Raspberry Pi - Temperature Sensor
This tutorial instructs you how to use Raspberry Pi to read temperature from the waterproof 1-wire DS18B20 temperature sensor. This sensor is cost-effective, simple to use and has an attractive appearance.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Buy Note: Many DS18B20 sensors available in the market are unreliable. We strongly recommend buying the sensor from the DIYables brand using the link provided above. We tested it, and it worked reliably.
Overview of One Wire Temperature Sensor - DS18B20
The Temperature Sensor Pinout
The DS18B20 temperature sensor has three pins:
- GND pin: which needs to be connected to GND (0V)
- VCC pin: which needs to be connected to VCC (5V or 3.3V)
- DATA pin: which is the 1-wire data bus and should be connected to a digital pin on Raspberry Pi.
The sensor is typically available in two forms: TO-92 package (which resembles a transistor) and a waterproof probe. For this tutorial, we will be using the waterproof probe form.
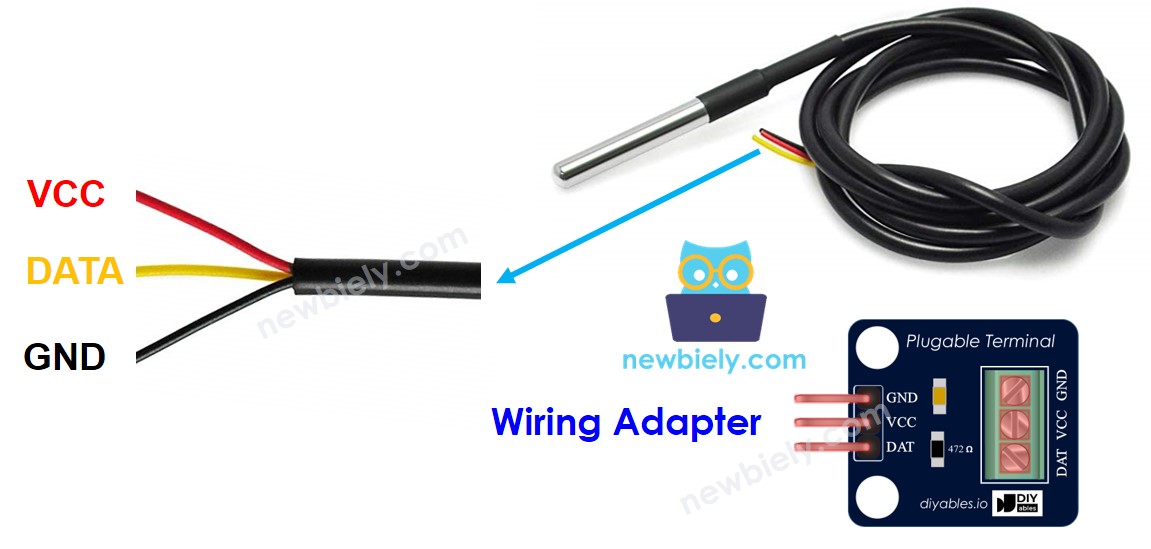
Connecting a DS18B20 temperature sensor to a Raspberry Pi requires a pull-up resistor, which can be a bit of a hassle. Fortunately, some manufacturers simplify the process by offering a wiring adapter that includes a built-in pull-up resistor and a screw terminal block.
Wiring Diagram
- Wiring diagram using a breadboard.
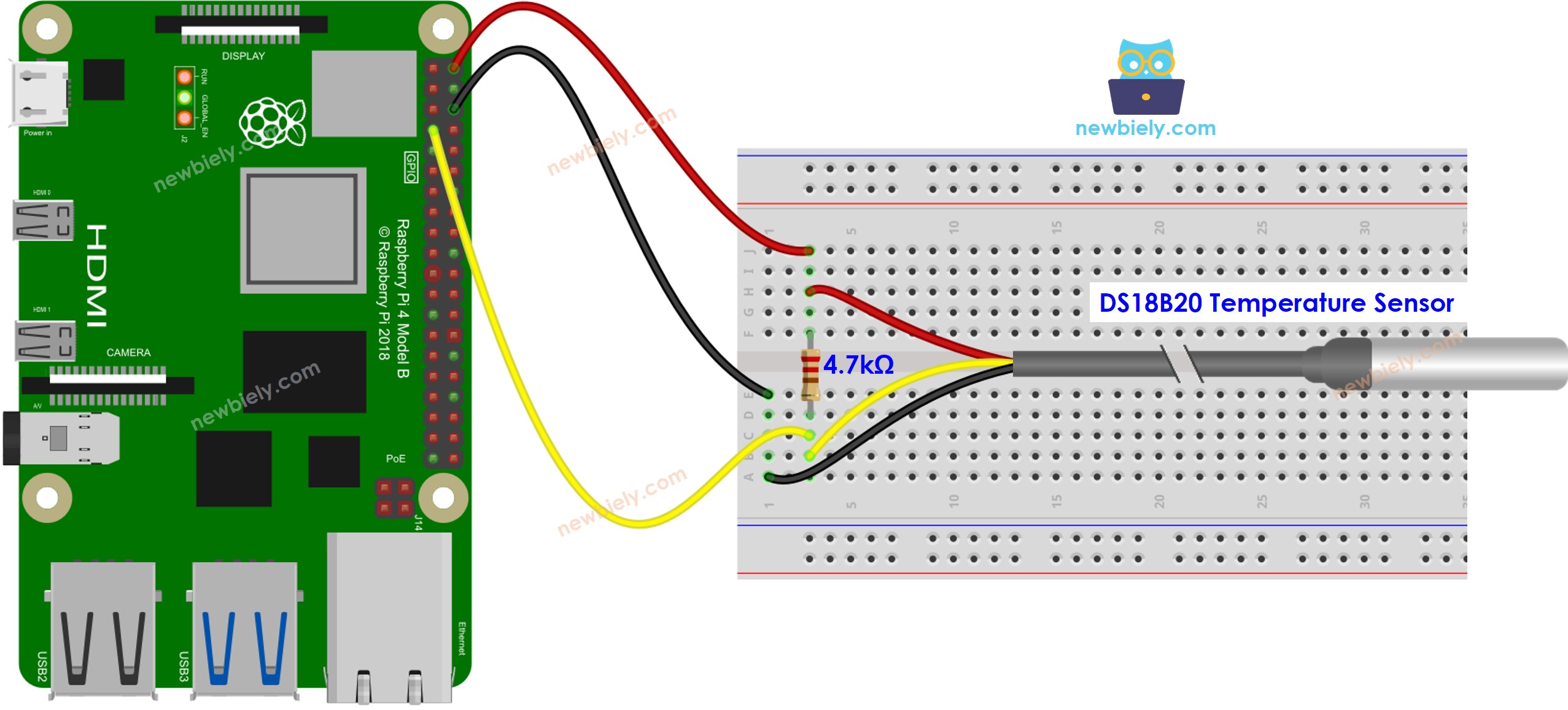
This image is created using Fritzing. Click to enlarge image
- Wiring diagram using a the wiring adapter (recommended).
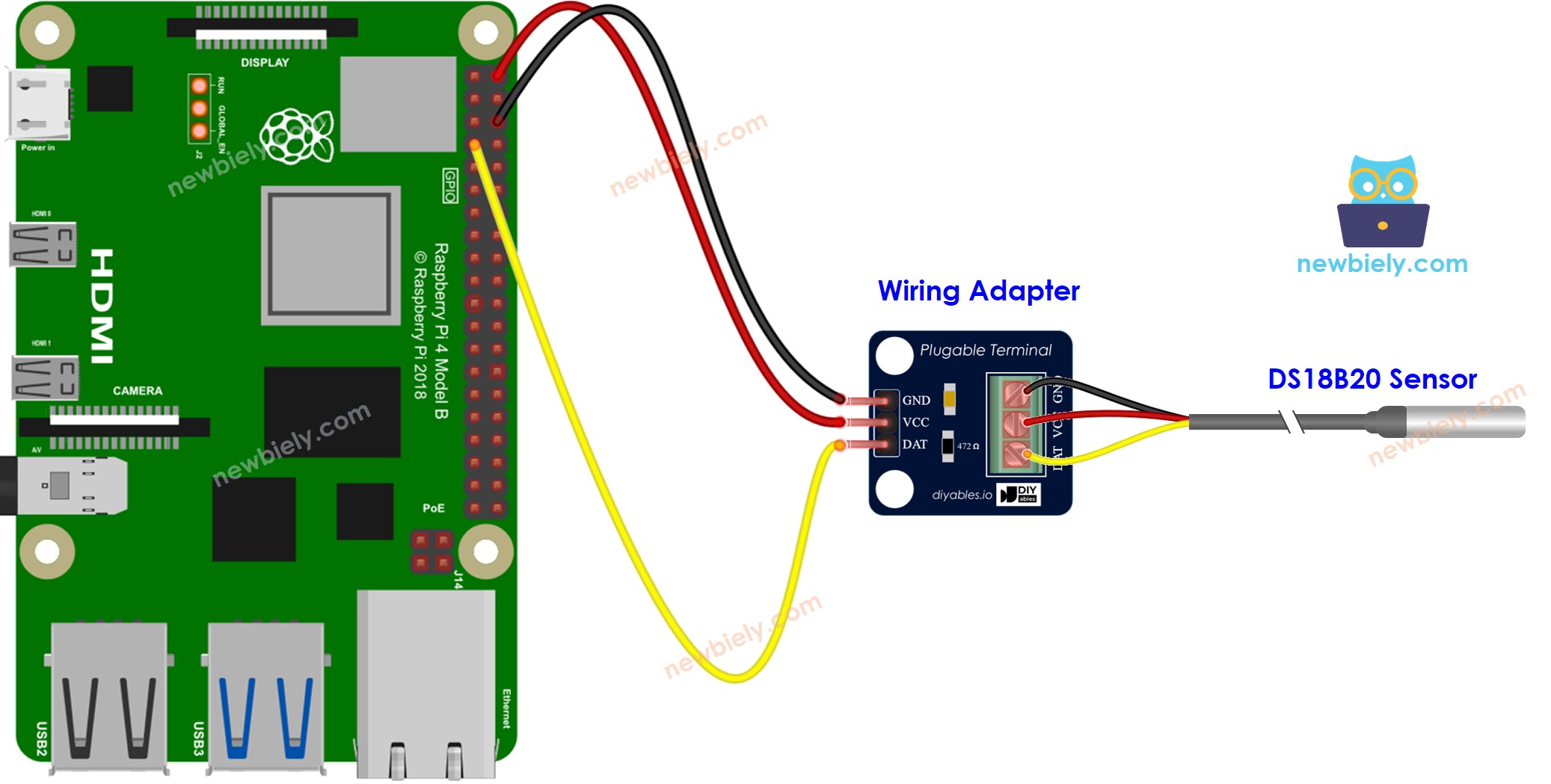
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
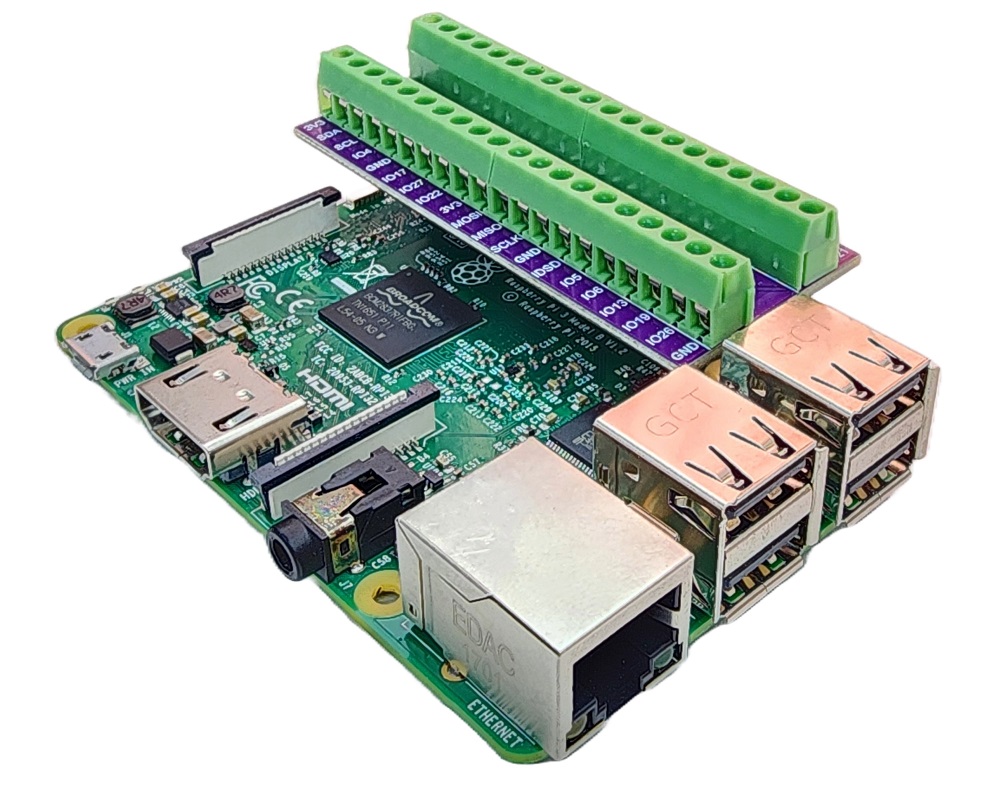
We recommend buying a DS18B20 sensor along with its accompanying wiring adapter for a seamless setup. This adapter includes an integrated resistor, removing the need for an additional resistor in the wiring. We also tested it and worked well.
Raspberry Pi Code
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Prior to utilizing the DS18B20 temperature sensor with a Raspberry Pi, we need to enable 1-Wire interface on Raspberry Pi. See How to enable 1-Wire interface on Raspberry Pi
- Install the library for DS18B20 temperature sensor by running the following command:
- Create a Python script file DS18B20.py and add the following code:
- Save the file and run the Python script by executing the following command in the Terminal:
- Place the sensor in hot and cold water, or hold it in your hand.
- Check the output on the Terminal.
The script runs in an infinite loop continuously until you press Ctrl + C in the Terminal.