Raspberry Pi - Touch Sensor - Relay
This tutorial instructs you how to use the Raspberry Pi and touch sensor to control the relay. By connecting the relay to a soleniod lock, light bulb, LED strip, motor, or actuator..., we can use a touch sensor to control the them. We will learn two different applications:
Application 1 - The relay state is synchronized with the touch sensor state. In detail:
- Raspberry Pi turns on the relay when the touch sensor is being touched.
- Raspberry Pi turns off the relay when the touch sensor is NOT being touched.
Application 2 - The relay state is toggled each time the touch sensor is touched. More specifically:
- If Raspberry Pi detects that the touch sensor has been touched (changing from a LOW state to a HIGH state), it will turn ON the relay if it's currently OFF, or turn OFF the relay if it's currently ON.
- Releasing the touch sensor does not affect to the relay state.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of Relay and Touch Sensor
If you are unfamiliar with relay and touch sensor (including pinout, operation, and programming), the following tutorials can help:
Wiring Diagram
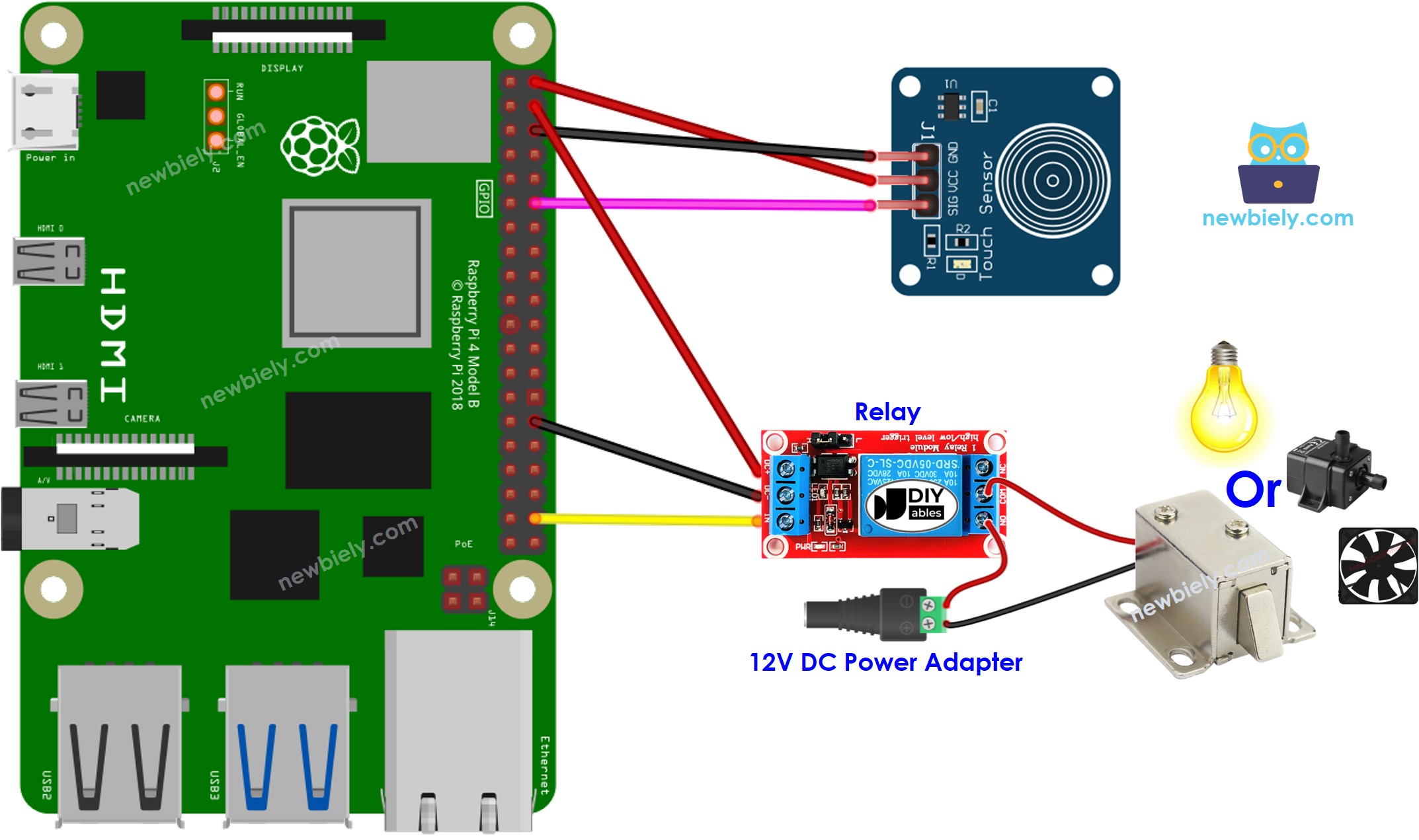
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
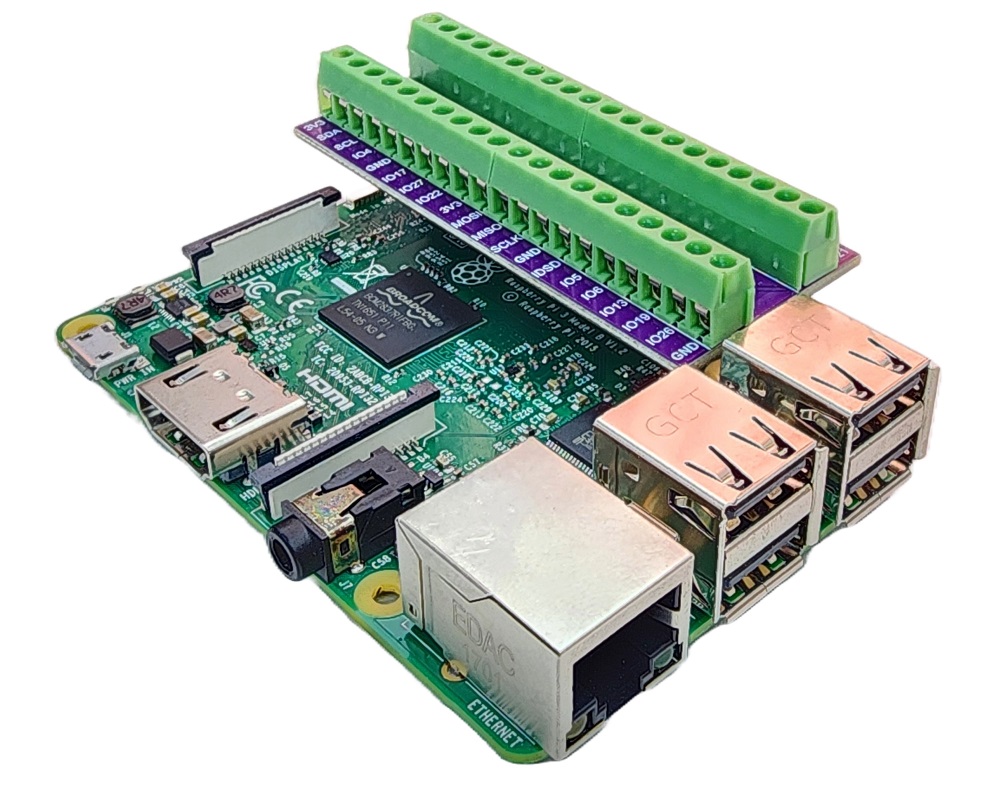
Application 1 - The relay state is in sync with the touch sensor state
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Create a Python script file touch_sensor_relay.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Touch your finger on the touch sensor and hold it for a few seconds, then release it.
- Check out the change in the relay's condition. You will see that the relay state is in sync with the touch sensor state.
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!
Application 2 - Touch Sensor toggles Relay
Detailed Instructions
- Create a Python script file touch_sensor_toggle_relay.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Touch and release the touch sensor several times.
- Check out the change in the relay's state. You'll notice that the relay will switch on or off one time whenever you place your finger on the touch sensor.