Raspberry Pi - Relay
In a prior tutorial, we discovered how to switch an LED on and off by using Raspberry Pi. This tutorial instructs you how to activate and deactivate certain devices that use a high voltage power supply (e.g. a light bulb, fan, electromagnetic lock, linear actuator, etc.) by using Raspberry Pi.
? What are the commons and differences between controlling an LED and controlling a light bulb by using Raspberry Pi?
The common: Similar to controlling an LED, we use the Raspberry Pi's output pin to switch them on and off.
The difference:
- LED can be connected directly to Raspberry Pi's pin since it requires low power.
- For the light bulb, we cannot connect it directly to Raspberry Pi's pin as it requires high voltage and/or high current which can damage Raspberry Pi. Thus, a relay is necessary to protect Raspberry Pi from the high voltage/current.
Hardware Preparation
1 | × | Raspberry Pi 4 Model B | |
1 | × | Relay | |
1 | × | LED Strip | |
1 | × | 12V Power Adapter | |
1 | × | DC Power Jack | |
1 | × | Breadboard | |
1 | × | Jumper Wires | |
1 | × | (Optional) Screw Terminal Adapter for Raspberry Pi |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Overview of Relay
A relay is an electrical switch that can be programmed using Raspberry Pi or any micro-controller. It is used to programmatically turn devices on and off that use high voltage and/or high current.
It acts as a bridge between Raspberry Pi and high voltage components.
WARNING
When you are creating projects that involve mains voltage, it is essential to be aware of the risks. This is a serious matter and we want you to be safe. If you are not completely sure what you are doing, do not attempt it. Ask someone who knows for help.
Although some relays can be used for both DC and AC devices, we strongly suggest using a DC device (up to 24V) for testing.
Relay Pinout
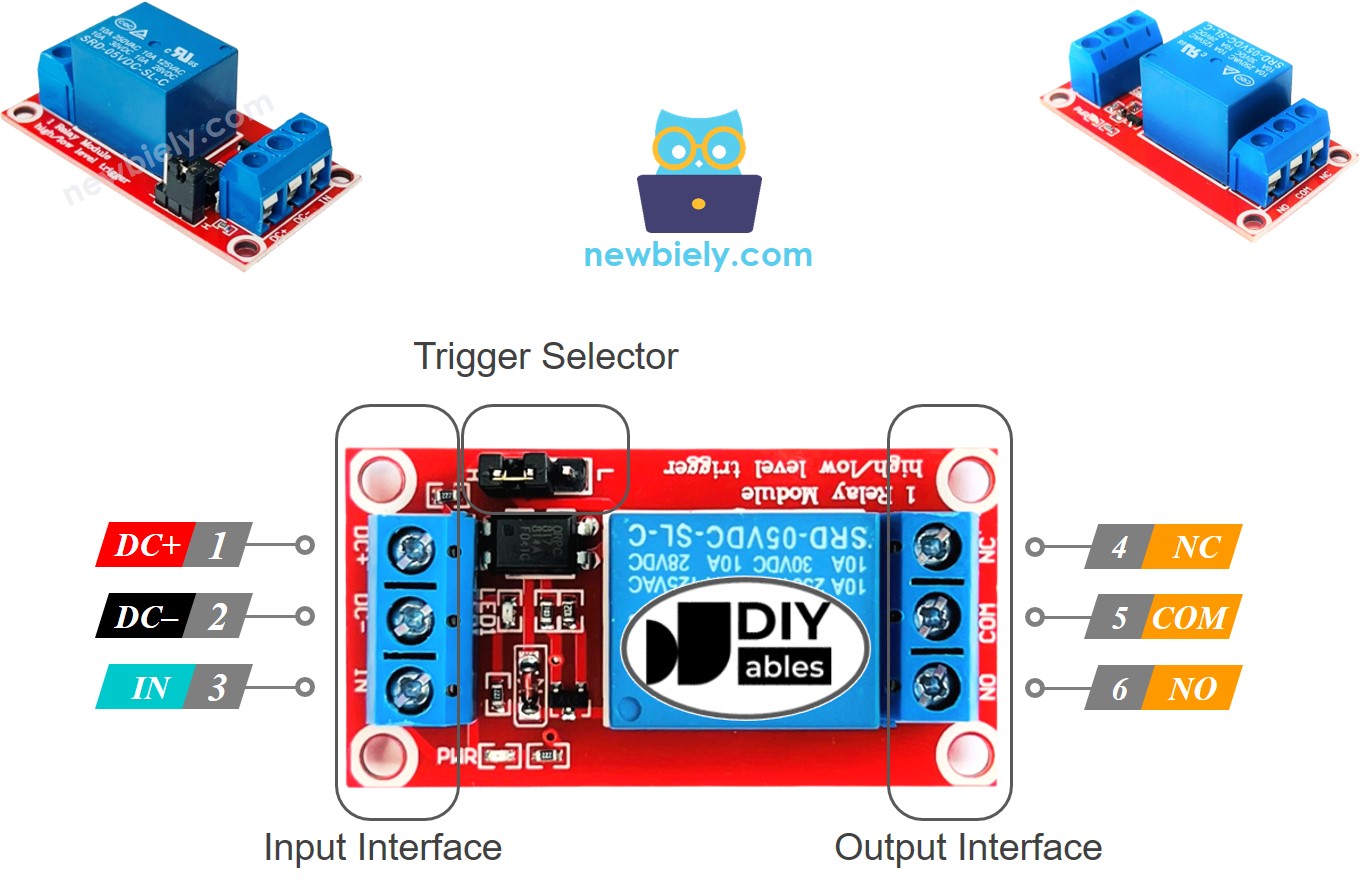
Relay has two sets of pins: an input (low voltage) group and an output (high voltage) group:
- The input group is connected to Raspberry Pi, and consists of three pins:
- DC- pin: must be linked to GND (0V)
- DC+ pin: must be linked to VCC (5V)
- IN pin: receives the control signal from Raspberry Pi
- The output group is connected to the high voltage device, and consists of three pins (usually in screw terminal):
- NO pin: is the normally open pin. It is used in the normally open mode
- NC pin: is the normally closed pin. It is used in the normally closed mode
- COM pin: is the common pin. It is used in both normally open and normally closed modes
- If we use the normally open mode, then COM pin and NO pin are used.
- If we use the normally closed mode, then COM pin and NC pin are used.
- The LOW level trigger mode
- The HIGH level trigger mode
- Normally Open
- Normally Closed. These are opposites.
- The normally open and normally closed modes work in opposite ways
- Most relay modules support both normally open and normally closed modes
- The LOW level trigger and HIGH level trigger modes work in opposite ways
- Not all relay modules support both LOW level trigger and HIGH level trigger modes
- At any given time, the relay module can only work in one of the two LOW level trigger and HIGH level trigger modes
- Linking a Raspberry Pi's pin to the IN pin of the relay
- Programming the pin to LOW or HIGH to control the relay
Generally, we do not employ all of the pins in the high voltage group. We only use two of them:
Furthermore, if the relay has both LOW and HIGH level triggers, typically there is a jumper to choose either LOW level trigger or HIGH level trigger.
※ NOTE THAT:
The arrangement of the pins on a relay module can differ among manufacturers. It is crucial to always refer to the labels printed on the relay module when working with it. Pay close attention!
How to Connect the High Voltage Device to Relay
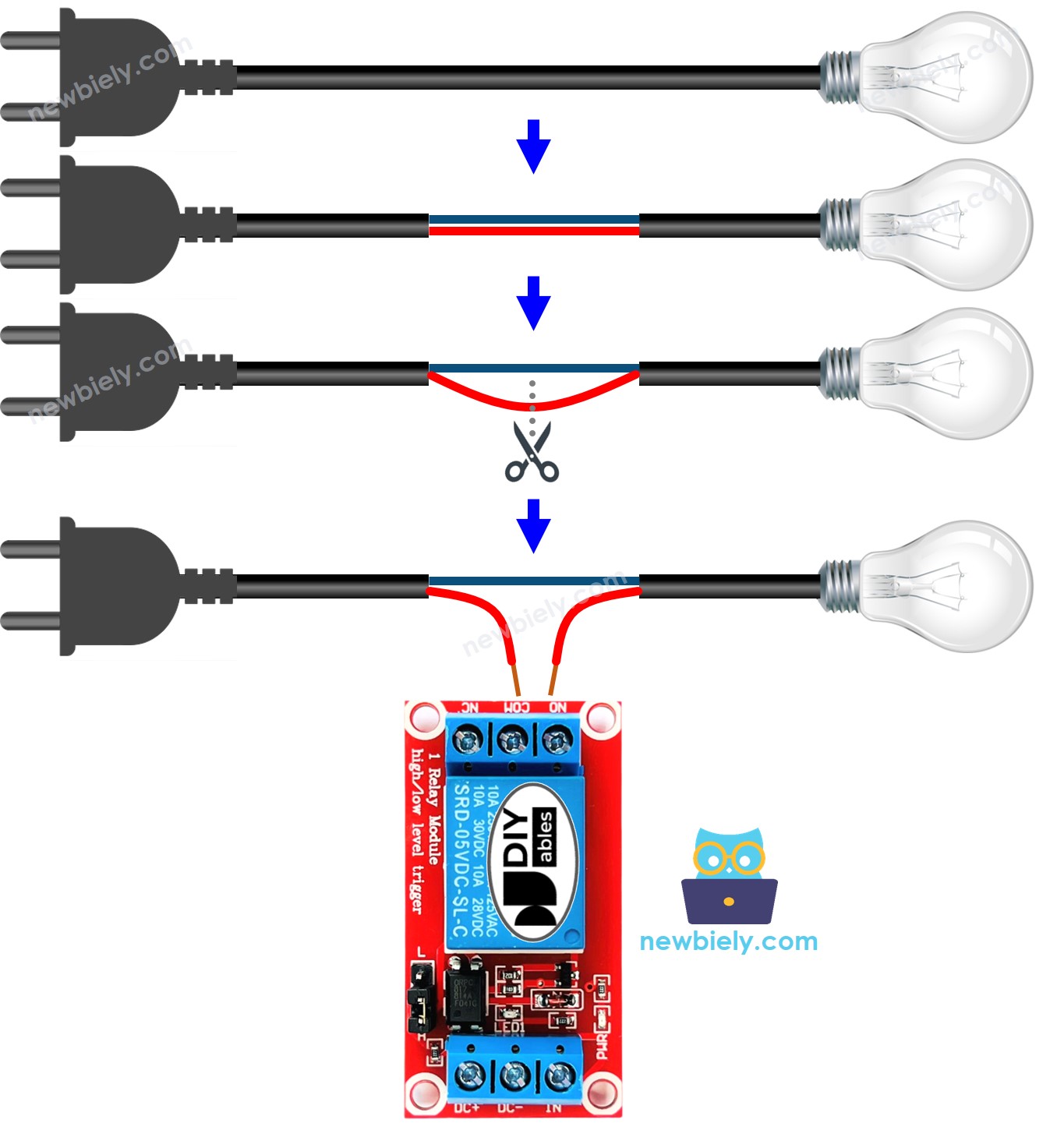
How It Works
Depending on the manufacturer and how it is installed, a relay may operate differently.
For the IN pin, there are two input modes that cause the relay to operate in opposite ways:
For the output pins, there are two modes of operation:
The term "normally" implies "if the IN pin is linked to LOW (0V)".
Before we go into detail, here is some quick information:
The combination of input modes and output modes generates multiple use cases. If you are a novice, we suggest employing HIGH level trigger mode and normally open mode.
The HIGH level trigger mode will be explained in detail as it works in the opposite way to the LOW level trigger. The LOW level trigger operates in the opposite manner.
HIGH Level Trigger - Normally Open Mode
In order to utilize this mode, we must attach the high voltage device to the COM pin and NO pin.
When the IN pin is connected to LOW (0V), the switch is open, resulting in the device being OFF (or inactive).
Conversely, when the IN pin is connected to HIGH (5V), the switch is closed, causing the device to be ON (or active).
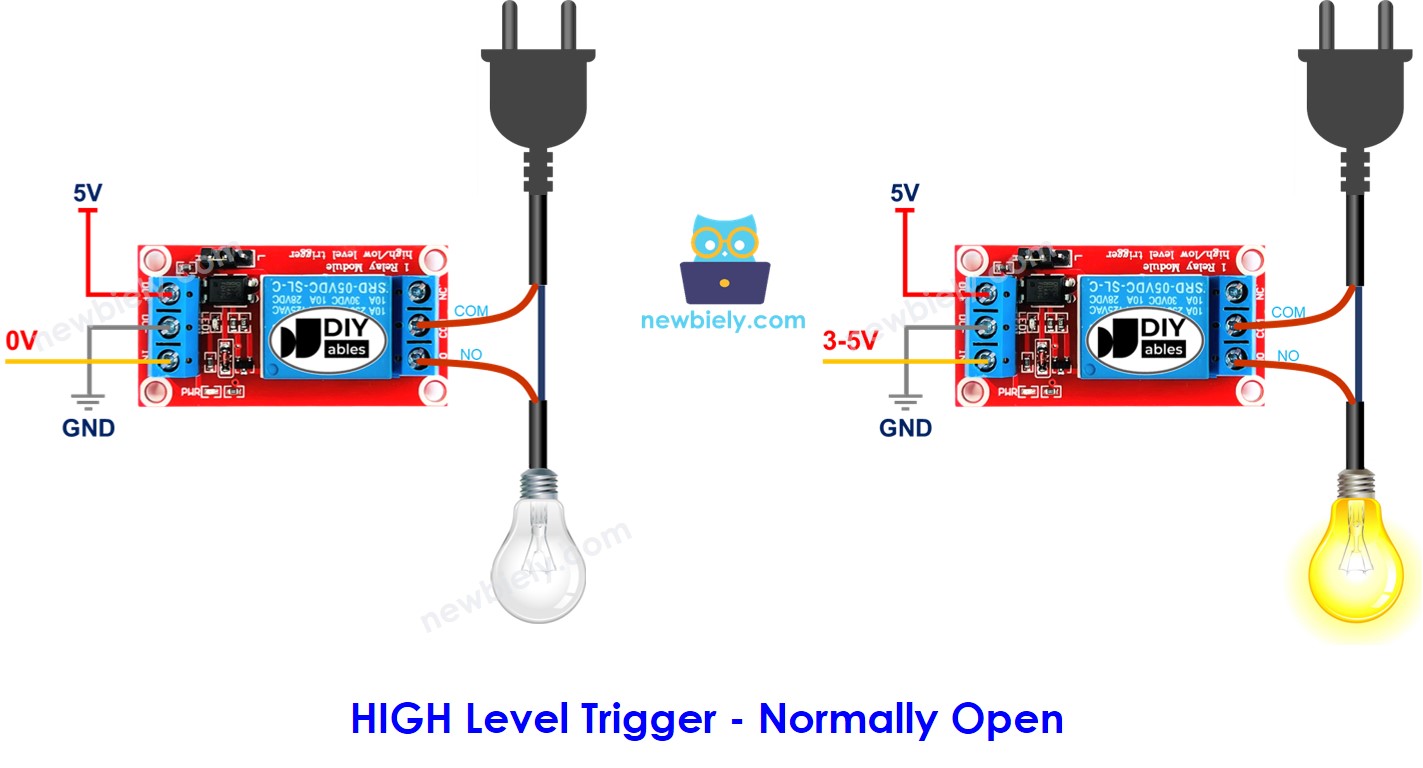
HIGH Level Trigger - Normally Closed Mode
To utilize this mode, we must attach the high voltage device to the COM pin and NC pin.
If the IN pin is linked to LOW (0V), the switch will be shut. The device will be ON (or active).
When the IN pin is connected to HIGH (5V), the switch will be open. The device will be OFF (or inactive).
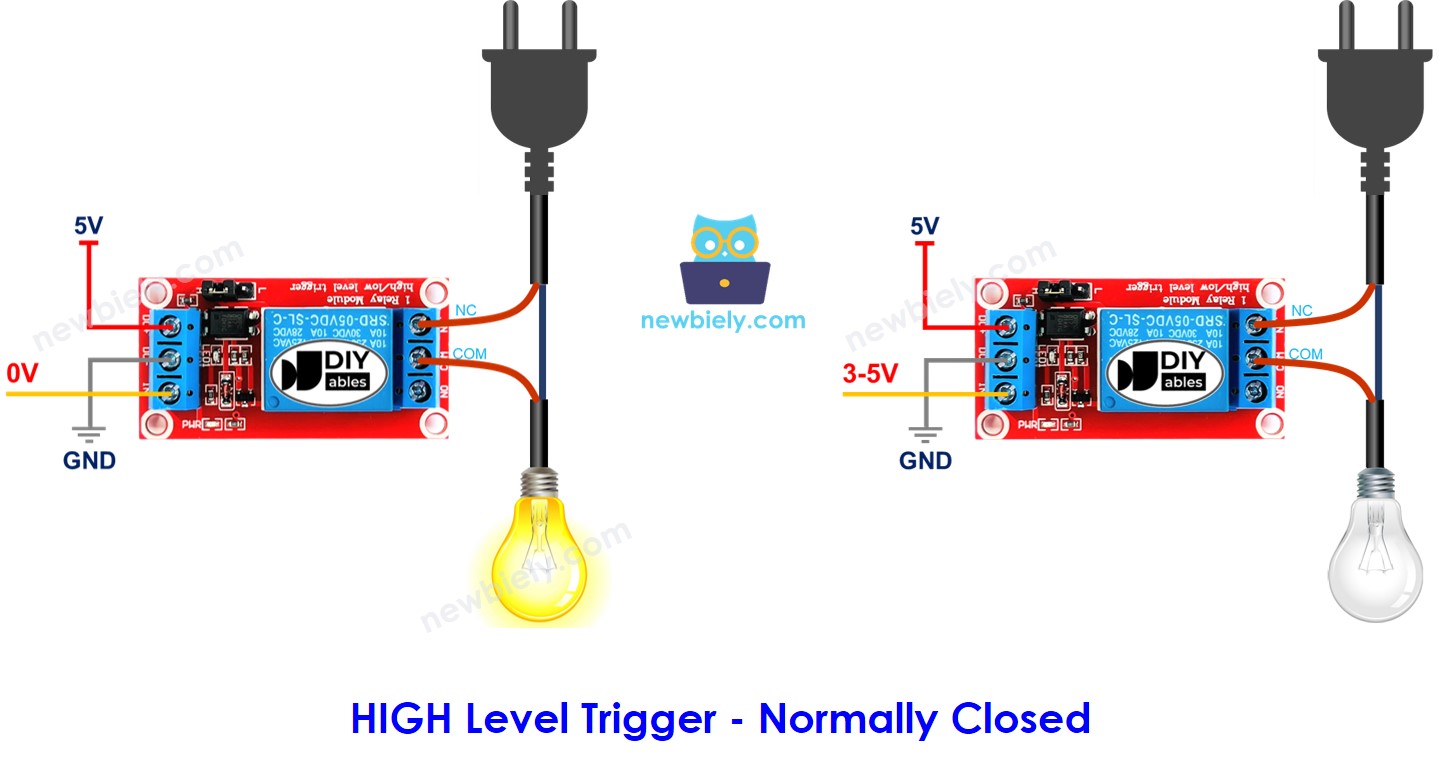
Summary
Input modes | Output Modes | IN pin (programmable) | Output pins | Relay state | Device state |
---|---|---|---|---|---|
HIGH Trigger | Normally Open | LOW | COM and NO pin | ⇒ open | ⇒ OFF |
HIGH Trigger | Normally Open | HIGH | COM and NO pin | ⇒ closed | ⇒ ON |
HIGH Trigger | Normally Closed | LOW | COM and NC pin | ⇒ closed | ⇒ ON |
HIGH Trigger | Normally Closed | HIGH | COM and NC pin | ⇒ open | ⇒ OFF |
LOW Trigger | Normally Open | LOW | COM and NO pin | ⇒ closed | ⇒ ON |
LOW Trigger | Normally Open | HIGH | COM and NO pin | ⇒ open | ⇒ OFF |
LOW Trigger | Normally Closed | LOW | COM and NC pin | ⇒ open | ⇒ OFF |
LOW Trigger | Normally Closed | HIGH | COM and NC pin | ⇒ closed | ⇒ ON |
There are a maximum of 8 use cases. It could be overwhelming. However, if you are a beginner, you only need to care about the first two scenarios, which involve HIGH level trigger and normally open. The rest of this tutorial will focus on these two use cases.
Raspberry Pi - Relay
Raspberry Pi controls a relay, which in turn controls a high voltage device.
Managing a relay is straightforward. All that is required is:
Wiring Diagram
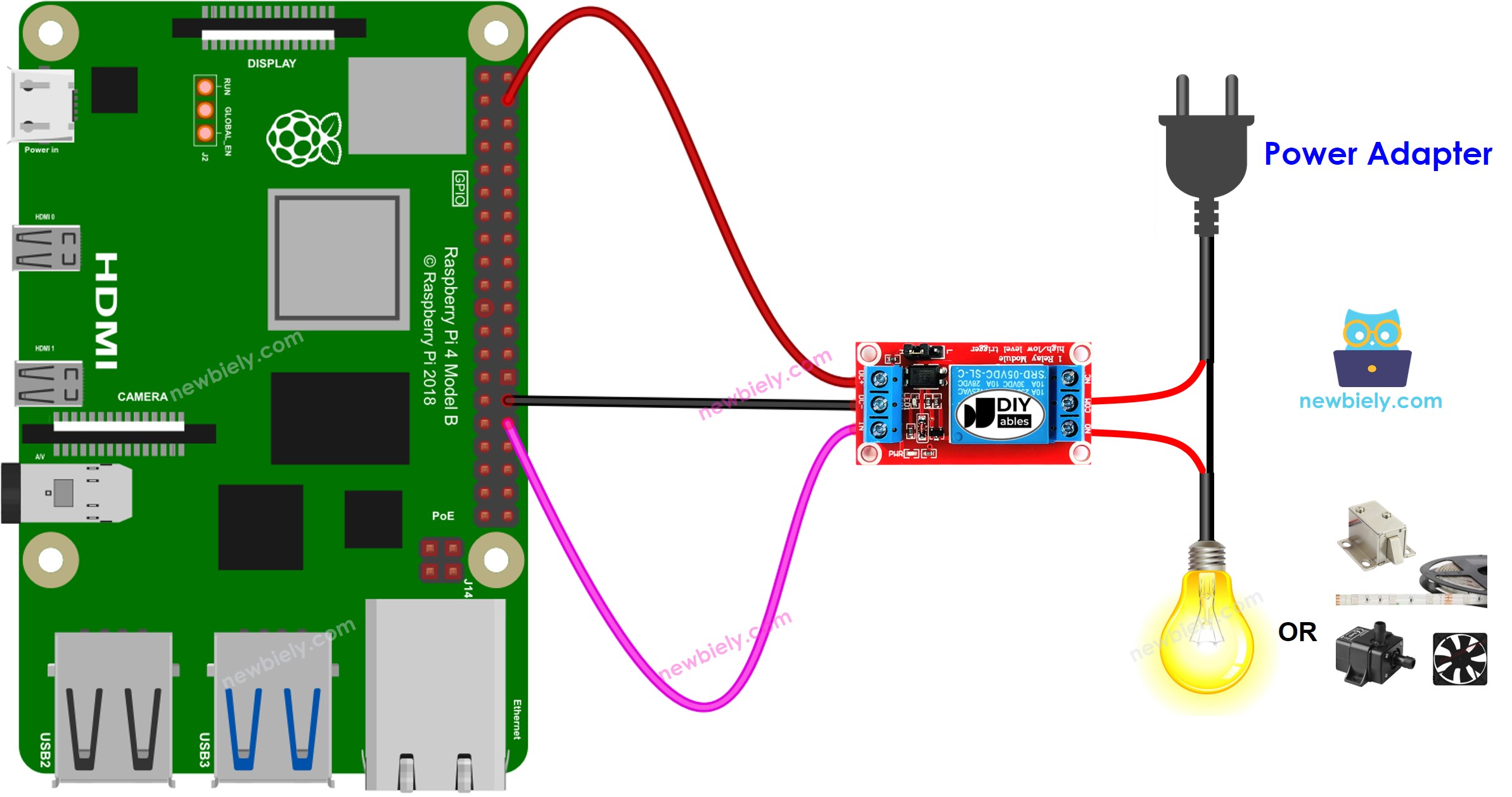
This image is created using Fritzing. Click to enlarge image
How To Program For Relay
- Set the pin 3 of a Raspberry Pi to digital output mode by utilizing the RPi.GPIO. For example:
- Set the pin to 0V by utilizing the GPIO.output() function:
- Set the pin to 5V by using the GPIO.output() function:
Raspberry Pi Code
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Create a Python script file relay.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Check out the LED strip, which should be blinking.
The script runs in an infinite loop continuously until you press Ctrl + C in the Terminal.
Video Tutorial
Challenge Yourself
- When you enter your room, the light will be automatically switched on. After you leave, it will turn off after 30 seconds. For more information, please refer to Raspberry Pi - Motion Sensor.