Raspberry Pi - Light Sensor
This tutorial instructs you how to use the light sensor with Raspberry Pi. In detail:
- How a light sensor works
- How to connect the light sensor to a Raspberry Pi
- How to program Raspberry Pi to read the value from the light sensor
If you're looking for a light sensor that outputs two level (LOW/HIGH) based on a settable threshold, We highly recommend checking out the LDR Light Sensor Module tutorial. It is much easier and more convenient to use.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
The LDR light sensor is very affordable, but it requires a resistor for wiring, which can make the setup more complex. To simplify the wiring, you can use an LDR light sensor module as an alternative.
Overview of Light Sensor
This tutorial utilizes a light sensor known as a photoresistor. It is also referred to as a light-dependent resistor, LDR, or photocell.
It is not only used to detect light, but also to measure the brightness/illuminance level of the environment.
The Light Sensor Pinout
A photoresistor has two pins which do not need to be distinguished, as it is a type of resistor that is symmetric.
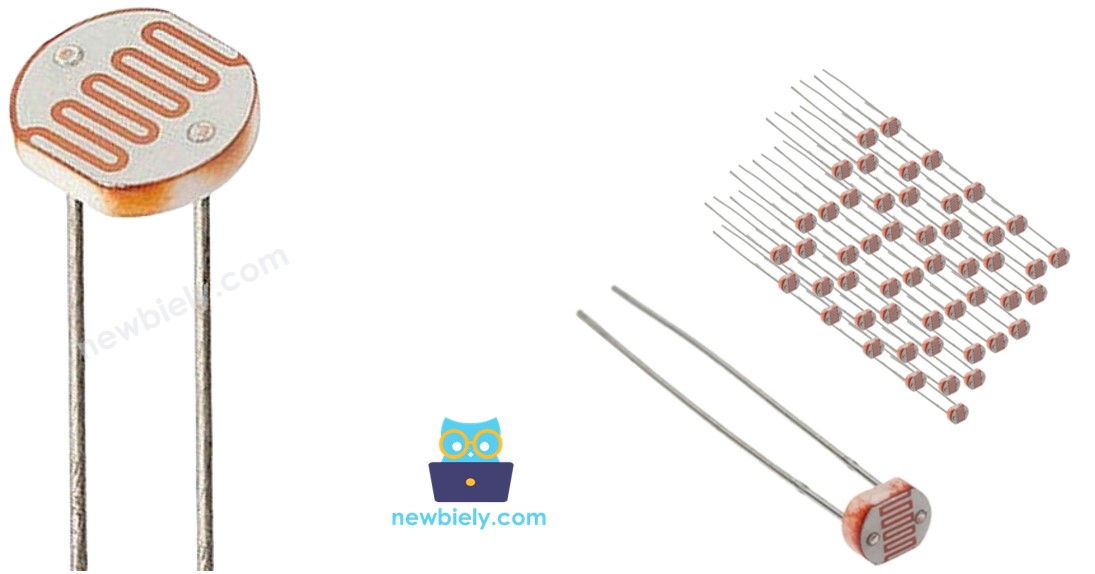
How It Works
The greater the amount of light that the photoresistor's face is exposed to, the lower its resistance will be. Thus, by measuring the photoresistor's resistance, we can determine the brightness of the surrounding illumination.
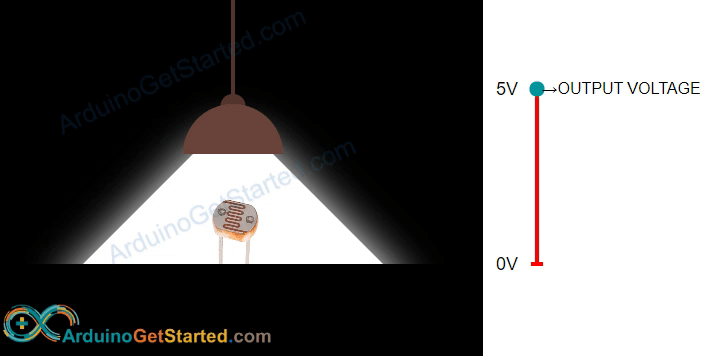
WARNING
The value obtained from the light sensor only gives an approximate indication of the intensity of light, not a precise measure of luminous flux. Therefore, it should not be used in situations where high accuracy is required.
Raspberry Pi - Light Sensor
The Raspberry Pi board does not come with a built-in ADC, so we will utilize an external ADC module, such as the ADS1115, to read analog voltage from a light sensor. Follow these steps to set up the system:
- Connect the light sensor to the ADS1115 module's analog input.
- The ADS1115 module will perform analog-to-digital conversion of the voltage from the light sensor, providing an ADC value.
- Establish a connection between the Raspberry Pi and the ADS1115 module using the I2C interface.
- Make sure I2C is enabled on the Raspberry Pi and set up the necessary configurations.
- Use suitable libraries or code to read the ADC value from the ADS1115 module via the I2C interface on the Raspberry Pi.
By following these steps, you can successfully read analog voltage from the light sensor by utilizing the ADS1115 module with your Raspberry Pi.
Wiring Diagram
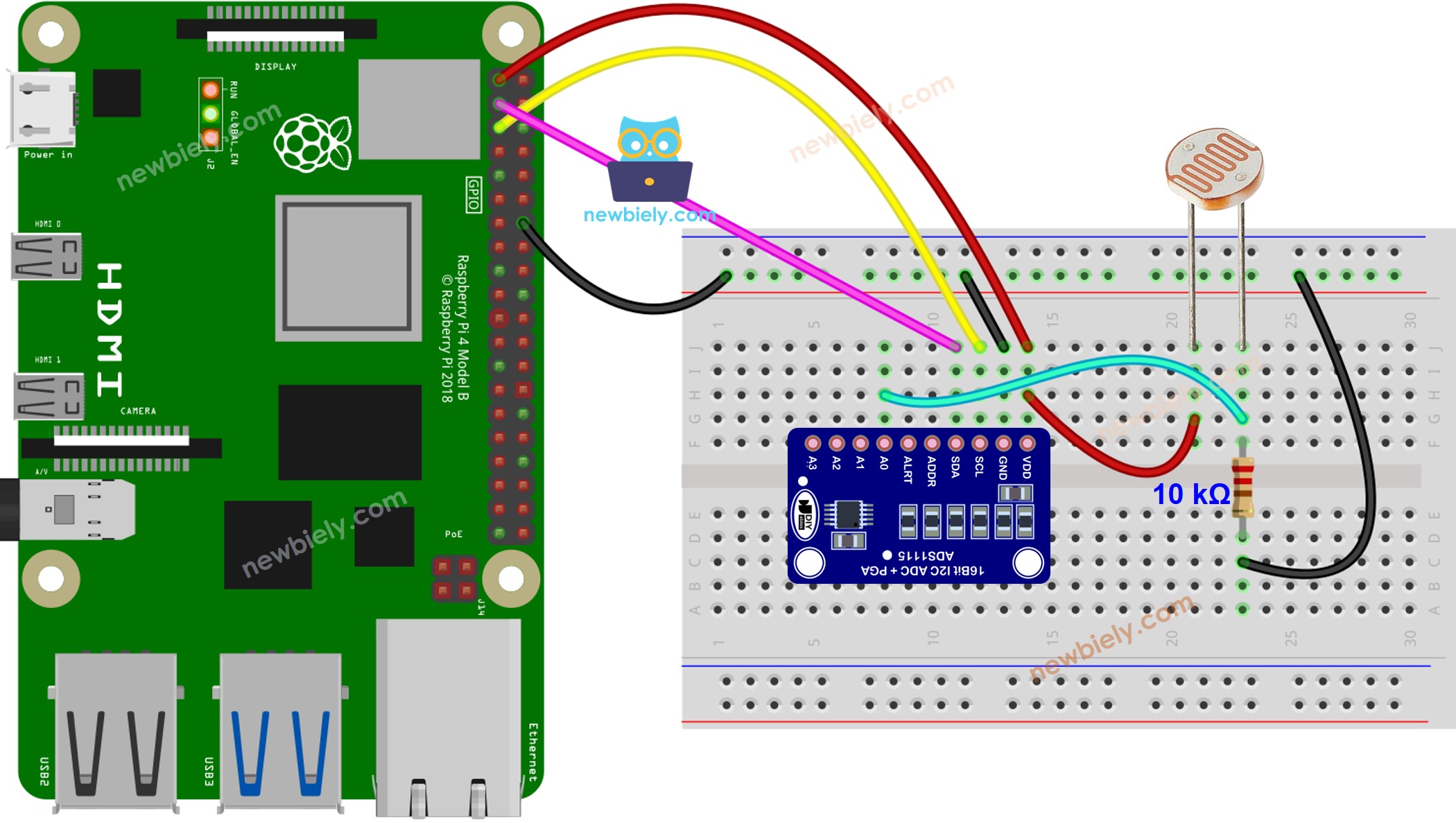
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
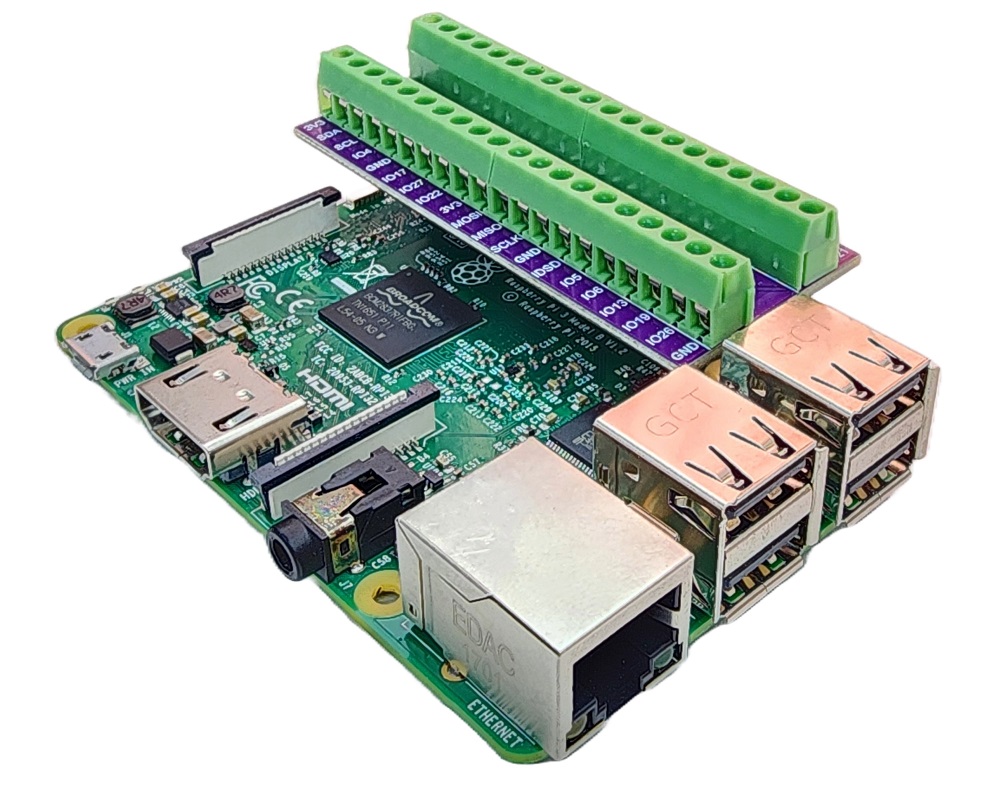
Raspberry Pi Code
This code reads the value from a photocell and evaluates the light level qualitatively.
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Install the Adafruit_ADS1x15 library by running the following commands on your Raspberry Pi terminal:
- Create a Python script file light_sensor.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Cover light sensor by your hand or shine light onto the sensor.
- Check the Terminal to view the result.
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.