Raspberry Pi - Button
This tutorial instructs you how to use Raspberry Pi with button. In detail, we will learn:
How to connect the button to Raspberry Pi
How to program Raspberry Pi to read the state of the button
How to program Raspberry Pi to detect the pressing and releasing events from button.
How to prevent the floating input problem when using the button with Raspberry Pi.
How to prevent the chattering problem when using the button with Raspberry Pi.
The button is referred to as a pushbutton, tactile button or momentary switch. It is a fundamental component and is used in many Raspberry Pi projects. It is easy to use. However, it may be perplexing for beginners due to mechanical, physical aspects and how it is used. This tutorial simplifies it for beginners.
Or you can buy the following kits:
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand,
DIYables .
When utilizing a button, beginners often encounter two common difficulties:
1. Floating input problem:.
Symptom: The value read from the input pin does not correspond to the state of the button press.
Cause: A pull-up or pull-down resistor is not in use on the input pin.
Solution: Use a pull-up or pull-down resistor, which will be covered in this tutorial..
2. Chattering phenomenon:.
Symptom: The Raspberry Pi code is detecting multiple button presses even when the button is pressed only once.
Cause: Mechanical and physical issues are causing the state of the button (or switch) to swiftly alternate between LOW and HIGH several times..
For applications that demand exact press detection, this is a consideration that should be made.
※ NOTE THAT:
Do not confuse the button with the following:
There are various types of push buttons, which can be broadly categorized into two groups:
Nevertheless, these pins are linked together internally in pairs. Consequently, we only need to utilize two of the four pins, which are not connected internally.
There are four methods of connecting to the button, two of which are symmetrical (refer to the image).
? Why is it that we only utilize two pins of a button, when it has four pins?
⇒ To ensure that it remains securely in place on the PCB (printed circuit board) and can withstand any pressure applied.
image source: diyables.io
GND: Connect this pin to ground.
VCC: Connect this pin to a 3.3V power supply.
OUT: Connect this pin to a digital input on your Raspberry Pi.
With this configuration, the module outputs LOW when the button is not pressed and outputs HIGH when the button is pressed.
When the button is not pressed, pin A and pin B are not linked.
However, when the button is pressed, pin A and pin B become connected.
One button's pin is connected to either VCC or GND. The other pin of the same button is linked to a Raspberry Pi pin. By checking the status of a Raspberry Pi pin set as an input, we can determine whether or not a button has been pressed.
The connection between the button and Raspberry Pi, as well as the configuration of the Raspberry Pi's pin, will determine the relationship between the button state and the pressing state.
There are two ways to use a button with Raspberry Pi:
Connect one button's pin to VCC and the other to a Raspberry Pi's pin with a pull-down resistor
When the button is pressed, the Raspberry Pi's pin state will be HIGH. Otherwise, it will be LOW
An internal or external resistor can be used. The internal resistor is built into the Raspberry Pi, and can be set via code.
Connect one button's pin to GND and the other to a Raspberry Pi's pin with a pull-up resistor
When the button is pressed, the Raspberry Pi's pin state will be LOW. Otherwise, it will be HIGH
An internal or external resistor can be used. The internal resistor is built into the Raspberry Pi, and can be set via code.
※ NOTE THAT:
When neither a pull-down nor a pull-up resistor is used, the input pin will enter a “floating” state when the button is not pressed, meaning that the state can be either HIGH or LOW (unstable) and will lead to incorrect detection.
The worst practice: initializes the Raspberry Pi pin as an input (by using GPIO.setup(BUTTON_PIN, GPIO.IN, pull_up_down=GPIO.PUD_OFF)) and does NOT use any external pull-down/pull-up resistor.
The best practice: initializes the Raspberry Pi pin as an internal pull-up input (by using GPIO.setup(BUTTON_PIN, GPIO.IN, pull_up_down=GPIO.PUD_UP)). It does NOT need to use any external pull-down/pull-up resistor.
For the convenience of newbies, this tutorial uses the most straightforward approach: setting up the Raspberry Pi pin as an internal pull-up input without the need of an external resistor. No need to worry about how to connect the pull-up/pull-down resistor. All that is required is to use the Raspberry Pi code.
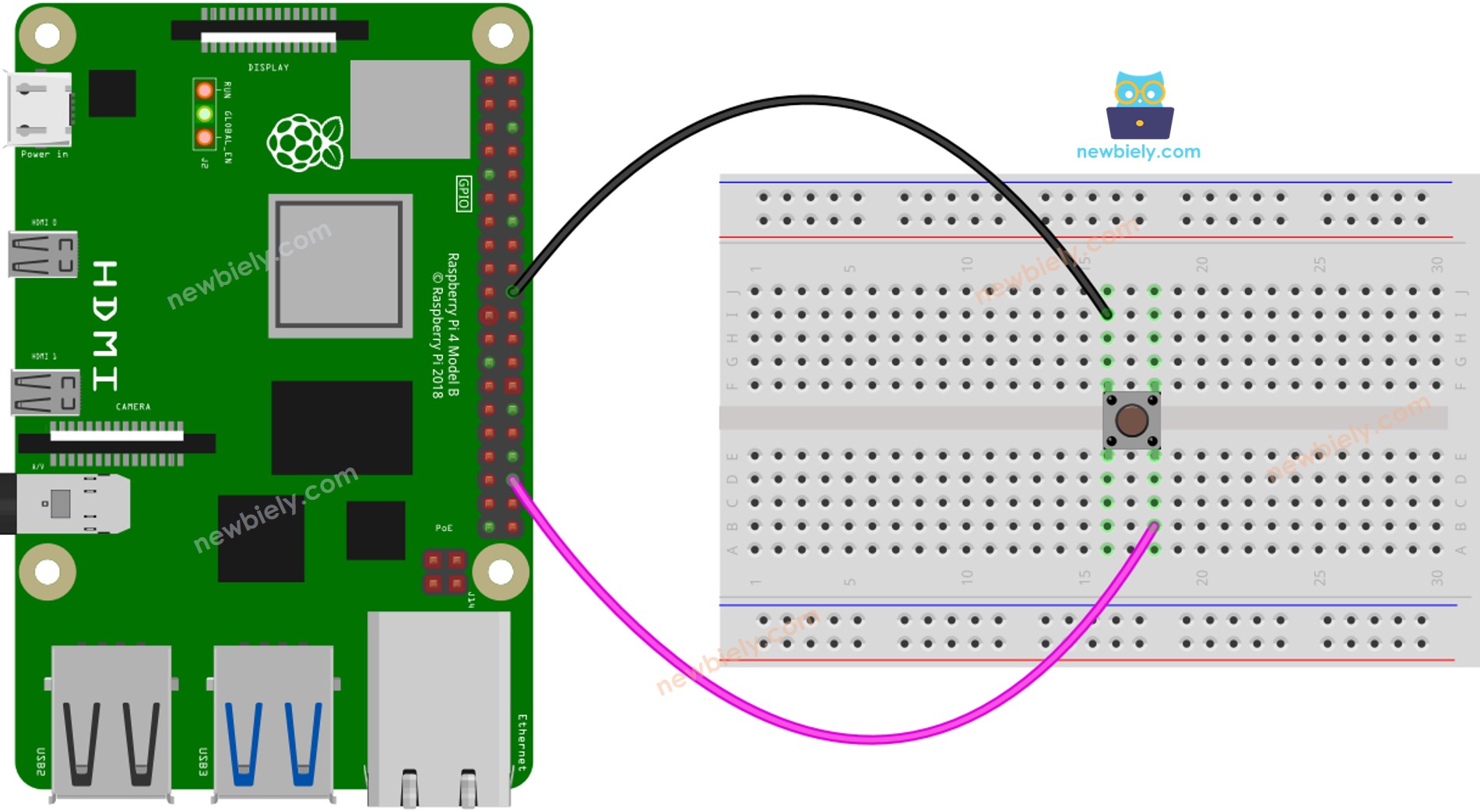
This image is created using Fritzing. Click to enlarge image
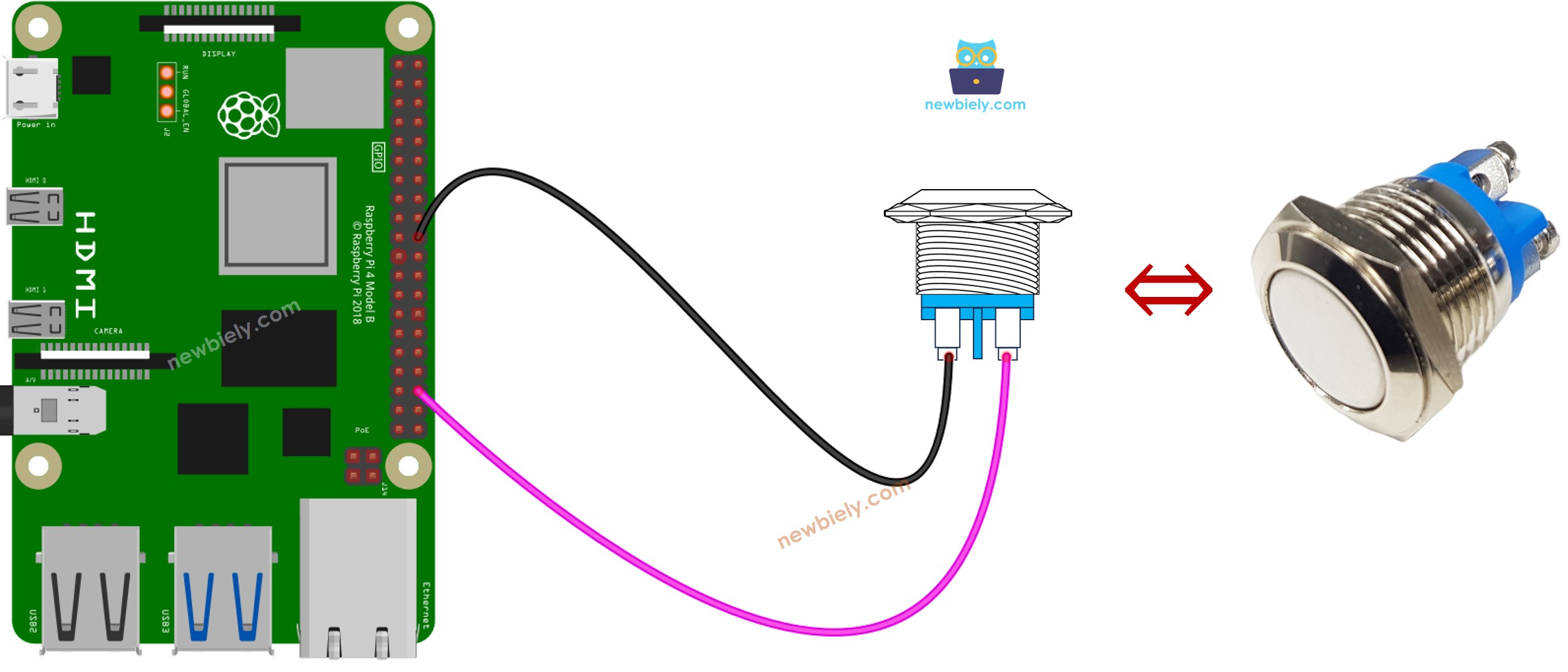
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
Utilize the
GPIO.setup() function to initialize the Raspberry Pi pin as an internal pull-up input. For instance, pin 16:
GPIO.setup(16, GPIO.IN, pull_up_down=GPIO.PUD_UP)
Utilizes the
GPIO.input() function to determine the state of the Raspberry Pi pin.
button_state = GPIO.input(16)
※ NOTE THAT:
Two common use cases are available:
The first: If the input state is HIGH, perform one action. If the input state is LOW, do the opposite.
The second: If the input state changes from LOW to HIGH (or HIGH to LOW), take some action.
We select one of these depending on the application. For example, when using a button to control an LED:
If we want the LED to be ON when the button is pressed and OFF when the button is NOT pressed, we SHOULD use the first use case.
If we want the LED to be toggled between ON and OFF each time we press the button, we SHOULD use the second use case.
Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
Make sure your Raspberry Pi is connected to the same local network as your PC.
Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
sudo apt-get update
sudo apt-get install python3-rpi.gpio
import RPi.GPIO as GPIO
import time
BUTTON_PIN = 16
GPIO.setmode(GPIO.BCM)
GPIO.setup(BUTTON_PIN, GPIO.IN, pull_up_down=GPIO.PUD_UP)
try:
while True:
button_state = GPIO.input(BUTTON_PIN)
print(button_state)
time.sleep(0.1)
except KeyboardInterrupt:
print("\nExiting...")
GPIO.cleanup()
1 is HIGH, 0 is LOW.
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
Check out the line-by-line explanation contained in the comments of the source code!
import RPi.GPIO as GPIO
import time
BUTTON_PIN = 16
GPIO.setmode(GPIO.BCM)
GPIO.setup(BUTTON_PIN, GPIO.IN, pull_up_down=GPIO.PUD_UP)
prev_button_state = GPIO.input(BUTTON_PIN)
try:
while True:
button_state = GPIO.input(BUTTON_PIN)
if button_state != prev_button_state:
if button_state == GPIO.LOW:
print("The button is pressed!")
else:
print("The button is released!")
prev_button_state = button_state
time.sleep(0.1)
except KeyboardInterrupt:
print("\nExiting...")
GPIO.cleanup()
python3 button_pressed.py
The button is pressed
The button is released
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
Check out the line-by-line explanation contained in the comments of the source code!
※ NOTE THAT:
Even if you press and release the button only once, the output in Terminal may display multiple pressed and released events. This is the expected behaviour of the button. This phenomenon is referred to as
“chattering”. To learn more about it, please refer to the
Raspberry Pi - Button Debounce tutorial.
If you are using the
button module, configure the pin to input mode using
GPIO.setup(BUTTON_PIN, GPIO.IN, pull_up_down=GPIO.PUD_OFF). With this setup, the module will output
LOW when the button is unpressed and
HIGH when the button is pressed.
When the button is pressed, the LED should be turned on.
When the button is not pressed, the LED should be turned off.
Each time the button is pressed, the LED should switch between ON and OFF.
What are the occasions when a pull-down/pull-up resistor should and should not be used for an input pin?
If the sensor has either closed or open states, a pull-up or pull-down resistor is required to make these states become LOW and HIGH. Examples of such sensors are push-button, switch, and magnetic contact switch (door sensor).
On the other hand, if the sensor has two defined voltage levels (LOW and HIGH), a pull-up or pull-down resistor is NOT necessary. Examples of such sensors are motion sensor and touch sensor.