Raspberry Pi - Ultrasonic Sensor - Servo Motor
This tutorial instructs you how to use Raspberry Pi and ultrasonic sensor to control servo motor. In detail:
- If the object is near the ultrasonic sensor, Raspberry Pi turns the servo motor to 90 degrees.
- If the object is distant from the ultrasonic sensor, Raspberry Pi turns the servo motor back to 0 degree.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Servo Motor and Ultrasonic Sensor
If you are not familiar with servo motor and ultrasonic sensor (pinout, how it works, how to program ...), the following tutorials can help you learn:
Wiring Diagram
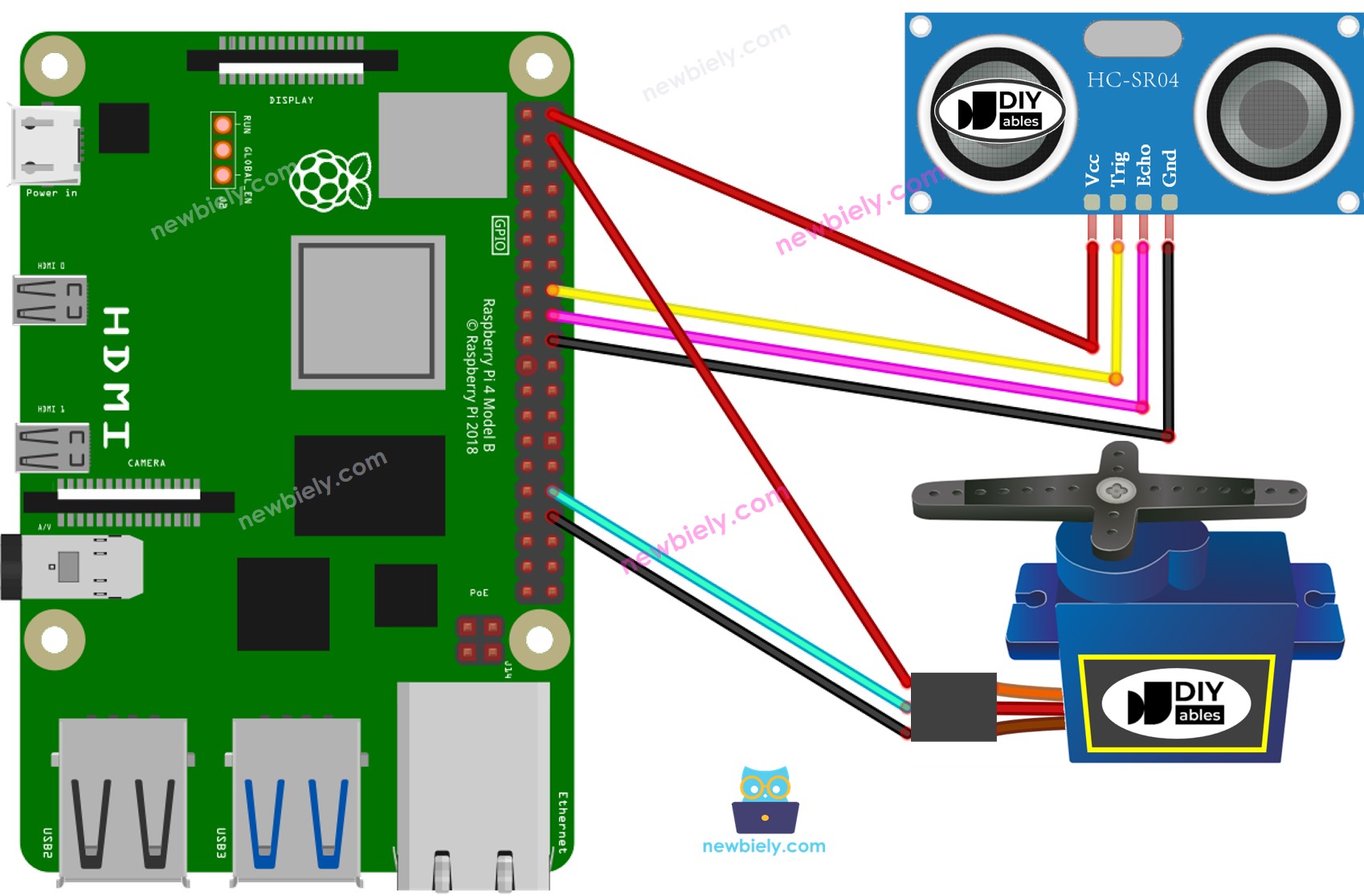
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
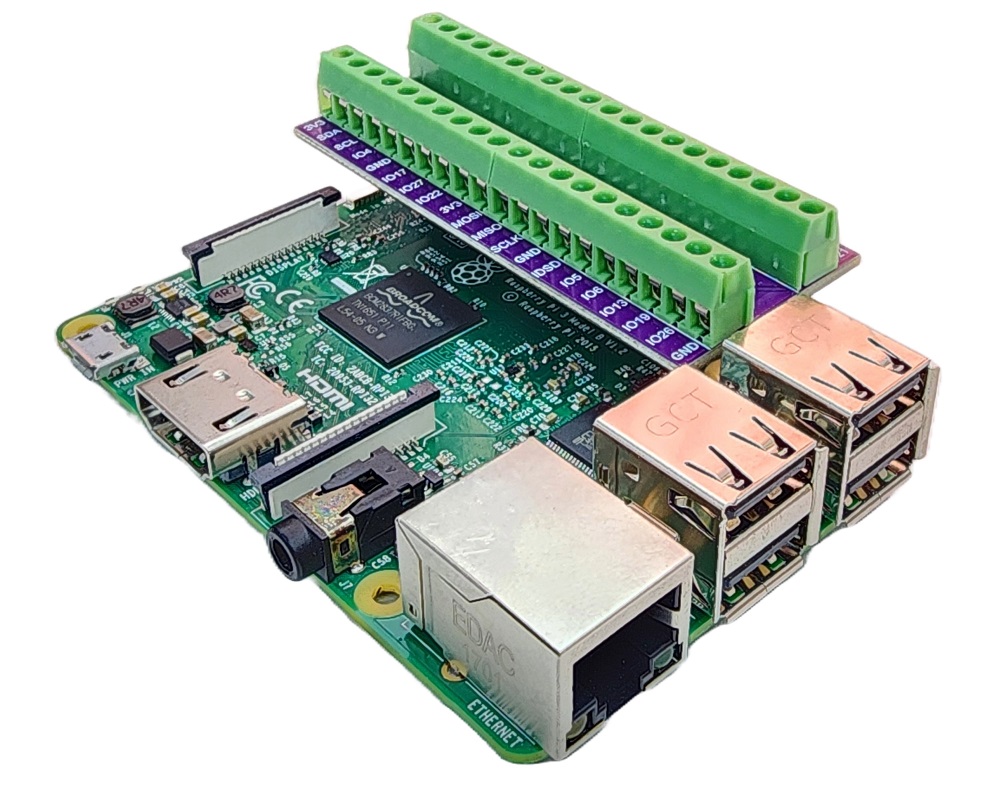
Please note that the wiring diagram shown above is only suitable for a servo motor with low torque. In case the motor vibrates instead of rotating, an external power source must be utilized to provide more power for the servo motor. The below demonstrates the wiring diagram with an external power source for servo motor.
TO BE ADD IMAGE
Please do not forget to connect GND of the external power to GND of Arduino Raspberry Pi.
Raspberry Pi Code - Ultrasonic Sensor Controls Servo Motor
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Create a Python script file ultrasonic_servo.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
- Wave your hand in front of the sensor.
- Check out the servo motor's movement.
※ NOTE THAT:
The code above is for educational purposes. The ultrasonic sensor is very susceptible to noise. If you plan to use the ultrasonic sensor in a practical setting, noise filtering should be applied. For more information on how to filter noise from distance measurements of an ultrasonic sensor, please refer to this tutorial.