Raspberry Pi - GPS
This tutorial instructs you how to use Raspberry Pi with a NEO-6M GPS module. In detail, we will learn:
- How to connect Raspberry Pi to a NEO-6M GPS module
- How to program Raspberry Pi to read GPS coordinates (longitude, latitude, and altitude) from a NEO-6M GPS module
- How to program Raspberry Pi to calculate the distance from the current GPS position to a predefined GPS coordinate (e.g. coordinates of London).
Apart from longitude, latitude, and altitude, Raspberry Pi is also able to read GPS speed (km/h), and date time from a NEO-6M GPS module.
Hardware Preparation
1 | × | Raspberry Pi 4 Model B | |
1 | × | NEO-6M GPS module | |
1 | × | Jumper Wires | |
1 | × | (Optional) Screw Terminal Adapter for Raspberry Pi |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you. We appreciate your support.
Overview of NEO-6M GPS module
NEO-6M GPS module is a GPS module that can provide the following information:
- Longitude
- Latitude
- Altitude
- GPS speed (km/h)
- Date time
The NEO-6M GPS Module Pinout
The NEO-6M GPS module has four pins:
- VCC pin: This should be connected to the VCC (3.3V or 5V)
- GND pin: This should be connected to GND (0V)
- TX pin: This is used for serial communication and should be connected to the Serial RX pin on Raspberry Pi.
- RX pin: This is used for serial communication and should be connected to the Serial TX pin on Raspberry Pi.
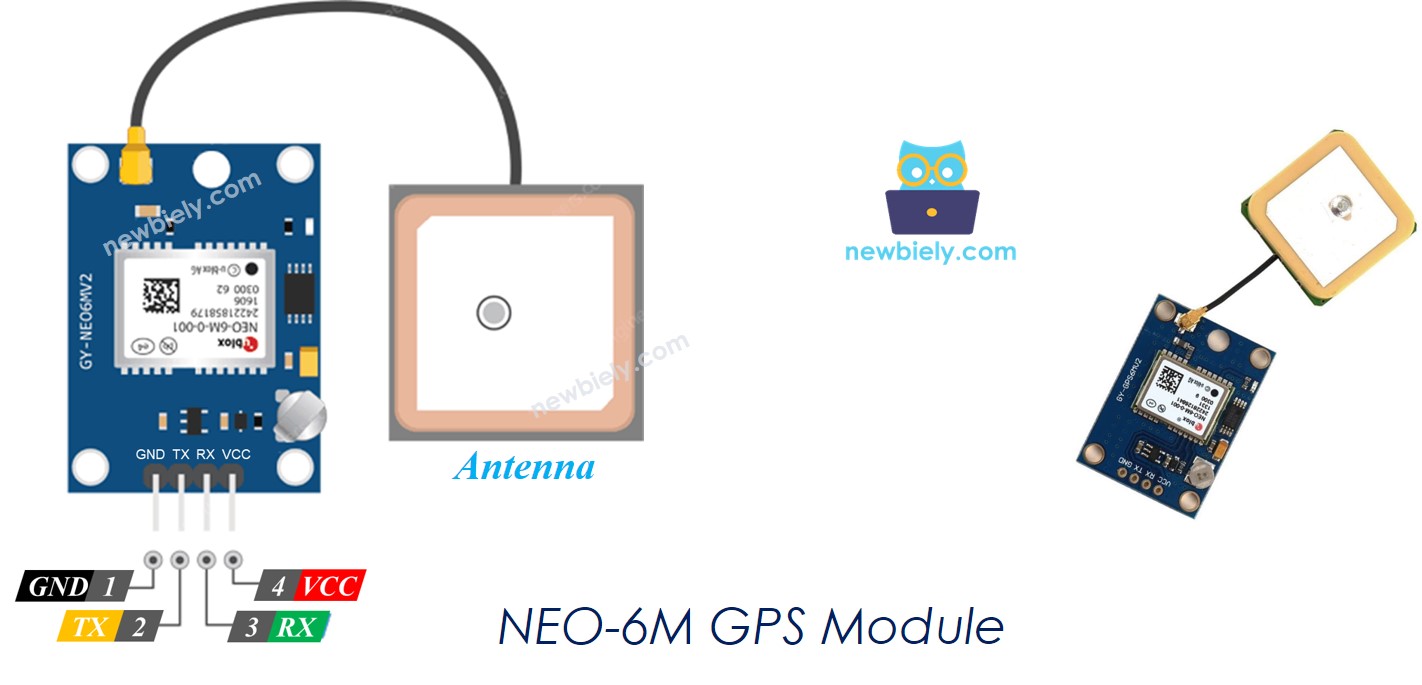
Wiring Diagram
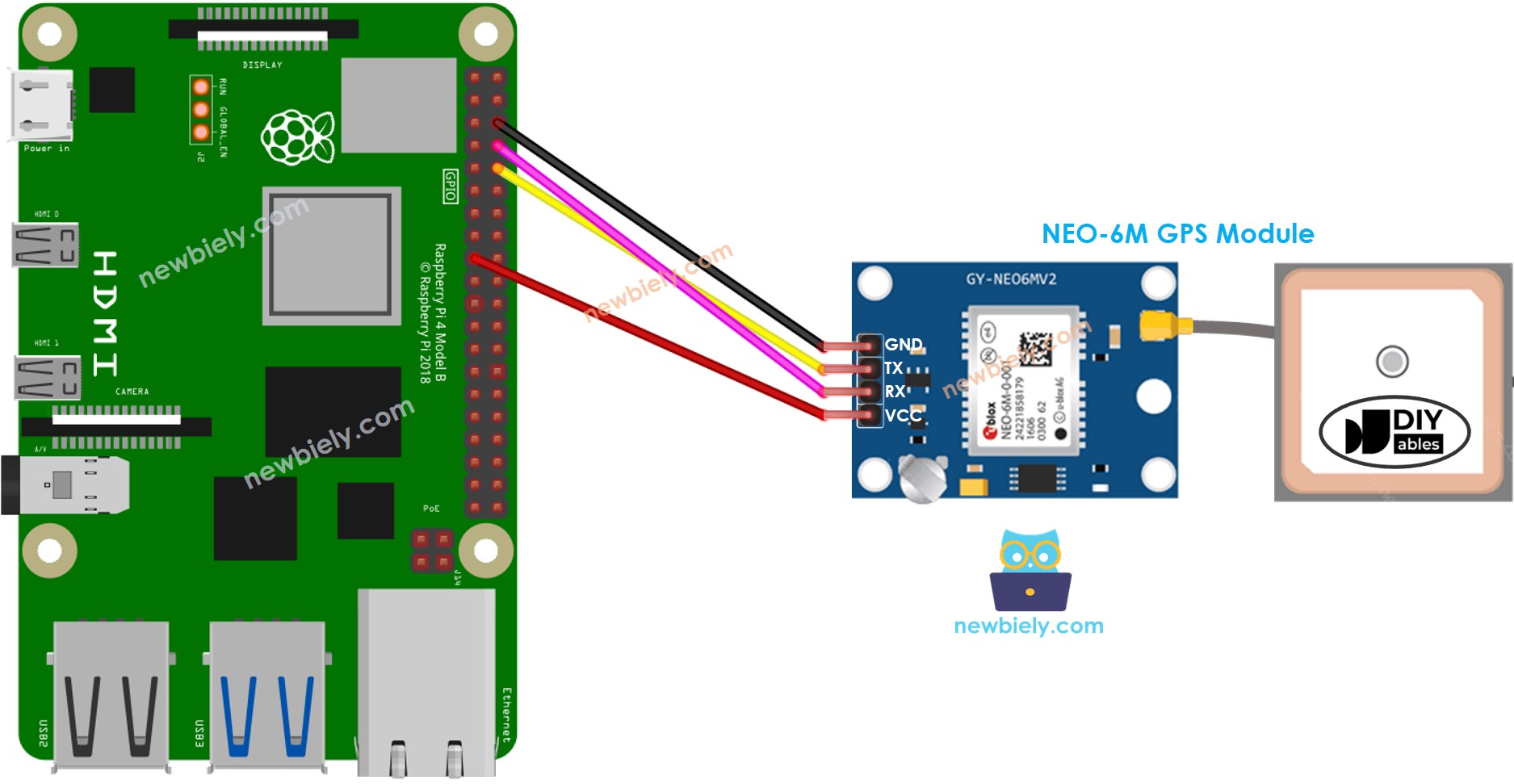
This image is created using Fritzing. Click to enlarge image
Raspberry Pi Code
Reading GPS coordinates, speed (km/h), and date time
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
sudo apt-get update
sudo apt-get install python3-rpi.gpio
- Enable the Serial interface on Raspberry Pi by following the instruction on Raspberry Pi - how to enable Serial inteface
- Install the pyserial library for communication with GPS module:
pip install pyserial
- Create a Python script file gps.py and add the following code:
# This Raspberry Pi code was developed by newbiely.com
# This Raspberry Pi code is made available for public use without any restriction
# For comprehensive instructions and wiring diagrams, please visit:
# https://newbiely.com/tutorials/raspberry-pi/raspberry-pi-gps
import serial
import time
from datetime import datetime
GPS_BAUD = 9600
# Create serial object for GPS
GPS = serial.Serial('/dev/serial0', GPS_BAUD, timeout=1)
print("Raspberry Pi - GPS Module")
try:
while True:
if GPS.in_waiting > 0:
gps_data = GPS.readline().decode('utf-8').strip()
if gps_data.startswith('$GPGGA'):
# Process GPS data using TinyGPS++
# You may need to adapt this part based on the structure of your GPS data
print(f"Received GPS data: {gps_data}")
# Extract relevant information
data_parts = gps_data.split(',')
latitude = data_parts[2]
longitude = data_parts[4]
altitude = data_parts[9]
# Print extracted information
print(f"- Latitude: {latitude}")
print(f"- Longitude: {longitude}")
print(f"- Altitude: {altitude} meters")
# You can add more processing as needed
time.sleep(1)
except KeyboardInterrupt:
print("\nExiting the script.")
GPS.close()
- Save the file and run the Python script by executing the following command in the terminal:
python3 gps.py
- Check out the result on the Terminal.
PuTTY - Raspberry Pi
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
Calculating the distance from current location to a predefined location
The below code computes the distance between the current spot and London (latitude: 51.508131, longitude: -0.128002).
Detailed Instructions
- Install the geopy library for the distance calculation:
pip3 install geopy
- Create a Python script file gps_distance.py and add the following code:
# This Raspberry Pi code was developed by newbiely.com
# This Raspberry Pi code is made available for public use without any restriction
# For comprehensive instructions and wiring diagrams, please visit:
# https://newbiely.com/tutorials/raspberry-pi/raspberry-pi-gps
import serial
import time
from geopy.distance import geodesic
GPS_BAUD = 9600
LONDON_LAT = 51.508131
LONDON_LON = -0.128002
# Create serial object for GPS
gps = serial.Serial('/dev/serial0', GPS_BAUD, timeout=1)
print("Raspberry Pi - GPS Module")
try:
while True:
if gps.in_waiting > 0:
gps_data = gps.readline().decode('utf-8').strip()
if gps_data.startswith('$GPGGA'):
# Process GPS data using TinyGPS++
# You may need to adapt this part based on the structure of your GPS data
print(f"Received GPS data: {gps_data}")
# Extract relevant information
data_parts = gps_data.split(',')
latitude = float(data_parts[2])
longitude = float(data_parts[4])
# Print extracted information
print(f"- Latitude: {latitude}")
print(f"- Longitude: {longitude}")
# Calculate distance to London using geopy
current_location = (latitude, longitude)
london_location = (LONDON_LAT, LONDON_LON)
distance_km = geodesic(current_location, london_location).kilometers
# Print calculated distance
print(f"- Distance to London: {distance_km:.2f} km")
time.sleep(1)
except KeyboardInterrupt:
print("\nExiting the script.")
gps.close()
- Save the file and run the Python script by executing the following command in the terminal:
python3 gps_distance.py
- Check out the result on the Terminal.
PuTTY - Raspberry Pi