Raspberry Pi - Keypad
This tutorial instructs you how to use Raspberry Pi with keypad 3x4 and 4x4. In detail, we will learn:
- How to connect Raspberry Pi to keypad 3x4 and keypad 4x4.
- How to program Raspberry Pi to read value from keypad 3x4 and keypad 4x4.
- How Raspberry Pi checks the password inputted from keypad 3x4 and keypad 4x4.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Keypad
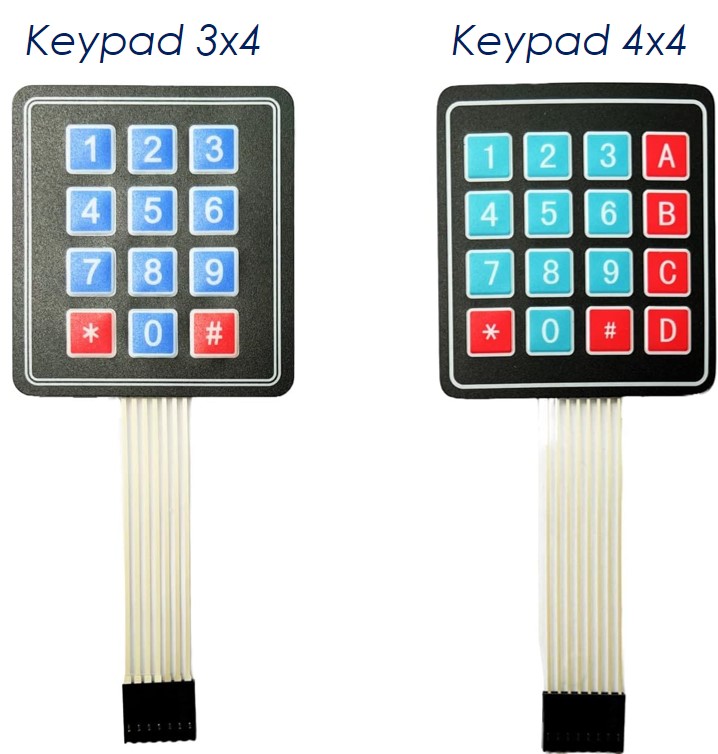
A keypad is a collection of keys that are organized in rows and columns, referred to as a matrix. Each individual button is known as a key. There are different kinds of keypads. Two of the most commonly used for DIY projects are the 3x4 (12 keys) and 4x4 (16 keys).
The Keypad Pinout
The pins are separated into two categories: rows and columns.
- A 3x4 keypad has seven pins:. Four of them are row-pins, labeled R1, R2, R3, and R4. The remaining three are column-pins, labeled C1, C2, and C3.
- A 4x4 keypad has eight pins:. Four of them are row-pins, labeled R1, R2, R3, and R4. The other four are column-pins, labeled C1, C2, C3, and C4.
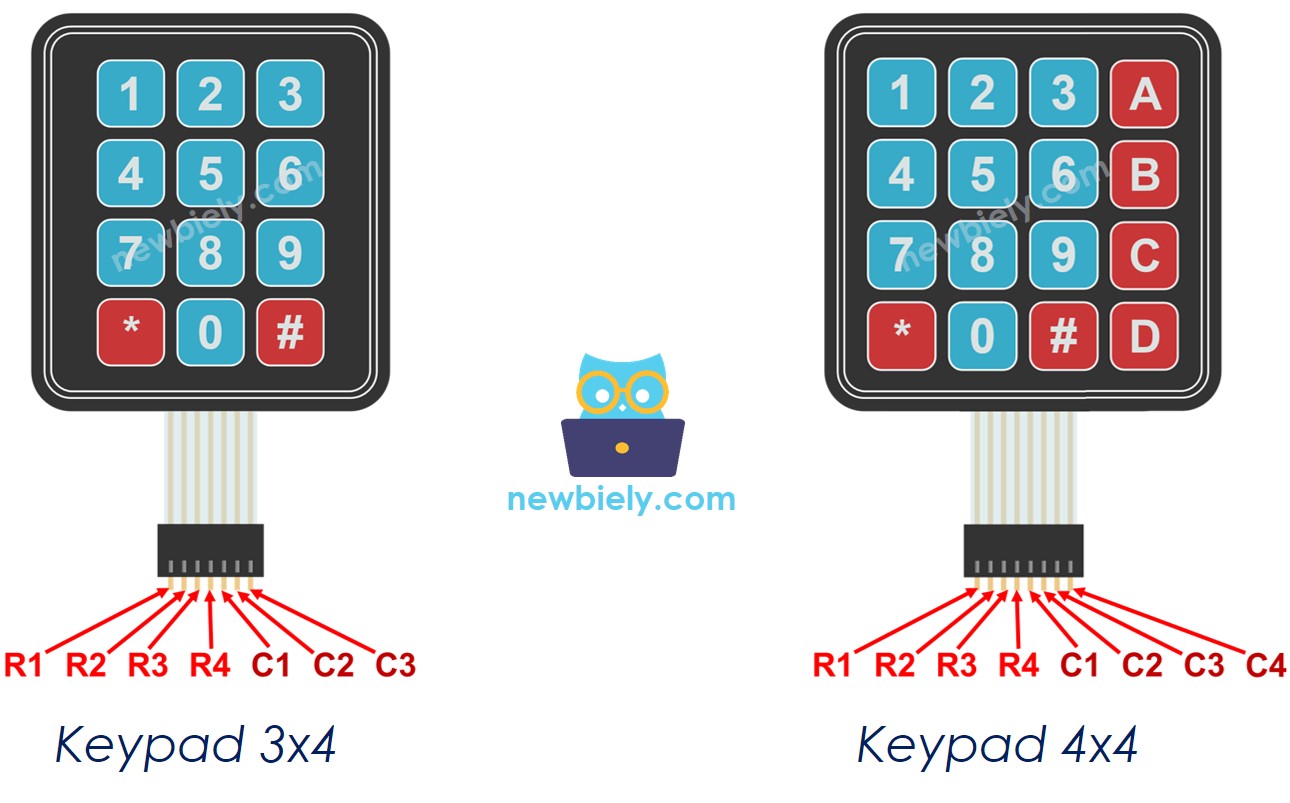
Wiring Diagram
- The wiring diagram between Raspberry Pi and keypad 3x4
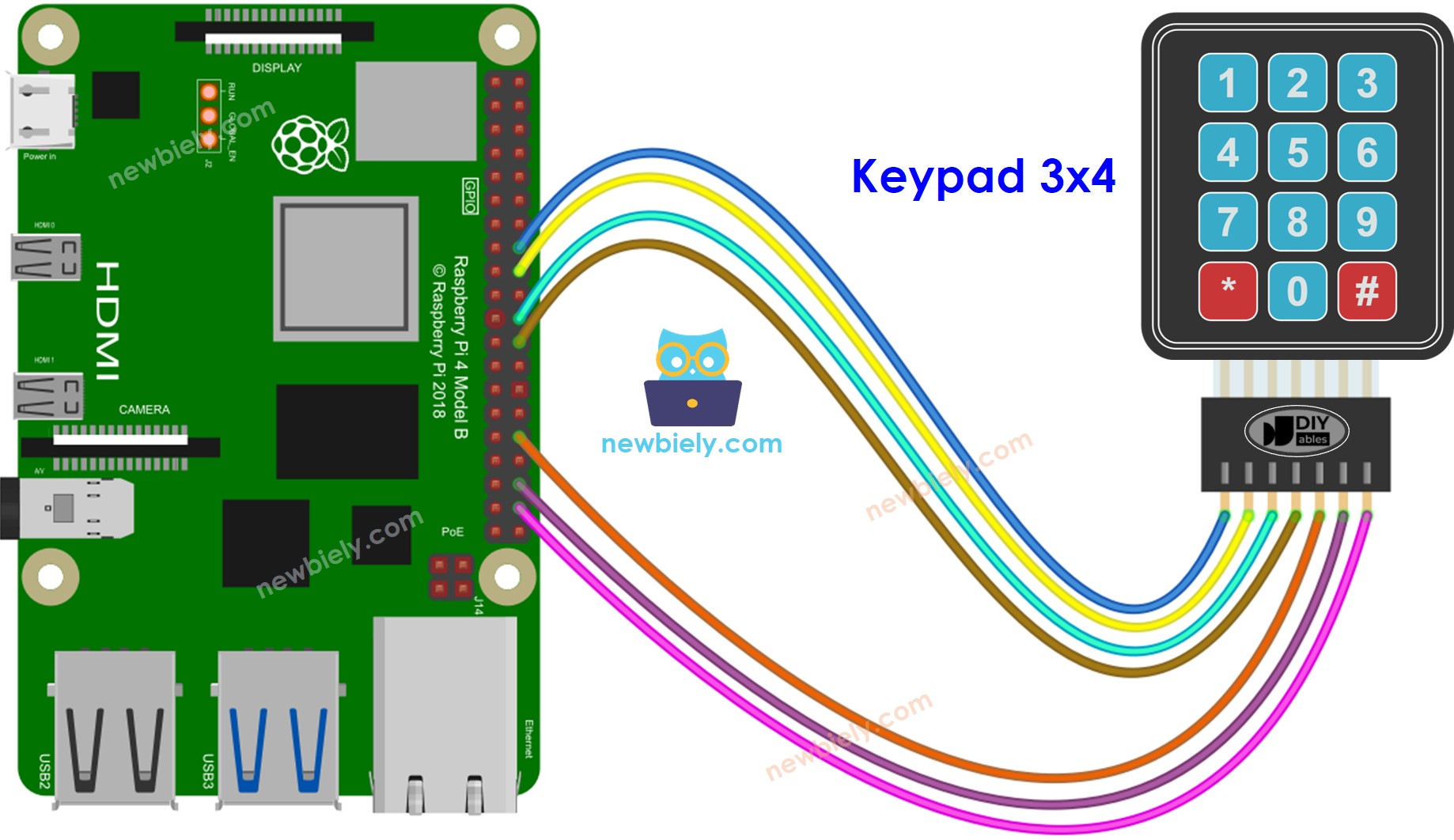
This image is created using Fritzing. Click to enlarge image
- The wiring diagram between Raspberry Pi and keypad 4x4
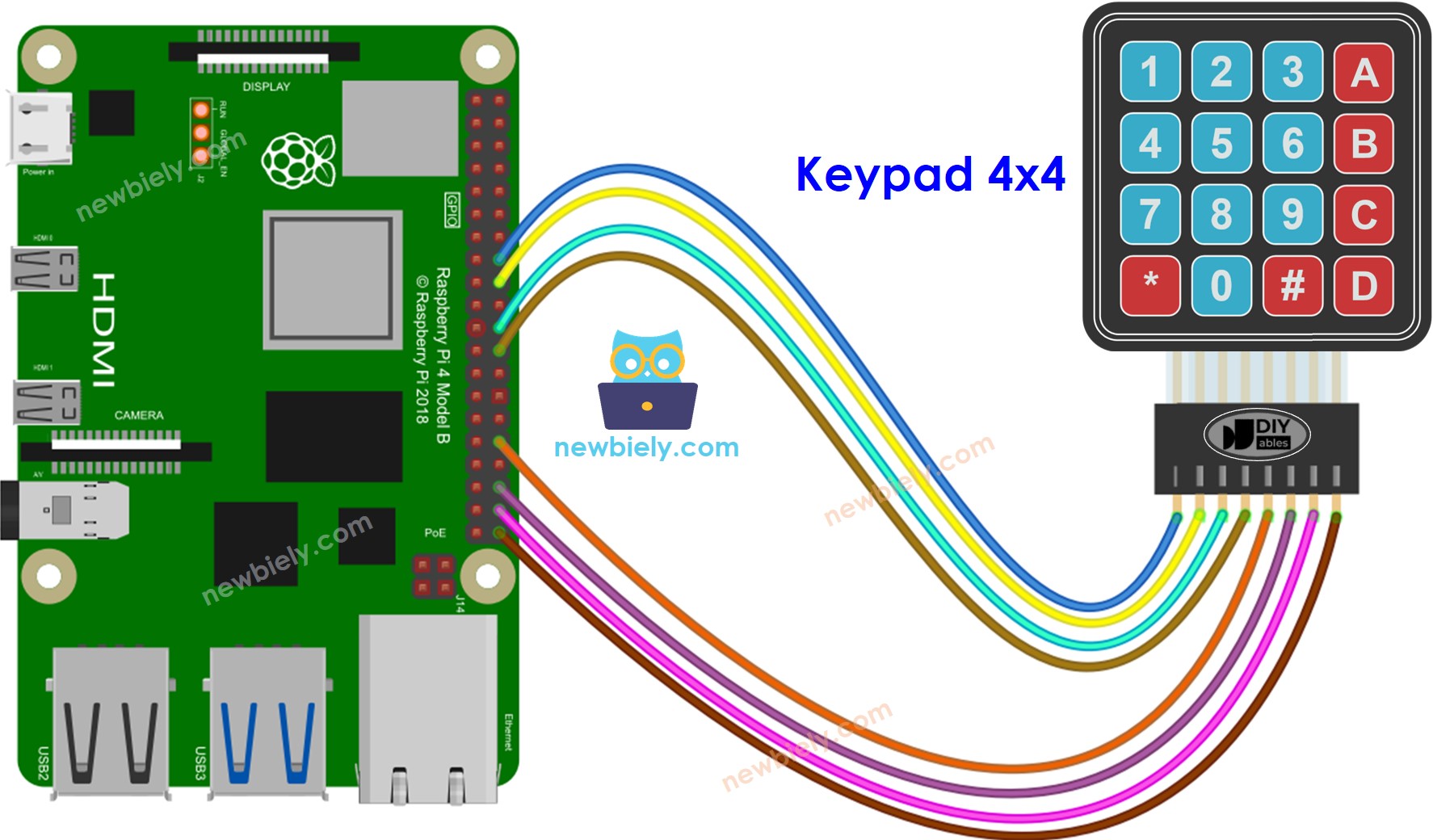
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
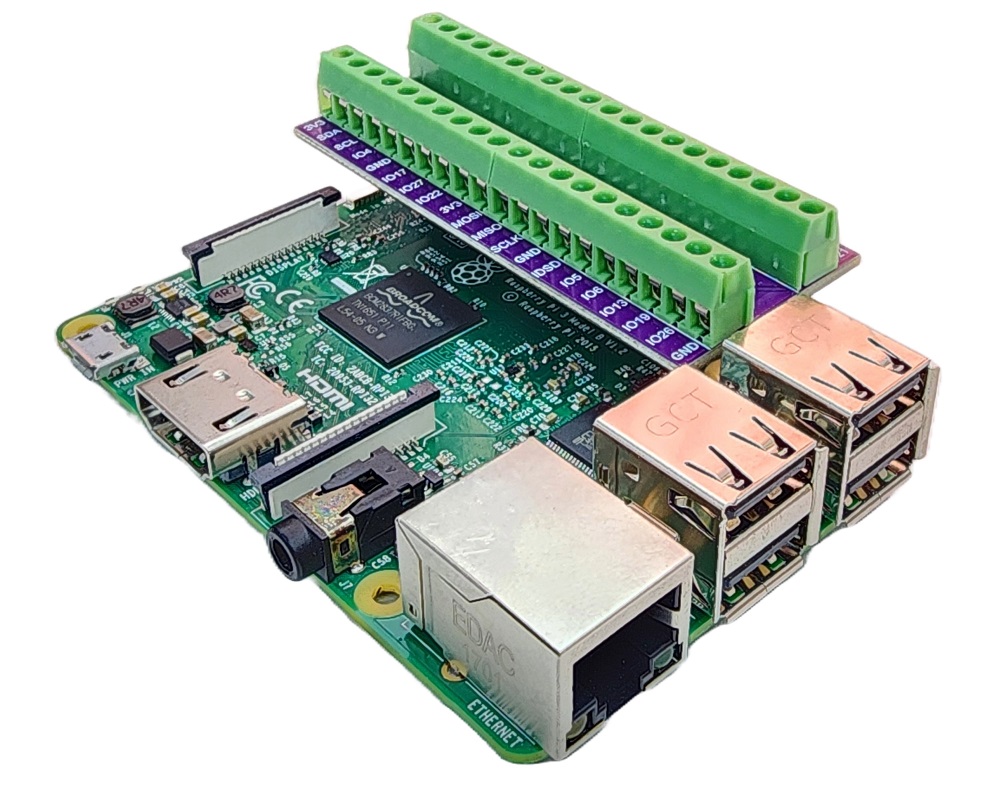
Raspberry Pi Code
Raspberry Pi Code for Keypad 3x4
Raspberry Pi Code for Keypad 4x4
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Create a Python script file keypad.py and add one of the above code.
- Save the file and run the Python script by executing the following command in the terminal:
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
- Press some keys on the keypad.
- Check out the result in the Serial Monitor.
Keypad and Password
A common use of a keypad is to enter a password. We designate two specific keys for this purpose:
- A key to initiate or restart the password input, such as the "*" key
- A key to end the password input, such as the "#" key
The password will comprise of a string that consists of all the other keys, apart from two specific special keys.
When a key is pressed:
- If the key is not "*" or "#", add the key to the user's input password string.
- If the key is "#", compare the user's input string with the valid passwords to determine if the input password is correct, then clear the user's input password string.
- If the key is "*", clear the user's input password string.
Keypad - Password Code
Detailed Instructions
- Create a Python script file keypad_password.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
- Run the code above.
- Open the Serial Monitor.
- Press "123456" followed by the "#" key.
- Press "1234" followed by the "#" key.
- Check out the result on the Serial Monitor.