Raspberry Pi - Keypad - Servo Motor
This tutorial instructs you how to use Raspberry Pi and keypad to control servo motor. In detail:
- When an authorized password is entered on the keypad, Raspberry Pi will rotate the servo motor to 90°.
- After a set amount of time, the Raspberry Pi will rotate the servo motor back to 0°.
The code for Raspberry Pi also allows for multiple passwords to be used.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Keypad and Servo Motor
If you are unfamiliar with keypad and servo motor (including pinout, functionality, programming, etc.), the following tutorials can help:
- Raspberry Pi - Keypad tutorial
- Raspberry Pi - Servo Motor tutorial
Wiring Diagram
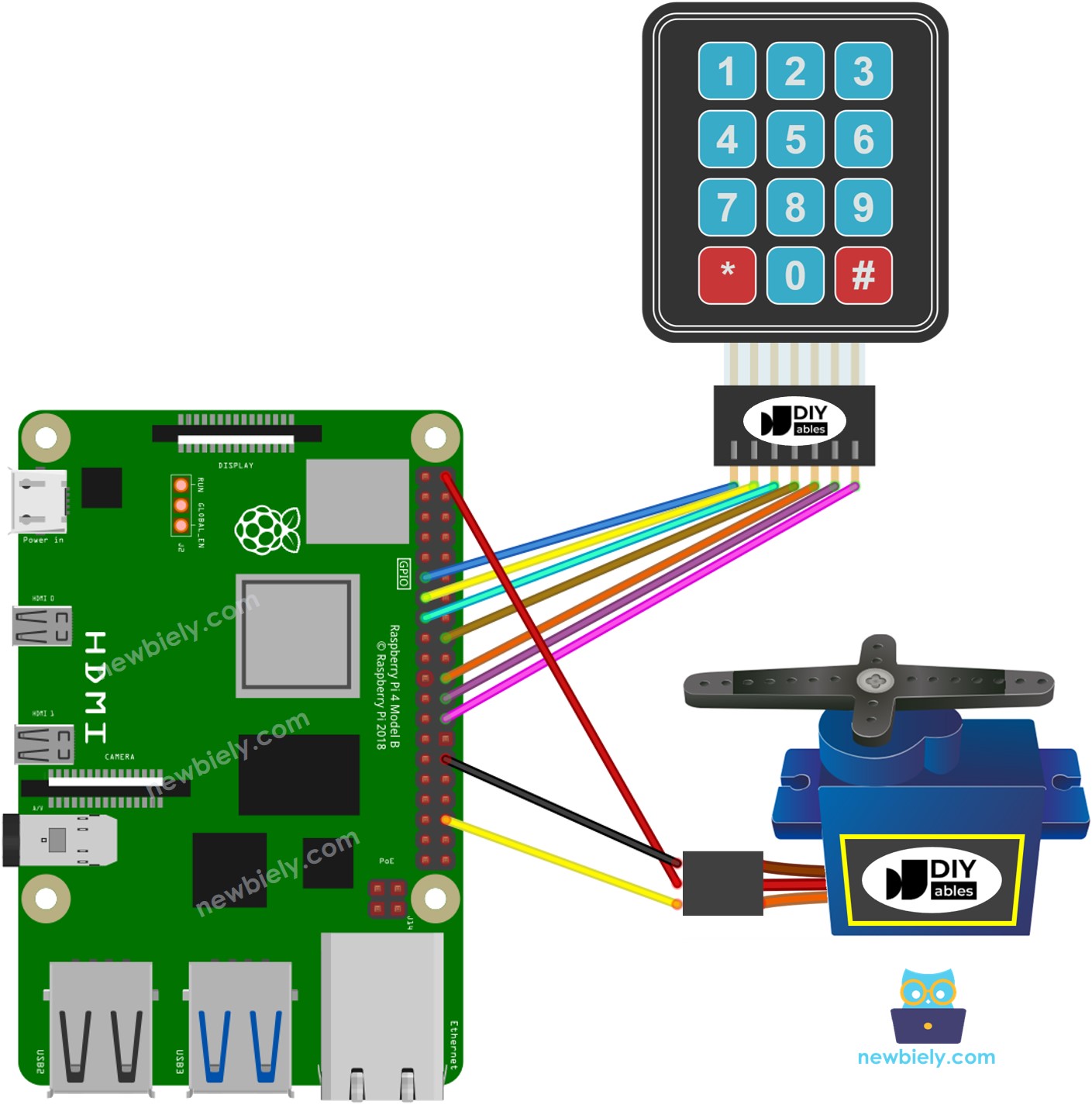
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
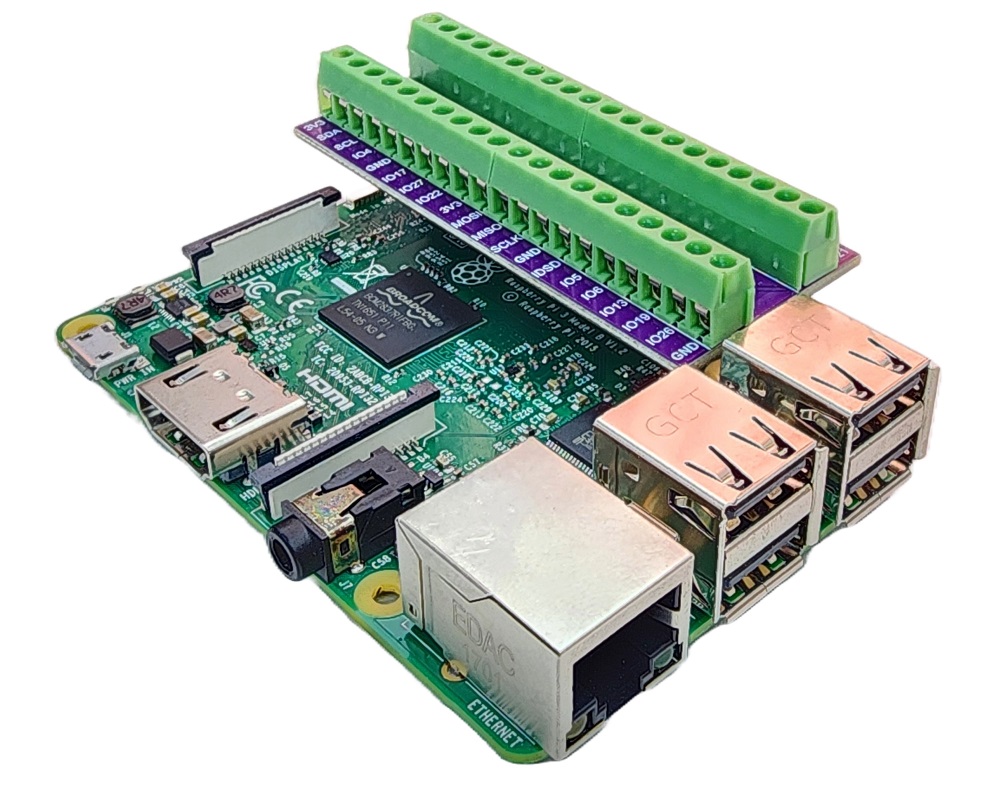
Please note that the wiring diagram shown above is only suitable for a servo motor with low torque. In case the motor vibrates instead of rotating, an external power source must be utilized to provide more power for the servo motor. The below demonstrates the wiring diagram with an external power source for servo motor.
TO BE ADD IMAGE
Please do not forget to connect GND of the external power to GND of Arduino Raspberry Pi.
Raspberry Pi Code - rotates Servo Motor if the password is correct
If the password is correct, the servo motor will be set to 90° for a duration of 5 seconds. Once the 5 seconds have elapsed, the servo motor will be reset to 0°.
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Create a Python script file keypad_servo.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
- Press 12345#
- Enter 5642B#
- Check out the outcome on Serial Monitor and the position of the servo motor.
Code Explanation
The valid passwords are pre-defined in the Raspberry Pi code. A string, referred to as input_password, is used to store the password inputted by users. On the keypad, two keys (* and #) are used for special purposes: clearing the password and terminating the password. When a key on the keypad is pressed:
- If the pressed key is not one of the two special keys, it will be added to the input_password.
- If the pressed key is *, the input_password will be cleared. This can be used to start or re-start inputting the password.
- If the pressed key is #:
- The Raspberry Pi checks if the input_password matche with one of the pre-defined passwords, the servo motor will rotate to 90°.
- Regardless of whether the password is correct or not, the input_password will be cleared for the next input.
- After a period of time, ESP8266 rotates the servo motor to 0°.