Raspberry Pi - Actuator
This tutorial instructs you how to use Raspberry Pi to control Linear Actuator. In detail, we will learn:
- How a linear actuator works
- How to program Raspberry Pi to make a linear actuator extend or retract
- How to program Raspberry Pi to control the speed of a linear actuator
There are two types of linear actuators:
- Linear actuator without feedback: This one usually extends or retracts all the way and cannot be precisely controlled to stop at a specific position.
- Linear actuator with feedback: This one has a feedback signal that allows it to be precisely controlled to extend or retract to a specific position.
This tutorial is for linear actuator without feedback. If you are looking to learn about linear actuator with feedback, please refer to this Raspberry Pi - Actuator with Feedback tutorial.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Overview of Linear Actuator
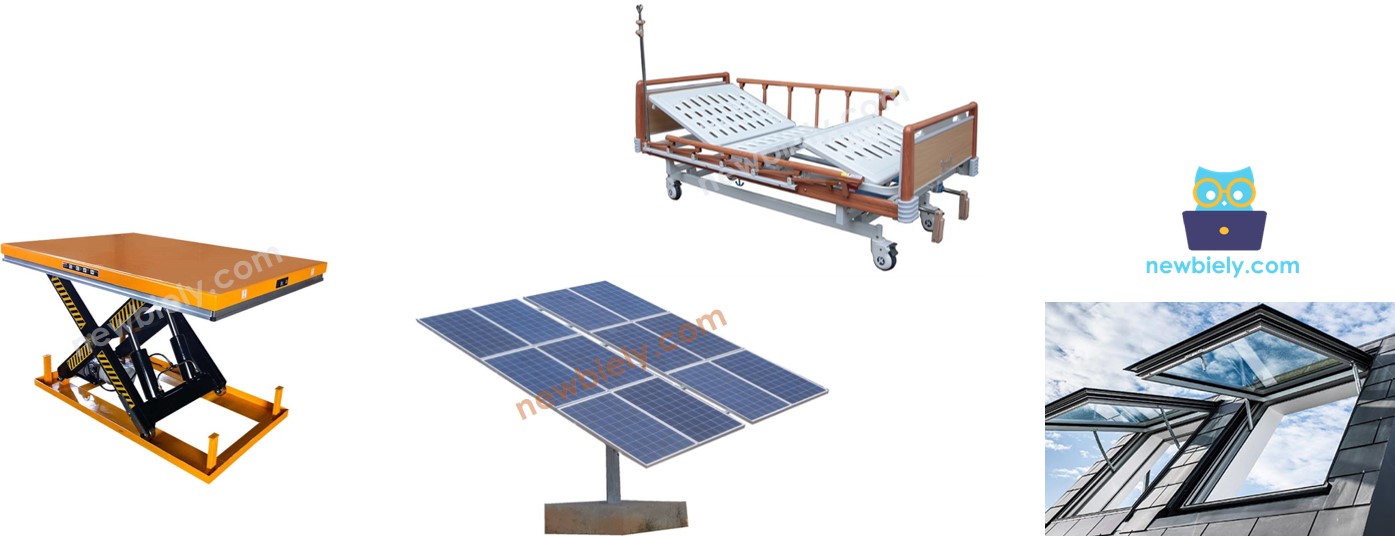
Linear Actuator Pinout
A Linear Actuator has two wires, with the positive wire typically being red and the negative wire usually black.
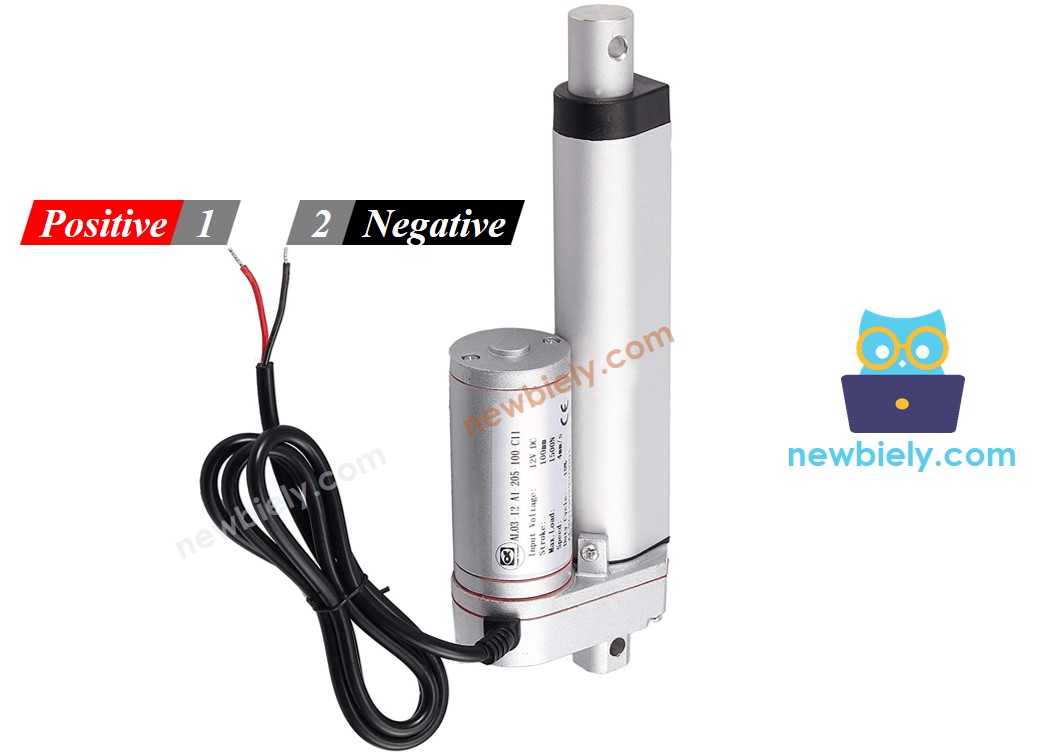
How It Works
When purchasing a linear actuator, it is important to understand what voltage it requires to operate. As an example, let's consider a 12V linear actuator.
When you power the 12V linear actuator by a 12V power source:
- Connect 12V to the positive wire and GND to the negative wire: the linear actuator full-speed extends until it reaches the limit.
- Connect 12V to the negative wire and GND to the positive wire: the linear actuator full-speed retracts until it reaches the limit.
When power is cut off to the actuator by connecting the ground wire to both the positive and negative wires, the process of extending or retracting will cease.
※ NOTE THAT:
For DC motor, servo motor, and stepper motor without gearing, when a load is present, if the power is cut off, they are unable to maintain their position. In contrast, an actuator can remain in its position even when the power is no longer supplied while it is carrying a load.
If the voltage of the power supply for linear actuators is less than 12V, the linear actuator will still extend/retract, but not at its maximum speed. This implies that we can alter the speed of the linear actuator by changing the voltage. Nevertheless, this method is not commonly used due to the difficulty in controlling the voltage of the power source. Therefore, the voltage of the power source is kept constant and the speed of the linear actuator is regulated with a PWM signal. The greater the duty cycle of the PWM, the faster the linear actuator extends/retracts.
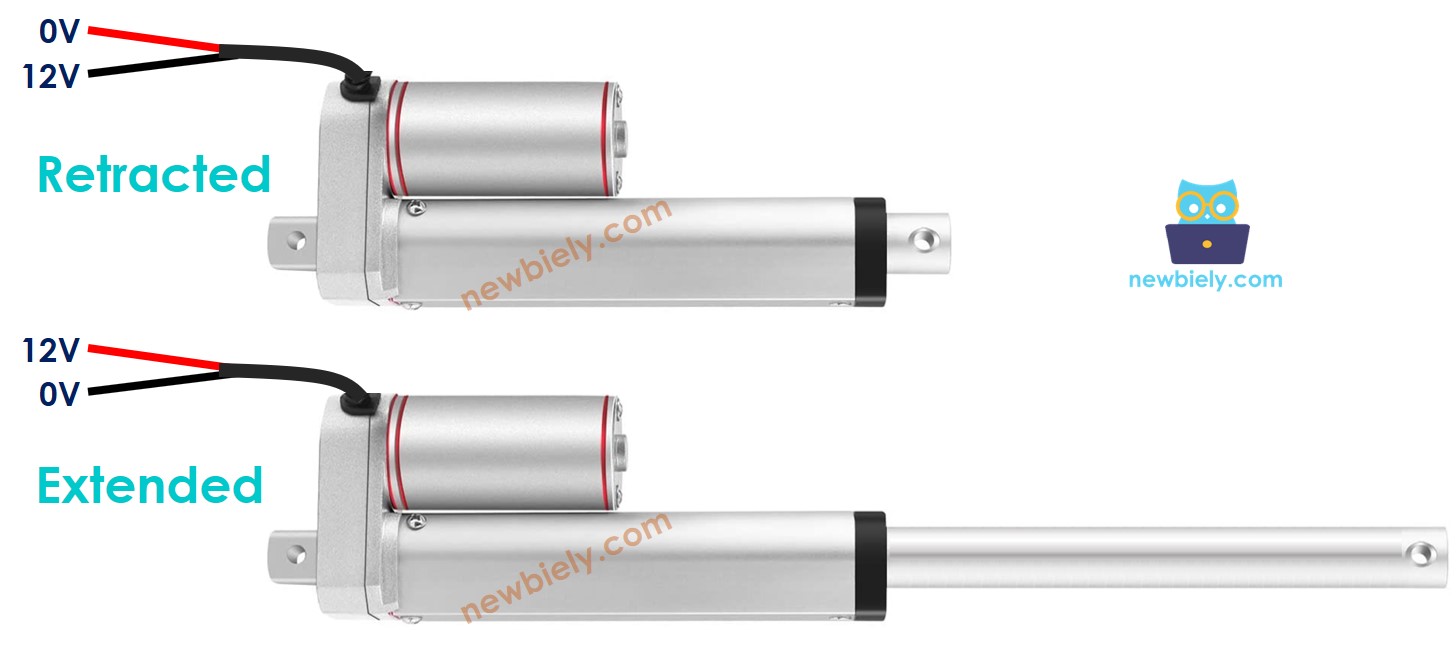
How to control a linear actuator using Raspberry Pi
Controlling a linear actuator involves:
- Extending the linear actuator at its maximum rate.
- Retracting the linear actuator at its maximum rate.
- (Optional) Regulating the extending/retracting speed.
Raspberry Pi is able to generate a signal to control the linear actuator, however, this signal has low voltage and current. Therefore, a hardware driver is required in between Raspberry Pi and linear actuator to amplify the control signal from Raspberry Pi, as well as to receive another control signal from Raspberry Pi to switch the pole of power supply for direction control.
※ NOTE THAT:
- This tutorial can be used with any linear actuator. 12V linear actuator is just one example.
- When controlling a 5V linear actuator, even though the Raspberry Pi pin outputs 5V (which is the same as the linear actuator voltage), a driver is still needed between the Raspberry Pi and the linear actuator because the Raspberry Pi pin does not provide enough current for the linear actuator.
There are numerous types of chips and modules available such as L293D and L298N that can be used as linear actuator drivers. In this tutorial, we will be using the L298N driver.
Overview of L298N Driver
The L298N Driver can be used to manage a linear actuator, a DC motor, and a stepper motor. This tutorial instructs you how to utilize it for controlling the linear actuator.
L298N Driver Pinout
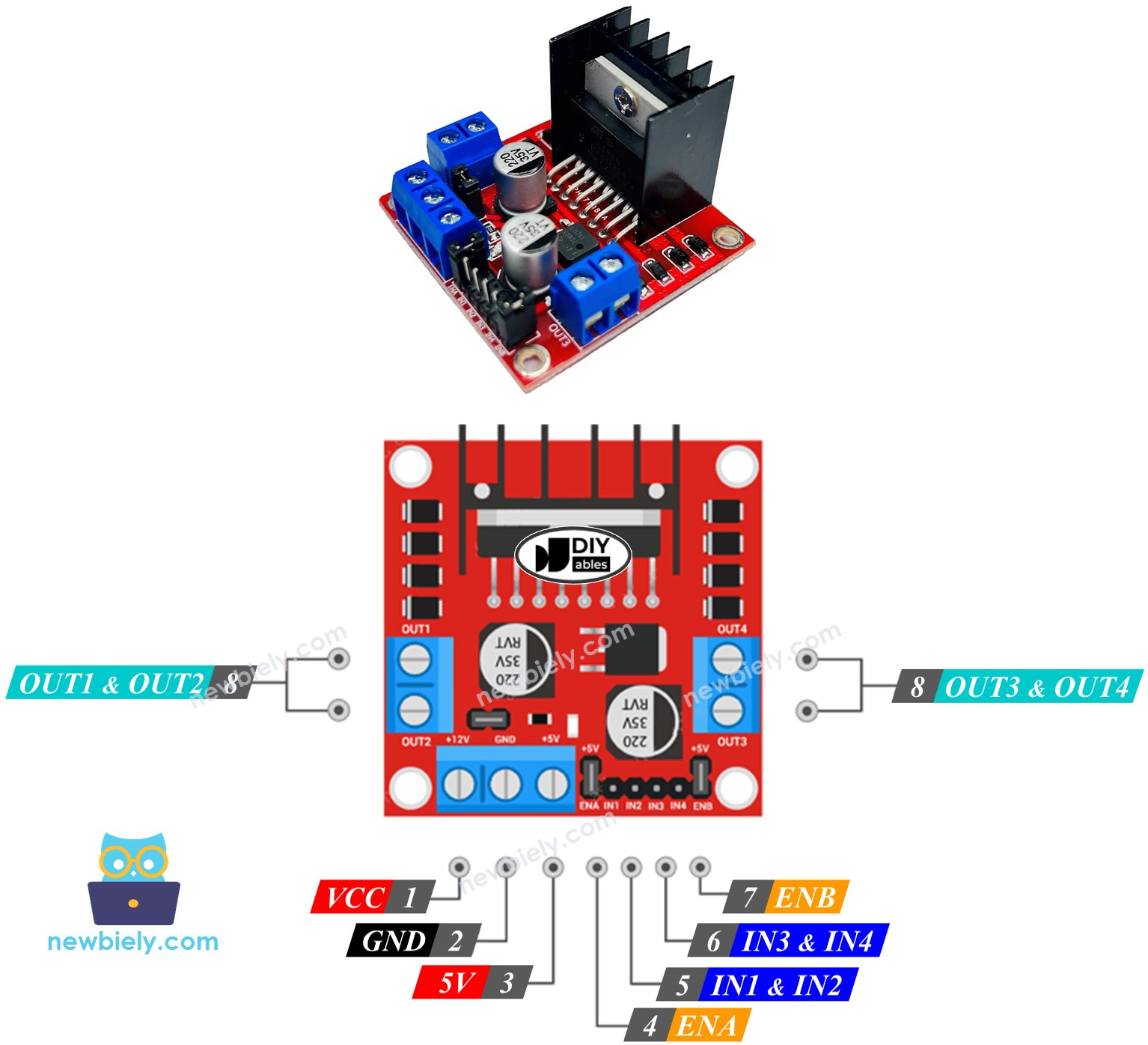
The L298N Driver has two channels, labelled A and B. This allows it to control two linear actuators independently at the same time. Suppose linear actuator A is connected to channel A and linear actuator B is connected to channel B. The L298N Driver has 13 pins in total.
The common pins for both channels:
- VCC pin: Supplies power for the linear actuator, with a voltage range of 5 to 35V.
- GND pin: This is a common ground pin, and must be connected to 0V (GND).
- 5V pin: Powers the L298N module, and can be supplied by 5V from a Raspberry Pi.
Channel A pins:
- ENA pins: These pins are used to control the speed of linear actuator A. By removing the jumper and connecting this pin to PWM input, we can control the rate of extension/retraction of linear actuator A.
- IN1 & IN2 pins: These pins are used to control the direction of a linear actuator. When one of them is HIGH and the other is LOW, the linear actuator will extend or retract. If both the inputs are either HIGH or LOW, then the linear actuator will stop.
- OUT1 & OUT2 pins: These pins are connected to linear actuator A.
Channel B pins:
- ENB pins: The jumper can be removed and the pin connected to a PWM input to control the speed of linear actuator B.
- IN3 & IN4 pins: By setting one of these pins to HIGH and the other to LOW, the linear actuator will move in either direction. If both are set to the same state (HIGH or LOW) the actuator will stop.
- OUT3 & OUT4 pins: These pins are connected to the linear actuator.
As previously stated, the L298N driver requires two power sources:
- One for the linear actuator (VCC and GND pins): ranging from 5 to 35V.
- One for the internal operation of the L298N module (5V and GND pins): ranging from 5 to 7V.
Remove all the jumpers from the L298N driver for the sake of simplicity.
We can manage two linear actuators independently simultaneously by utilizing a Raspberry Pi and an L298N Driver. To command each linear actuator, we only require three pins from the Raspberry Pi.
※ NOTE THAT:
The remainder of this tutorial will focus on controlling a linear actuator through channel A. Operating the other linear actuator is similar.
How To Control Linear Actuator
We will be studying the technique of utilizing the L298N driver to manage a Linear Actuator.
Wiring Diagram
Take out all three jumpers from the L298N module prior to connecting it.
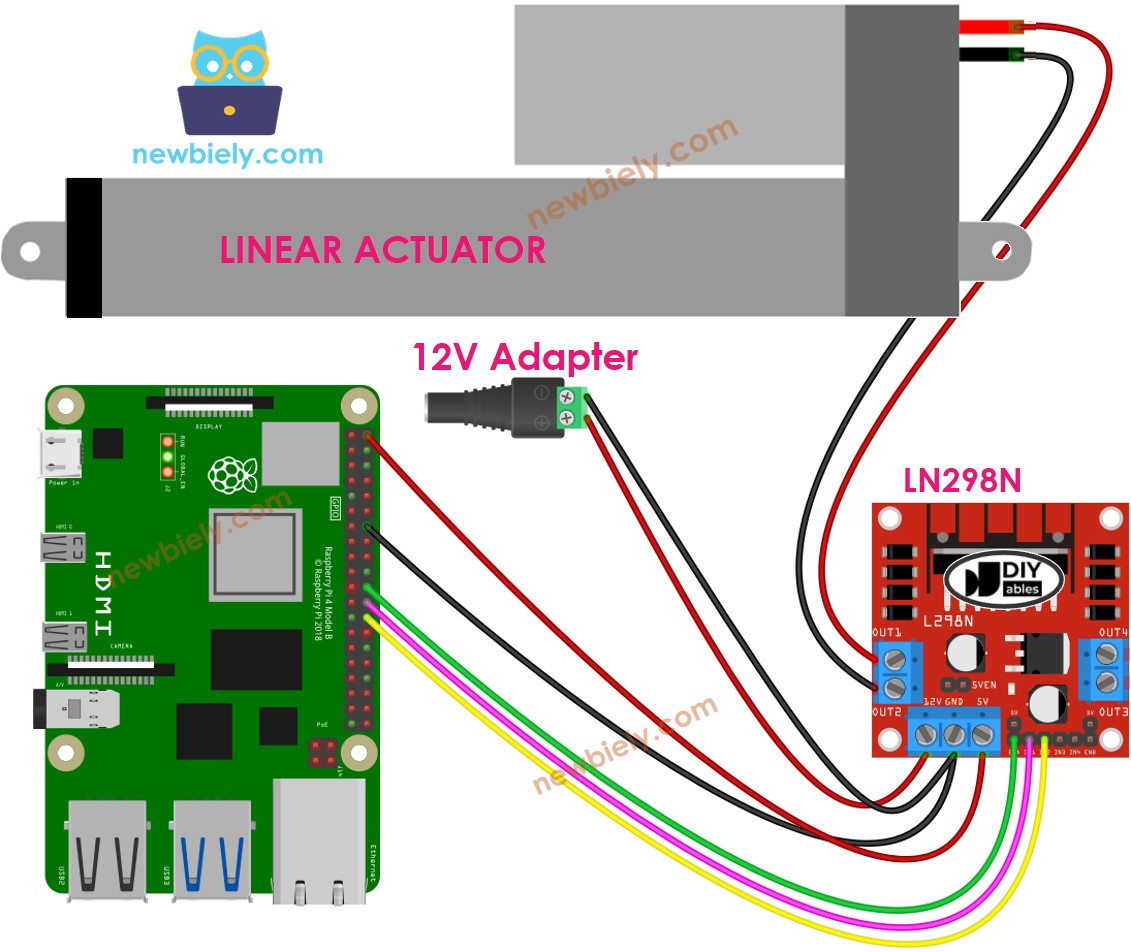
This image is created using Fritzing. Click to enlarge image
How To Make Linear Actuator Expand/Retract
The Linear Actuator's moving direction can be regulated by providing a logic HIGH/LOW to IN1 and IN2 pins. The table below shows how to control the direction in both channels.
IN1 pin | IN2 pin | Direction |
---|---|---|
LOW | LOW | Linear Actuator A stops |
HIGH | HIGH | Linear Actuator A stops |
HIGH | LOW | Linear Actuator A extends |
LOW | HIGH | Linear Actuator A retracts |
- Increase the length of Linear Actuator A.
- Reverse the Linear Actuator A
※ NOTE THAT:
The orientation of the linear actuator can be reversed by connecting the OUT1 and OUT2 pins in a different order. To do this, either the OUT1 and OUT2 pins must be swapped or the control signals on the IN1 and IN2 pins must be altered in the code.
How To Stop Linear Actuator from Extending or Retracting
The linear actuator will cease extending/retracting when it reaches the limit. It is also possible to programmatically halt its extension/retraction before it reaches the limit.
There are two methods to halt linear actuator:
- Regulating the speed to 0
- Disconnecting the power source
- Sets the IN1 and IN2 pins to the same value, either LOW or HIGH.
- Or
How To Control the Speed of Linear Actuator via L298N Driver
It is easy to regulate the speed of the linear actuator. Instead of setting the ENA pin to HIGH, we can generate a PWM signal to the ENA pin. This can be done by:
- Connecting a Raspberry Pi pin to the ENA of the L298N
- Generating a PWM signal to the ENA pin by using the pwm.ChangeDutyCycle() function. The L298N Driver amplifies the PWM signal to the linear actuator.
The speed is a value ranging from 0 to 255. When the speed is 0, the linear actuator will cease movement. At a speed of 255, the linear actuator will extend/retract at its maximum rate.
Raspberry Pi Example Code
The code below:
- Controls the linear actuator to its fullest extent at maximum speed
- Controls the linear actuator to its minimum extent at maximum speed
Detailed Instructions
Take out all three jumpers from the L298N module. And then:
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Create a Python script file actuator.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
You should observe:
- The linear actuator extending until it reaches the limit, then halting.
- The linear actuator staying in that position for a certain amount of time.
- The linear actuator retracting until it reaches the limit, then halting.
- The linear actuator staying in that position for a certain amount of time.
- This cycle repeating itself.