Raspberry Pi - Touch Sensor
This tutorial instructs you how to use the capacitive touch sensor with Raspberry Pi. In detail, we will learn:
- How the touch sensor works
- How to connect the touch sensor to Raspberry Pi
- How to program Raspberry Pi to read the state from the touch sensor
- How to program Raspberry Pi to detect the touching/untouching event.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Touch Sensor
A capacitive touch sensor, also known as a touch button or touch switch, is frequently used to operate devices (e.g. a touchable lamp). It has the same purpose as a button. Many modern devices are equipped with it instead of a button, as it gives the product a more polished look.
The Touch Sensor Pinout
The touch sensor has three pins:
- GND pin: This must be connected to the ground (0V).
- VCC pin: This must be connected to the VCC (5V or 3.3v).
- SIGNAL pin: This is an output pin. It will be LOW when not touched and HIGH when touched. This pin needs to be connected to a Raspberry Pi's input pin.
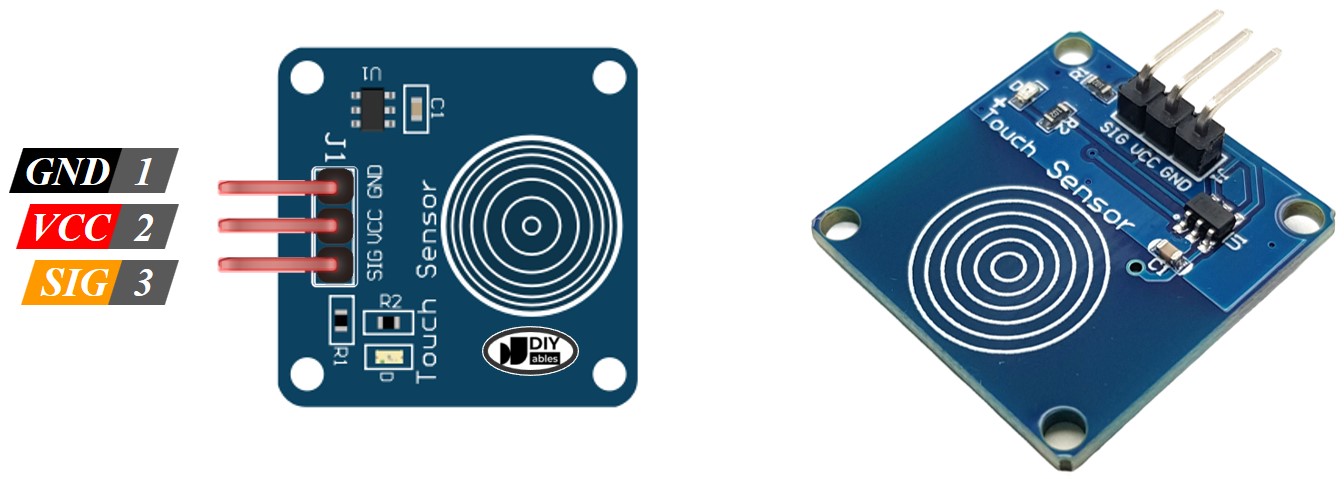
How It Works
- When the sensor is not being touched, the SIGNAL pin of the sensor will be at a LOW level.
- However, when the sensor is touched, the SIGNAL pin of the sensor will be at a HIGH level.
Raspberry Pi - Touch Sensor
The SIGNAL pin of the touch sensor is linked to an input pin of a Raspberry Pi.
By examining the status of a Raspberry Pi pin (set up as an input pin), we can detect if the touch sensor has been activated or not.
Wiring Diagram
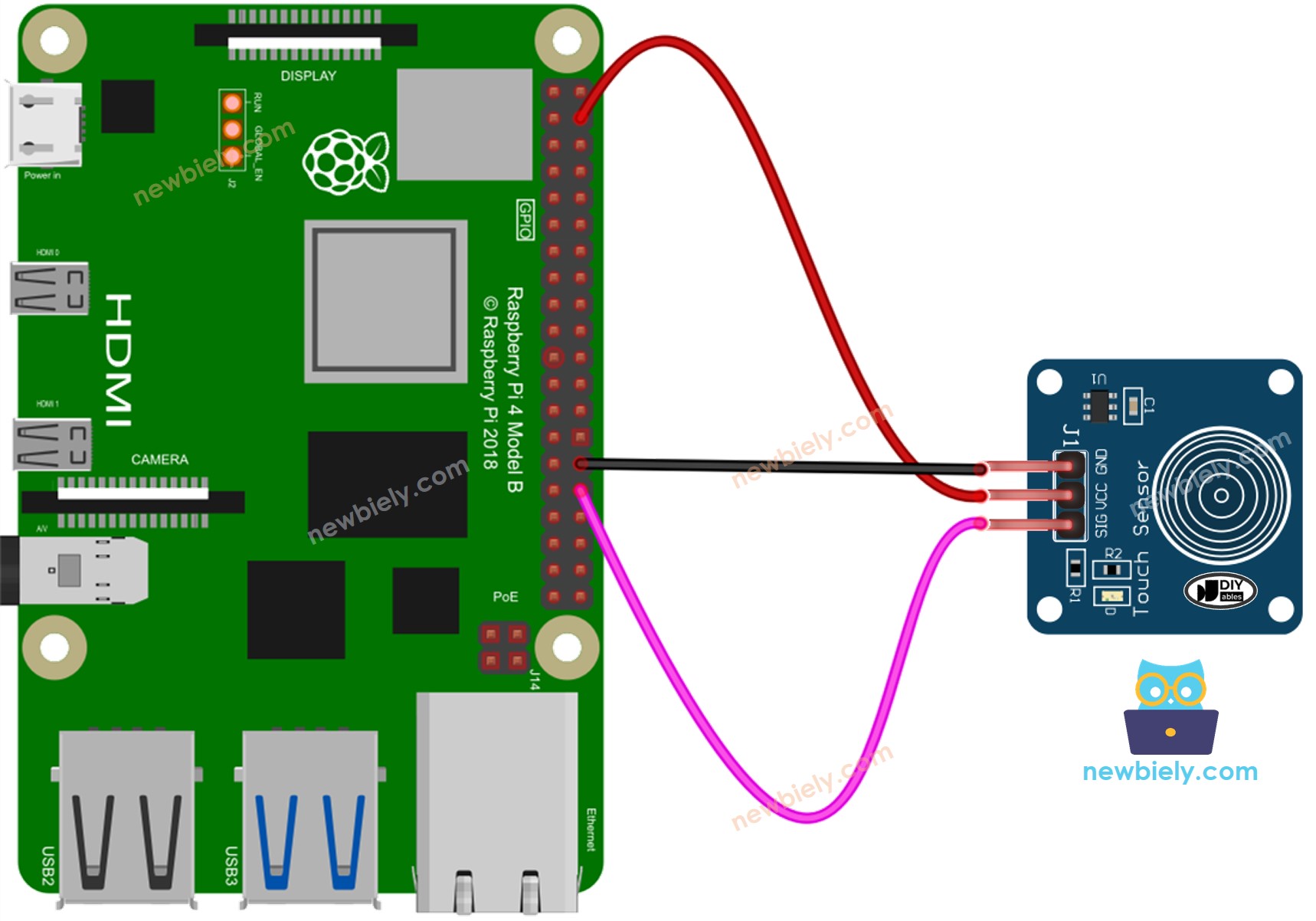
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
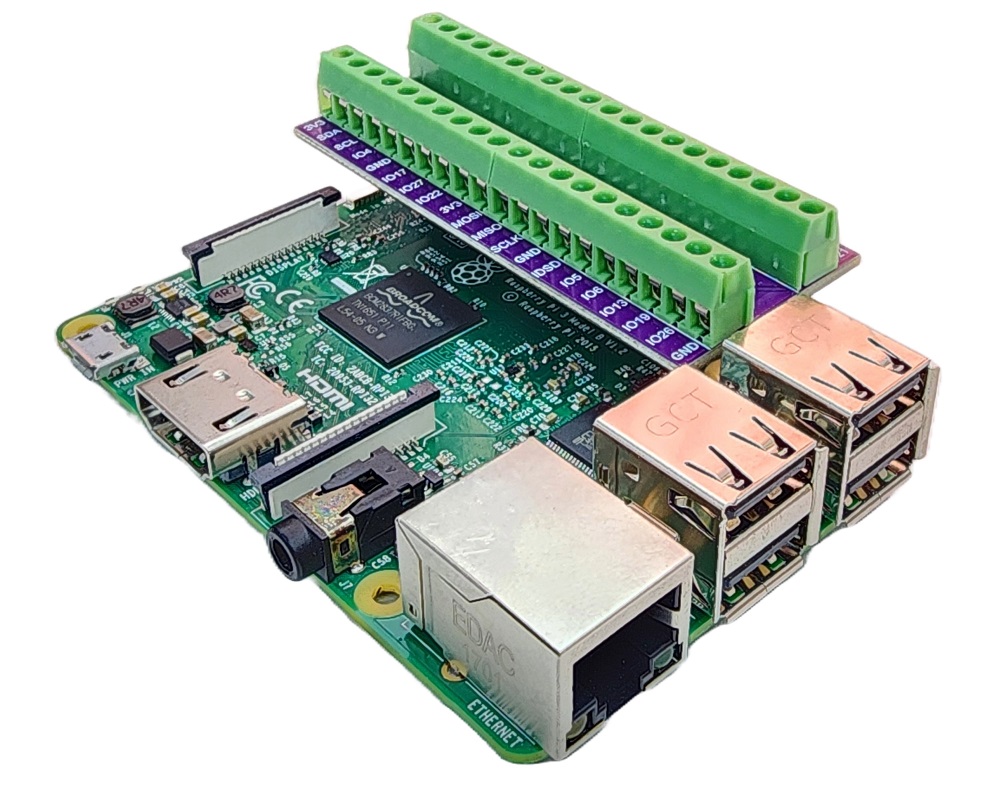
How To Program For Touch Sensor
- Sets up the Raspberry Pi pin to digital input mode with the GPIO.setup() function.
- Utilizes the GPIO.input() function to ascertain the status of the Raspberry Pi pin.
There are two common use cases for the touch sensor:
- The first: If the input state is HIGH, perform one action. If the input state is LOW, take the opposite action.
- The second: If the input state changes from LOW to HIGH (or HIGH to LOW), do an action.
Depending on the application, one of these is selected. For example, when using a touch sensor to control an LED:
- If the goal is to have the LED be ON when the sensor is touched and OFF when the sensor is NOT touched, the first use case should be used.
- If the aim is to have the LED toggle between ON and OFF each time the sensor is touched, the second use case should be used.
Raspberry Pi Code for Touch Sensor
We will learn two sample codes:
- Raspberry Pi reads the value from the touch sensor and prints it on the Terminal.
- Raspberry Pi checks if the sensor is touched or released.
Raspberry Pi reads the value from the touch sensor and prints to the Terminal
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Create a Python script file touch_sensor.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Put your finger on the sensor and then take it away.
- Check the outcome in the Terminal.
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
Raspberry Pi detects the sensor touched or released
Detailed Instructions
- Create a Python script file TO_BE_UPDATED.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Touch and keep your finger on the sensor.
- Check the output in the Terminal.
- Take your finger off the sensor.
- Check the output on the Terminal.