Raspberry Pi - Motion Sensor
Have you ever asked yourself, “How can it do that?” when you come across places with automatic doors, lights, and escalators? If so, this tutorial will not only answer your question, but also show you how to make it happen by using Raspberry Pi and motion sensor. Let's get started!
This tutorial instructs you how to use Raspberry Pi with motion sensor. In detail, we will learn:
- How HC-SR501 motion sensor works
- How to connect the HC-SR501 motion sensor to Raspberry Pi
- How to program Raspberry Pi to read the state from the HC-SR501 motion sensor
- How to use Raspberry Pi and HC-SR501 motion to detect the motion state changes.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of HC-SR501 Motion Sensor
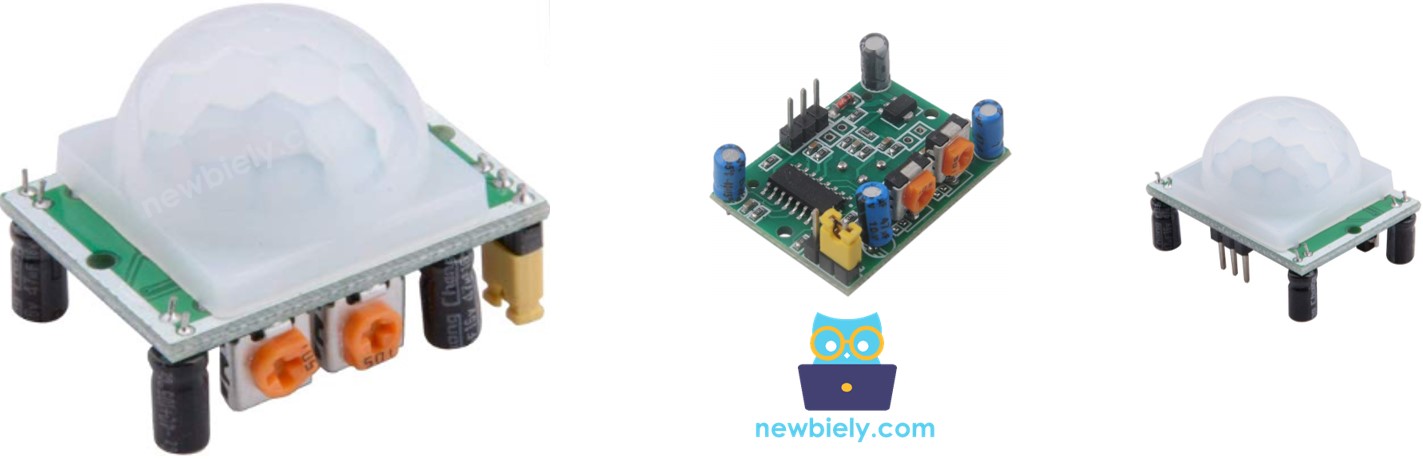
The HC-SR501 PIR sensor is capable of detecting the motion of humans (or animals). It is widely used in a variety of applications, such as automatically turning on/off light bulbs, opening/closing doors, activating/deactivating escalators, and detecting intruders.
The Motion Sensor Pinout
The HC-SR501 motion sensor has 3 pins:
- GND pin: must be linked to GND (0V)
- VCC pin: must be linked to VCC (5V)
- OUTPUT pin: is an output pin that is LOW when no motion is detected and HIGH when motion is detected. This pin should be connected to a Raspberry Pi's input pin.
The HC-SR501 has one jumper and two potentiometers. These are used to adjust the settings of the sensor.
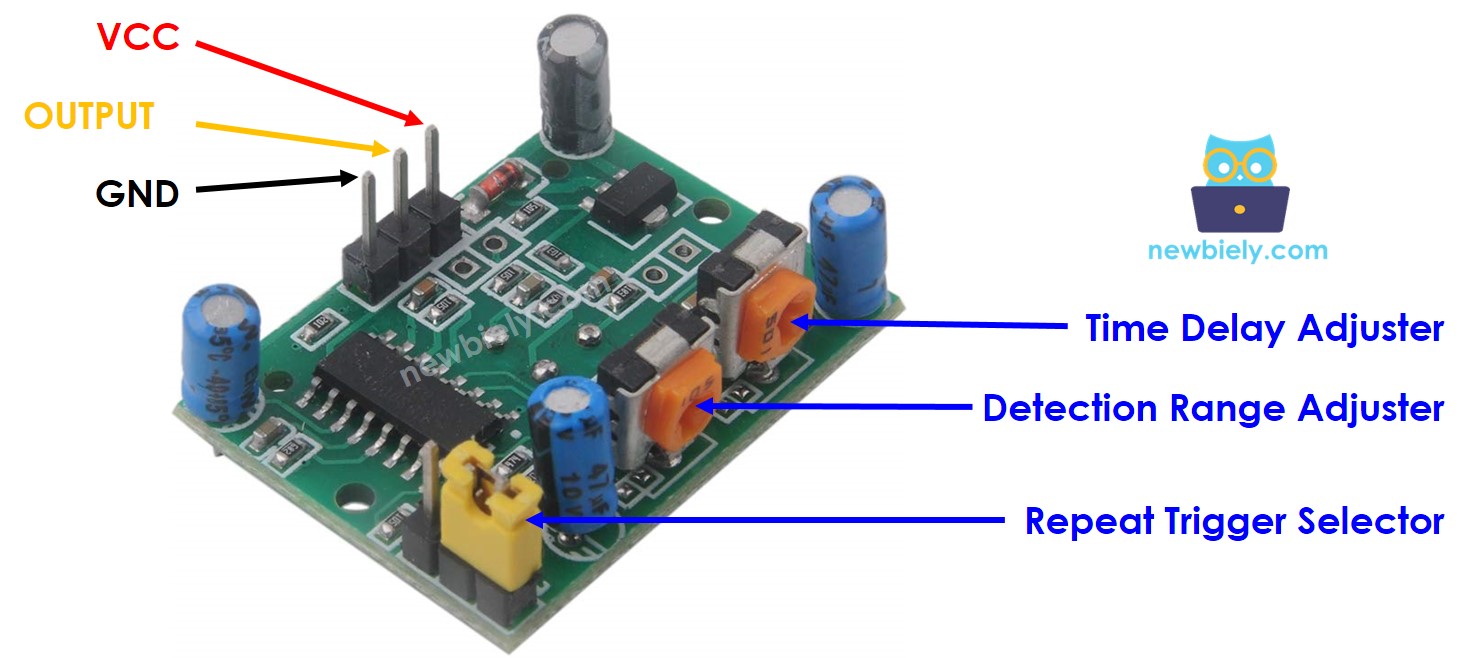
How It Works
The HC-SR501 sensor is able to recognize motion based on changes in the infrared radiation emitted by a moving object. In order to be identified by the HC-SR501 sensor, the object must satisfy two criteria:
- It must be in motion or vibrating.
- It must be radiating infrared light.
Thus:
- If an object is in motion but not emitting infrared rays (e.g., a robot or vehicle toy), it will not be detected by the sensor.
- If an object is emitting infrared rays but is not moving (e.g., a person standing still), it will not be detected by the sensor.
Humans and animals are sources of infrared radiation. Consequently, the sensor can recognize their movements.
State of OUTPUT pin:
- When no human (or animal) is present in the range detected by the sensor, the OUTPUT pin is LOW.
- If a human (or animal) enters the range detected by the sensor, the OUTPUT pin changes from LOW to HIGH, indicating motion has been detected.
- If the human (or animal) leaves the range detected by the sensor, the OUTPUT pin changes from HIGH to LOW, indicating the motion has ended.
The video above shows the way the motion sensor operates in theory. In reality, the motion sensor works slightly differently, depending on the sensor settings (which are discussed in the Advanced Uses section).
Detect the Presence of Human
The sensor does not recognize the presence of humans. It only detects motion. We use a Raspberry Pi to infer people's presence based on the motion detected by the sensor, following this principle:
- If motion is observed, then people are present.
- If no motion is detected, then people are absent.
This rule is imperfect in a practical scenario: when humans are within the range of the sensor but are not moving, no motion is detected. Consequently, the Raspberry Pi concludes that the human is not present.
For instance, in your meeting room, the motion sensor is used to switch the light on and off. When people enter the room, the light will be activated automatically. If everyone remains still during the meeting, no motion will be detected, indicating that no one is present, thus the light will be switched off. To turn the light back on, someone needs to move.
However, this issue is NOT serious and the sensor is inexpensive. Therefore, it is widely used to detect humans in many applications.
Raspberry Pi - HC-SR501 Motion Sensor
Connect a Raspberry Pi's digital input pin to the OUTPUT pin of the HC-SR501 sensor. Using the Raspberry Pi code, we can check the value of the OUTPUT pin to detect motion.
Wiring Diagram
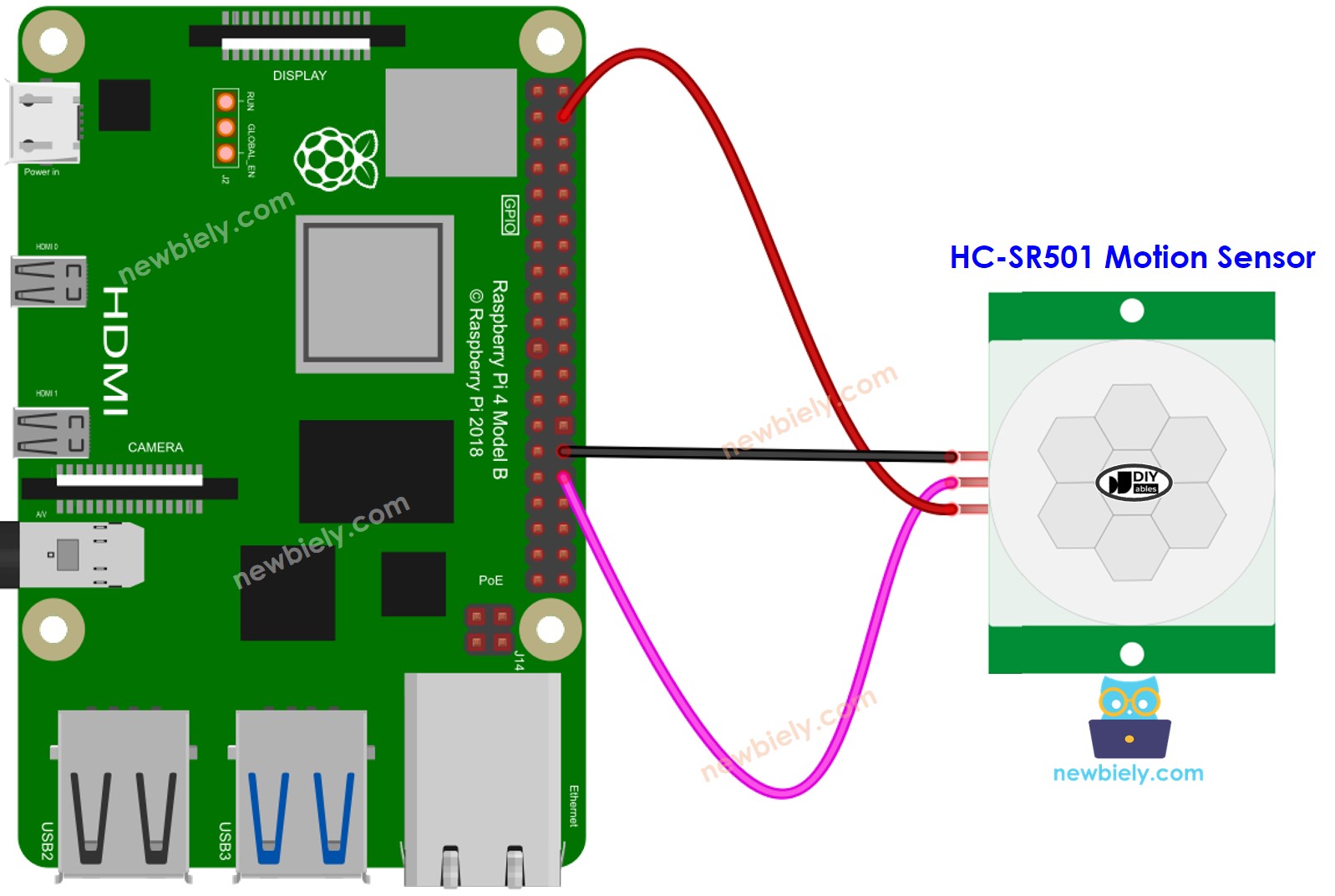
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
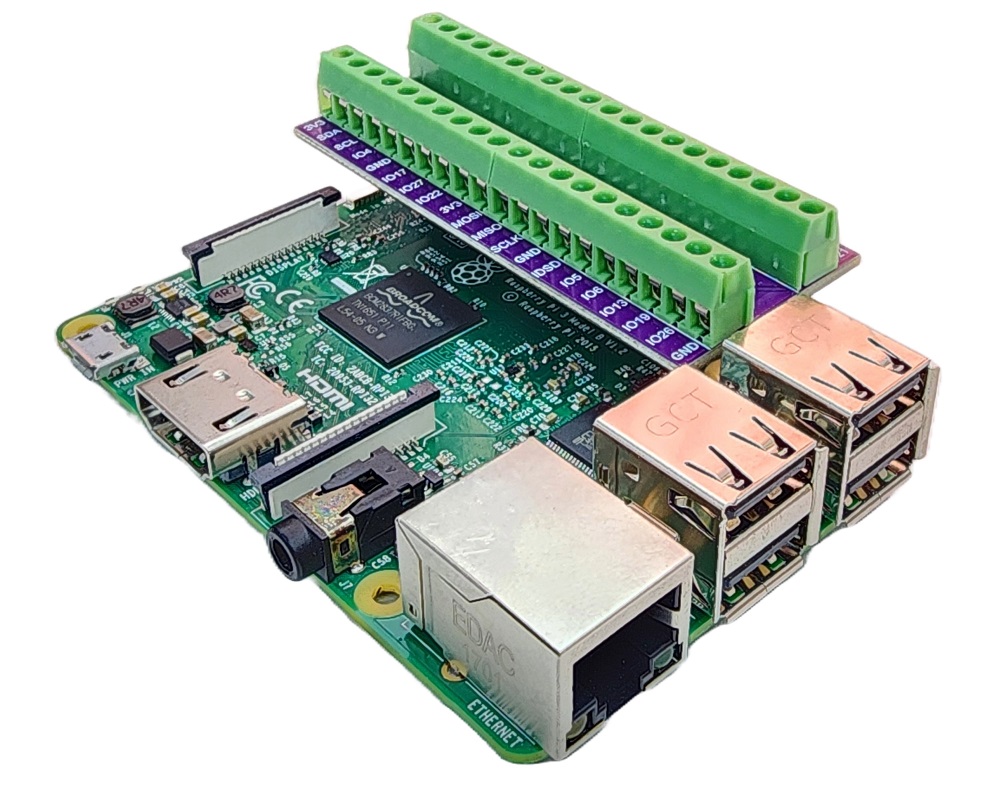
Initial Setting
Time Delay Adjuster | Screw it in anti-clockwise direction fully. |
Detection Range Adjuster | Screw it in clockwise direction fully. |
Repeat Trigger Selector | Put jumper as shown on the image. |
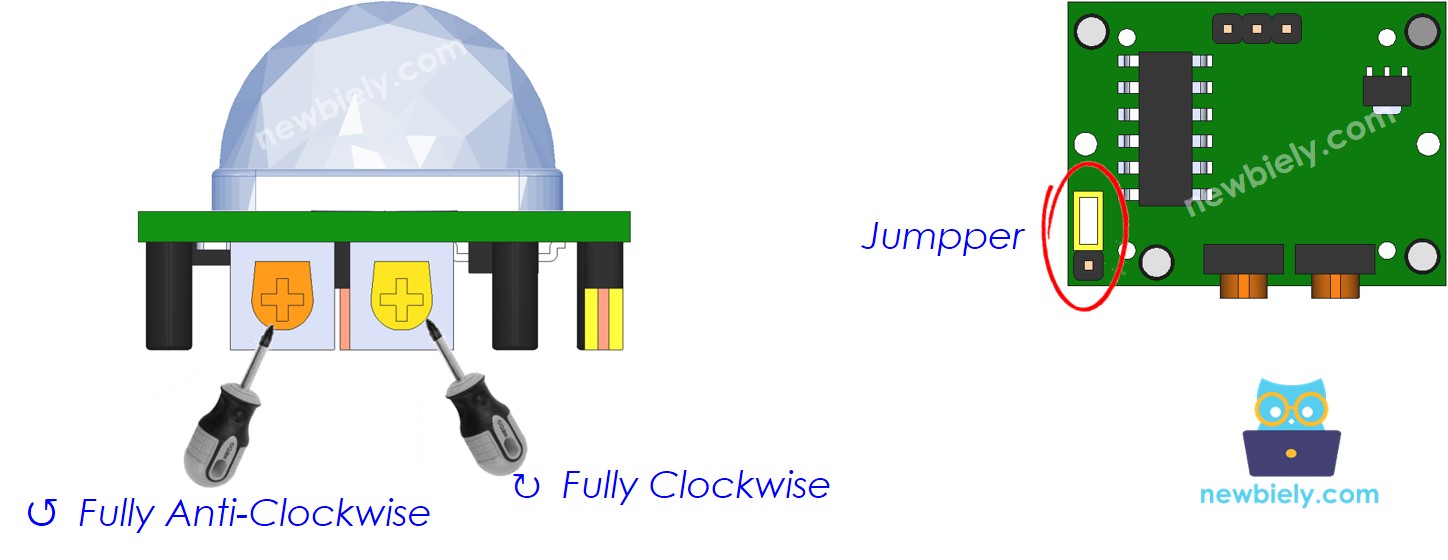
How To Program For Motion Sensor
- Set up a digital input on a Raspberry Pi's pin by utilizing the GPIO.setup() function.
- Access the OUTPUT pin of the sensor by utilizing the GPIO.input() function.
Raspberry Pi Code - Print the motion state
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Create a Python script file motion_sensor.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Move your hand in front of the range of the sensor.
- Check the output in the Terminal.
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
The code above continuously checks the motion state and prints it. In certain cases, you might desire to print or take action upon detecting a change in the motion state. If such a scenario arises, check out the next session.
Raspberry Pi Code - Detects the Motion State Changes
- Create a Python script file motion_sensor_events.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Move your hand in front of the range of the sensor.
- Check the output in the Terminal.
Video Tutorial
Advanced Uses
As stated previously, one jumper and two potentiometers can be used to modify the sensor's configuration.
Detection Range Adjuster
This potentiometer is used to change how far something can be detected (between about 3 to 7 meters).
- Turning it all the way to the right makes it detect things up to about 3 meters away.
- Turning it all the way to the left makes it detect things up to about 7 meters away.
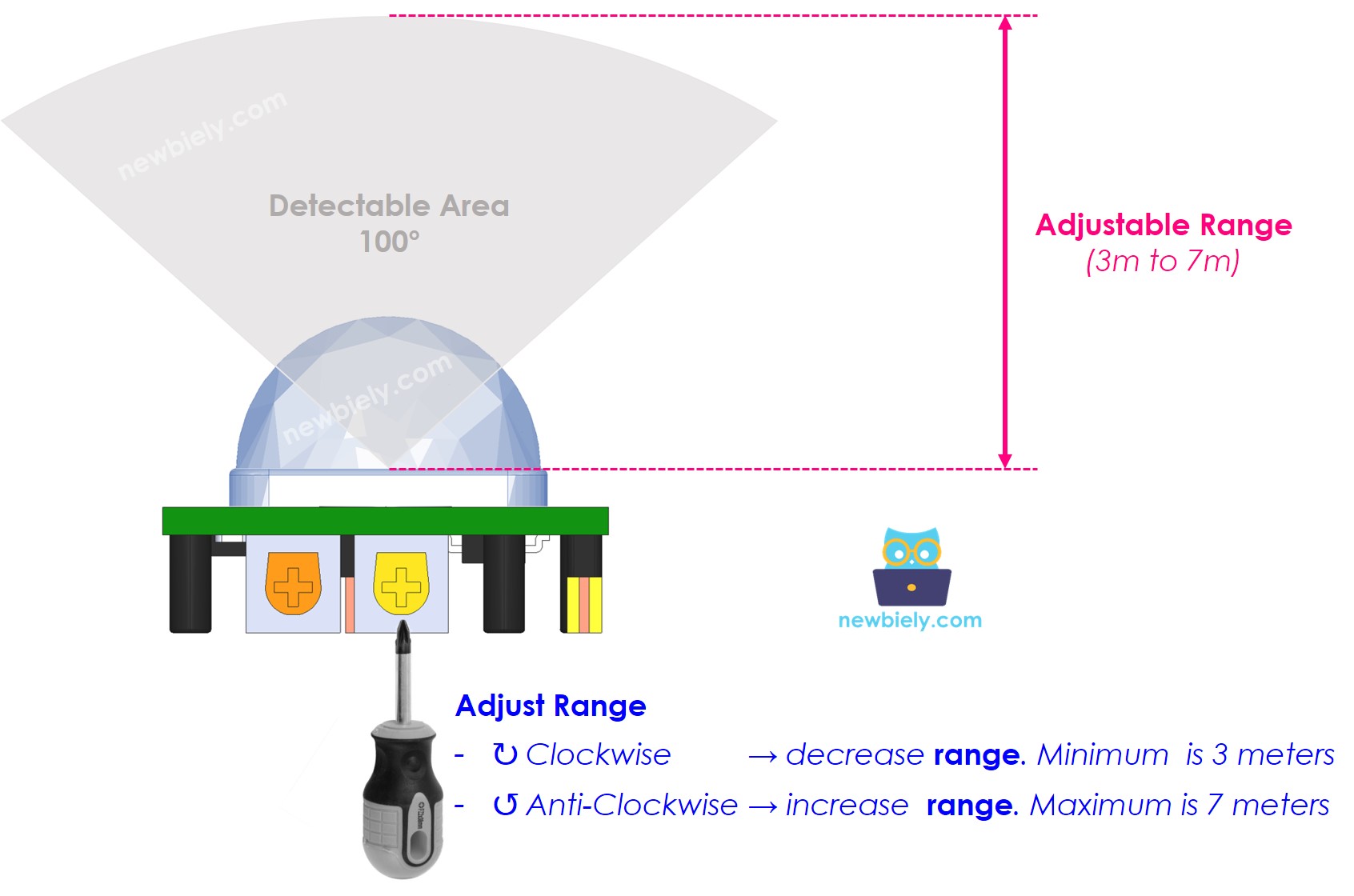
Time Delay Adjuster
This potentiometer is utilized to modify the time delay:
- When it is turned all the way clockwise, the time delay is around 5 minutes.
- When it is turned all the way counterclockwise, the time delay is roughly 3 seconds.
The next part elucidates the concept of time delay in relation to Repeat Trigger.
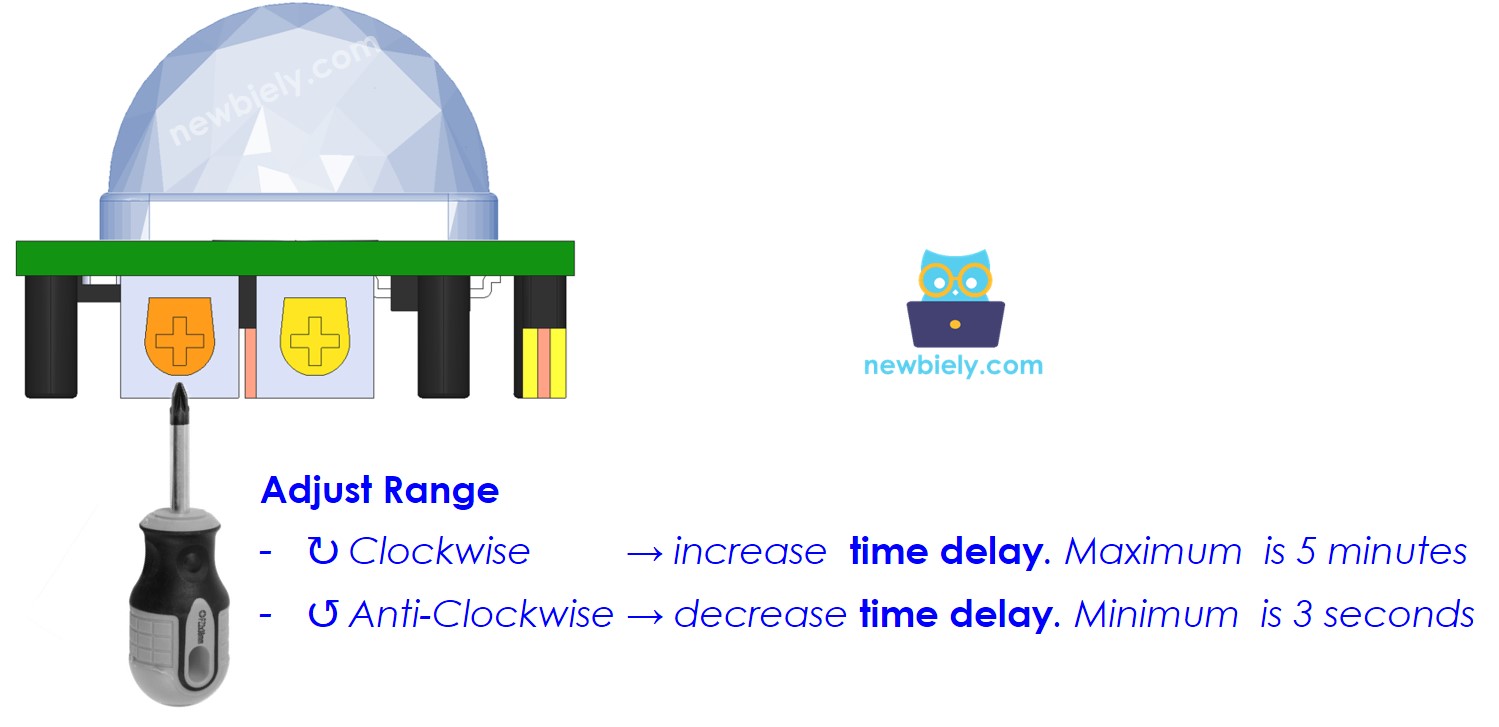
Repeat Trigger Selector
A jumper exists that is utilized to pick trigger modes: single trigger or repeatable trigger.
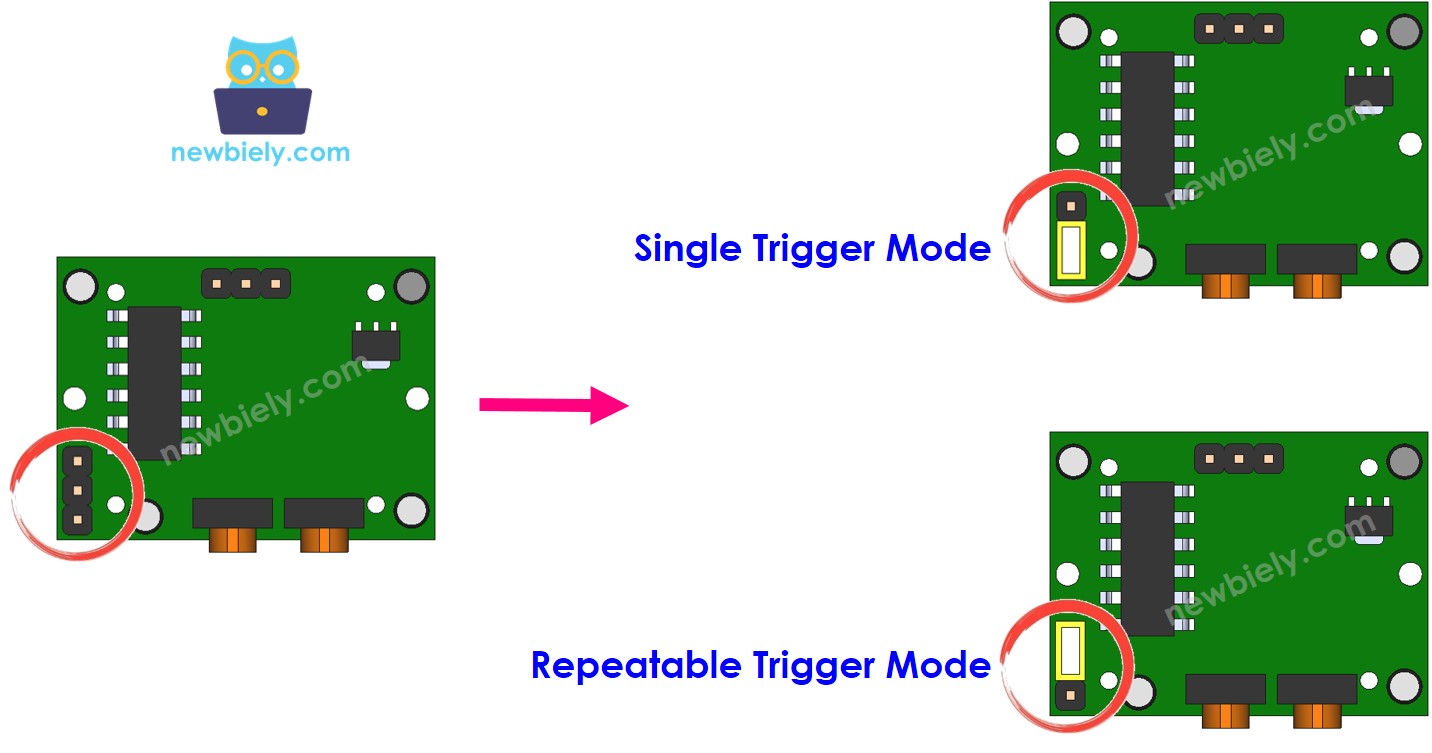
Let's refer to the time delay setting, which is set via the Time Delay Adjuster, as time_delay. If you keep moving in the range of the sensor for a long time (called motion_time, which is several times longer than time_delay), then in Single Trigger Mode, the OUTPUT pin's state will be toggled between LOW and HIGH several times. The HIGH duration will be equal to time_delay, while the LOW duration will be fixed to 3 seconds.
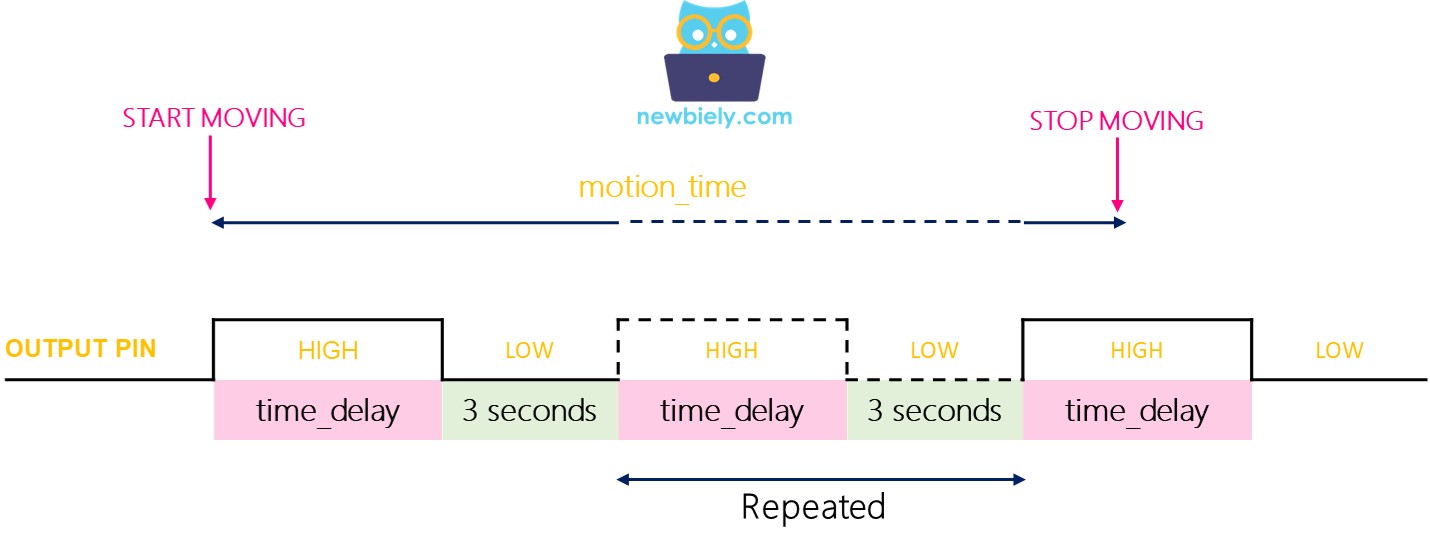
- Repeatable trigger mode: The state of the OUTPUT pin will remain HIGH for a period of (motion_time + time_delay).
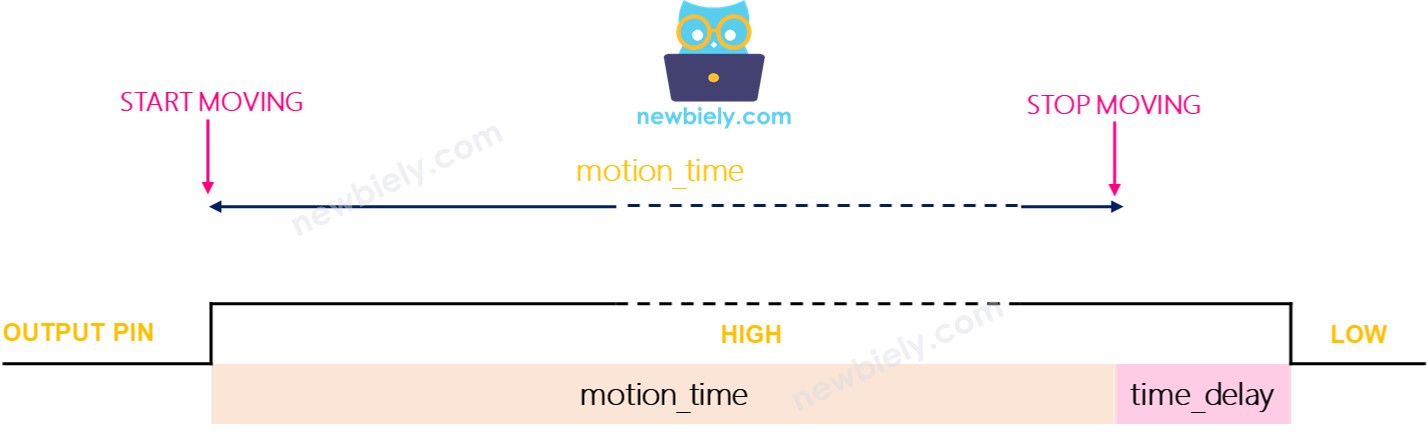
Testing
- Single trigger mode:
- Place the jumper to select single trigger mode
- Wave your hand in front of the sensor for around 10 seconds
- Move your hand away from the sensor
- Wait 3 seconds and you will observe the output in the serial monitor like this:
- Place the jumper to select Repeatable trigger mode
- Move your hand in front of the sensor for approximately 10 seconds
- Remove your hand from the sensor's range
- Wait for 3 seconds and you will observe the output in the serial monitor as follows:
- We switch on or activate machines/devices when a person is present
- We do not switch off or deactivate machines/devices right away when a person is no longer present. We turn them off or deactivate them after a timeout.
Repeatable trigger mode:
We can observe that when in single trigger mode, the sensor activates two or three times. Whereas, when in repeatable trigger mode, the sensor only triggers once.
※ NOTE THAT:
During the LOW (3 seconds) time, which is a fixed and unadjustable value, the sensor cannot detect any motion. In other words, it is blocked for this period of time, but this does not cause any issues.
It is suggested to use the repeatable trigger mode.
For many real-world applications:
How To Use Time Delay
If no human is detected, the automation system will wait for a period of time before taking action.
The motion sensor has a Time Delay Adjuster that can be set to a minimum of 3 seconds and a maximum of 5 minutes. Additionally, any value can be set on the Raspberry Pi code by coding.
If we do not specify a timeout in the Raspberry Pi code, the timeout will be equivalent to the time delay set in the sensor.