Raspberry Pi - Light Sensor LED
This tutorial instructs you how to use Raspberry Pi and light sensor module to trigger LED. In detail:
- When the light is not present, Raspberry Pi turns on the LED
- When the light is present, Raspberry Pi turns off the LED
The light sensor is also known as photoresistor, light-dependent resistor, photocell, LDR. The Raspberry Pi employs a light sensor to measure the ambient light level. If the surroundings are dark, the Raspberry Pi activates the LED, and conversely, if they are bright, it deactivates it.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables.
Overview of LED and Light Sensor
If you are not familiar with LED and light sensor (pinout, how it works, how to program ...), the following tutorials can help you out:
Wiring Diagram
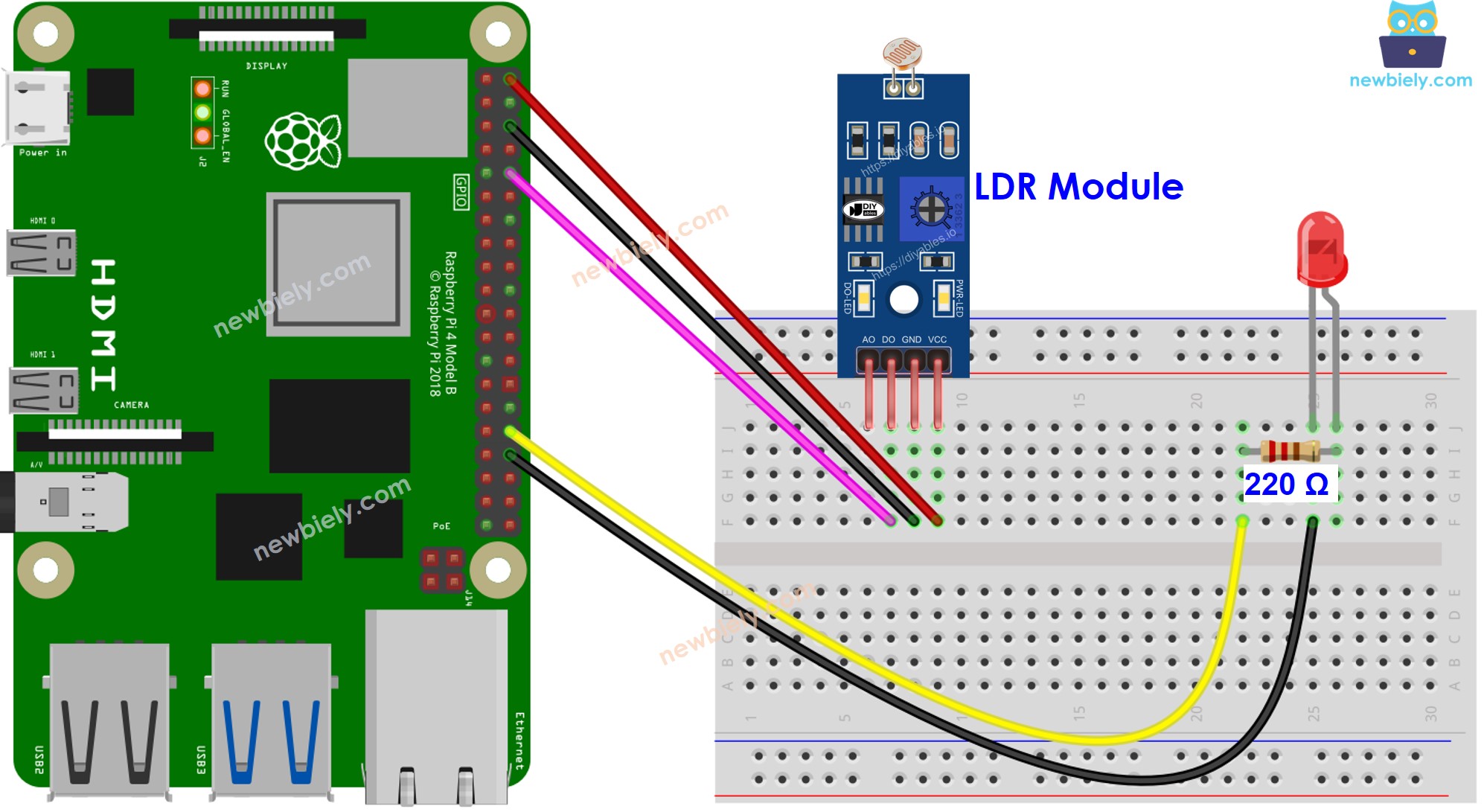
This image is created using Fritzing. Click to enlarge image
Raspberry Pi Code
The light sensor module outputs LOW when light is present and HIGH when light is not detected. The Raspberry Pi is programmed to read the value from the light sensor module and, based on the readings, activate the LED when light is not present and turn it off when light is detected.
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Create a Python script file ldr_led.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Cover the LRD light sensor module by your hand
- Check out the LED's state
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!