Raspberry Pi - Ultrasonic Sensor
This tutorial instructs you how to use Raspberry Pi and ultrasonic sensor to measure the distance to obstacles or objects. In detail, we will learn:
- How ultrasonic sensor works
- How to connect the ultrasonic sensor to Raspberry Pi
- How to program Raspberry Pi to measure distance using the ultrasonic sensor
- How to filter out noise from the distance measurements of the ultrasonic sensor in Raspberry Pi code
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Ultrasonic Sensor
The HC-SR04 ultrasonic sensor is used to calculate the distance to an object by utilizing ultrasonic waves.
The Ultrasonic Sensor Pinout
The HC-SR04 ultrasonic sensor has four pins:
- VCC pin: must be connected to VCC (5V)
- GND pin: must be connected to GND (0V)
- TRIG pin: this pin receives a control signal (pulse) from Raspberry Pi
- ECHO pin: this pin sends a signal (pulse) to Raspberry Pi. Raspberry Pi measures the length of the pulse to calculate the distance.
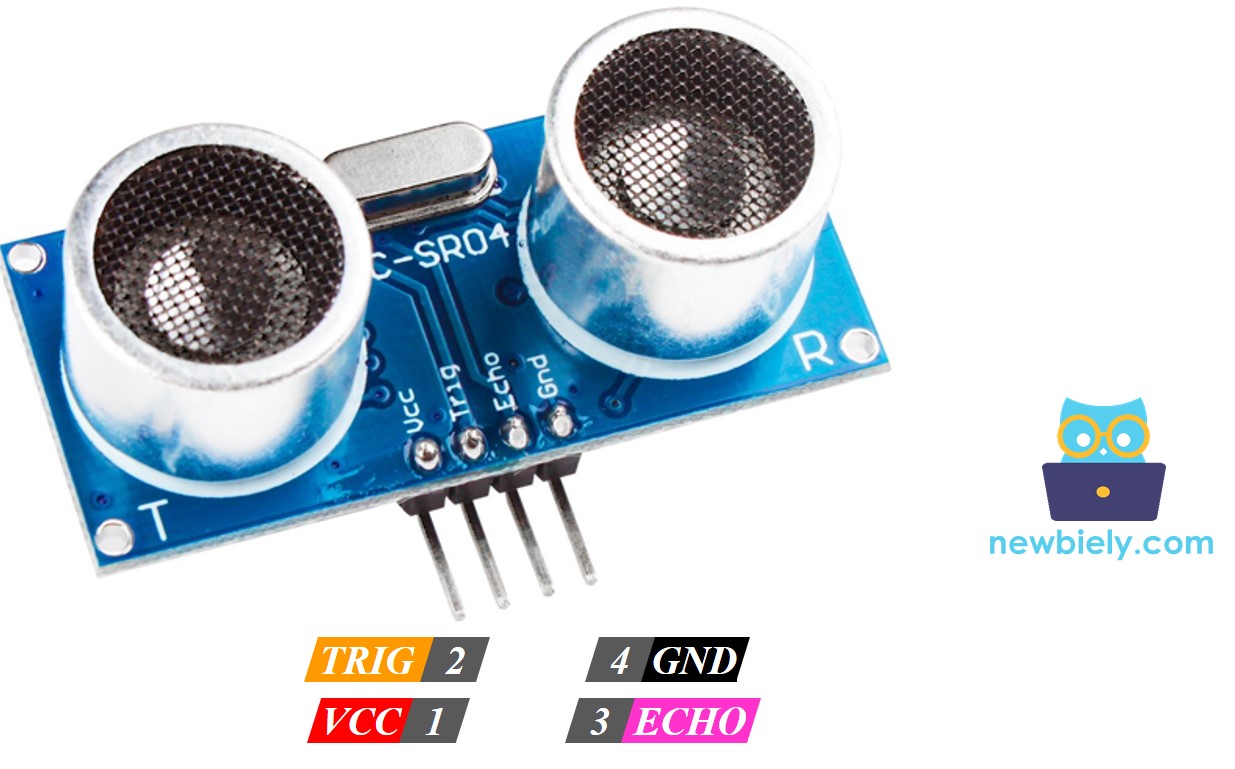
How It Works
- The micro-controller generates a 10-microsecond pulse on the TRIG pin, which causes the ultrasonic sensor to automatically emit ultrasonic waves.
- When the waves hit an obstacle, they are reflected back to the sensor.
- The ultrasonic sensor then detects the reflected wave and measures its travel time.
- As a result, the ultrasonic sensor generates a pulse to the ECHO pin, with the duration of the pulse being equal to the travel time of the ultrasonic wave.
- The micro-controller measures the pulse duration in the ECHO pin and calculates the distance between the sensor and the obstacle.
How to Get Distance From Ultrasonic Sensor
- To calculate the distance from the ultrasonic sensor, two steps must be taken as outlined in the "How It Works" section (steps 1 and 6):
- Generate a 10-microsecond pulse on the TRIG pin.
- Measure the pulse duration in the ECHO pin.
- Utilize the measured pulse duration to calculate the distance between the sensor and the obstacle.
Distance Calculation
We have:
- The travel time of the ultrasonic wave (µs): travel_time = pulse_duration
- The speed of the ultrasonic wave: speed = SPEED_OF_SOUND = 340 m/s = 0.034 cm/µs
So:
- The travel distance of the ultrasonic wave (cm): travel_distance = speed × travel_time = 0.034 × pulse_duration
- The distance between sensor and obstacle (cm): distance = travel_distance / 2 = 0.034 × pulse_duration / 2 = 0.017 × pulse_duration
Raspberry Pi - Ultrasonic Sensor
The pins of a Raspberry Pi can create a 10-microsecond pulse and measure the length of the pulse. This allows us to calculate the distance from the ultrasonic sensor by using two Raspberry Pi pins. So, we just need to use two Raspberry Pi's pins:
- One pin is connected to the TRIG pin of the ultrasonic sensor in order to generate a 10µs pulse.
- The other pin is connected to the ECHO pin of the ultrasonic sensor to measure the pulse from the sensor
Wiring Diagram
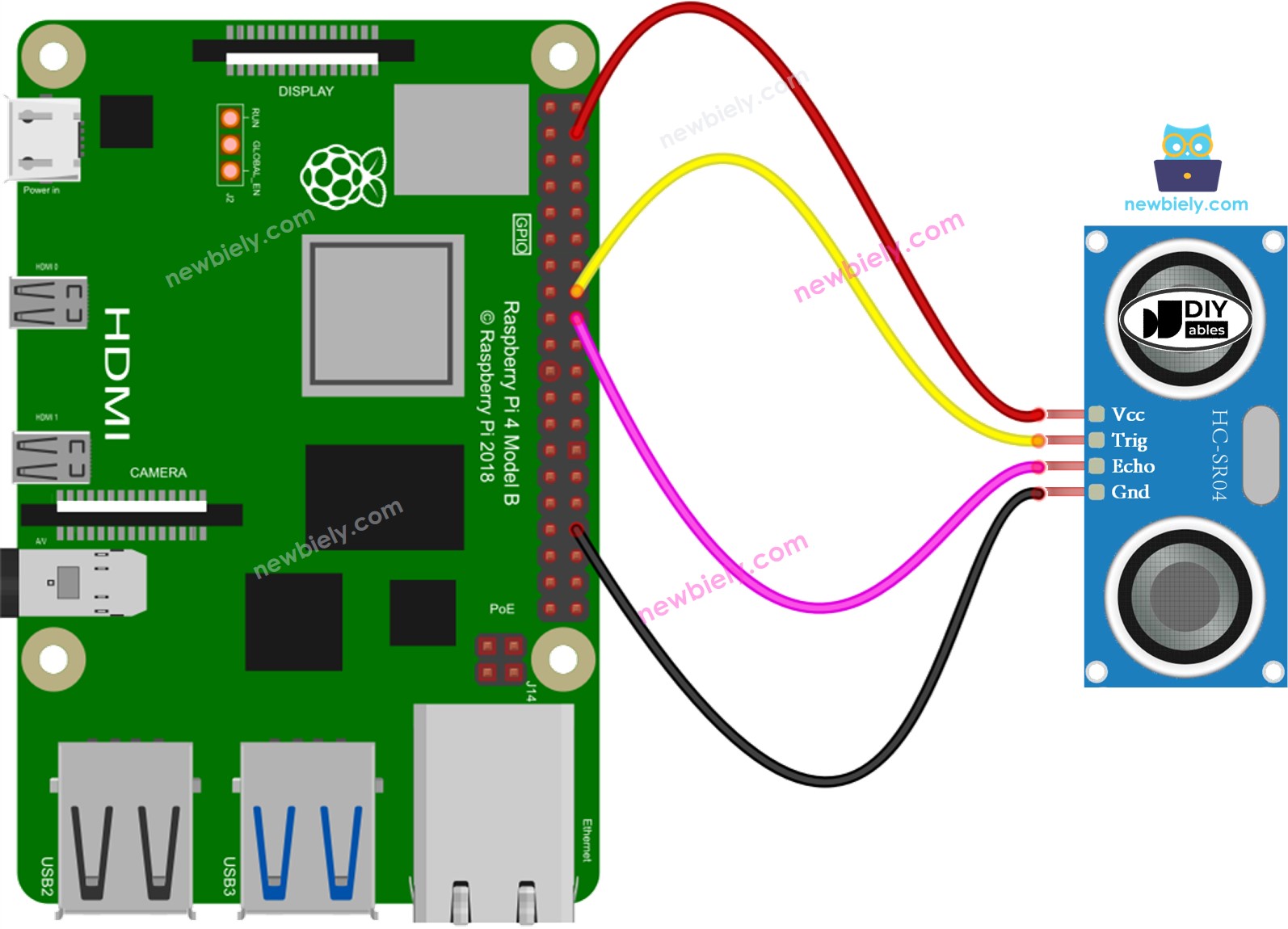
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
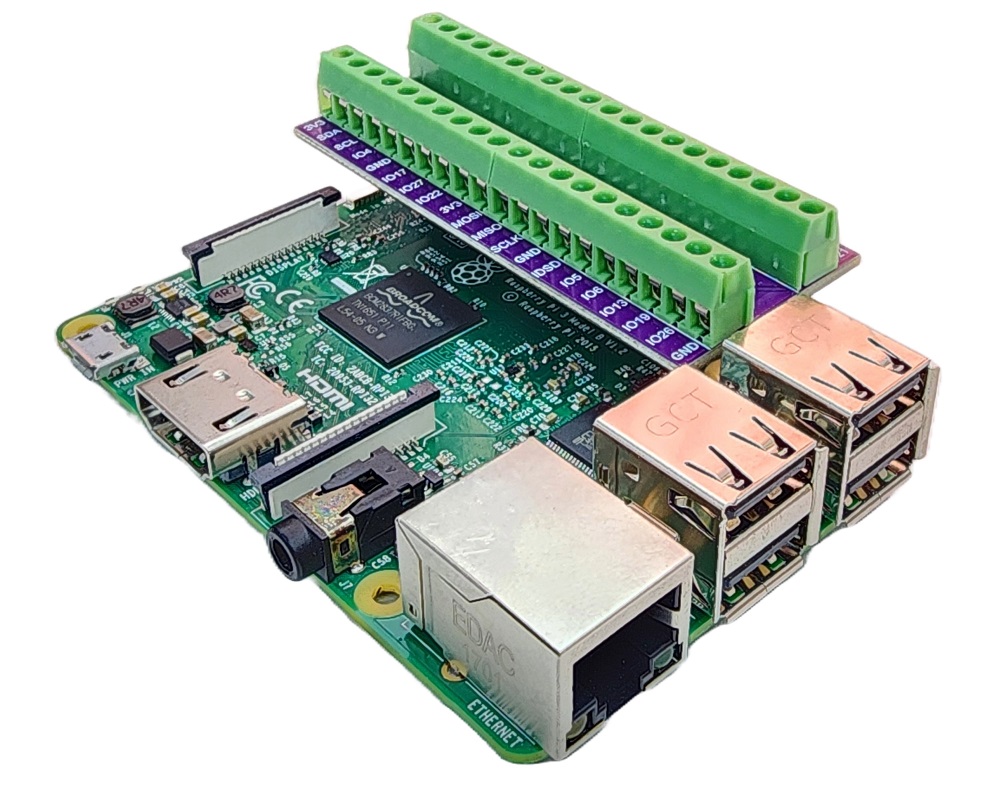
How To Program For Ultrasonic Sensor
- Create a 10-microsecond pulse on Raspberry Pi's pin 9 by utilizing the GPIO.output() and time.sleep() functions.
- For instance:
- Set pin 9 to HIGH with digitalWrite() and then delay for 10 microseconds with delayMicroseconds().
- Determine the pulse duration (µs) on Raspberry Pi's pin 8 by using the pulseIn() function. For example:
- Determine the distance (cm):
Raspberry Pi Code
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Create a Python script file ultrasonic_sensor.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Place your hand in front of the ultrasonic sensor
- Check out the distance between the sensor and your hand displayed on the Terminal
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!
How to Filter Noise from Distance Measurements of Ultrasonic Sensor
The measurement result from an ultrasonic sensor may contain noise which can lead to undesired operation in some applications. To remove this noise, the following algorithm can be used:
- Take multiple measurements and store them in an array
- Sort the array in ascending order
- Filter out the noise by:
- The smallest samples are considered as noise and should be ignored
- The biggest samples are considered as noise and should be ignored
- The average of the middle samples should be taken as measured value
- Ignore the five smallest samples, which are considered as noise.
- Ignore the five biggest samples, which are also considered as noise.
- Get the average of the 10 middle samples (from 5th to 14th).
The following example code takes 20 measurements:
Video Tutorial
Challenge Yourself
Utilize an ultrasonic sensor to complete one of the following projects:
- Construct a collision avoidance system for a remote-controlled car.
- Measure the amount of material in a dustbin.
- Monitor the level of the dustbin.
- Automatically open and close the dustbin. Hint: Refer to Raspberry Pi - Servo Motor.
Ultrasonic Sensor Applications
- Avoiding Collisions
- Detecting Fullness
- Measuring Level
- Detecting Proximity