Raspberry Pi - Potentiometer Piezo Buzzer
This tutorial instructs you how to use Raspberry Pi and potentiometer to control piezo buzzer. In detail:
- Raspberry Pi determines if the potentiometer's analog value is above or below a threshold, and make sound accordingly
- Raspberry Pi determines if the potentiometer's output voltage is above or below a threshold, and make sound accordingly
- If the potentiometer's output voltage is greater than a threshold, Raspberry Pi can also play a melody of a song
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Piezo Buzzer and Potentiometer
If you are unfamiliar with piezo buzzer and potentiometer (including pinout, functioning, and programming), the following tutorials can help:
Wiring Diagram
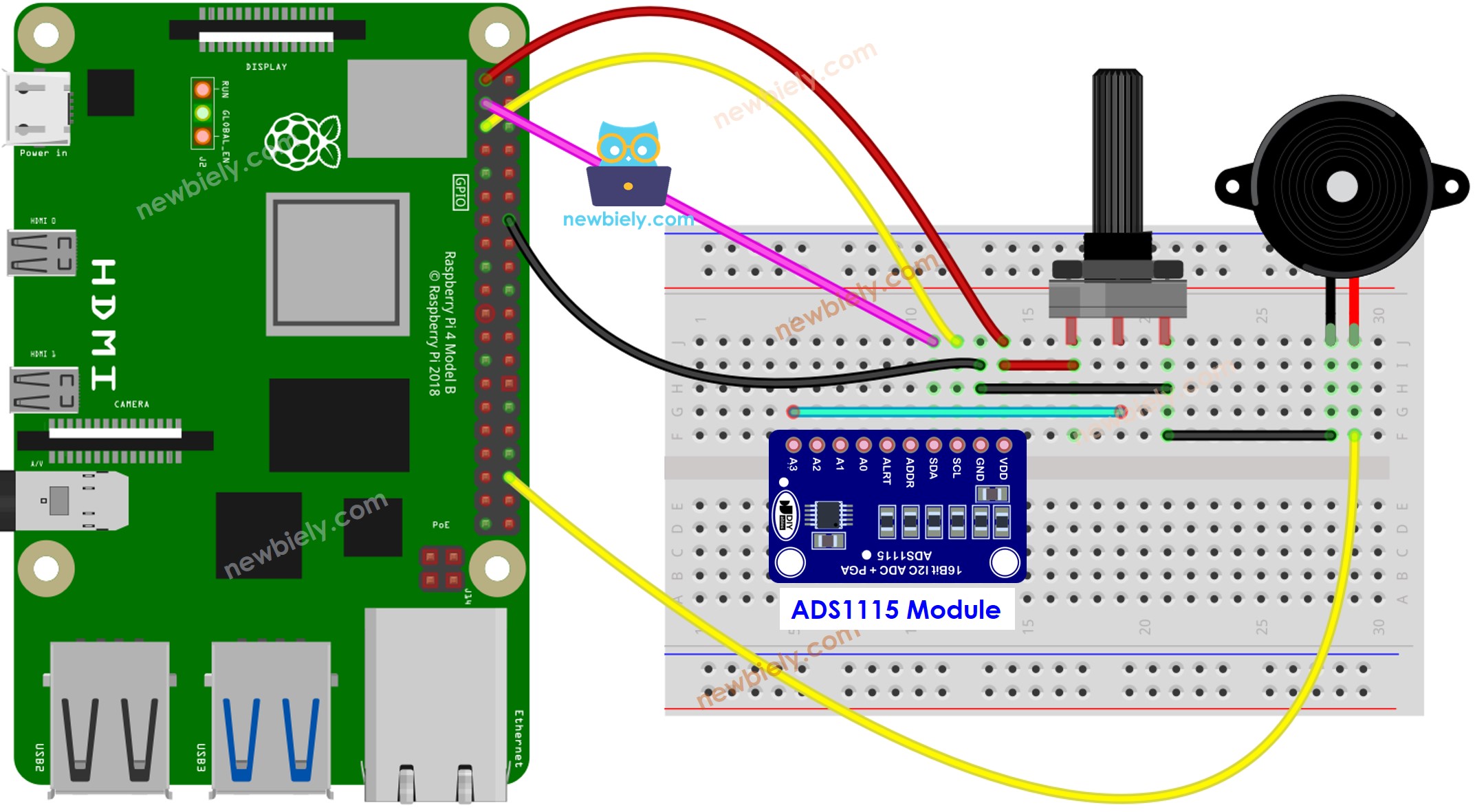
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
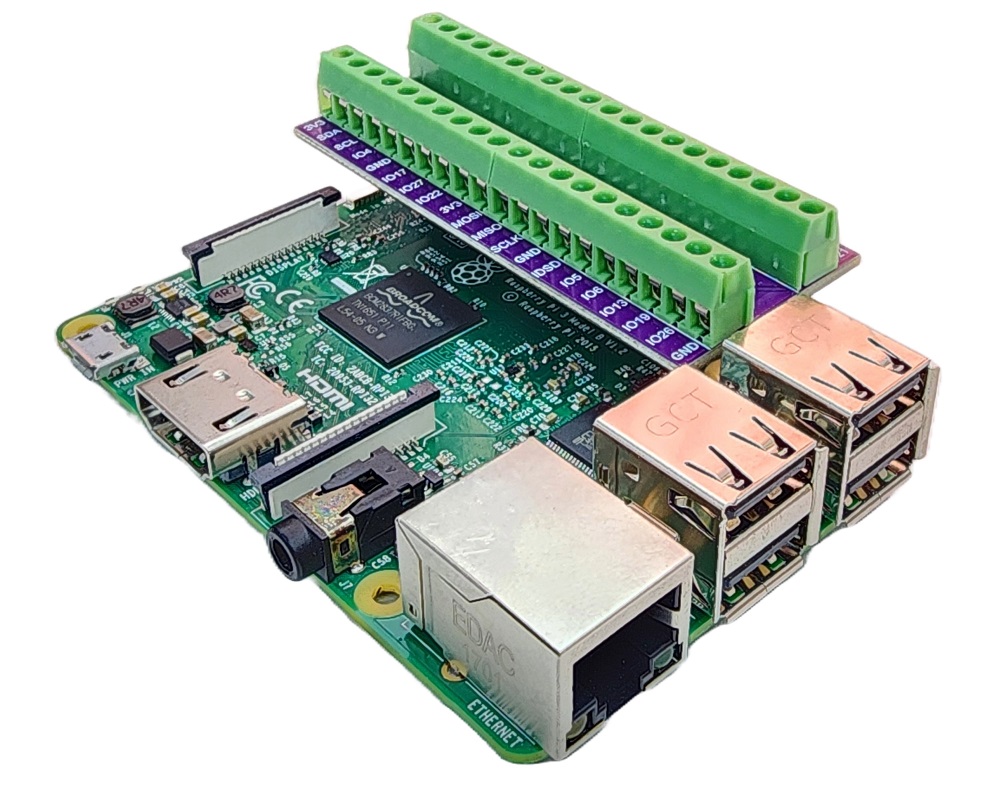
Raspberry Pi Code - Simple Sound
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Install the Adafruit_ADS1x15 library by running the following commands on your Raspberry Pi terminal:
- Create a Python script file potentiometer_buzzer.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Turn the potentiometer knob.
- Listen to the sound coming from the piezo buzzer.
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!
Raspberry Pi plays the melody of the song
Let play the "Jingle Bells" melody when the potentiometer is turned to a threshold value.
Detailed Instructions
- Create a Python script file potentiometer_buzzer_song.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Turn the potentiometer.
- Hear the song from the piezo buzzer.
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!