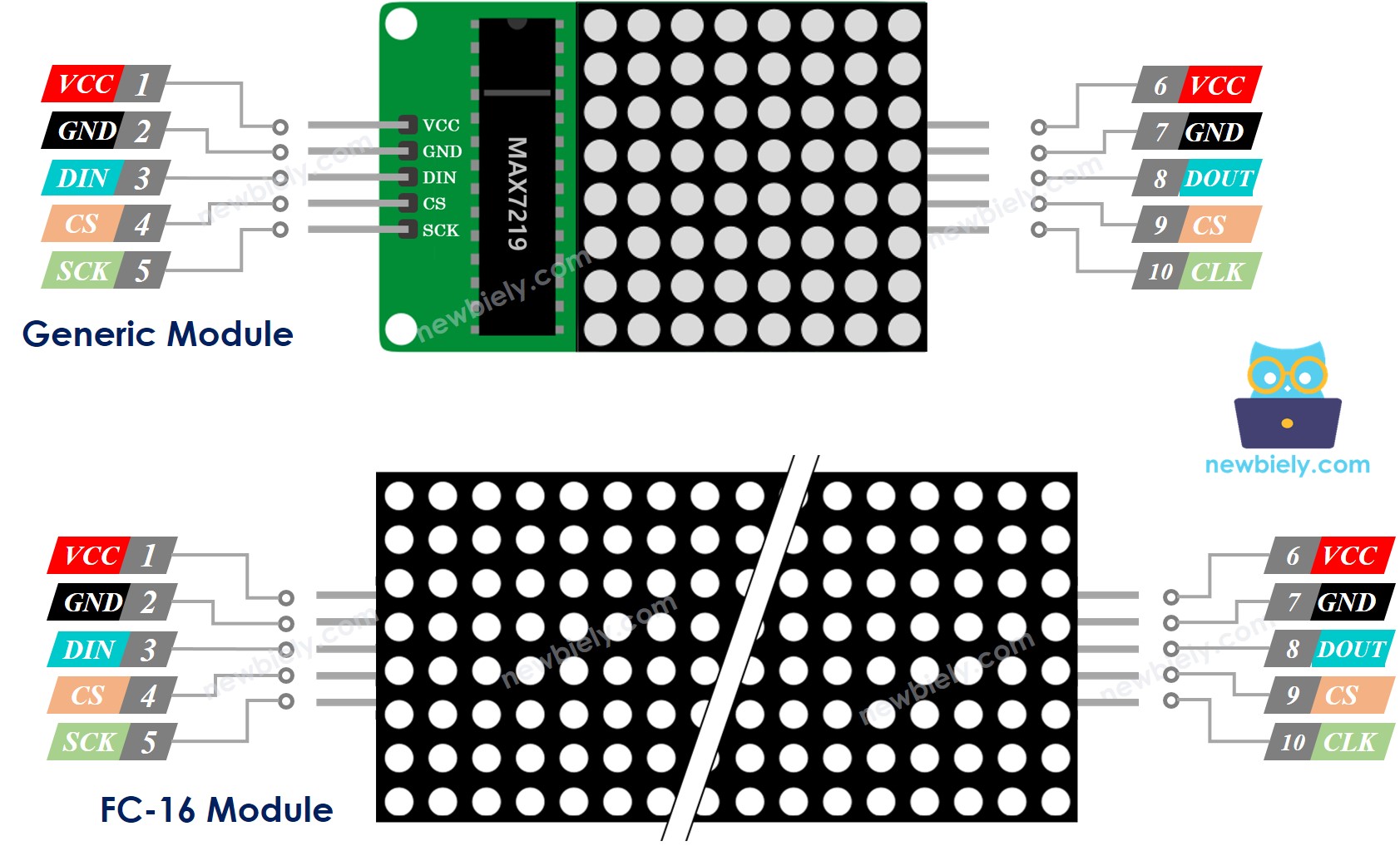
A LED Matrix is made of one or more blocks. Each block has two sets of pins:
- Input Pins Group:
- VCC: Connects to 5V.
- GND: Connects to ground.
- DIN: This is the Data pin. Connect it to any digital pin on the Arduino Nano 33 IoT.
- CS: This stands for Chip Select. Connect it to any digital pin on the Arduino Nano 33 IoT.
- CLK: This is the Clock pin. Connect it to any digital pin on the Arduino Nano 33 IoT.
- Output Pins Group:
- VCC: Connects to the VCC pin of the input group on the next module.
- GND: Connects to the ground (GND) pin of the input group on the next module.
- DOUT: This is the Data Out pin. Connect it to the DIN pin of the input group on the next module.
- CS: Connects to the CS pin of the input group on the next module.
- CLK: Connects to the CLK pin of the input group on the next module.
Wiring Diagram
If the LED screen is built as one piece:
- Attach the input pin groups to the Arduino Nano 33 IoT.
- Leave the output pin group disconnected.
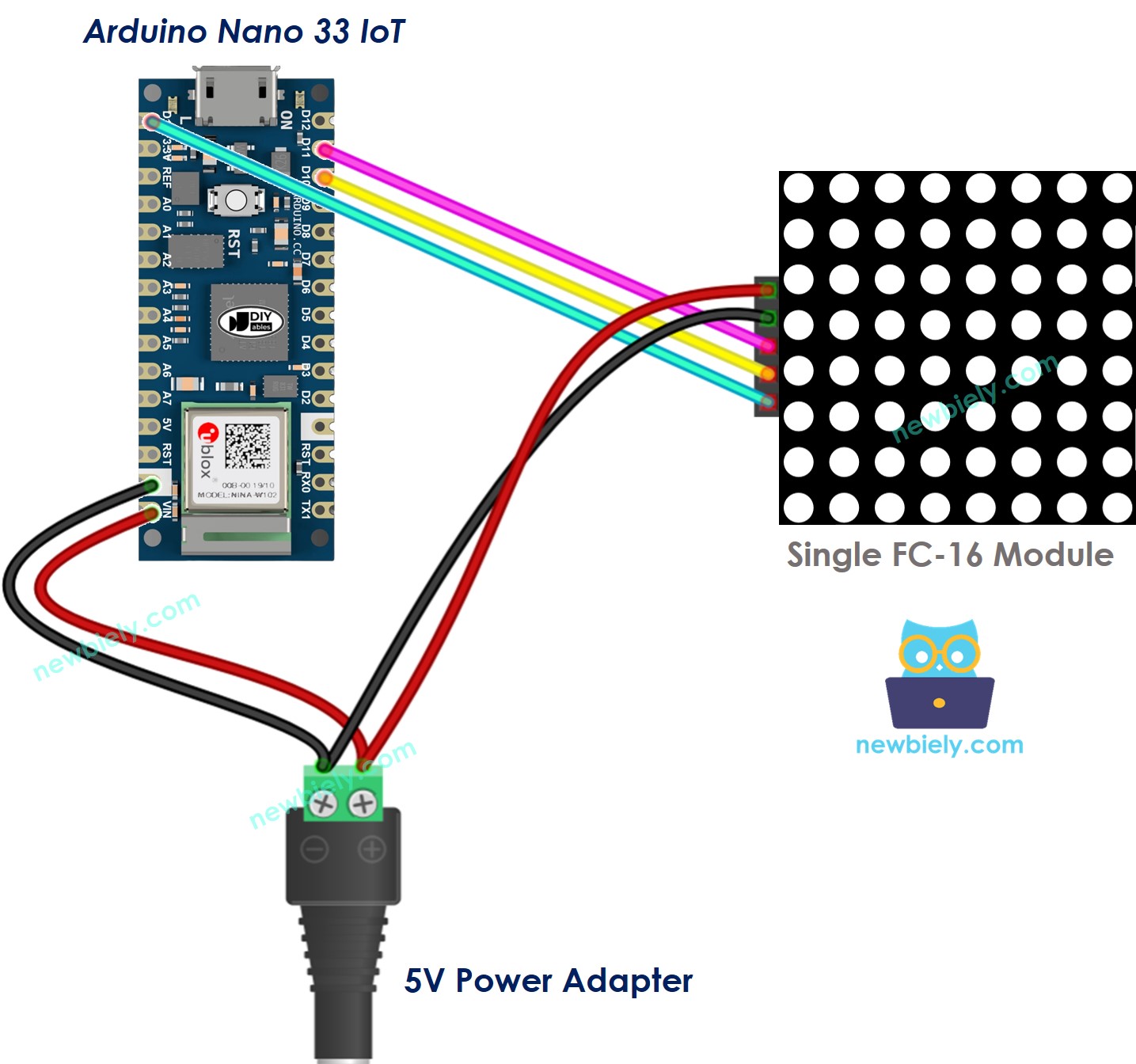
This image is created using Fritzing. Click to enlarge image
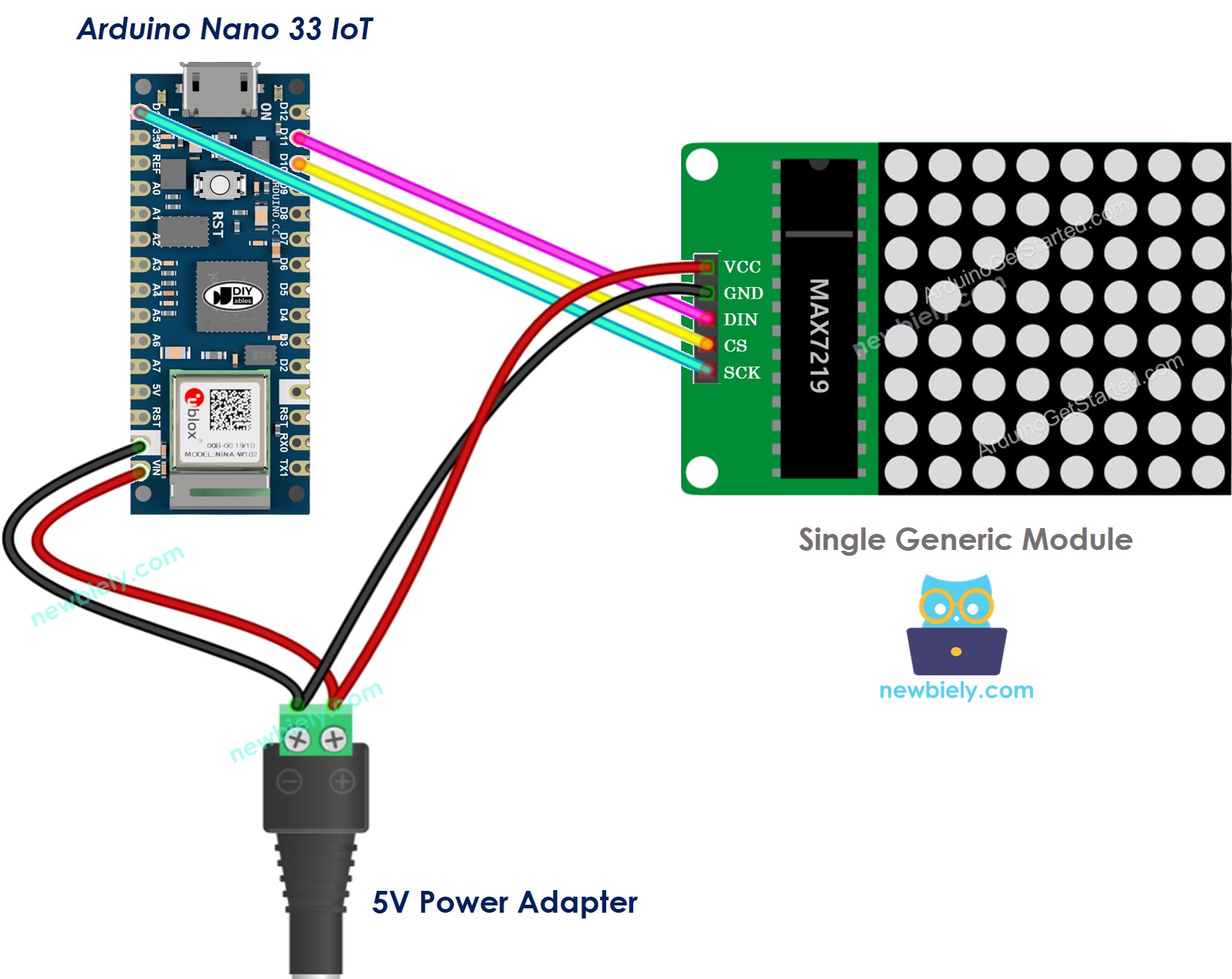
This image is created using Fritzing. Click to enlarge image
If the LED matrix is made of several pre-built blocks:
- Connect the group of input pins to the Arduino Nano 33 IoT.
- Leave the group of output pins not connected.
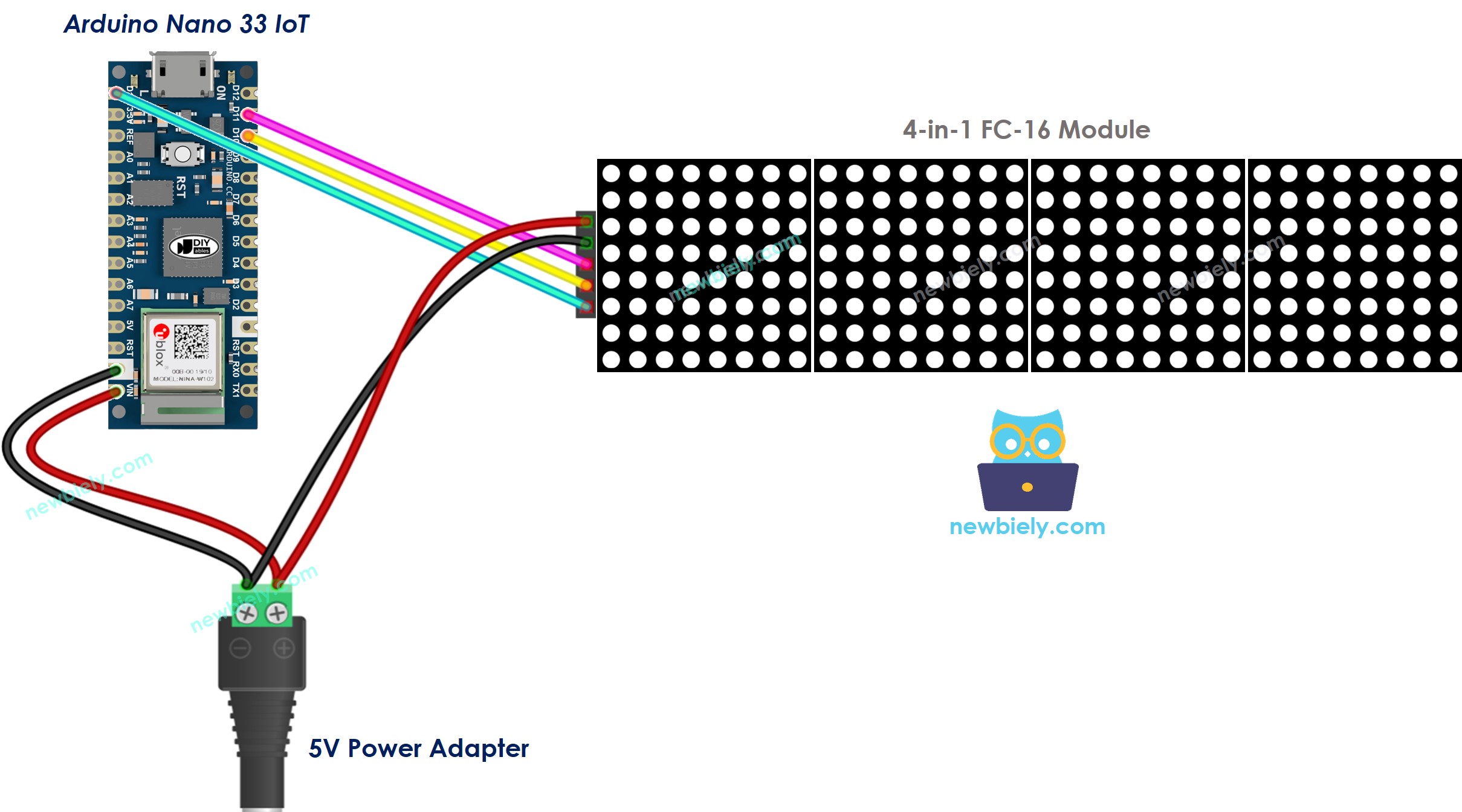
This image is created using Fritzing. Click to enlarge image
If you built the LED matrix from several blocks by yourself:
- Attach the first block's input pins to the Arduino Nano 33 IoT.
- Connect the output pins of each block to the input pins of the next block.
- Leave the output pins of the last block unconnected.
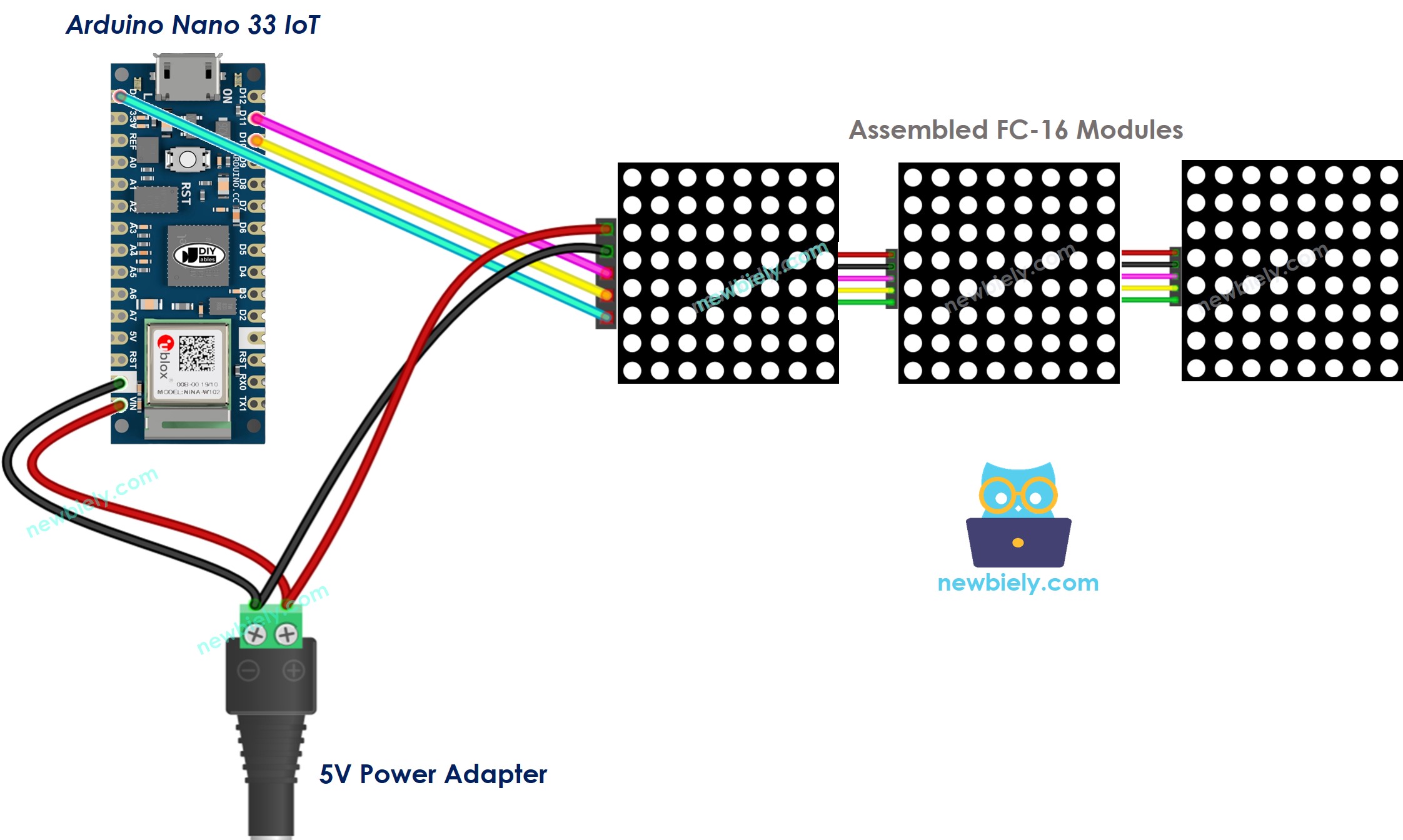
This image is created using Fritzing. Click to enlarge image
Because the screen uses a lot of power (up to 1 amp at full brightness):
- Don't use the power from the Arduino Nano 33 IoT’s 5V pin.
- Instead, use an external 5V power supply. Both the Arduino Nano 33 IoT and the LED matrix can get power from the same 5V adapter.
Because the Arduino Nano 33 IoT connects to the LED matrix using SPI pins.
- Use D13 (SCK) and D11 (MOSI) on the Arduino Nano 33 IoT board.
- You can change Pin D10 (CS) to any other pin you want.
How To Program For LED Matrix
Controlling the LED matrix is not simple. Luckily, there are libraries to help make it easier. Here is a simple guide on how to write Arduino Nano 33 IoT code to control the LED matrix.
- Add libraries:
- Choose the hardware you are using: GENERIC_HW or FC16_HW.
- Count the number of LED blocks used. For example, a 4-in-1 LED matrix has 4 blocks.
- Choose the pin that connects to the LED matrix's chip select (CS) pin. For example, use pin D10.
- Make a new MD_Parola object for the LED matrix display.
- Write the instructions inside the setup() function:
- Show text, numbers, and animated effects. Check the next section.
Arduino Nano 33 IoT - LED Matrix Code
This code is made for a 32x8 FC-16 LED matrix display (4 parts). You can also change it easily to work with 8x8, 16x8, or 64x8 displays.
Detailed Instructions
If you are new to the Arduino Nano 33 IoT, be sure to check out our Getting Started with Arduino Nano 33 IoT tutorial. Then, follow these steps:
- Connect the components to the Arduino Nano 33 IoT board as depicted in the diagram.
- Use a USB cable to connect the Arduino Nano 33 IoT board to your computer.
- Launch the Arduino IDE on your computer.
- Select the Arduino Nano 33 IoT board and choose its corresponding COM port.
- Click the Library Manager icon on the left side of the Arduino IDE.
- Type MD_Parola in the search box and find the MD_Parola library.
- Click the Install button.
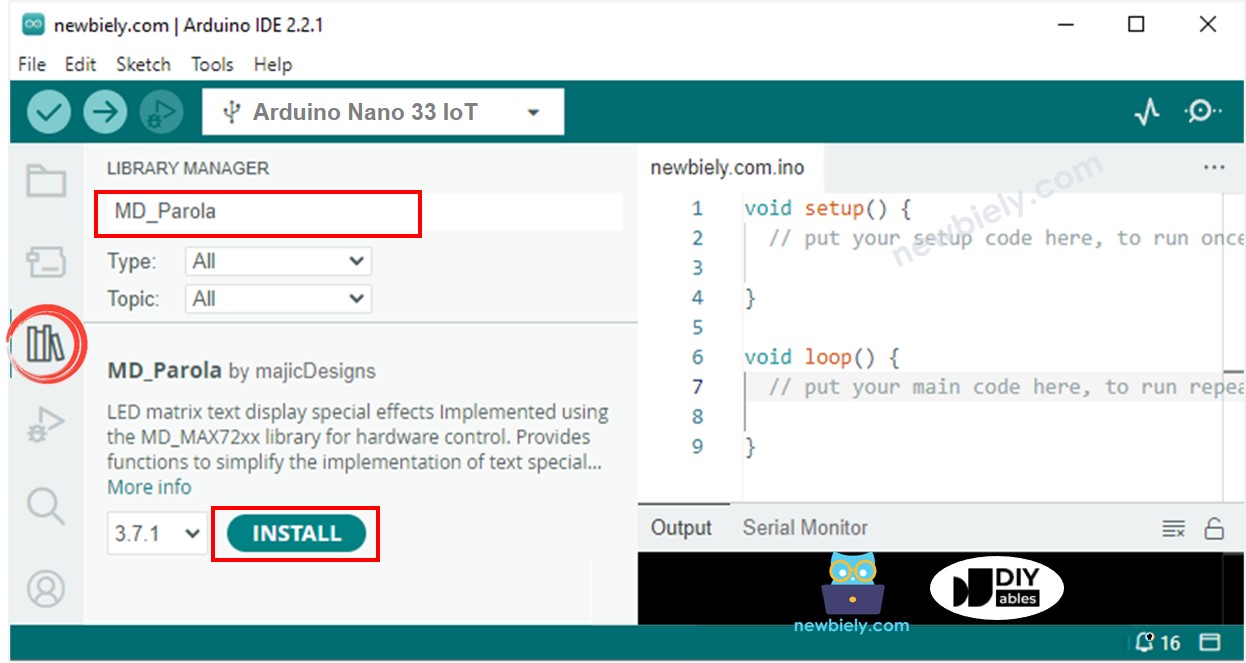
- You may need to add the MD_MAX72XX library.
- Click the Install All button to add the necessary files.
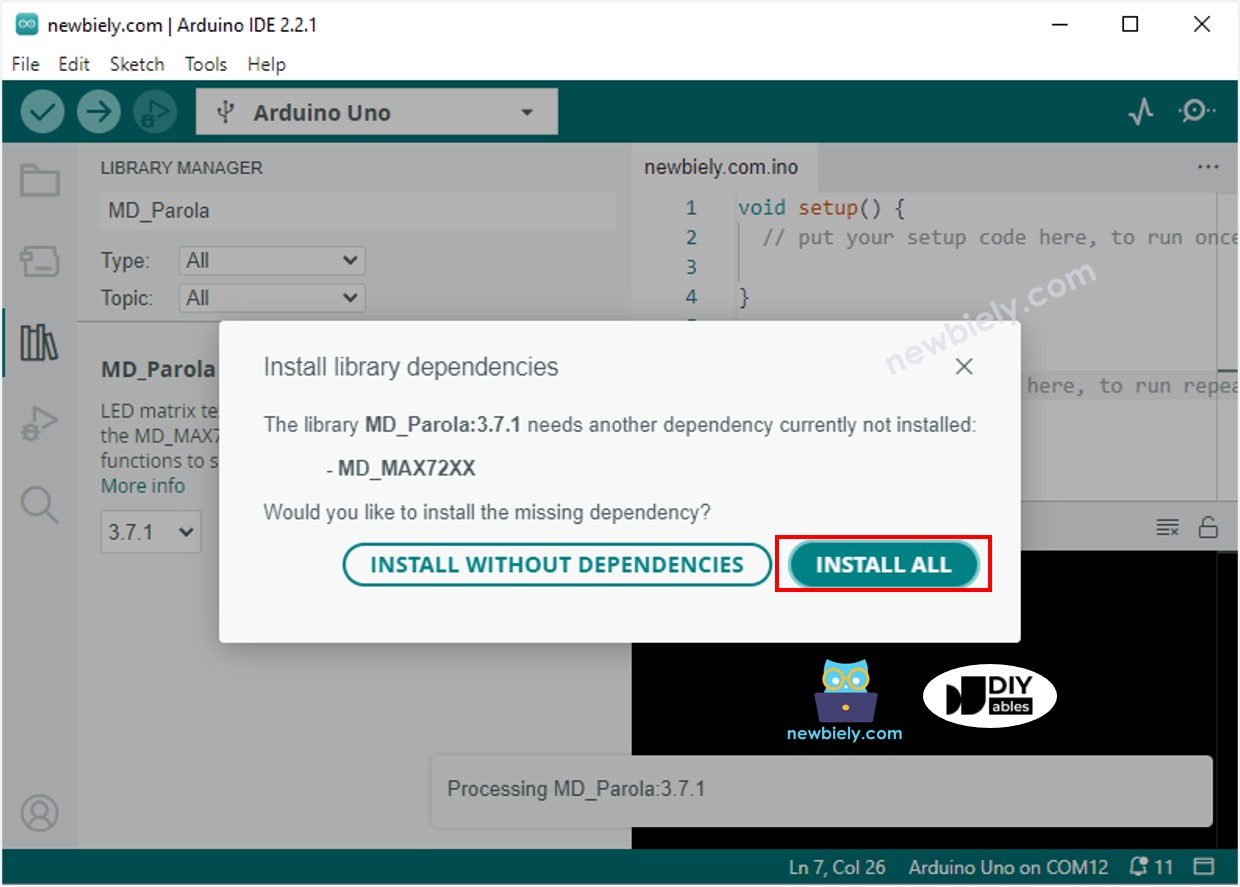
- Copy the code above and open it in Arduino IDE.
- Click the Upload button in Arduino IDE to send the code to your Arduino Nano 33 IoT.
- Watch the LED matrix display light up.
Arduino Nano 33 IoT LED Matrix Code – Scrolling Text
If you want to show a long message that doesn't fit on an LED display, you can use a scrolling text effect.
Here is the Arduino Nano 33 IoT code that shows you how to scroll text on an LED display.
To see more text effects, please check out the MD_Parola Library Reference.