Raspberry Pi - Irrigation
This tutorial instructs you how to create an automatic irrigation system by using Raspberry Pi, a soil moisture sensor, relay, and pump. In detail, we learn how to make two application use-cases:
- Application 1:
- Raspberry Pi will control the relay to turn on the pump when the soil moisture is dry, thus watering the plants.
- Raspberry Pi will control the relay to turn off the pump when the soil moisture is wet.
- Application 2:
- Raspberry Pi will control the relay to turn on the pump 5 seconds if the soil moisture is dry, and then turn the pump off, and check the soil moisture again.
- Raspberry Pi - Soil Moisture Sensor tutorial
- Raspberry Pi - Controls Pump tutorial
- The Raspberry Pi will control the relay to turn on the pump when the soil moisture is dry, watering the plants.
- The Raspberry Pi will control the relay to turn off the pump when the soil moisture is wet.
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Install the Adafruit_ADS1x15 library by running the following commands on your Raspberry Pi terminal:
- Calibrate the sensor to get the wet-dry THRESHOLD value by following the instructions in Raspberry Pi - Calibrates Soil Moisture Sensor.
- Create a Python script file auto_irrigation_1.py and add the following code:
- Update the calibrated THRESHOLD value in the code.
- Save the file and run the Python script by executing the following command in the terminal:
- Check the result on the Terminal.
- The Raspberry Pi will turn on the pump when the soil is dry for 5 seconds, and then it will turn off the pump.
- Create a Python script file auto_irrigation_2.py and add the following code:
- Update the calibrated THRESHOLD value in the code.
- Save the file and run the Python script by executing the following command in the terminal:
- Check the result on the Terminal.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Buy Note: Many soil moisture sensors available in the market are unreliable, regardless of their version. We strongly recommend buying the sensor with TLC555I Chip from the DIYables brand using the link provided above. We tested it, and it worked reliably.
Overview of soil moisture sensor and Pump
If you are unfamiliar with pump and soil moisture sensor (including pinout, operation, programming, etc.), the following tutorials can help:
Wiring Diagram
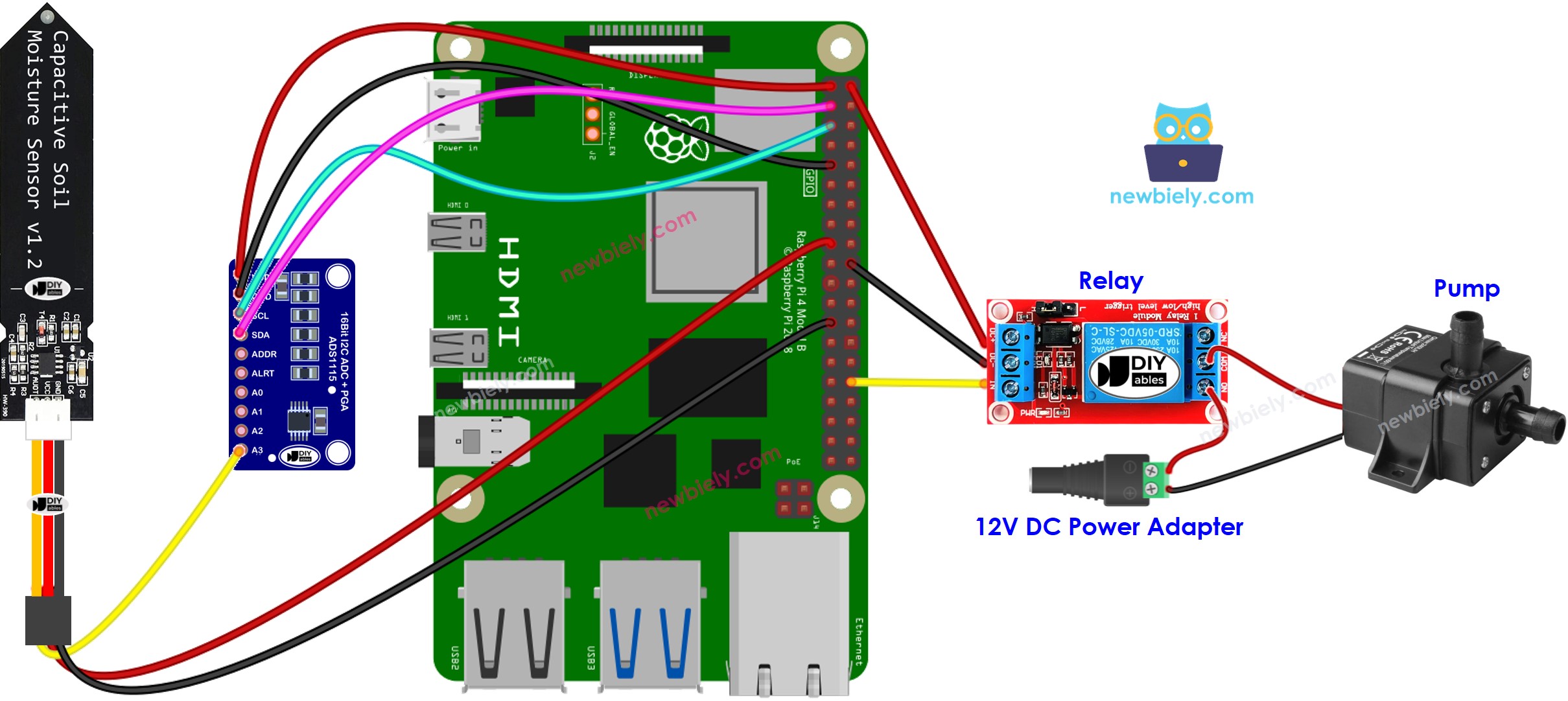
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
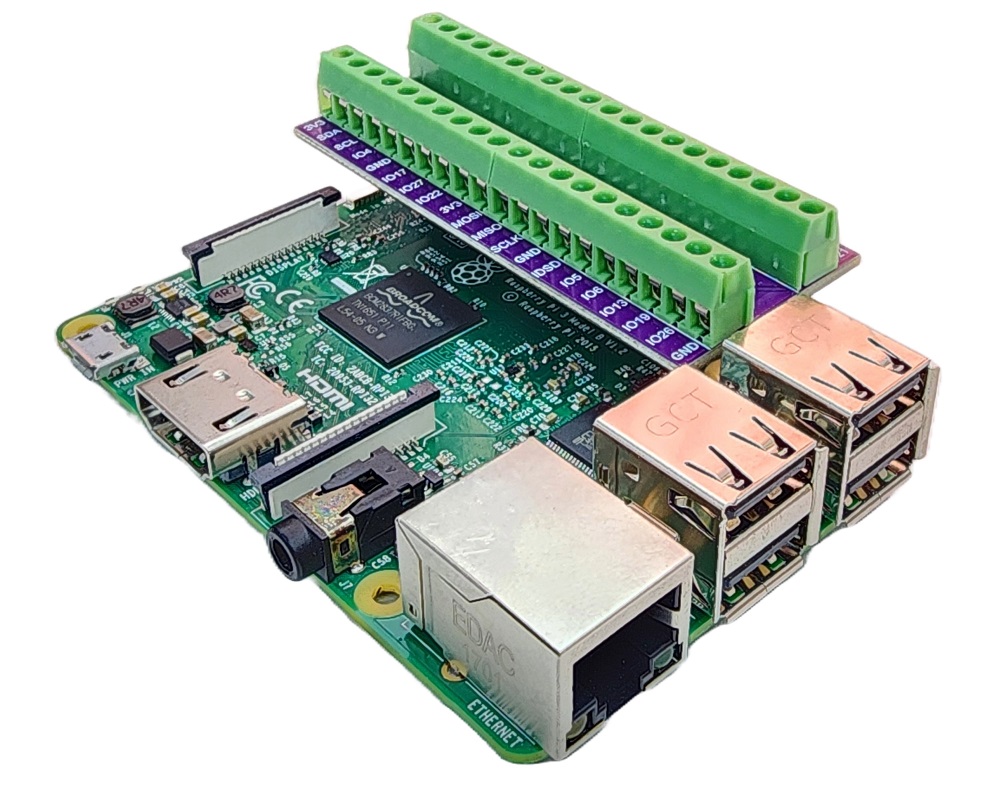
Raspberry Pi Code - Application 1
We'll create the irrigation system as follows:
Detailed Instructions
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!
Raspberry Pi Code - Application 2
We'll make the irrigation system work like this: