Raspberry Pi - Cooling System using DS18B20 Temperature Sensor
This tutorial instructs you how to regulate temperature with the help of a Raspberry Pi, a fan and a DS18B20 temperature sensor.
- When the temperature is too high, Raspberry Pi turns on the cooling fan.
- When the temperature is low, Raspberry Pi turns off the cooling fan.
If you would like to use either a DHT11 or DHT22 instead of the DS18B20 sensor, please refer to Raspberry Pi - Cooling System using DHT Sensor.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Buy Note: Many DS18B20 sensors available in the market are unreliable. We strongly recommend buying the sensor from the DIYables brand using the link provided above. We tested it, and it worked reliably.
Overview of Cooling Fan and DS18B20 Temperature Sensor
The fan utilized in this tutorial requires a 12v power supply. If the power is supplied, the fan will turn on, and if not, it will not. To control the fan with Raspberry Pi, a relay must be placed between them.
If you are unfamiliar with temperature sensors and fans (their pinouts, how they operate, how to program them, etc.), the following tutorials can help you out:
Wiring Diagram
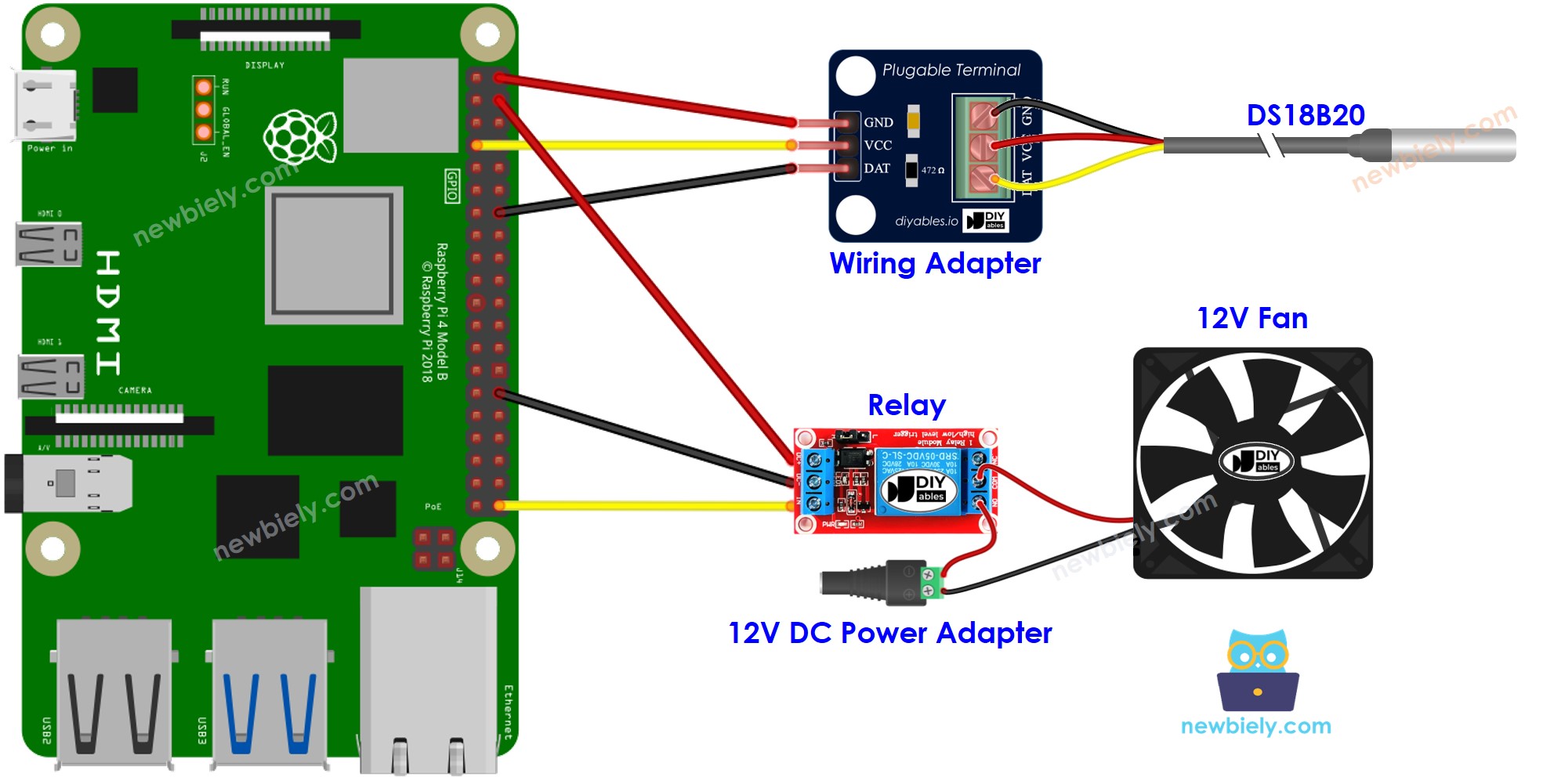
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
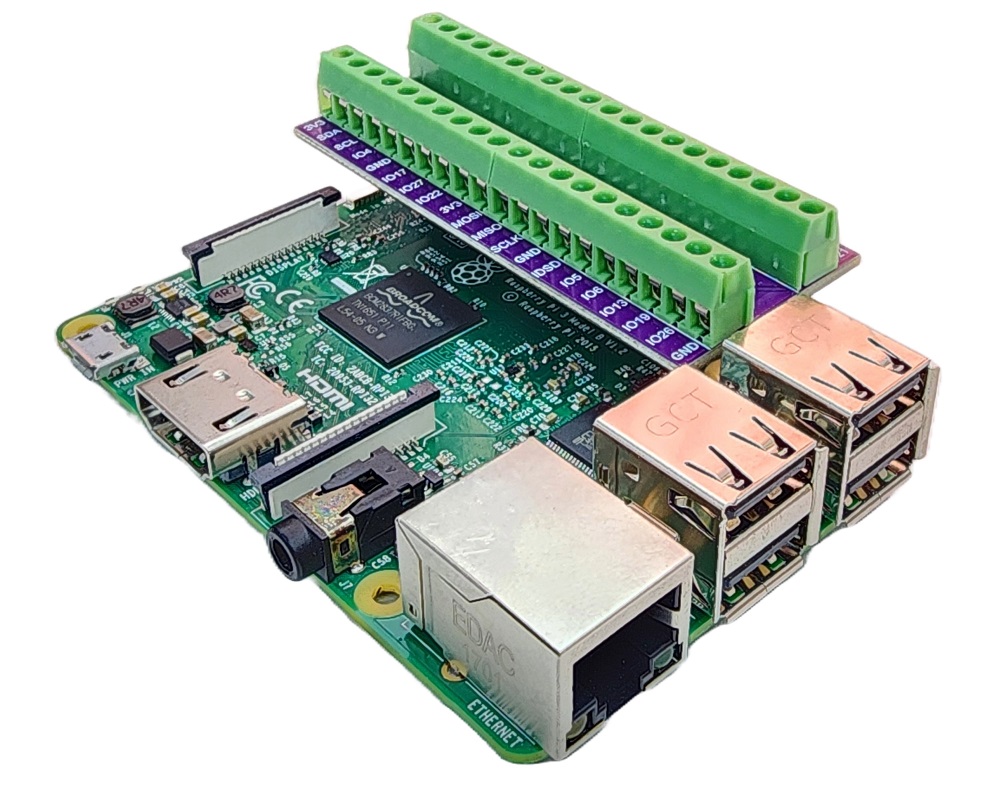
We recommend buying a DS18B20 sensor along with its accompanying wiring adapter for a seamless setup. This adapter includes an integrated resistor, removing the need for an additional resistor in the wiring.
How System Works
- The Raspberry Pi will take a reading from the temperature sensor.
- If the temperature is higher than the maximum allowed, the fan will be activated by the Raspberry Pi.
- When the temperature drops below the minimum accepted level, the Raspberry Pi will switch off the fan.
The loop is repeated continuously.
If you wish to activate and deactivate the fan when the temperature is above or below a certain value, all you have to do is set the upper and lower limits to the same number.
Raspberry Pi Code for Cooling System with DS18B20 sensor
Let's write the code that make the Raspberry Pi activate the fan when the temperature is higher than 25°C and will keep it running until the temperature drops below 20°C.
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Prior to utilizing the DS18B20 temperature sensor with a Raspberry Pi, we need to enable 1-Wire interface on Raspberry Pi. See How to enable 1-Wire interface on Raspberry Pi
- Install the library for DS18B20 temperature sensor by running the following command:
- Create a Python script file cooling.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
- Change the temperature of the environment around the sensor.
- Check the status of the fan on the Serial Monitor.
Advanced Knowledge
This controlling method is referred to as an on-off controller, which is also known as a signaller or "bang-bang" controller. It is easy to implement this method.
An alternative approach, known as the PID controller, exists. This method of temperature control is more stable, but it is complex and challenging to comprehend and implement. As a result, the PID controller is not widely used.