Raspberry Pi - Button - Long Press Short Press
This tutorial instructs you how to use Raspberry Pi to delect the button's long press and short press. To make it simple, we will break it down into the following examples:
- The Raspberry Pi detects the button's short press.
- The Raspberry Pi detects the button's long press.
- The Raspberry Pi detects the both button's short press and long press.
- The Raspberry Pi debounces both long press and short press.
In the final section, we will explore how to utilize debounce in a practical setting. For further information on why debouncing is necessary for buttons, please refer to this article. Without debouncing, it is possible to incorrectly detect a short press of the button.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Wiring Diagram
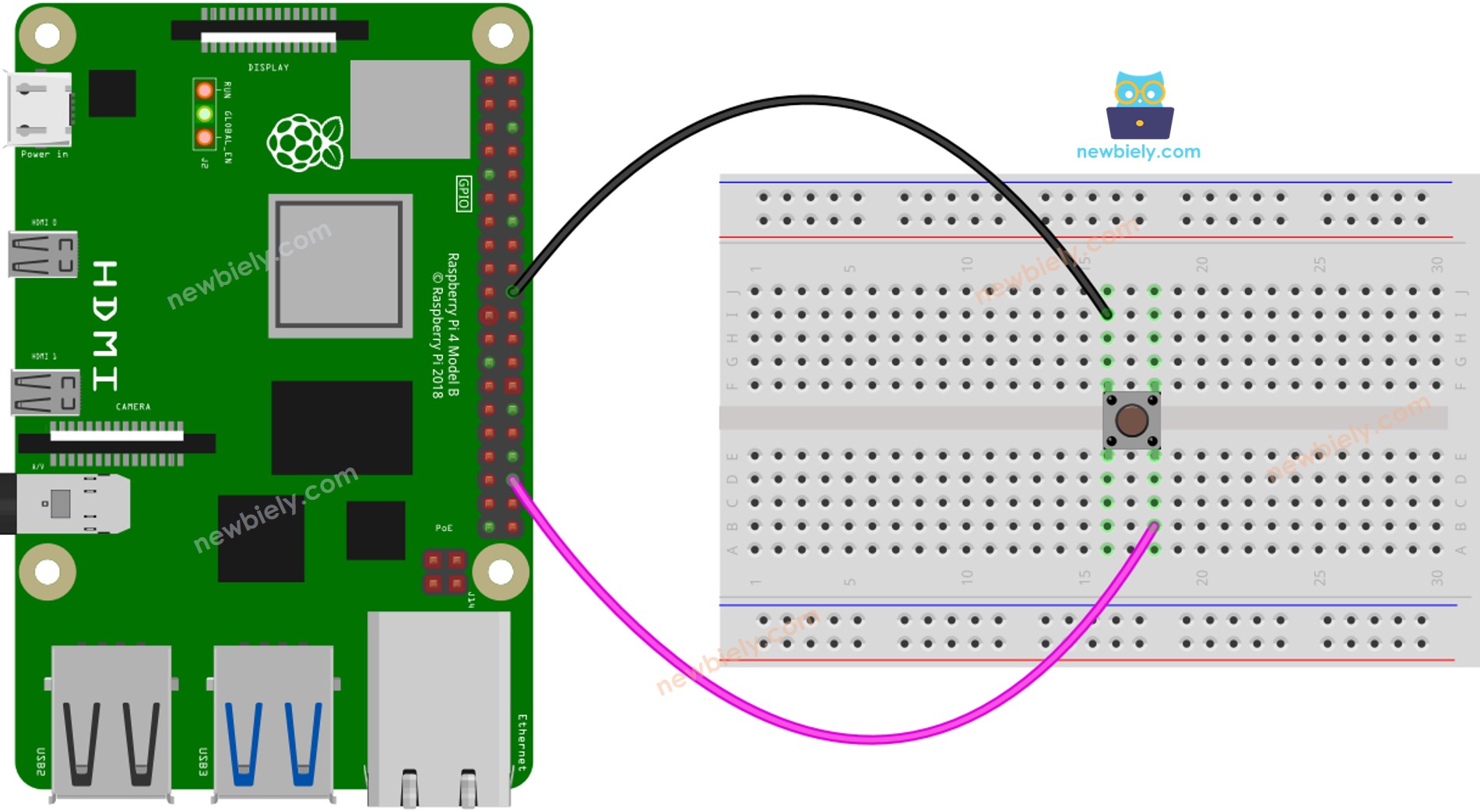
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
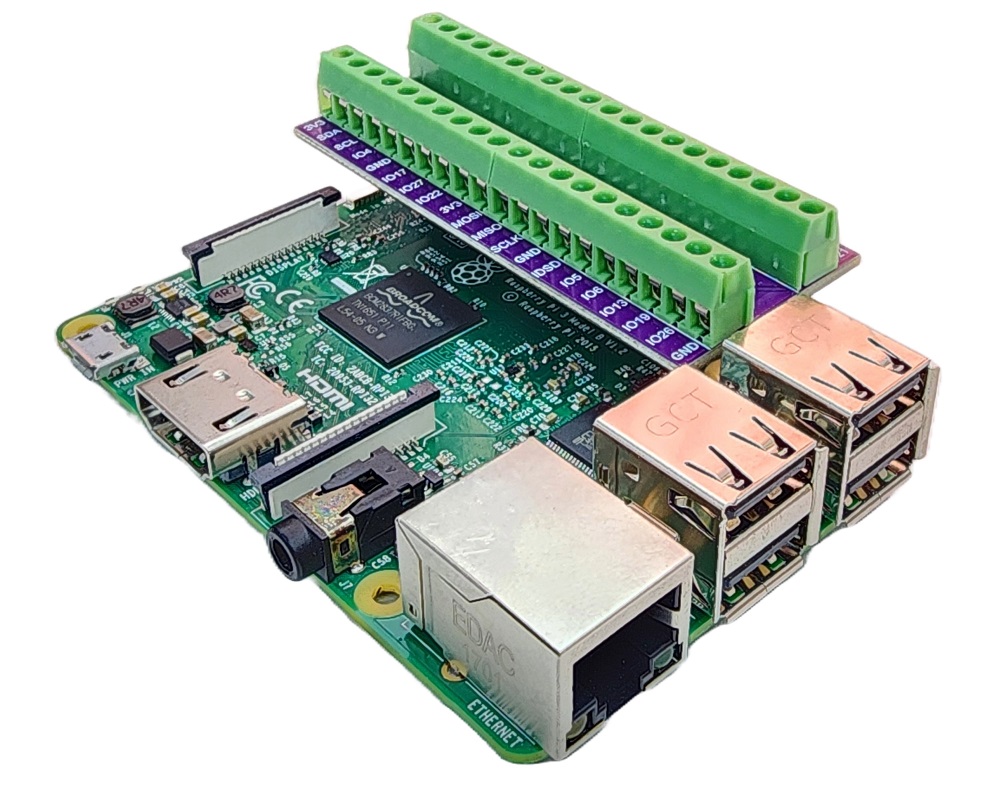
In this tutorial, we will make use of the internal pull-up resistor. As a result, when the button is not pressed, its state is HIGH, and when it is pressed, its state is LOW.
How To Detect Short Press
We calculate the amount of time between the pressed and released events. If the period is less than a predetermined time, we detect a short press event.
Specify the duration of a short press.
- Detect if the button has been pressed and record the time of the press.
- Determine when the button has been released and record the time of release.
- Determine the press duration.
- Compare the press duration to the defined short press time to identify a short press.
Raspberry Pi Code for detecting the short press
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Create a Python script file short_press.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Press the button briefly multiple times.
- Check out the outcome in the Terminal.
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
How To Detect Long Press
There are two scenarios for recognizing a long press:
- The long-press event is identified immediately after the button is released
- The long-press event is identified while the button is being held down, even before it is released.
In the first scenario, the duration between the pressed and released events is calculated. If this duration exceeds a predetermined amount of time, then a long-press event is identified.
In the second scenario, when the button is pressed, the pressing duration is continuously checked until the button is released. As the button is held down, if the duration exceeds a predetermined amount of time, the long-press event is detected.
Raspberry Pi Code for detecting long press when released
Detailed Instructions
- Create a Python script file long_press_1.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Press the button during 2 seconds and then release the button.
- Check the result on the Terminal.
The event of long-pressing is only identified when the button is released.
Raspberry Pi Code for detecting long press during pressing
Detailed Instructions
- Create a Python script file long_press_2.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Press the button and hold it for a few seconds then release the button.
- Check out the result on the Serial Monitor.
The event of long-pressing is only detected even when the button has not been released.
How To Detect Both Long Press and Short Press
Detailed Instructions
- Create a Python script file long_short_press.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Press the button, both for a short and long duration.
- Check out the results on the Terminal.
Video Tutorial
Why Needs Long Press and Short Press
- To minimize the number of buttons, one button can be used to perform multiple functions. For instance, a short press can be used to switch the operation mode, while a long press can be used to turn off the device.
- Using a long press helps to prevent accidental short presses. For example, some devices use a button to initiate a factory reset. If the button is pressed unintentionally, it could be hazardous. To avoid this, the device is designed to only initiate a factory reset when the button is held down for a certain amount of time (e.g. 5 seconds).