Arduino Nano 33 IoT - Button - Long Press Short Press
This guide explains how to use the Arduino Nano 33 IoT to tell the difference between a long press and a short press. In simple terms, you will learn:
- How to check if the button is pressed quickly
- How to check if the button is pressed for a long time
- How to check if the button is pressed quickly or for a long time
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Wiring Diagram
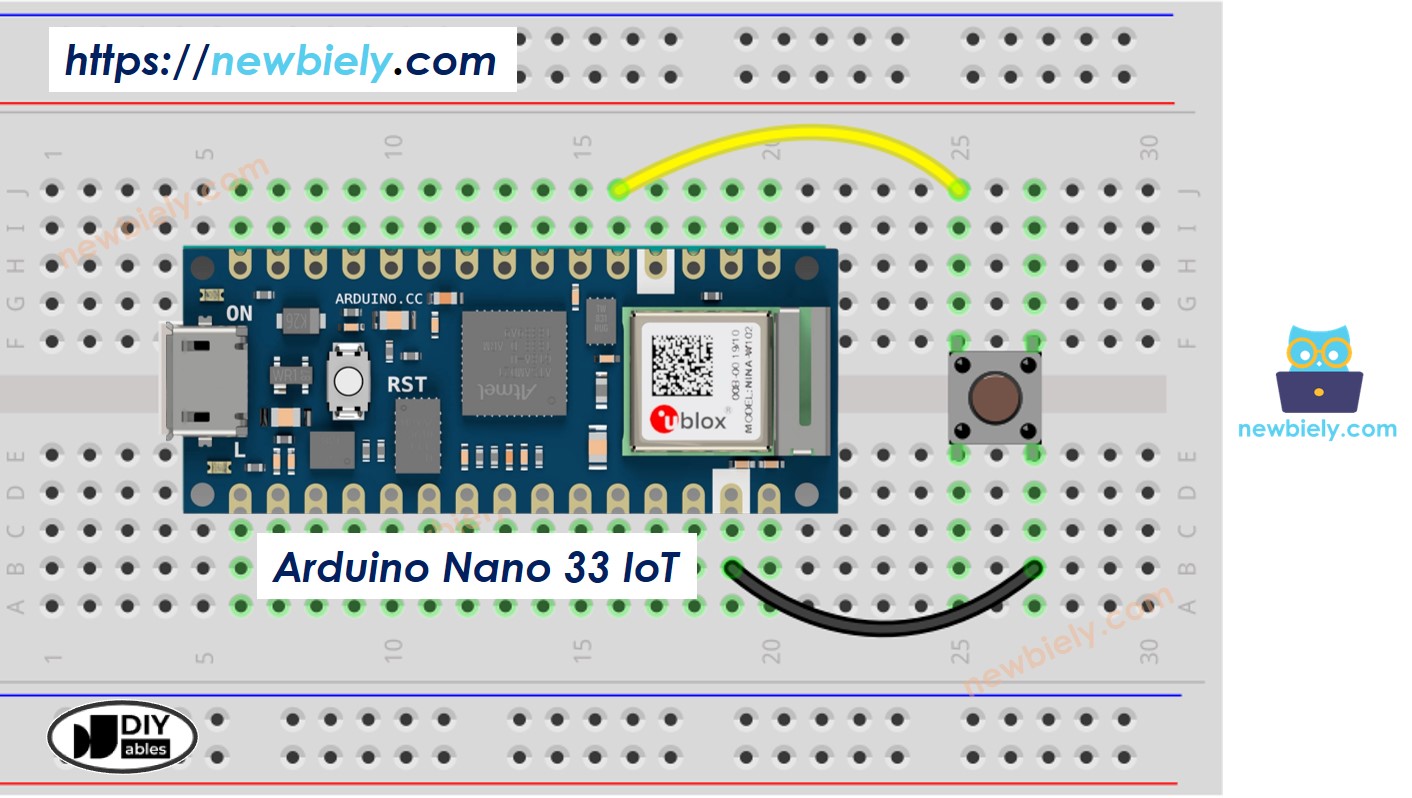
This image is created using Fritzing. Click to enlarge image
This guide uses the built-in pull-up resistor. The button is normally HIGH and goes LOW when pressed.
※ NOTE THAT:
Please note that the Arduino Nano 33 IoT pins A4 and A5 have built-in pull-up resistors for I2C communication. Although these pins can be used as digital input pins, it is recommended to avoid using them for digital input. If you must use them, do NOT use internal or external pull-down resistors for these pins
How To Detect Short Press
- Measure the time from when the button is pressed until it is released. If this time is shorter than the set amount, it counts as a short press.
Let's go through it one step at a time.
- Set the maximum time a short press can last.
- Check if the button is pressed and record the time it was pressed.
- Detect when the button is no longer pressed and record the time.
- Find out how long the button is pressed.
- Figure out if the button press is short by comparing how long it lasted to the set short press time.
Arduino Nano 33 IoT Code for detecting the short press
Detailed Instructions
If you are new to the Arduino Nano 33 IoT, be sure to check out our Getting Started with Arduino Nano 33 IoT tutorial. Then, follow these steps:
- Connect the components to the Arduino Nano 33 IoT board as depicted in the diagram.
- Use a USB cable to connect the Arduino Nano 33 IoT board to your computer.
- Launch the Arduino IDE on your computer.
- Select the Arduino Nano 33 IoT board and choose its corresponding COM port.
- Upload the code above to your Arduino Nano 33 IoT using the Arduino IDE.
- Press the button quickly a few times.
- Look at the result on the Serial Monitor. It should look like this:
※ NOTE THAT:
The Serial Monitor might show several short presses even when you press the button once. This is normal and is called the "chattering phenomenon." Later in this tutorial, we will explain how to fix this problem.
How To Detect Long Press
There are two situations where you might check for a long press.
- The long press is noticed right after you let go of the button.
- The long press is noticed while you are still holding the button.
For the first example:
- Measure how long the press lasts. If it goes on longer than a set time, it counts as a long press.
In the second case, while the button is pressed, repeat the following steps:
- Check how long the button is pressed.
- If it is pressed longer than the set time, it counts as a long press.
- Otherwise, keep checking until the button is released.
Arduino Nano 33 IoT Code for detecting long press when released
Detailed Instructions
- Upload the code to the Arduino Nano 33 IoT using the Arduino IDE.
- After one second, press the button and then let go.
- Look at the result on the Serial Monitor. It should look like the image below.
Arduino Nano 33 IoT Code for detecting long press during pressing
Detailed Instructions
- Load the code above onto your Arduino Nano 33 IoT using the Arduino IDE.
- Wait a few seconds, then press and release the button.
- Open the Serial Monitor to see the result. It should look like this.
How To Detect Both Long Press and Short Press
Short Press and Long Press after released
Detailed Instructions
- Use Arduino IDE to upload the code to your Arduino Nano 33 IoT.
- Press the button quickly and then hold it down.
- Open the Serial Monitor to see the result. It should look like the example shown.
※ NOTE THAT:
The Serial Monitor might show several quick presses when you hold the button down. This is normal and is called the bouncing effect. We will explain how to fix it at the end of this guide.
Short Press and Long Press During pressing
Detailed Instructions
- Upload the code above to the Arduino Nano 33 IoT using the Arduino IDE.
- Press the button quickly and then press it for a longer time.
- Open the Serial Monitor to see the result. It should look like this:
Long Press and Short Press with Debouncing
It is very important to make sure the button doesn't send extra signals in many uses.
Debouncing can be tricky, especially when you use several buttons. To make it easier for beginners, we created a library called ezButton.
We will use this library in the code below.
Short Press and Long Press with debouncing after released
Detailed Instructions
- Install the ezButton library. For instruction, visit ezButton Library Reference
- Upload the code to your Arduino Nano 33 IoT using the Arduino IDE.
- Press the button briefly and press it for a long time.
- Look at the Serial Monitor to see the results, which should look similar to the example below.
Short Press and Long Press with debouncing During Pressing
Detailed Instructions
- Copy and upload the code above to your Arduino Nano 33 IoT using the Arduino IDE.
- Press the button briefly and then press it for a longer time.
- Look at the result in the Serial Monitor. It will appear similar to the example shown below.
Video Tutorial
Why Needs Long Press and Short Press
- Use one button to do many tasks and reduce the number of digital input pins. For example, you can set a short press to turn on the light and a long press to start the fan.
- Choose a long press instead of a short press to stop accidental button presses. Some devices use the button for a full reset, and pressing it by mistake could be risky.