Raspberry Pi - Bluetooth
This tutorial instructs you how to use Raspberry Pi to control Bluetooth HC-05 Module. In detail, we will learn:
- The process of utilizing Bluetooth with Raspberry Pi
- Sending data from Raspberry Pi to a smartphone application through Bluetooth
- Receiving data on Raspberry Pi from the smartphone app via Bluetooth
- Controlling Raspberry Pi from the smartphone app by means of Bluetooth
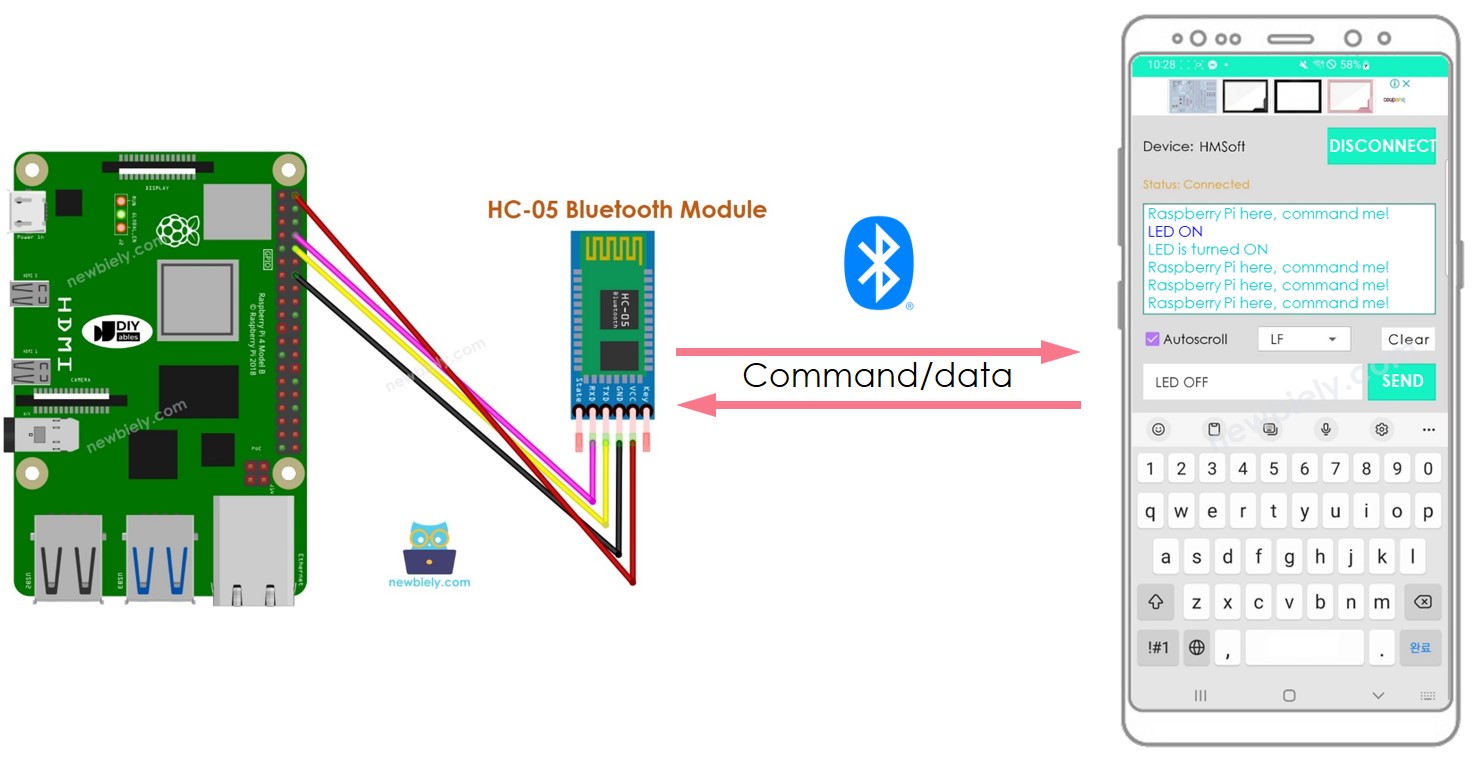
The purpose of this tutorial:
- To show how Raspberry Pi can exchange data with a smartphone app.
- To demonstrate the use of the HC-05 Bluetooth Module.
- To explain how to use the Bluetooth Serial Monitor App on Android.
- To illustrate how to control LED and servo motor from a smartphone app.
This tutorial is about utilizing Classic Bluetooth (Bluetooth 2.0). If you are searching for a Bluetooth Low Energy - BLE (Bluetooth 4.0), please refer to this similar tutorial: Raspberry Pi - Bluetooth Low Energy
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of HC-05 Bluetooth Module
HC-05 is a Serial Bluetooth module that functions as a Serial to Bluetooth Converter. It has the capability to:
- Receive data from the Serial RX pin and transmit it to the paired device (such as a smartphone) over Bluetooth
- Receive data from Bluetooth (from the paired device) and send it to the Serial TX pin.
Specifically, for Raspberry Pi to communicate with a smartphone App (Android/iOS):
- The Raspberry Pi is connected to an HC-05 Bluetooth Module through its Serial pins.
- The HC-05 Bluetooth Module is paired with the smartphone App.
- Raspberry Pi sends data to the smartphone App simply by sending it to the Serial.
- Raspberry Pi receives data from the smartphone App simply by reading it from the Serial.
- No special Bluetooth code is necessary on the Raspberry Pi.
The Bluetooth HC-05 Module Pinout
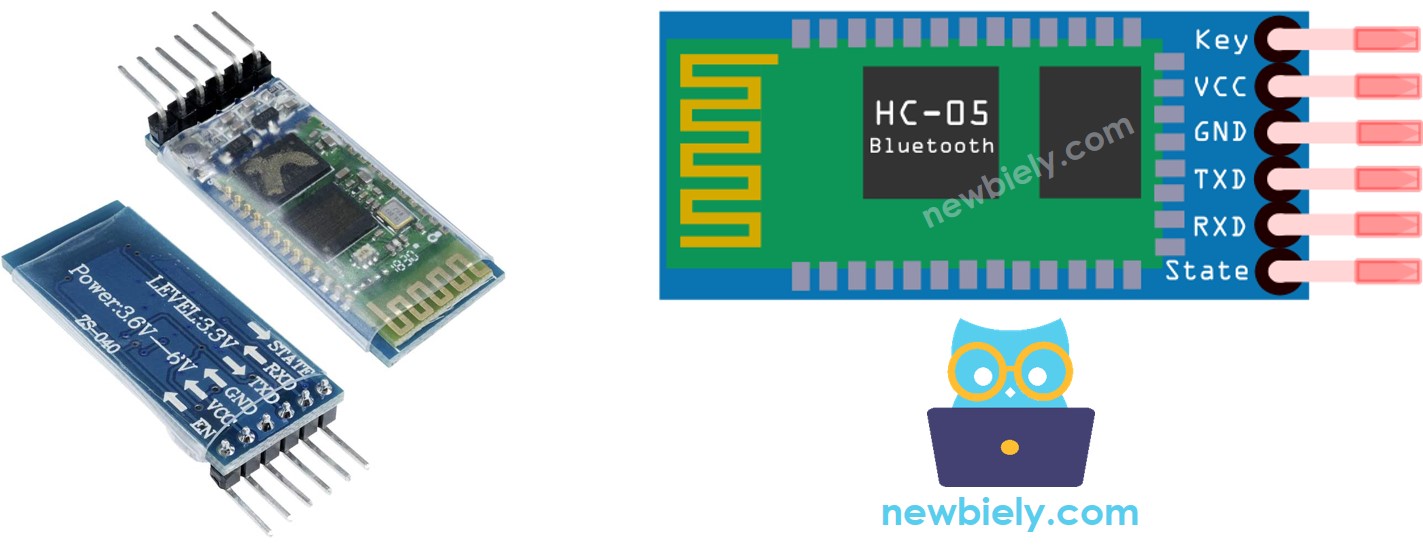
The HC-05 Bluetooth Module has 6 pins:
- Enable/Key pin: This pin is used to switch between Data Mode (set LOW) and Command mode (set HIGH). If it is not connected, it is in Data mode by default.
- VCC pin: power pin, this should be connected to +5V of the Supply voltage.
- GND pin: power pin, this should be connected to the GND of the power source.
- TX pin: Serial data pin, this should be connected to the RX pin of Raspberry Pi. The data received via Bluetooth will be sent to this pin as serial data.
- RX pin: Serial data pin, this should be connected to the TX pin of Raspberry Pi. The data received from this pin will be sent to Bluetooth.
- State: The state pin is connected to the onboard LED, it can be used as feedback to determine if Bluetooth is working properly.
Nevertheless, for fundamental operations, we only require 4 pins of The HC-05 Bluetooth Module to be linked to Raspberry Pi.
The HC-05 Bluetooth Module also includes two built-in elements:
- A LED: which indicates the status of the Module
- Blinking once every two seconds: indicating that the Module has entered Command Mode
- Repeated blinking: meaning it is waiting for connection in Data Mode
- Blinking twice per second: signifying a successful connection in Data Mode
- A Button: used to control the Key/Enable pin to choose the operation mode (Data or Command Mode)
How It Works
The HC-05 Bluetooth module has two operation modes:
- Data mode which is used to exchange data with the paired device
- Command Mode which is used to configure parameters.
Fortunately, the HC-05 Bluetooth module is capable of working with Raspberry Pi without any configuration, using its default setting.
HC-05 Default Settings
Default Bluetooth Name | “HC-05” |
---|---|
Default Password | 1234 or 0000 |
Default Communication | Slave |
Default Mode | Data Mode |
Default Data Mode Baud Rate | 9600, 8, N, 1 |
Default Command Mode Baud Rate | 38400, 8, N, 1 |
Overview of Bluetooth Serial Monitor App
The Bluetooth Serial Monitor App allows communication with Raspberry Pi via Bluetooth without any additional code for the Bluetooth module in the Raspberry Pi code. To use it, the following steps should be followed:
- Connect Raspberry Pi to HC-05 Bluetooth module
- Install Bluetooth Serial Monitor App on your smartphone
- Open the App and pair it with the HC-05 Bluetooth module
Now, you can transmit and receive data from Raspberry Pi.
Wiring Diagram
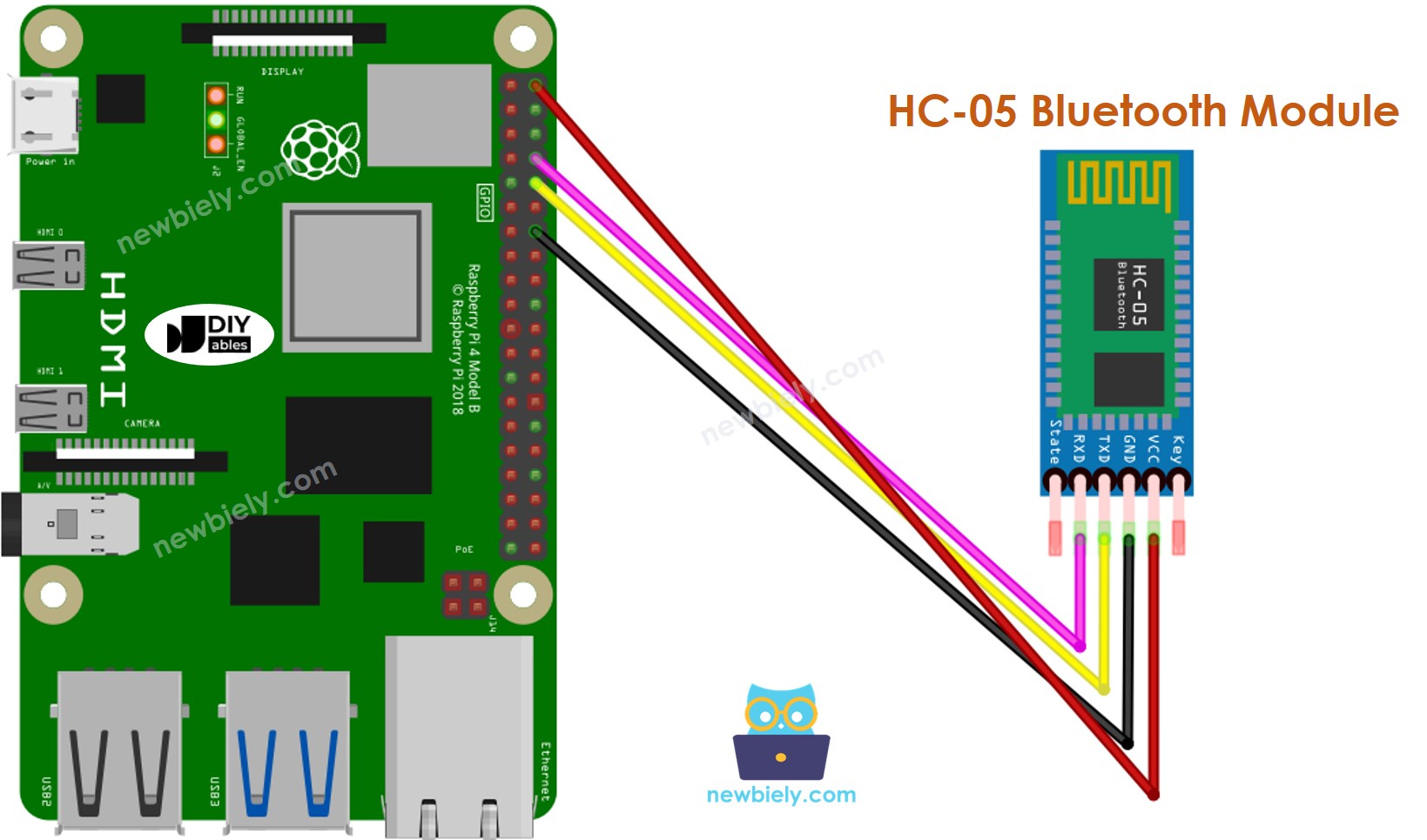
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
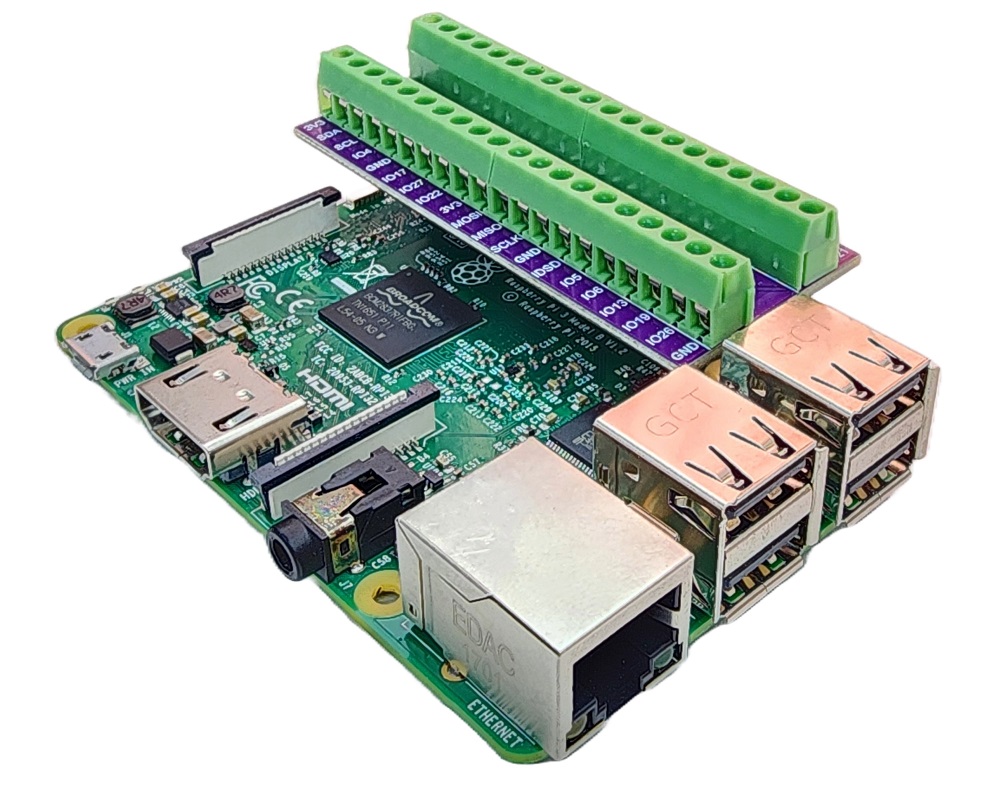
Table of Wiring Connections. Wiring Chart. Chart of Wiring Links
Raspberry Pi Pins | HC-05 Bluetooth Pins |
---|---|
RX (GPIO15) | TX |
TX (GPIO14) | RX |
5V | VCC |
GND | GND |
Enable/Key (NOT connected) | |
State (NOT connected) |
Raspberry Pi sends data to Bluetooth App on Smartphone
In order to transmit data from a Raspberry Pi to a Bluetooth App on a Smartphone, the following code for the Raspberry Pi must be used:
In this example, we will have Raspberry Pi send “Raspberry Pi here, command me!” to the Bluetooth App on a Smartphone every second.
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Enable the Serial interface on Raspberry Pi by following the instruction on Raspberry Pi - how to enable Serial inteface
- Install the pyserial library for communication with bluetooth module:
- Create a Python script file bluetooth_send.py.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
- Install the Bluetooth Serial Monitor App on your smartphone.
- Wire the HC-05 Bluetooth module to Raspberry Pi as per the wiring diagram.
- Open the Bluetooth Serial Monitor App on your smartphone and select the Classic Bluetooth mode.
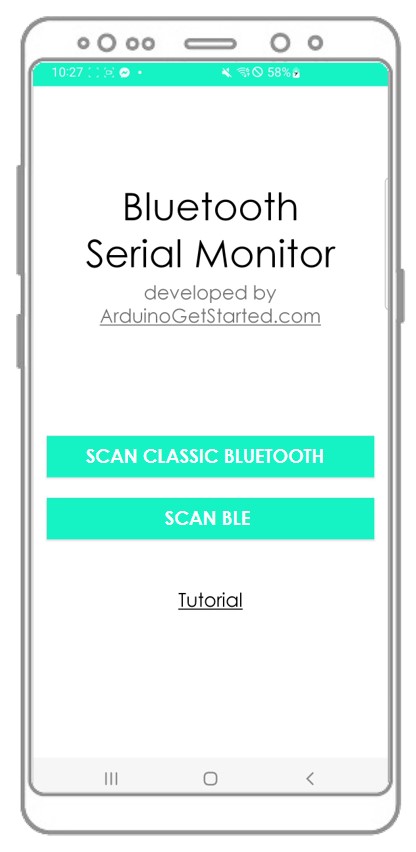
- Connect it to the HC-05 Bluetooth module.
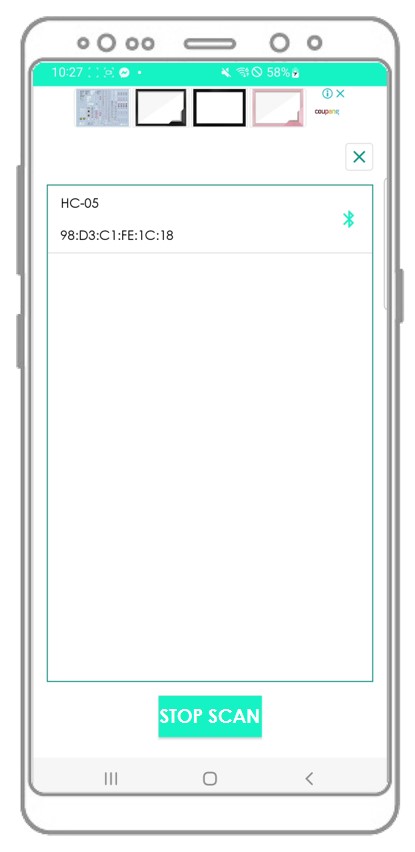
- Check out the result on the Android App.
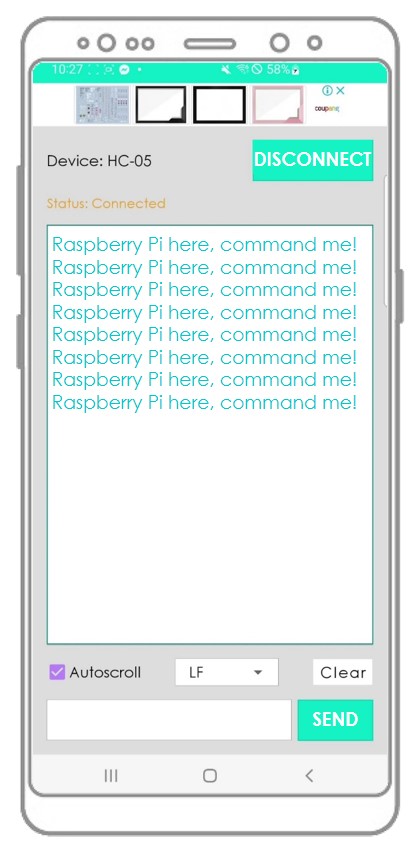
- Check out the result in the Terminal.
Bluetooth App Send data To Raspberry Pi
The following code:
- Allows a Bluetooth App to send data to a Raspberry Pi
- Enables the Raspberry Pi to read the data and send a response back to the Bluetooth App
Detailed Instructions
- Create a Python script file bluetooth_send_receive.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Connect the Android App to the HC-05 Bluetooth module, as done previously.
- Once connected, type either "LED ON" or "LED OFF" on the Android App and press the "SEND" button.
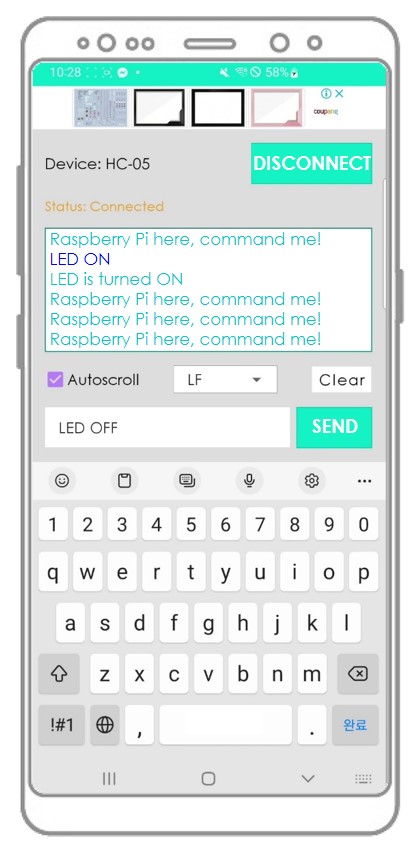
- Raspberry Pi receives the data and prints the response to the Serial port.
- This response will then be sent to the Bluetooth app.
- The result can be viewed on the Android App.
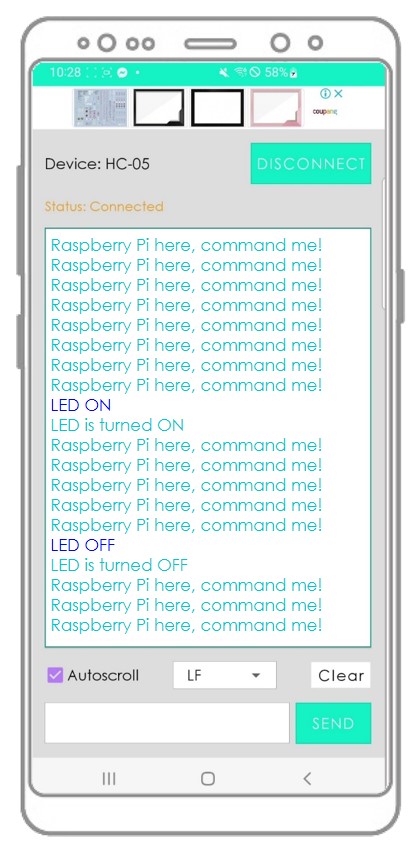
- Check out the output in the Terminal.
You will observe that the information displayed on the Android App are the same.
Raspberry Pi Code - Control LED with smartphone App via Bluetooth
Please refer to the Raspberry Pi controls LED via Bluetooth tutorial.
Raspberry Pi Code - Control Servo Motor with smartphone App via Bluetooth
Please refer to the Raspberry Pi controls Servo Motor via Bluetooth tutorial.