Raspberry Pi - Sound Sensor
The sound sensor has the capability to detect the presence of sound in its surroundings. It can be employed to create projects that respond to sound, like lights that activate with a clap or a pet feeder that responds to sound cues.
This tutorial instructs you how to use the Raspberry Pi and a sound sensor to detect sound. We will explore:
- How to connect the sound sensor to the Raspberry Pi
- How to program the Raspberry Pi to detect sound using the sound sensor.
Following that, you have the flexibility to modify the code and customize it to trigger an LED or a light (using a relay) upon sound detection. Alternatively, you can also configure it to control the rotation of a servo motor.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Sound Sensor
The sound sensor can detect sound in its surroundings. You can easily adjust the sensitivity of the sensor by using the built-in potentiometer.
Pinout
The sound sensor includes three pins:
- VCC pin: needs to be connected to VCC (3.3V to 5V)
- GND pin: needs to be connected to GND (0V)
- OUT pin: is an output pin: HIGH if quiet and LOW if sound is detected. This pin needs to be connected to Raspberry Pi's input pin.
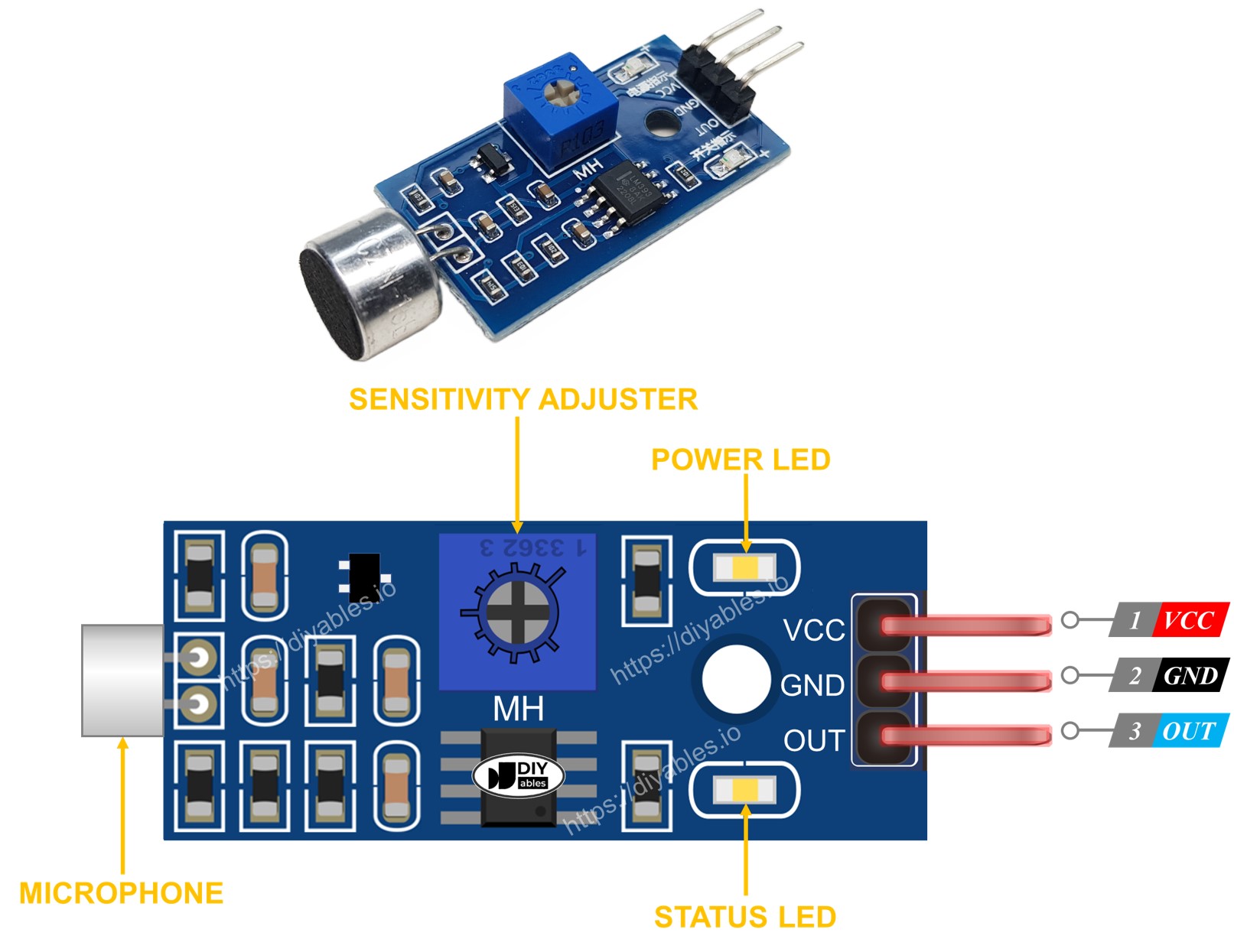
The sound sensor has a handy built-in potentiometer that allows you to adjust its sensitivity. Additionally, it features two LED indicators:
- One LED indicates the power status.
- Another LED indicates the sound state, turning on when there is sound and off when it's quiet.
How It Works
The module includes a convenient potentiometer that allows you to adjust the sound sensitivity. Here's how the output pin of the sensor behaves:
- When sound is detected, the output pin is set to LOW.
- When sound is not detected, the output pin is set to HIGH.
Wiring Diagram
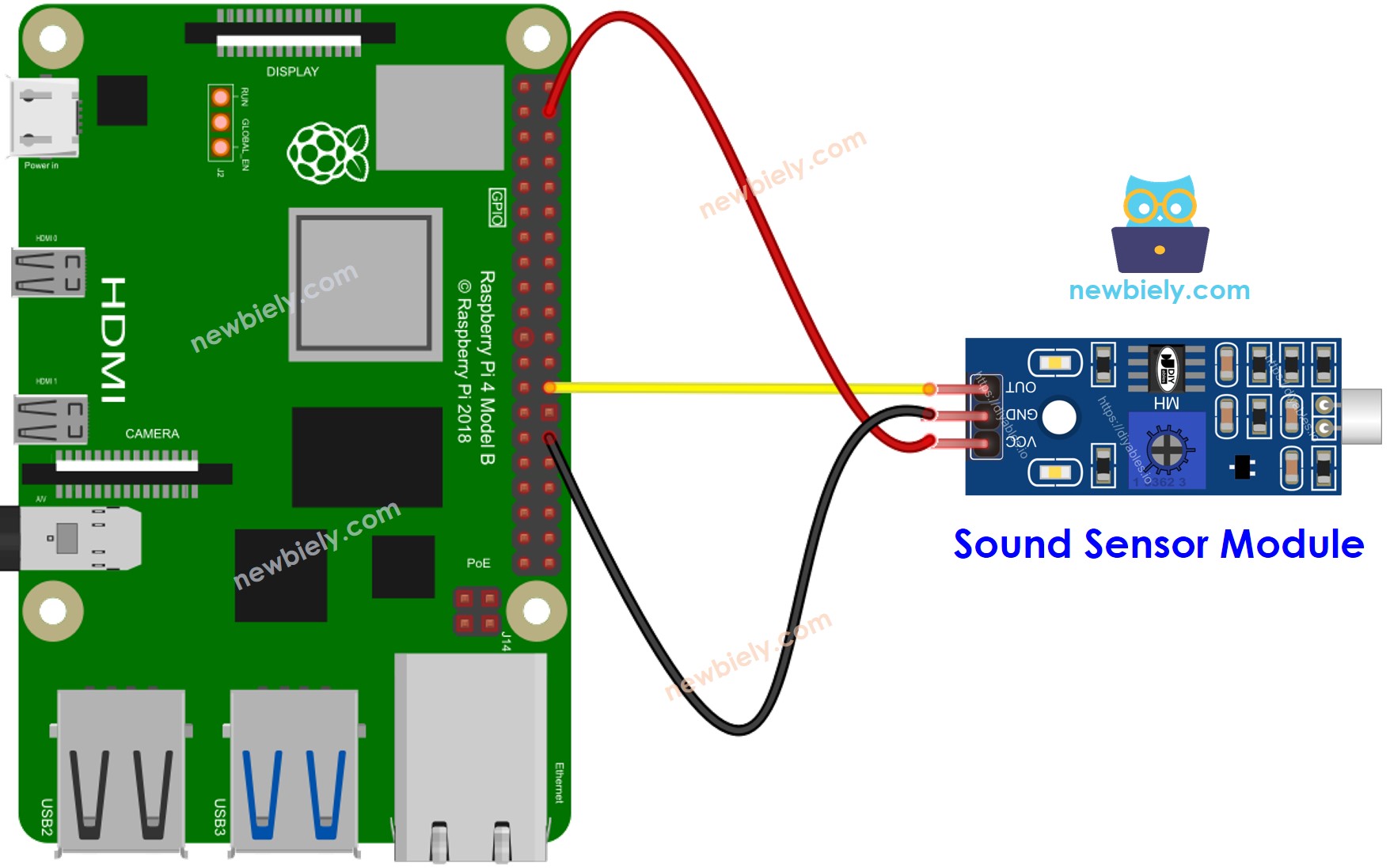
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
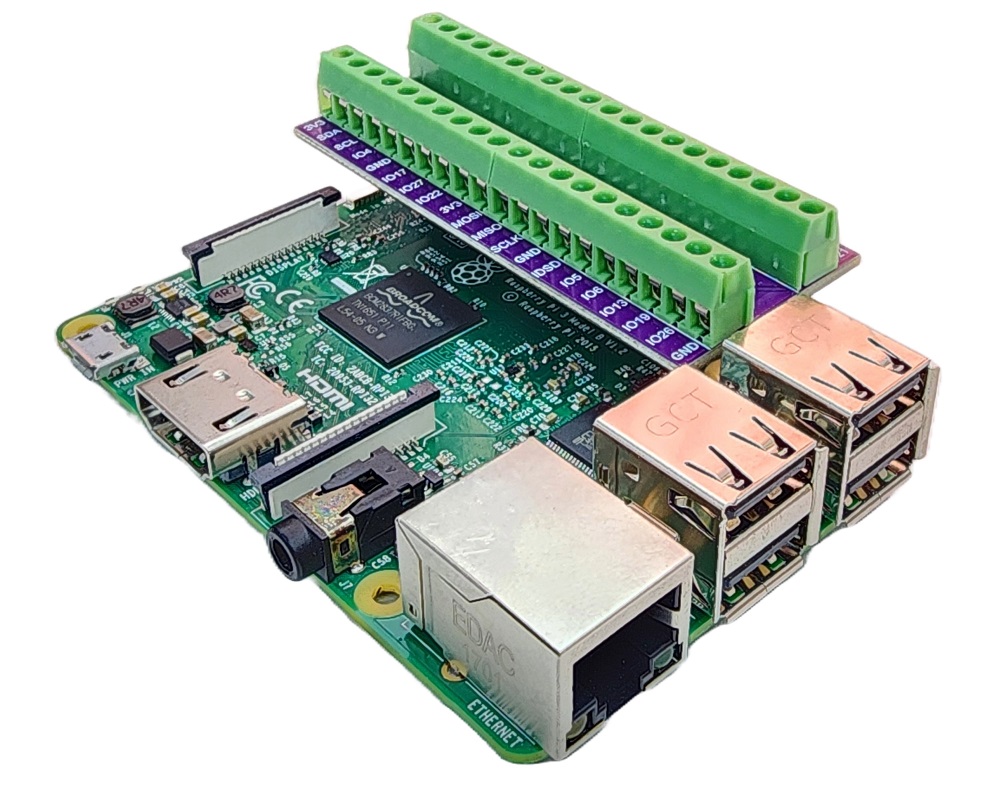
How To Program For Sound Sensor
- Initializes the Raspberry Pi pin to the digital input mode by using GPIO.setup() function.
- Reads the state of the Raspberry Pi pin by using GPIO.input() function.
Raspberry Pi Code - Detecting the sound
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Create a Python script file sound_sensor.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Clap your hand in front of the sound sensor
- See the result in the Terminal.
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
Please keep in mind that if you notice the LED status being continuously on or off, even when there is sound present, you may need to adjust the potentiometer to fine-tune the sound sensitivity of the sensor.
Now, we have the flexibility to modify the code and make it trigger an LED or a light when sound is detected. Additionally, we can even program it to rotate a servo motor. For detailed instructions and more information, please refer to the tutorials provided at the end of this guide.
Troubleshooting
If you encounter any issues with the sound sensor's functionality, try the following troubleshooting steps:
- Reduce vibrations: The sound sensor is sensitive to mechanical vibrations and wind noise. Mounting it on a stable surface can help minimize these disturbances.
- Consider the sensing range: Keep in mind that this sound sensor has a limited sensing range of approximately 10 inches. To obtain accurate readings, make sure the sound source is positioned closer to the sensor.
- Check the power supply: Ensure that the power supply is stable and free from any electrical noise. The sound sensor, being an analog circuit, can be affected by power supply disturbances.
By following these steps, you should be able to address any potential issues with the sound sensor.