Raspberry Pi - Obstacle Sensor
This tutorial instructs you how to use Raspberry Pi with the infrared obstacle avoidance sensor. In detail, we will learn:
- How to connect the Raspberry Pi to the infrared obstacle avoidance sensor.
- How to program the Raspberry Pi to read the state of the infrared obstacle avoidance sensor.
- How to program the Raspberry Pi to detect obstacles.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of IR Obstacle Avoidance Sensor
The infrared obstacle sensor is capable of detecting the presence of an object in front of it by using infrared signals. The range of detection is from 2cm to 30cm, and can be adjusted with a built-in potentiometer.
The Infrared Obstacle Avoidance Sensor Pinout
The IR obstacle avoidance sensor has three pins:
- GND pin: must be connected to GND (0V)
- VCC pin: must be connected to VCC (5V or 3.3v)
- OUT pin: is an output pin, LOW when an obstacle is present, HIGH when there is no obstacle. This pin should be connected to a Raspberry Pi input pin.
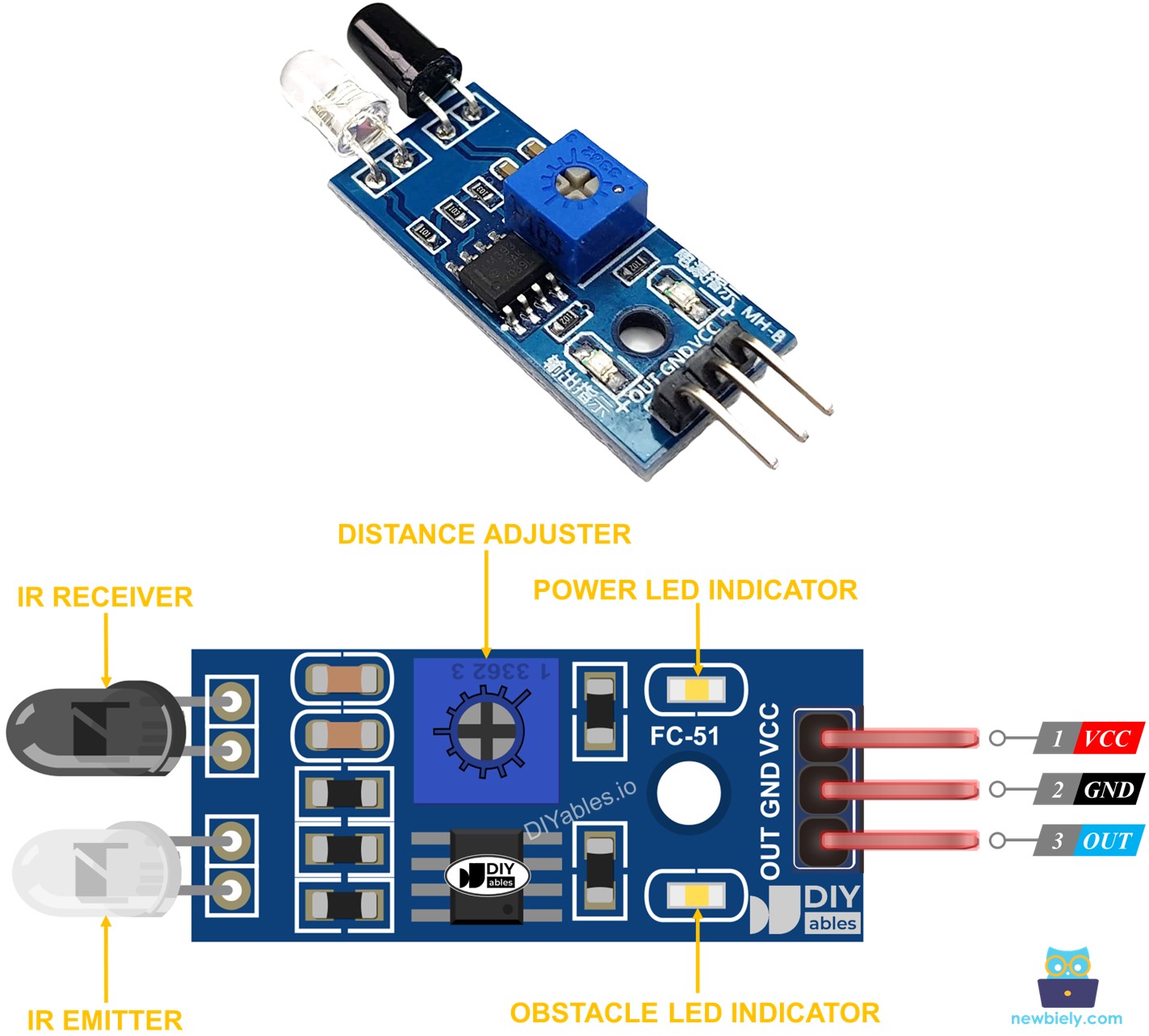
How It Works
The infrared obstacle sensor module has a built-in IR transmitter and IR receiver. The IR transmitter sends out the IR signal. The IR receiver looks for the reflected IR signal to detect if an object is present or not. The OUT pin of the sensor reflects the presence of an obstacle:
- If there is an obstacle in front of the sensor, the OUT pin of the sensor will be LOW
- If there is no obstacle in front of the sensor, the OUT pin of the sensor will be HIGH
※ NOTE THAT:
During shipment, the sensor may get deformed, which can cause malfunctions. If the sensor is not working properly, adjust the IR transmitter and receiver to ensure they are parallel to each other.
Wiring Diagram
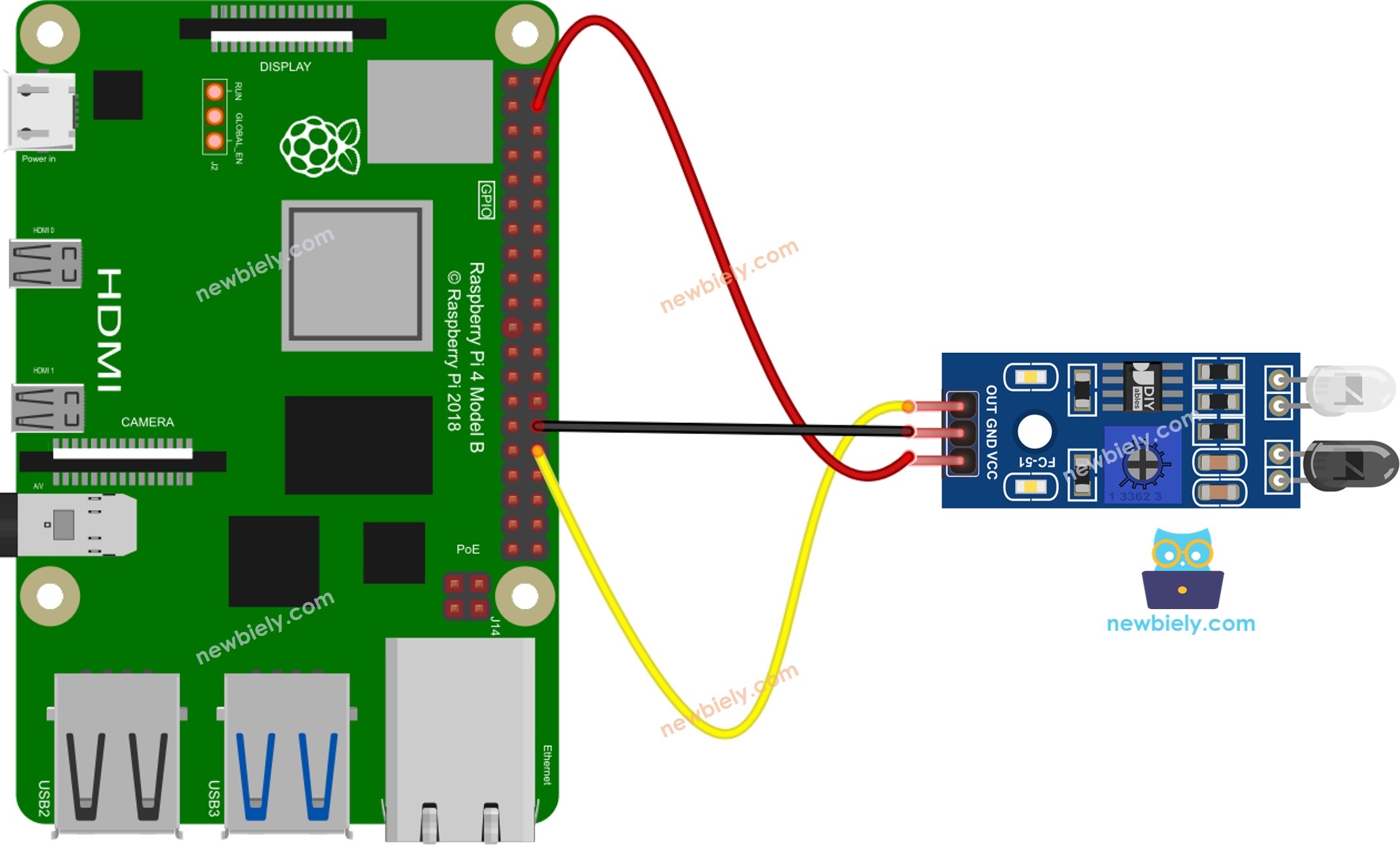
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
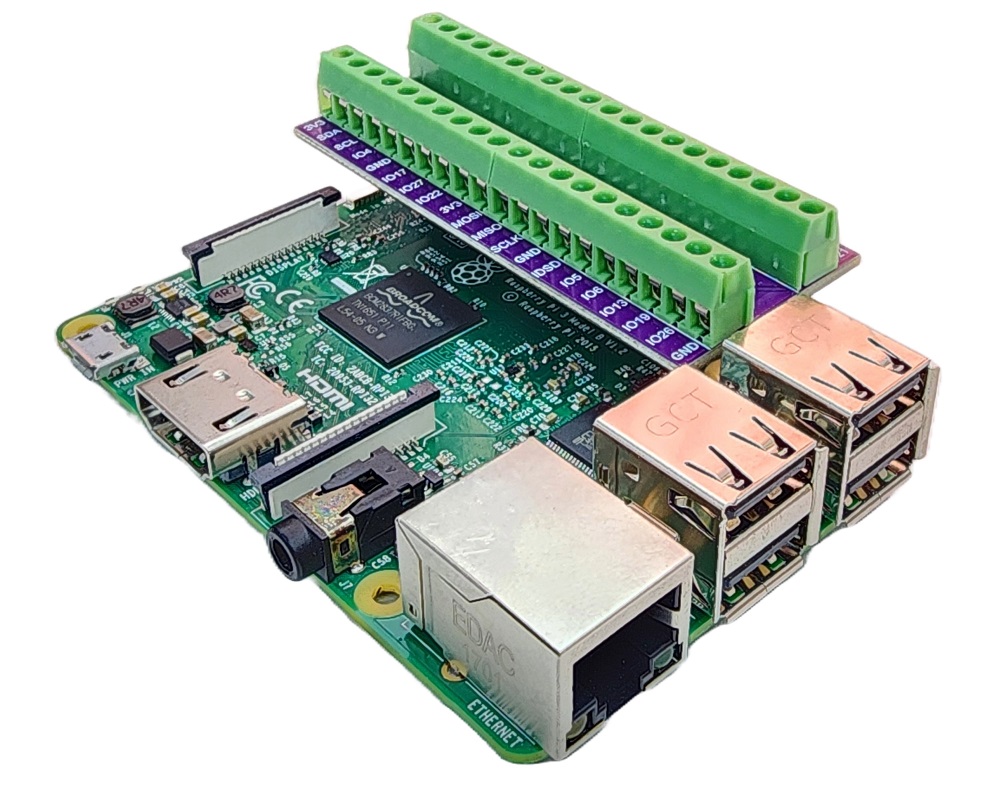
How To Program For IR Obstacle Avoidance Sensor
- Initializes the Raspberry Pi pin to digital input mode using the GPIO.setup() function.
- Utilize the GPIO.input() function to examine the state of the Raspberry Pi pin.
Raspberry Pi Code
Two approaches can be taken when programming for an obstacle avoidance application:
- Taking action depending on whether the obstacle is present or not
- Taking action based on whether the obstacle has been detected or removed
Raspberry Pi code for checking if the obstacle is present
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Create a Python script file obstacle_sensor.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Place an obstacle in front of the sensor for a period of time, then take it away.
- Check the outcome in the Terminal.
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
Raspberry Pi code for detecting obstacle
Detailed Instructions
- Create a Python script file obstacle_sensor_events.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Place an obstacle in front of the sensor for a while, then take it away.
- Check the results on the Terminal.