Raspberry Pi - Stepper Motor Limit Switch
This tutorial instructs you how to use Raspberry Pi to control a stepper motor via a limit switch and an L298N driver. Specifically, we will cover:
- How to program Raspberry Pi to stop the stepper motor when a limit switch is touched.
- How to program Raspberry Pi to change direction of the stepper motor when a limit switch is touched.
- How to program Raspberry Pi to change direction of the stepper motor by two limit switches installed in opposite position.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Stepper Motor and Limit Switch
If you are unfamiliar with stepper motor and limit switch (including pinout, functionality, programming, etc.), the following tutorials can help:
- Raspberry Pi - Limit Switch tutorial
- Raspberry Pi - Stepper Motor tutorial
Wiring Diagram
This tutorial provides the wiring diagram for two cases: One stepper motor + one limit switch, One stepper motor + two limit switches.
- Wiring Diagram between Raspberry Pi, stepper motor and, and a limit switch
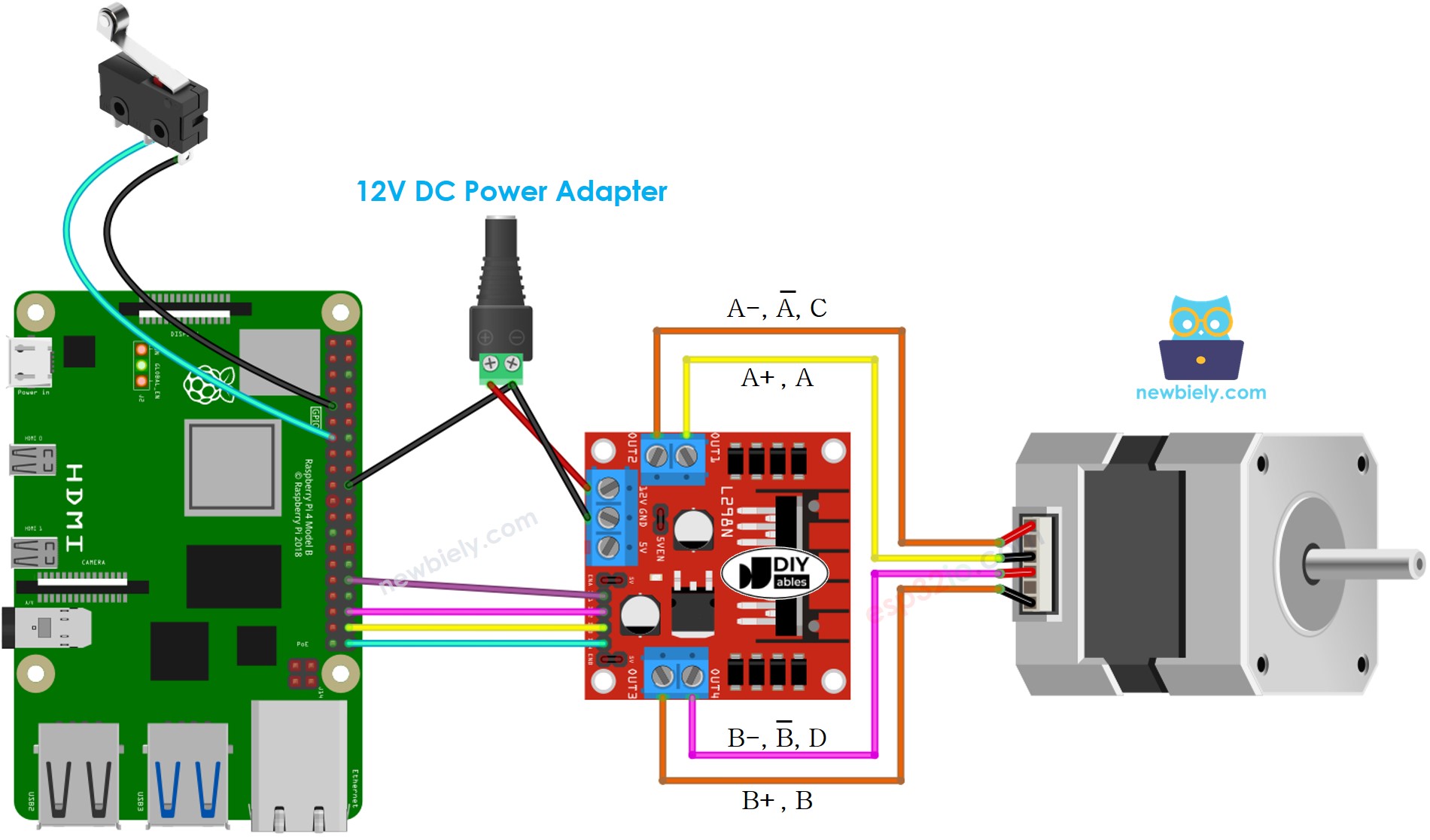
This image is created using Fritzing. Click to enlarge image
- Wiring Diagram between Raspberry Pi, stepper motor and, and two limit switches
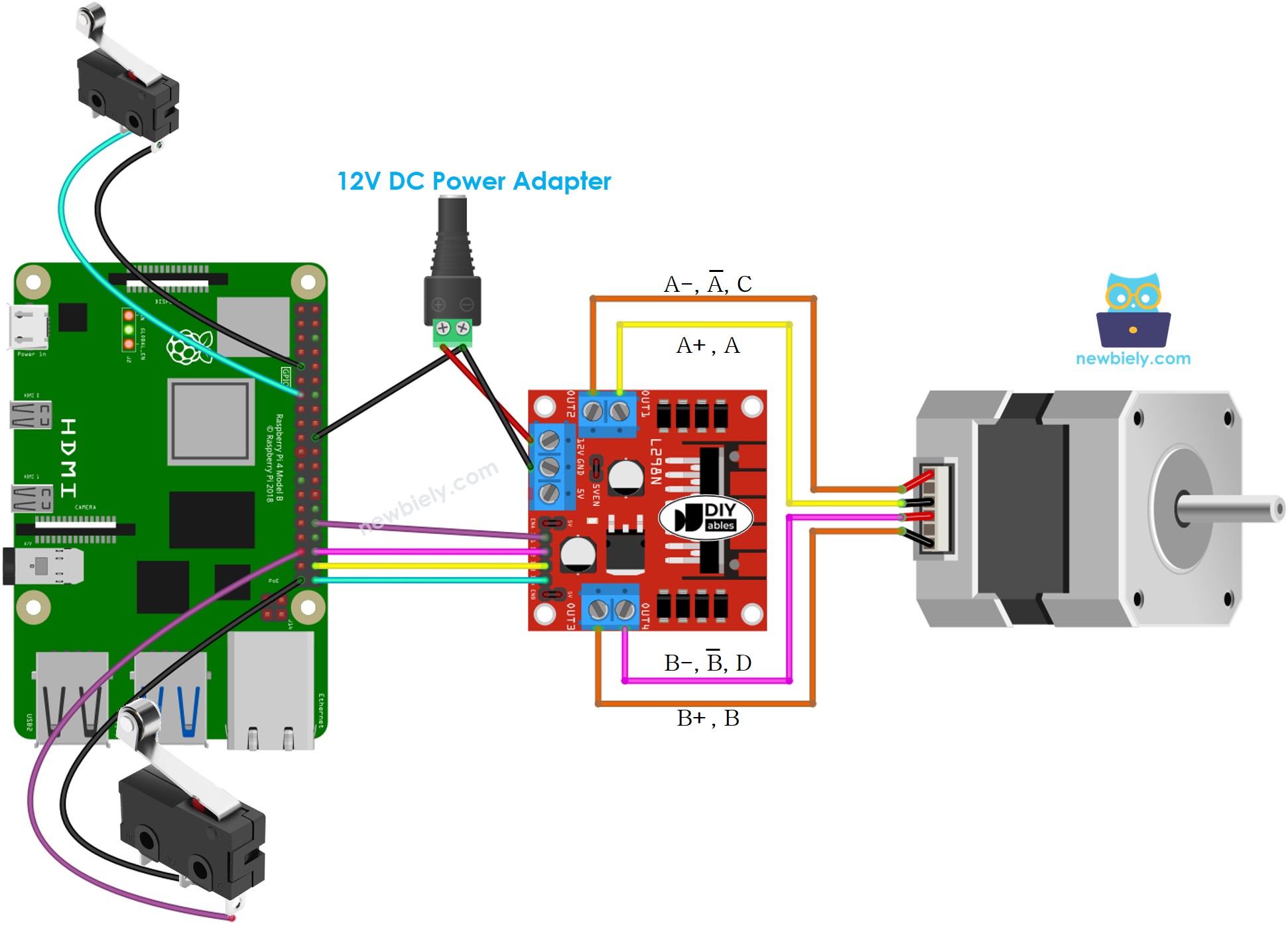
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
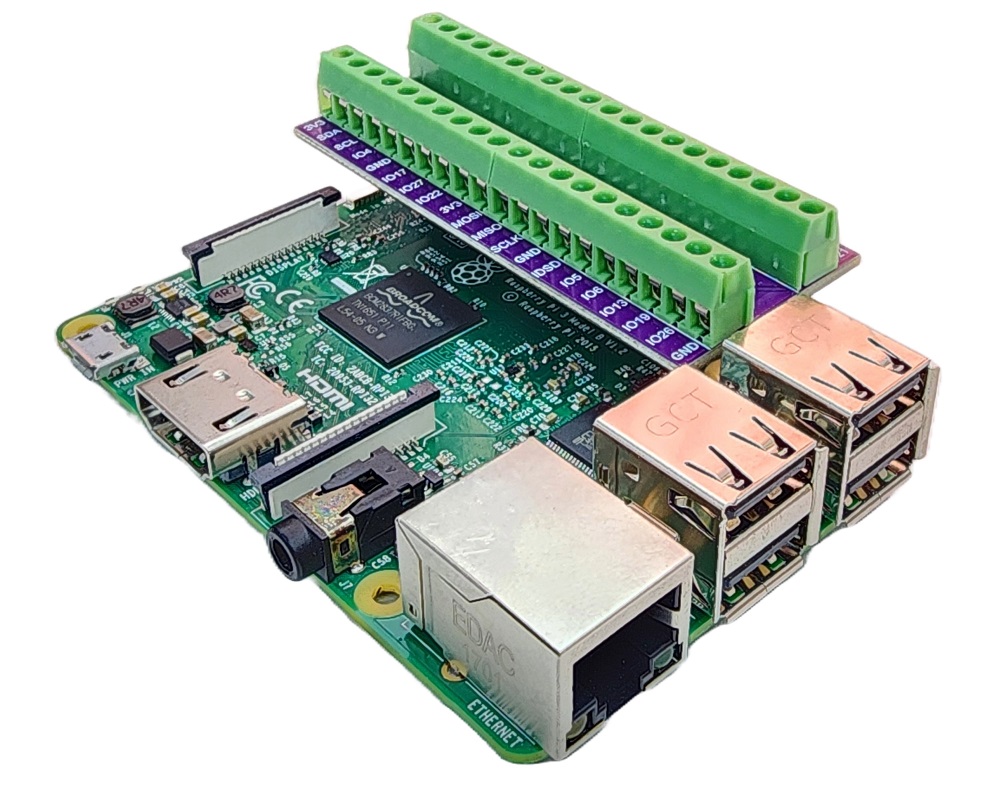
※ NOTE THAT:
The wiring connection between the stepper motor and L298N may vary depending on the type of stepper motor. Carefully examine the Raspberry Pi - Stepper Motor tutorial to learn how to properly link the stepper motor to the L298N motor driver.
Raspberry Pi Code - Stop Stepper Motor by a Limit Switch
A stepper motor is programmed to spin continuously with the following code, and will stop instantly when a limit switch is touched, and resume moving if the limit switch is released.
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Create a Python script file stepper_limit_switch.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- If the wiring is correct, the motor should rotate in a clockwise direction.
- When the limit switch is touched, the motor should stop immediately.
- Then if the limit switch is released, the motor rotates again.
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
By changing the value of delay variable in the code, you can change the speed of the stepper motor.
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!
Raspberry Pi Code - Change Direction of Stepper Motor by a Limit Switch
A stepper motor will be set in motion continuously and its direction will be altered when a limit switch is touched.
Detailed Instructions
- Create a Python script file stepper_direction.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- If the wiring is correct, the motor should spin in a clockwise direction.
- When you touch the limit switch, the stepper motor's direction will change to counterclockwise.
- Touch the limit switch again and the stepper motor's direction will go back to clockwise.
Raspberry Pi Code - Change Direction of Stepper Motor by two Limit Switches
Let's see the code that makes a stepper motor spin continuously, and when either of two limit switches is touched, switches the motor's direction.
Detailed Instructions
- Create a Python script file stepper_two_limit_switches.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
- If the wiring is correct, the motor should spin in a clockwise direction.
- When you touch limit switch 1, the stepper motor's direction will be reversed to anti-clockwise.
- Touching limit switch 2 will cause the stepper motor to rotate clockwise again.