Raspberry Pi - LED - Blink Without Delay
Let us envision that Raspberry Pi is required to accomplish two tasks: blinking an LED and monitoring the state of a button which can be pressed at any time. If we utilize the time.sleep() function (as discussed in a previous tutorial), Raspberry Pi may not detect some of the button presses. In other words, Raspberry Pi is unable to completely carry out the second task.
This tutorial instructs you how Raspberry Pi can blink an LED and monitor the state of a button without missing any pressing events.
We will go over three examples and compare the distinctions between them:
- Raspberry Pi blinking an LED with the time.sleep() function
- Raspberry Pi blinking an LED with the millis() function
This method is not only limited to blinking LED and checking the button's state. It allows Raspberry Pi to carry out multiple tasks simultaneously without interfering with each other.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Wiring Diagram
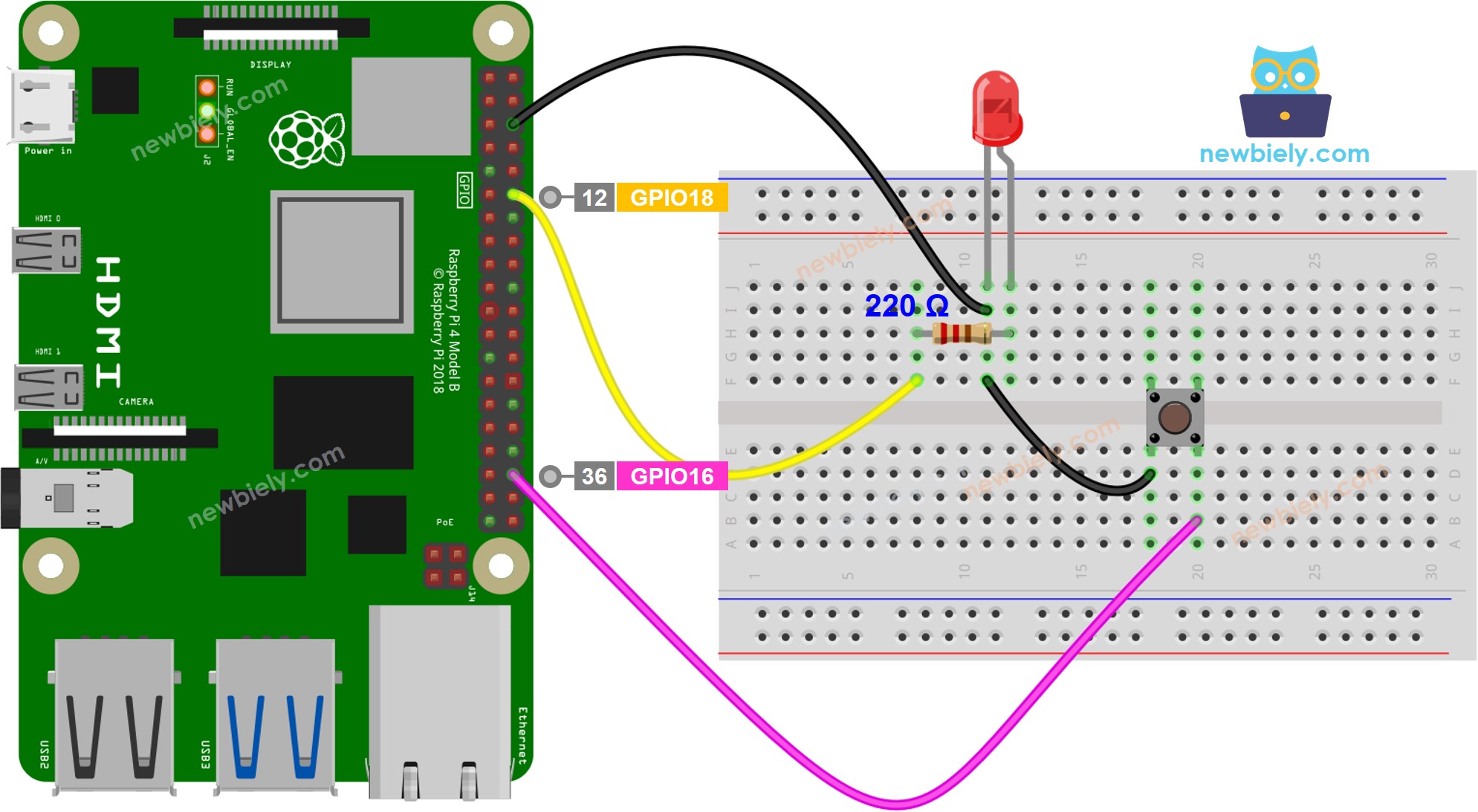
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
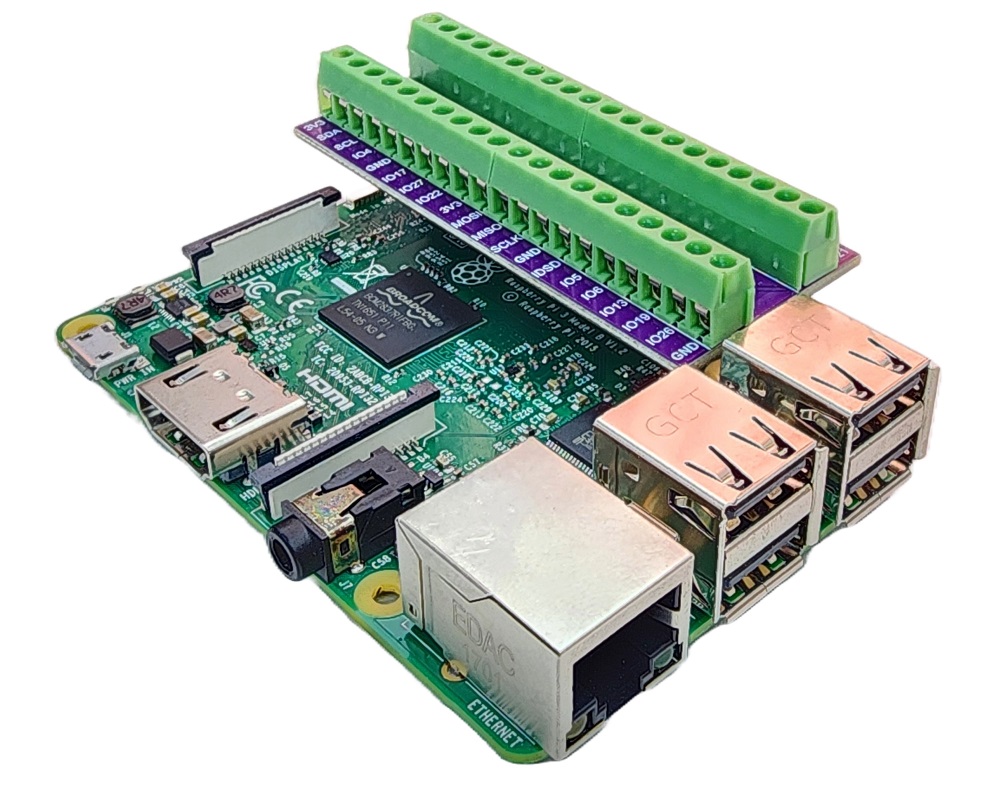
Raspberry Pi Code - With Delay
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Create a Python script file led_blink_with_delay.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Press the button four times.
- Check out the LED; it will alternate between being on and off every second.
- Look at the output in the Terminal.
- On the terminal, some pressing times were not recorded. This is due to the fact that Raspberry Pi is unable to do anything during delay time, thus it is not able to detect the pressing event.
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
Raspberry Pi Code - Without Delay
Detailed Instructions
- Create a Python script file led_blink_without_delay.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Press the button 4 times.
- Check out the LED, which will alternate between ON and OFF every second.
- Check the output in the Terminal.
- All pressed events were identified while the led was blinking.
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!
Video Tutorial
Extendability
This method allows Raspberry Pi to carry out multiple tasks concurrently without interfering with each other. For instance, sending a request to the Internet and waiting for the response, while at the same time blinking some LED indicators and checking the cancel button.