Raspberry Pi - Traffic Light
In this tutorial, we are going to learn how to use Raspberry Pi control the traffic light module. In detail, we will learn:
- How to connect the traffic light module to Raspberry Pi
- How to program Raspberry Pi to control RGB traffic light module
- How to program Raspberry Pi to control RGB traffic light module without using delay() function
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Traffic Light Module
Pinout
A traffic light module includes 4 pins:
- GND pin: The ground pin, connect this pin to GND of Raspberry Pi.
- R pin: The pin to control the red light, connect this pin to a digital output of Raspberry Pi.
- Y pin: The pin to control the yellow light, connect this pin to a digital output of Raspberry Pi.
- G pin: The pin to control the green light, connect this pin to a digital output of Raspberry Pi.
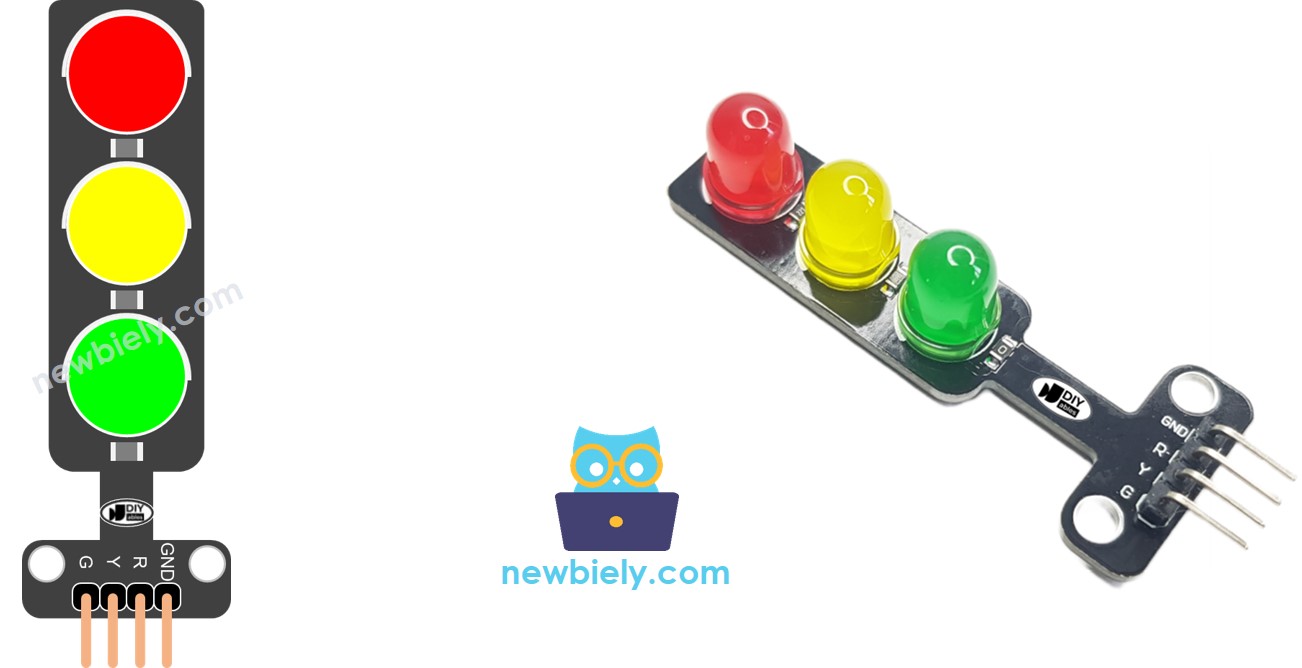
How It Works
Wiring Diagram
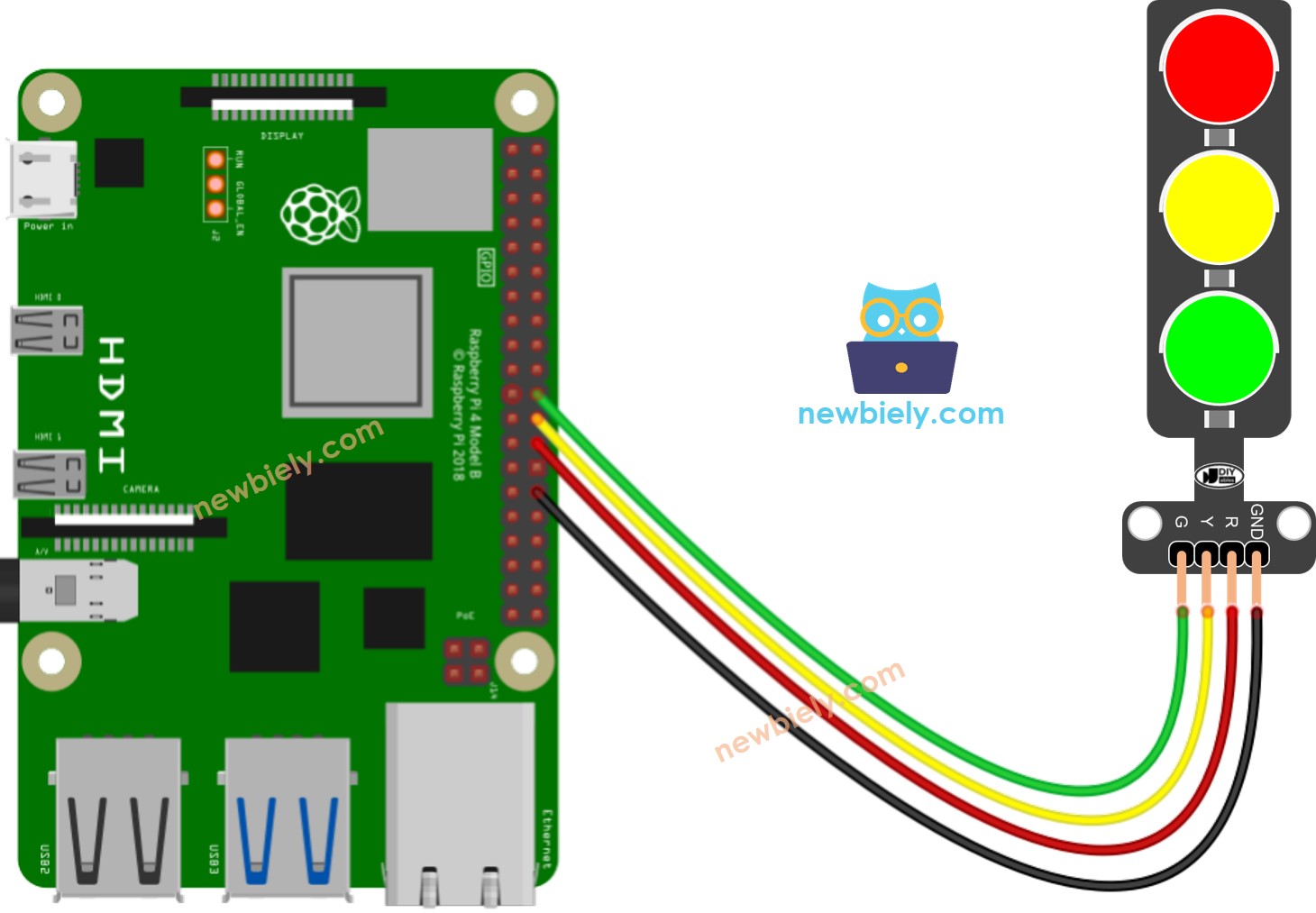
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
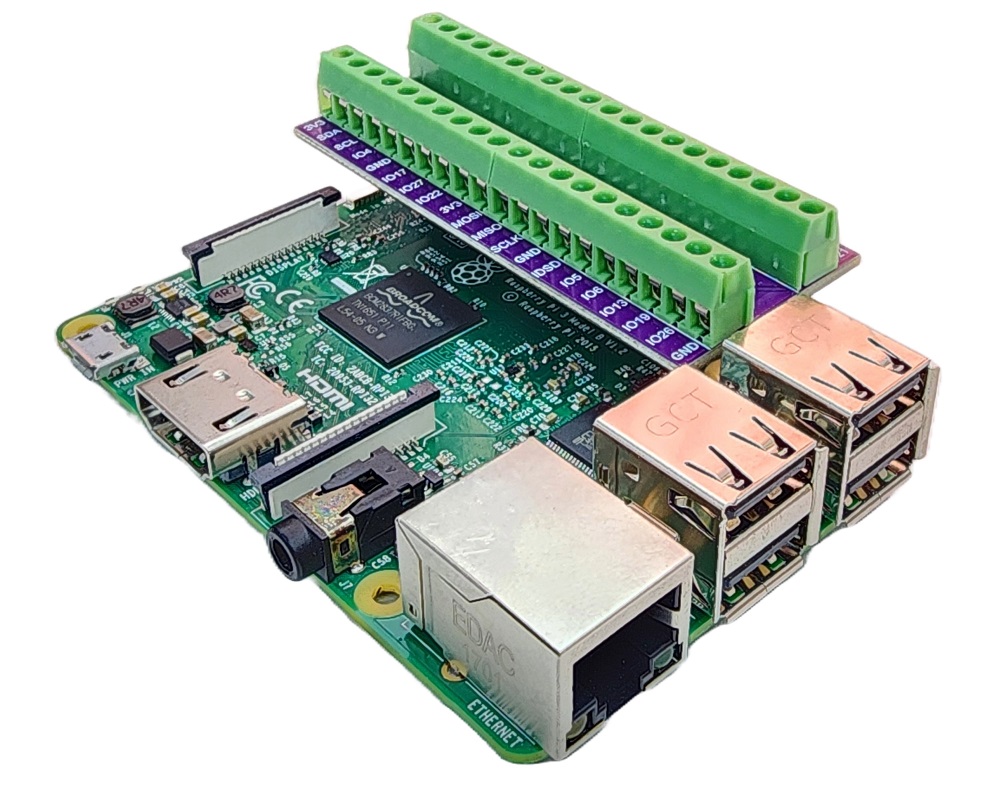
Raspberry Pi Code
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Create a Python script file traffic_light.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
- Check out the traffic light module
It's important to note that the exact workings of a traffic light can vary depending on the specific design and technology used in different regions and intersections. The principles described above provide a general understanding of how traffic lights operate to manage traffic and enhance safety on the roads.
The code above demonstrates individual light control. Now, let's enhance the code for better optimization.
Raspberry Pi Code Optimization
- Let's improve the code by implementing a function for light control.
- Let's improve the code by using a for loop.
- Let's improve the code by using millis() function intead of delay().