Raspberry Pi - Button - Piezo Buzzer
This tutorial instructs you how to use an Raspberry Pi and a button to control a piezo buzzer. The following actions will be discussed in detail:
- When the button is pressed, the piezo buzzer will produce a sound.
- When the button is not pressed, the piezo buzzer will stop producing sound.
- When the button is pressed, the piezo buzzer will generate a melody.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Wiring Diagram
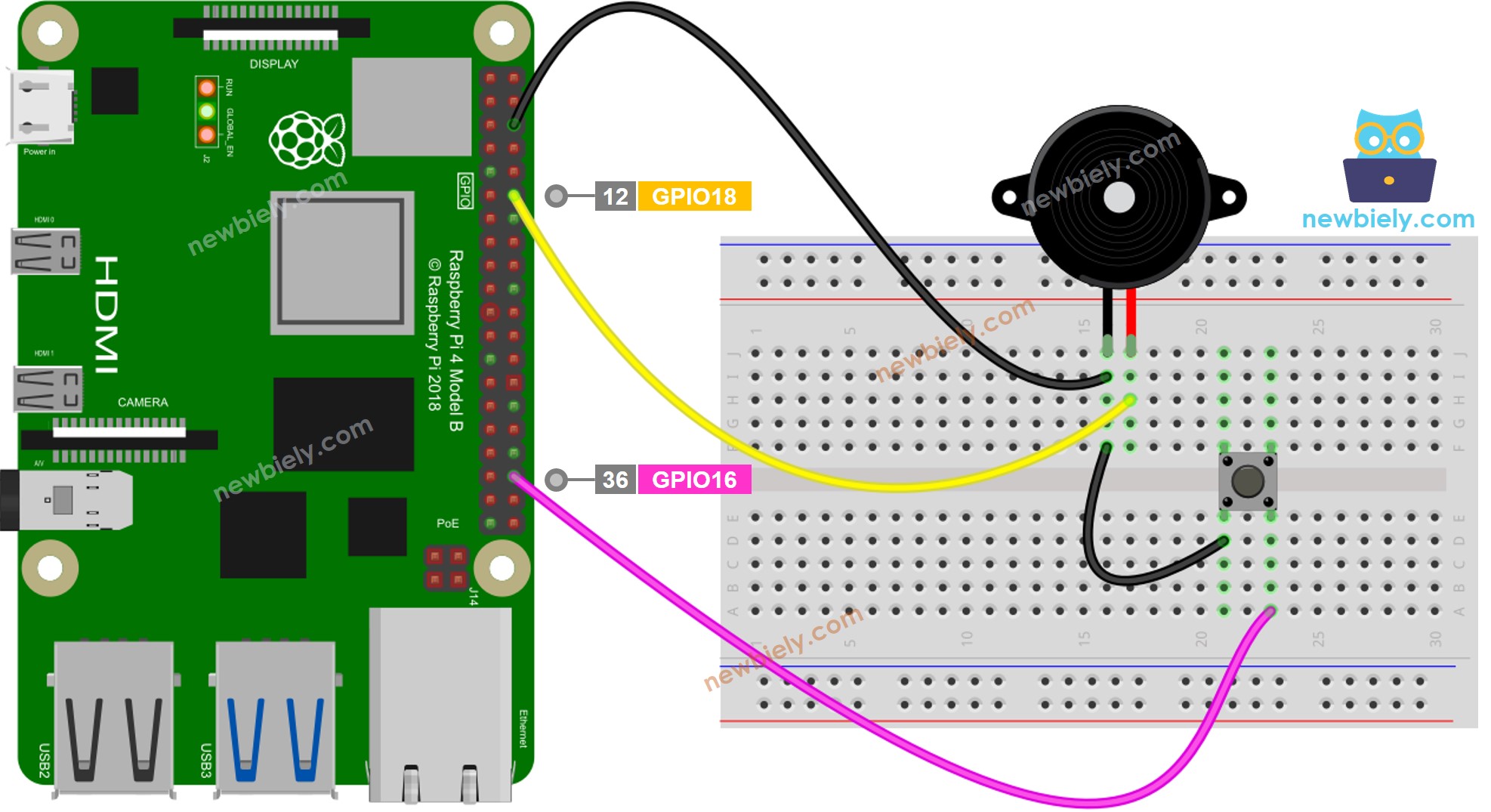
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
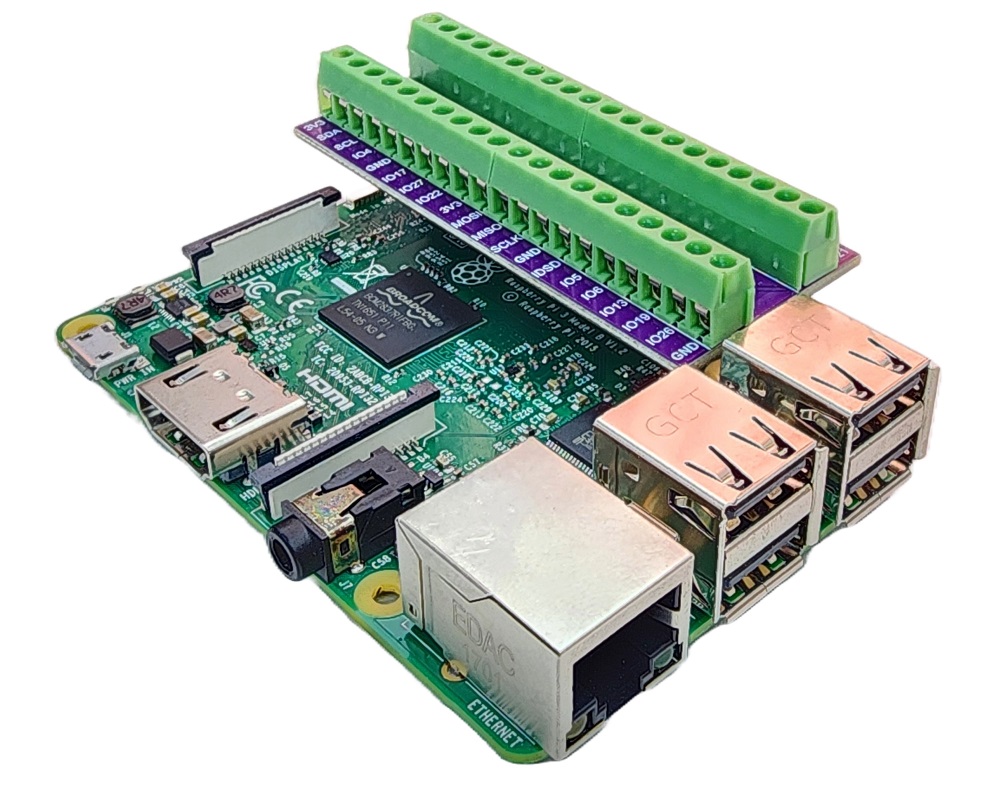
Raspberry Pi Code - Simple Sound
In this part, we will learn how to use a piezo buzzer to make a simple sound when the button is pressed using Raspberry Pi.
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Create a Python script file button_buzzer.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Press and hold the button for several seconds.
- Listen to the sound of the piezo buzzer.
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!
Raspberry Pi plays the melody of the song
In this part, we will make Raspberry Pi trigger the piezo buzzer to play the "Jingle Bells" song when a button is pressed
Detailed Instructions
- Create a Python script file button_buzzer_Jingle_Bells.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Press the button
- Then, listen to the piezo buzzer's melody.
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!