Raspberry Pi - Temperature Humidity Sensor - OLED
This tutorial instructs you how to use Raspberry Pi to read the temperature and humidity from a DHT11/DHT22 sensor and display it on an OLED.
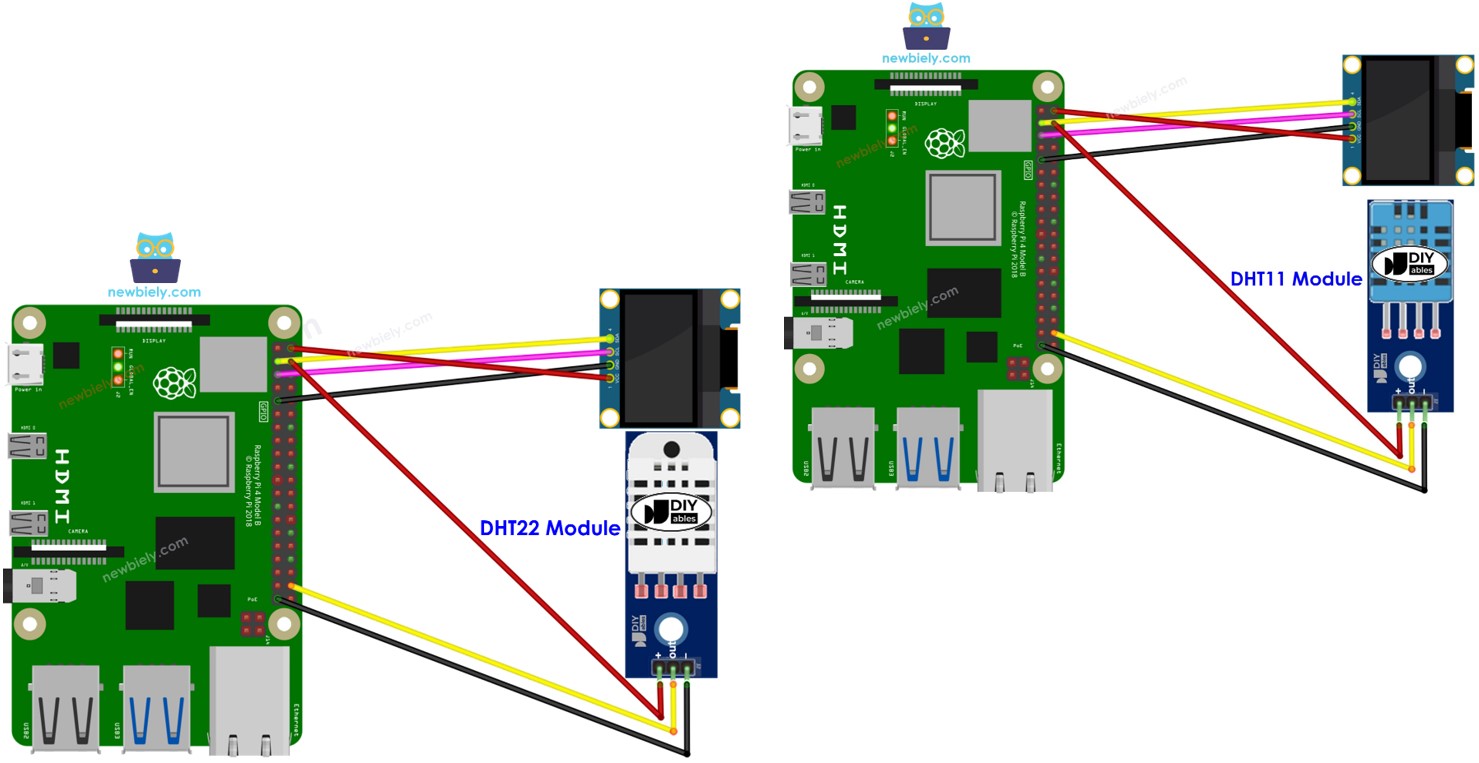
Hardware Preparation
1 | × | Raspberry Pi 5 | |
1 | × | SSD1306 I2C OLED Display 128x64 | |
1 | × | SSD1306 I2C OLED Display 128x32 | |
1 | × | DHT11 Temperature and Humidity Sensor | |
1 | × | Jumper Wires |
You can use DHT22 sensor instead of DHT11 sensor.
1 | × | Recommended: Screw Terminal Block Shield for Raspberry Pi | |
1 | × | Recommended: Raspberry Pi Prototyping Base Plate & Breadboard Kit | |
1 | × | Recommended: HDMI Touch Screen Monitor for Raspberry Pi |
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of OLED display, DHT11 and DHT22 Temperature Humidity Sensor
If you are unfamiliar with OLED display, DHT11 and DHT22 temperature humidity sensor (including pinout, functionality, programming, etc.), the following tutorials can help you out:
- Raspberry Pi - OLED tutorial
Wiring Diagram
Raspberry Pi - DHT11 Module LCD Wiring
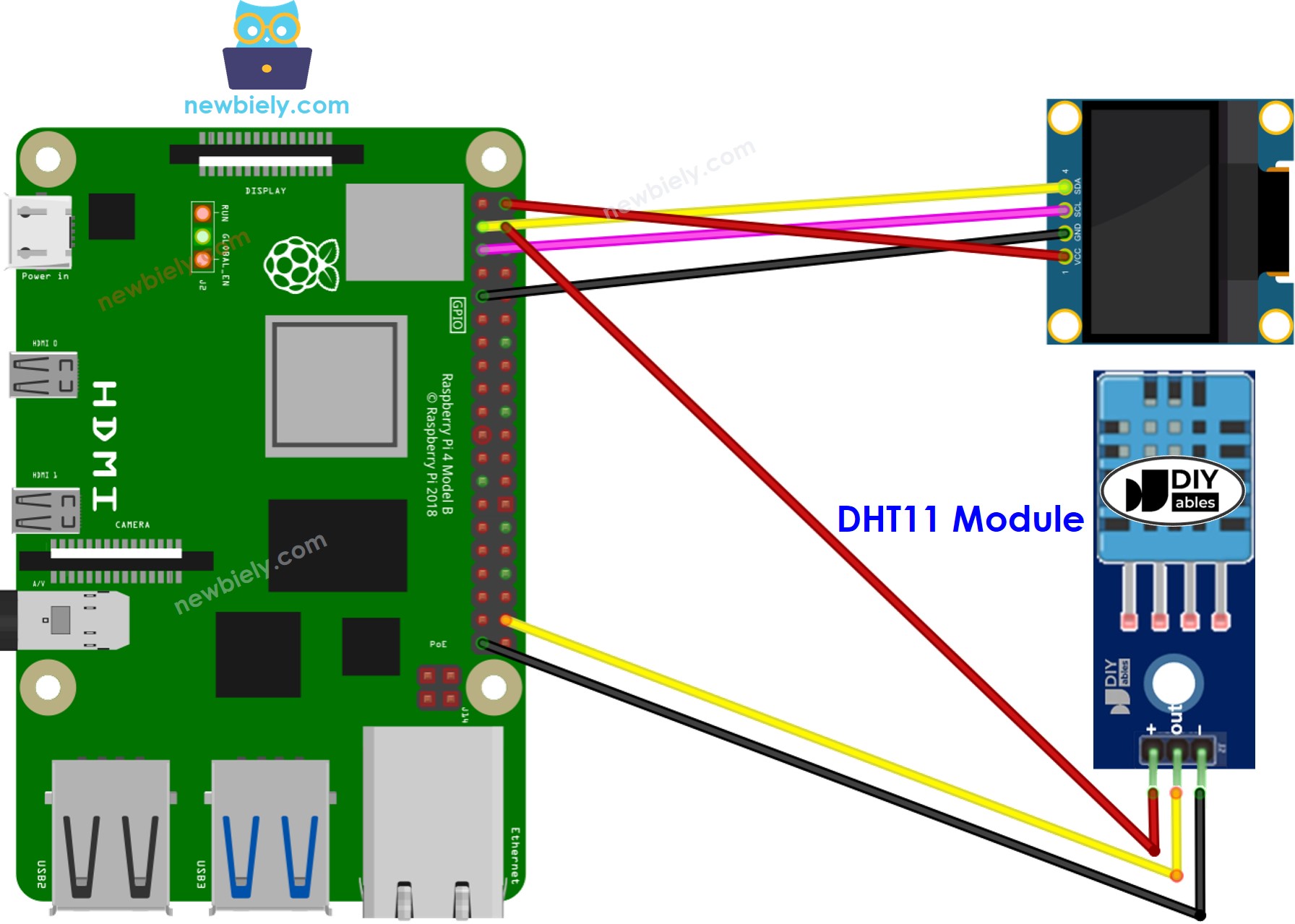
This image is created using Fritzing. Click to enlarge image
Raspberry Pi - DHT22 Module LCD Wiring
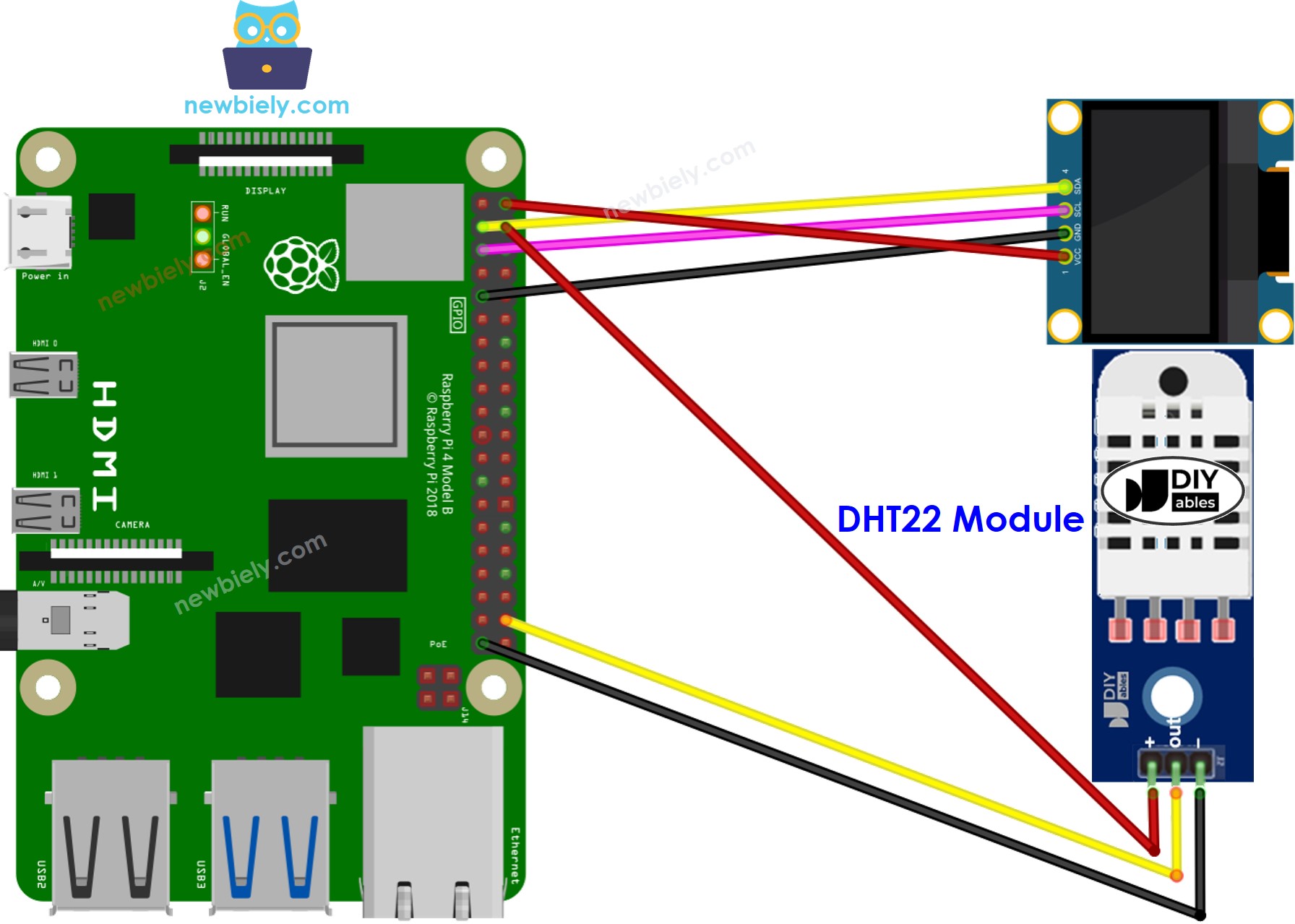
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
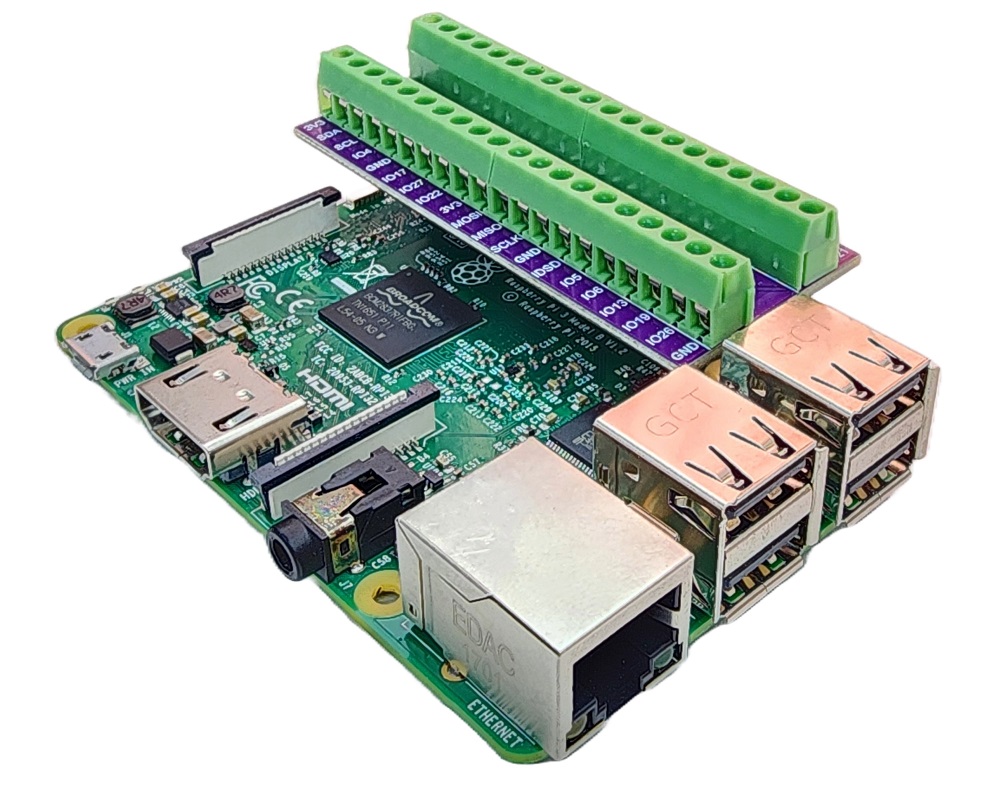
Raspberry Pi Code - DHT11 Sensor - OLED
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Prior to utilizing the OLED display with a Raspberry Pi, we need to enable I2C interface on Raspberry Pi. See How to enable I2C interface on Raspberry Pi
- Install the OLED library by running the following command:
- Install the library for DHT11 temperature and humidity sensor by running the following command:
- Create a Python script file dht11_oled.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
- Make the enviroment around the temperature sensor hot, or hold the sensor in your hand.
- Check out the results on the OLED display.