Raspberry Pi Control Servo Motor via Bluetooth
This tutorial instructs you how to program a Raspberry Pi to manage a Servo Motor by utilizing either Bluetooth (HC-05 module) or BLE (HM-10 module). Step-by-step instructions for both modules are given.
We will use the Bluetooth Serial Monitor App on a smartphone to transmit the angle value to Raspberry Pi. Raspberry Pi will adjust the servo motor based on the received value.
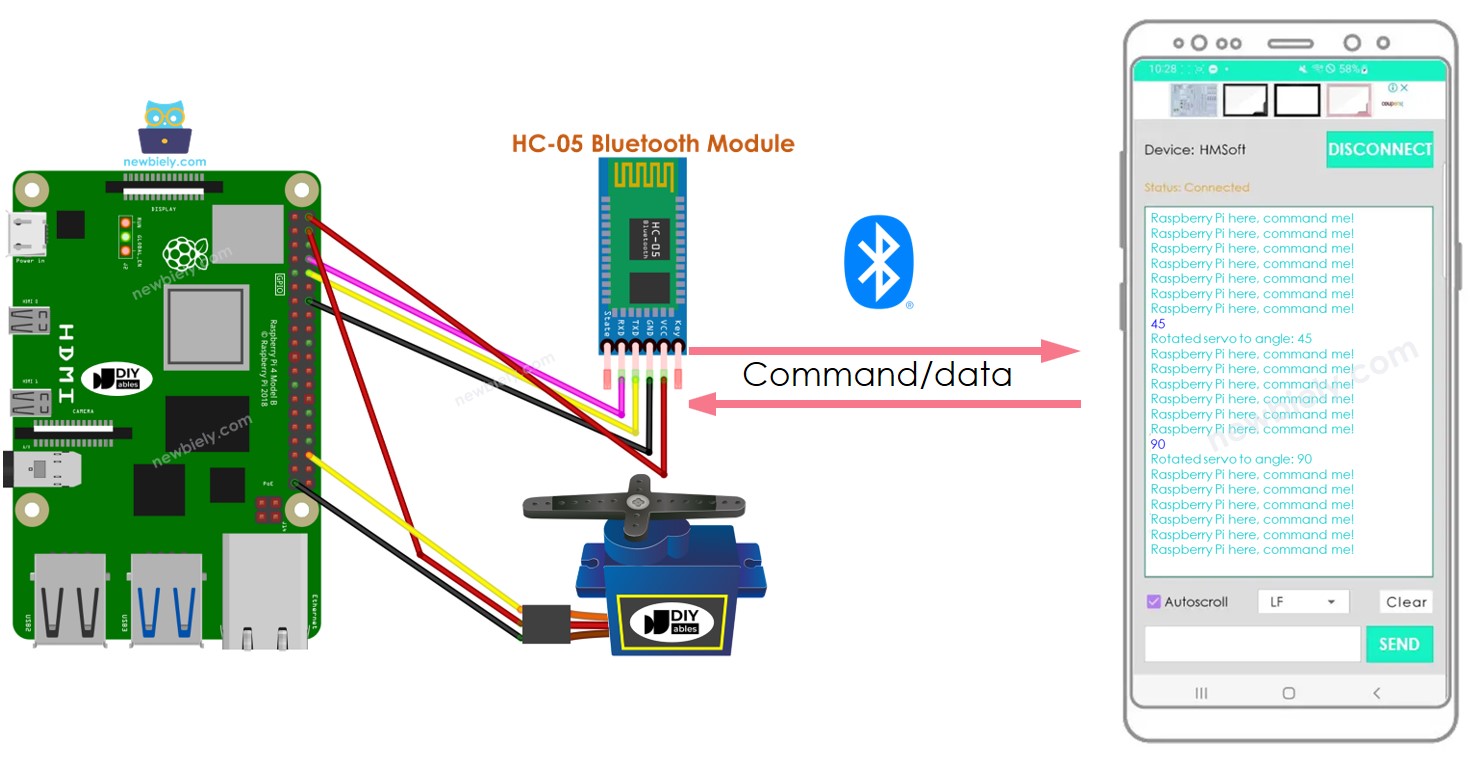
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Overview of Servo Motor and Bluetooth Module
If you are not familiar with Servo Motors, Bluetooth Modules, their pinouts, capabilities, and programming, please refer to the following tutorials for more information:
Wiring Diagram
- To manage a Servo Motor with Classic Bluetooth, the HC-05 Bluetooth module should be used and the wiring diagram provided should be consulted.
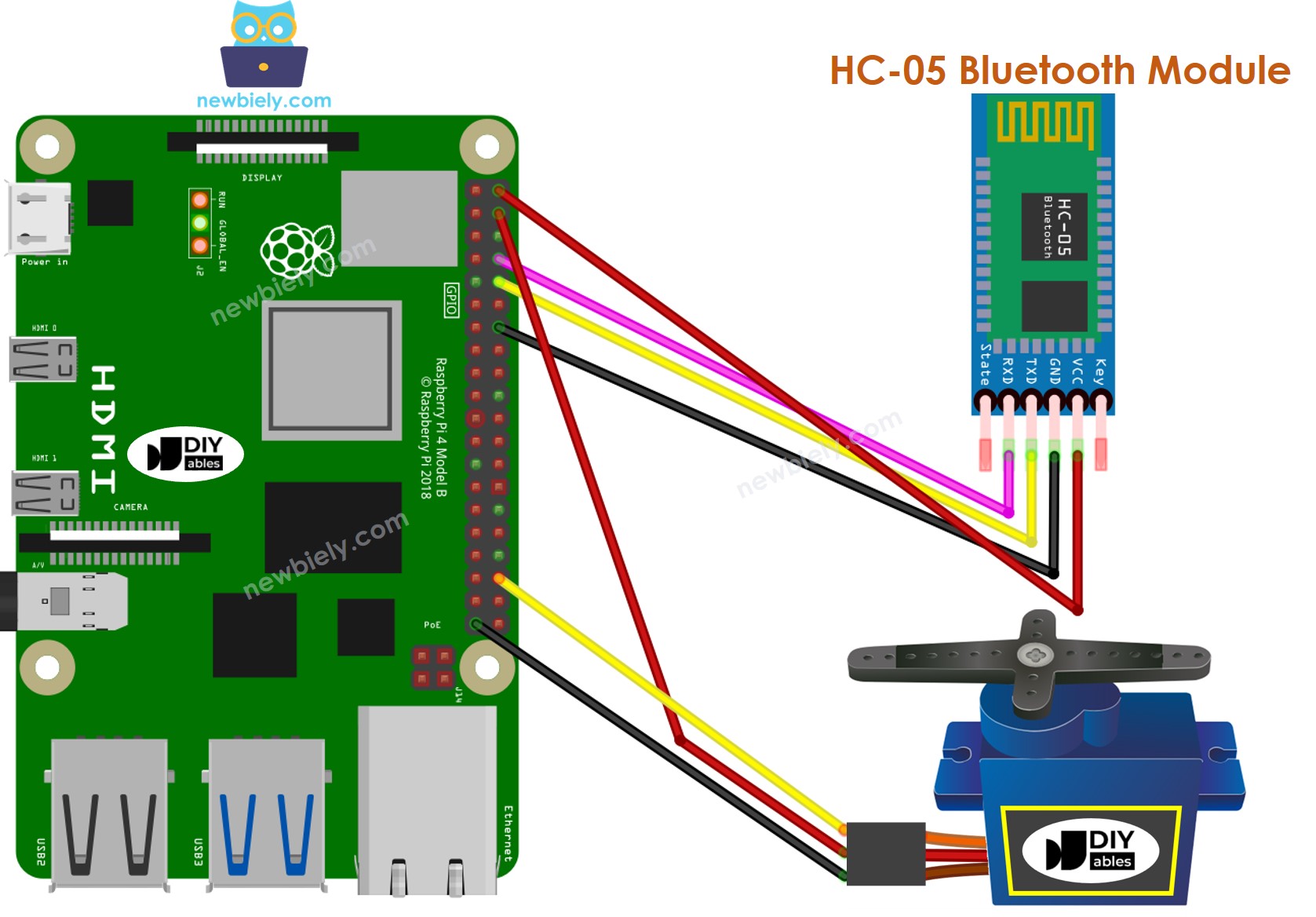
This image is created using Fritzing. Click to enlarge image
- To manage a Servo Motor with BLE, the HM-10 BLE module should be used. The wiring diagram is given below for reference.
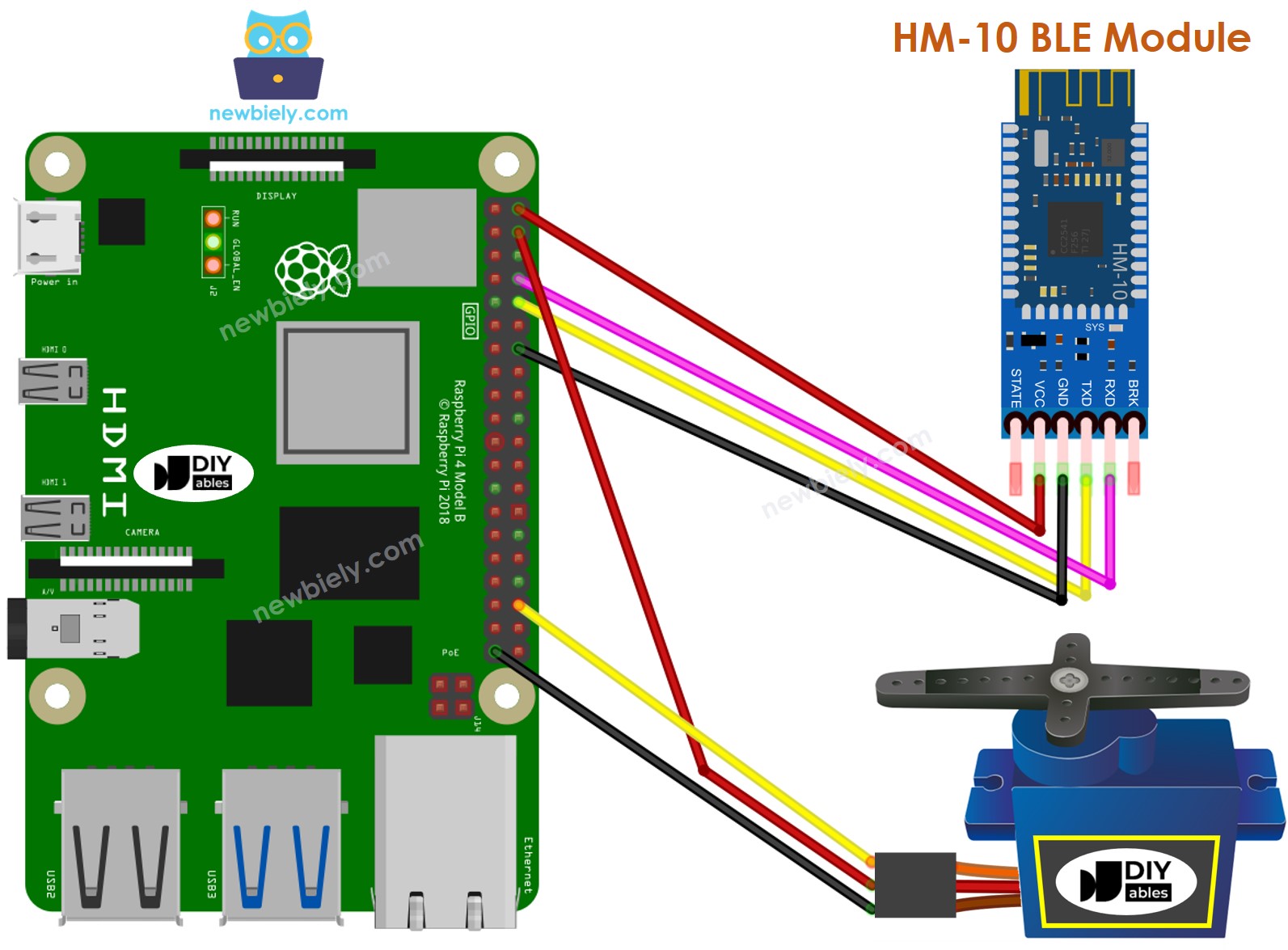
This image is created using Fritzing. Click to enlarge image
Raspberry Pi Code - controls Servo Motor via Bluetooth/BLE
The code given here is able to be used with both the HC-10 Bluetooth module and the HM-10 BLE module.
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Enable the Serial interface on Raspberry Pi by following the instruction on Raspberry Pi - how to enable Serial inteface
- Install the pyserial library for communication with bluetooth module:
- Create a Python script file bluetooth_servo.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
- Install the Bluetooth Serial Monitor App on your smartphone.
- Open the Bluetooth Serial Monitor App on your smartphone and choose either the Classic Bluetooth or BLE option, depending on the module you are using.
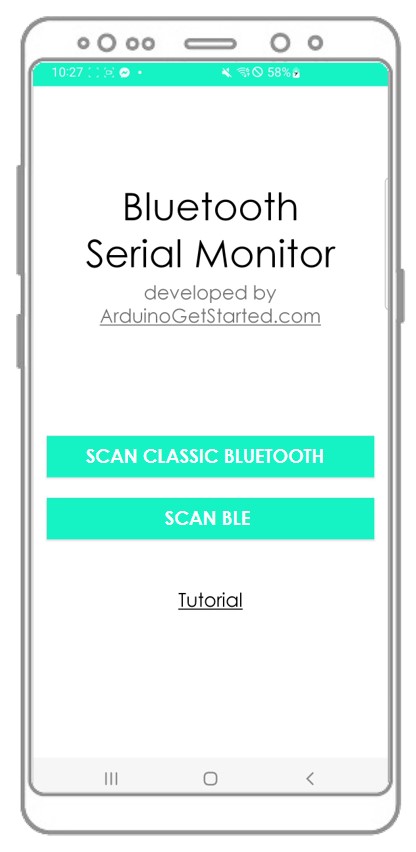
- Connect the app to the HC-05 Bluetooth module or HM-10 BLE module.
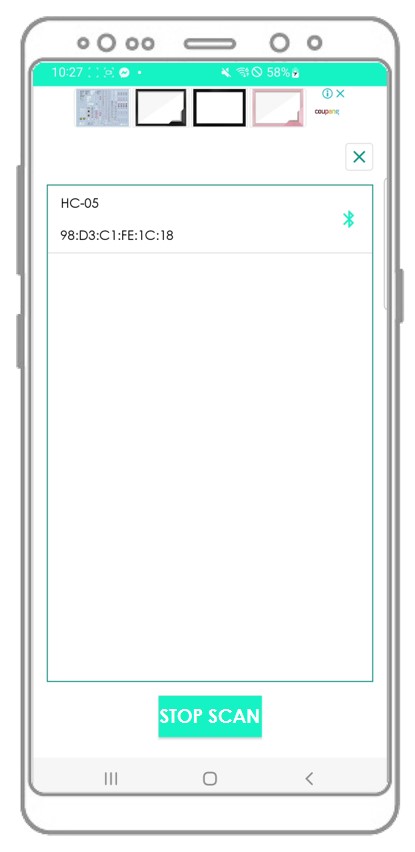
- Enter an angle, such as 45 or 90, and then press the Send button.
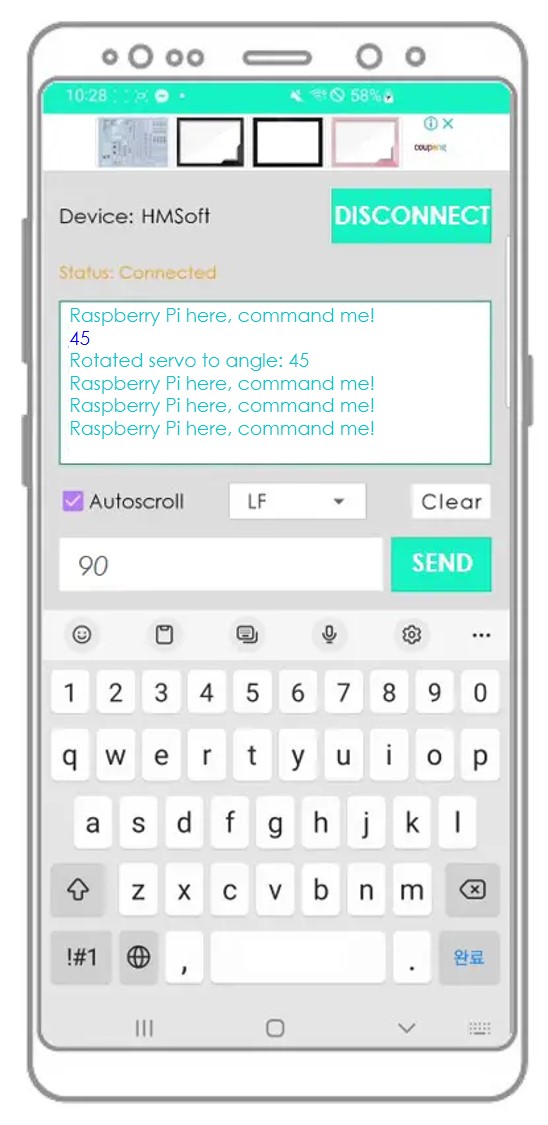
- Witness the Servo Motor's angle alteration.
- Examine the outcomes on the Android App.
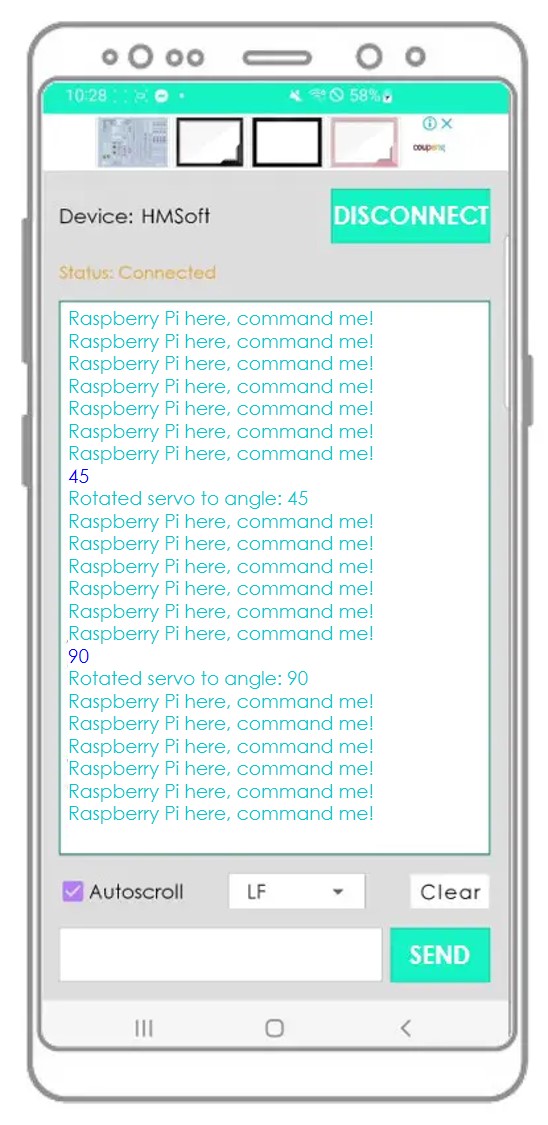
If you find the Bluetooth Serial Monitor app helpful, please rate it 5 stars on Play Store. Thank you for your support!