Raspberry Pi - 28BYJ-48 Stepper Motor ULN2003 Driver
This tutorial instructs you how to use Raspberry Pi to control 28BYJ-48 Stepper Motor using ULN2003 Driver. In detail, we will learn:
- How to connect Raspberry Pi to 28BYJ-48 stepper motor via ULN2003 driver
- How to program Raspberry Pi to control a single 28BYJ-48 stepper motor via ULN2003 driver
- How to program Raspberry Pi to control multiple 28BYJ-48 stepper motors via ULN2003 drivers
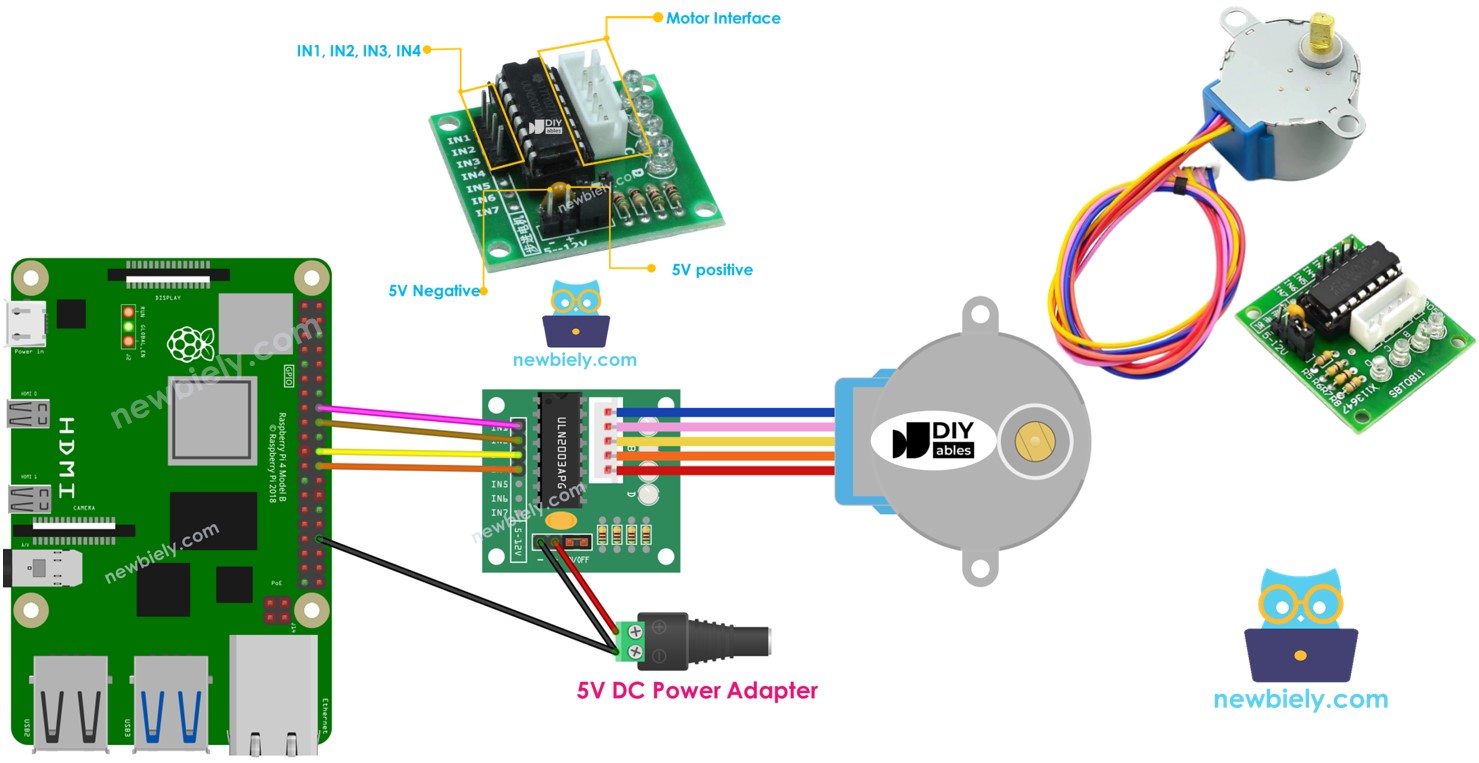
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of 28BYJ-48 Stepper Motor
Stepper motors are ideal for position control. They break a full revolution into a series of equal "steps". These motors are frequently found in printers, 3D printers, CNC machines, and industrial automation applications.
One of the cost-effective methods to gain knowledge about stepper motors is to utilize 28BYJ-48 stepper motors. These usually come with a ULN2003 based driver board, making them incredibly easy to use.
As per the data sheet, when the 28BYJ-48 motor is in full-step mode, each step is equivalent to an 11.25° rotation. Therefore, there are 32 steps for a single revolution (360°/11.25° = 32).
Furthermore, the motor has a 1/64 reduction gear set. This translates to 32 x 64 = 2048 steps. Each step is equal to 360°/2048 = 0.1758°.
Conclusion: If the motor is set to 2048 steps in full-step mode, it will rotate one revolution.
The 28BYJ-48 Stepper Motor using ULN2003 Driver Pinout
The 28BYJ-48 stepper motor has 5 pins. We do not need to be concerned with the specifics of these pins. All we need to do is plug it into the ULN2003 motor driver connector.
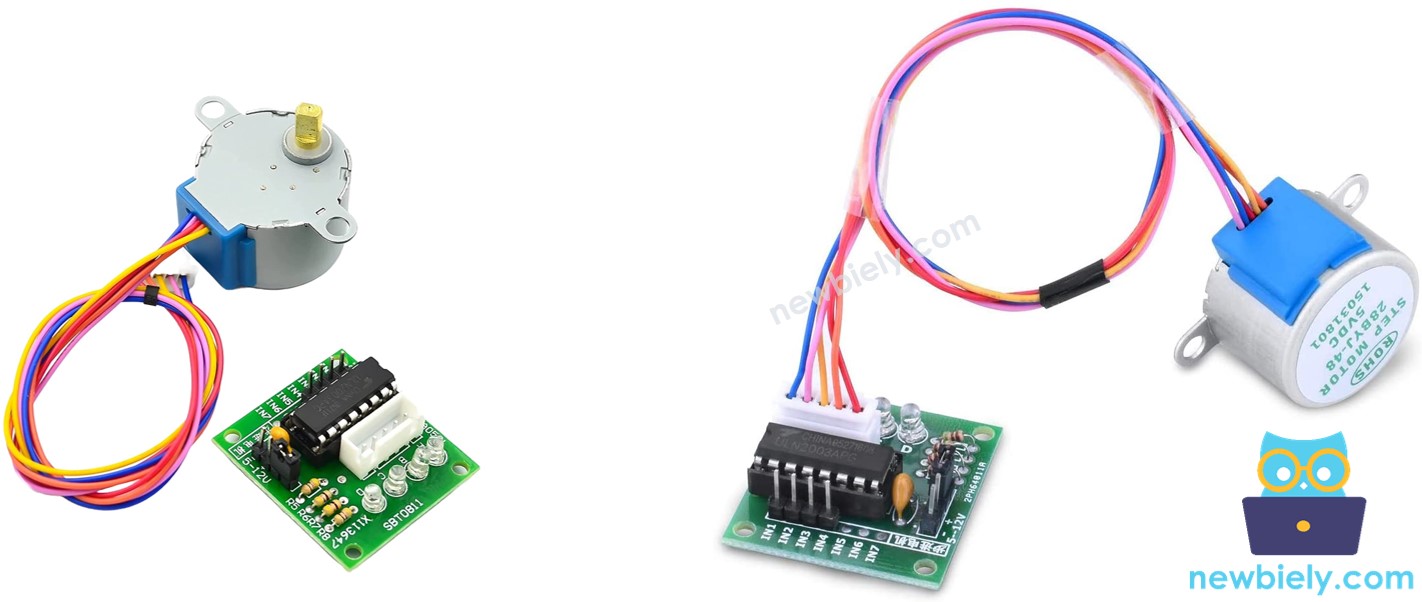
Overview of ULN2003 Stepper Motor Driver Module
The ULN2003 is a widely used motor driver Module for stepper motor.
- It has four LEDs that indicate the activity of the four control input lines, giving a great visual effect when stepping.
- Additionally, it comes with an ON/OFF jumper to cut off power supply to the stepper motor.
ULN2003 Pinout
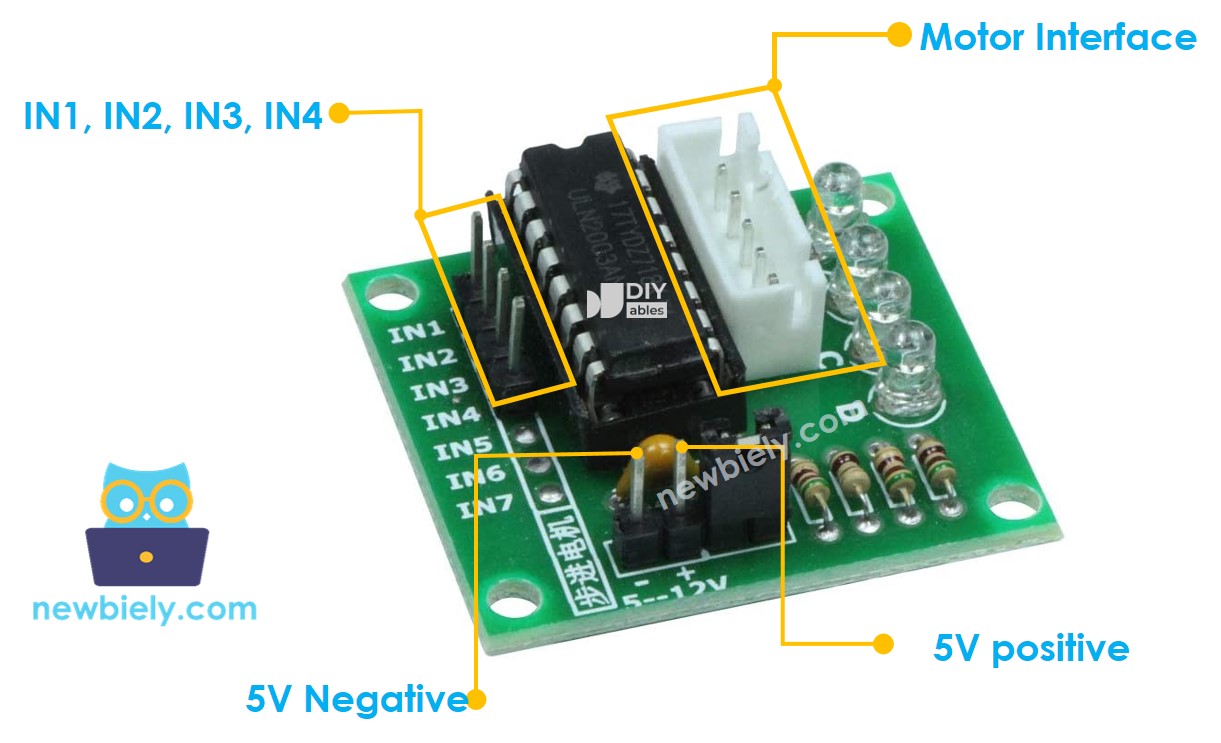
The ULN2003 module has 6 pins and a female connector:
- IN1 pin: this is used to drive the motor and should be connected to an output pin on Raspberry Pi.
- IN2 pin: this is used to drive the motor and should be connected to an output pin on Raspberry Pi.
- IN3 pin: this is used to drive the motor and should be connected to an output pin on Raspberry Pi.
- IN4 pin: this is used to drive the motor and should be connected to an output pin on Raspberry Pi.
- GND pin: this is a common ground pin and must be connected to both GNDs of Raspberry Pi and the external power supply.
- VDD pin: this supplies power for the motor and should be connected to the external power supply.
- Motor Connector: this is where the motor plugs into.
※ NOTE THAT:
- The voltage of the external power supply must match the voltage of the stepper motor. For instance, if a stepper motor needs 12V DC, we must use a 12V power supply. If the stepper motor is a 28BYJ-48, it requires 5V DC, so we will use a 5V power supply.
- However, even if the stepper motor requires 5V power supply, do NOT connect the VDD pin to the 5V pin on the Raspberry Pi. Instead, connect it to an external 5V power supply, as the stepper motor draws too much power.
Wiring Diagram
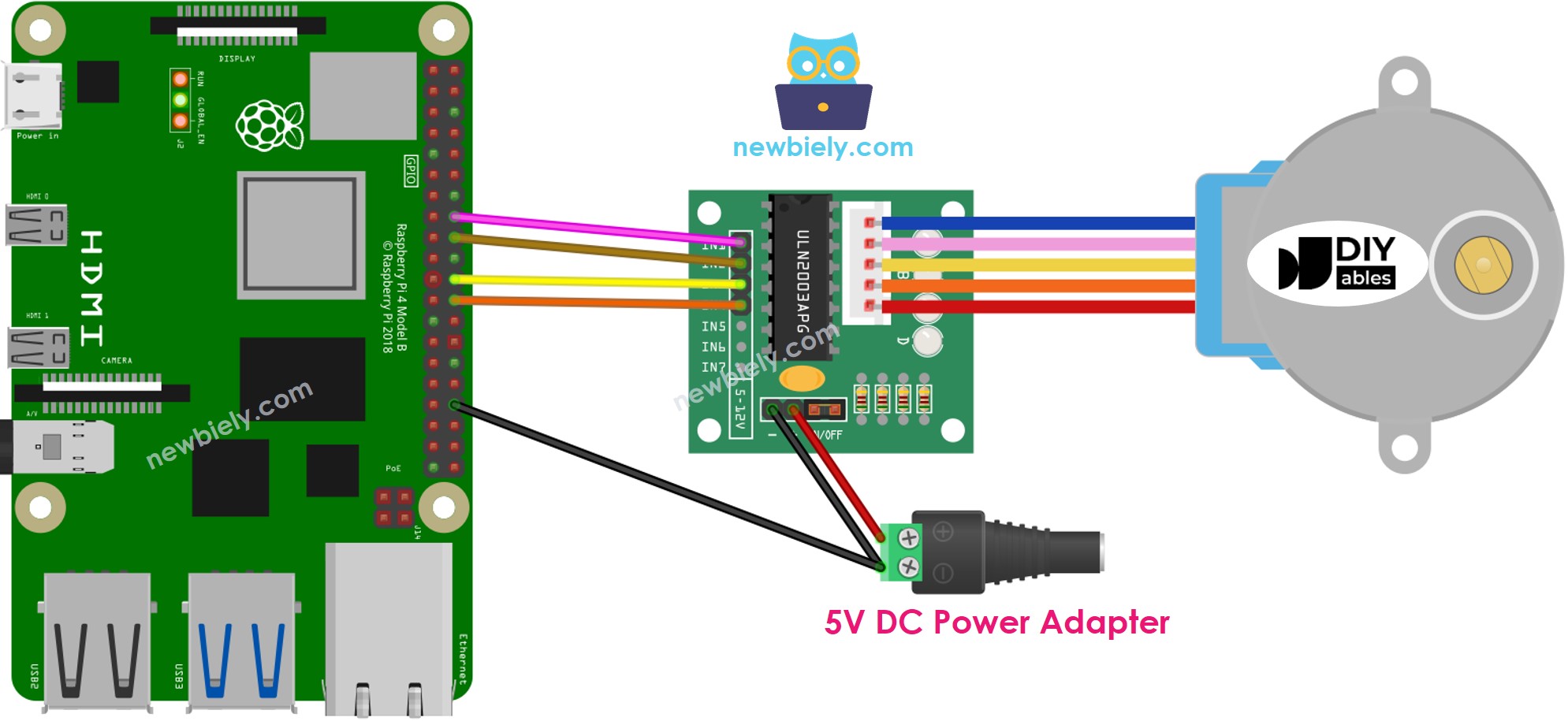
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
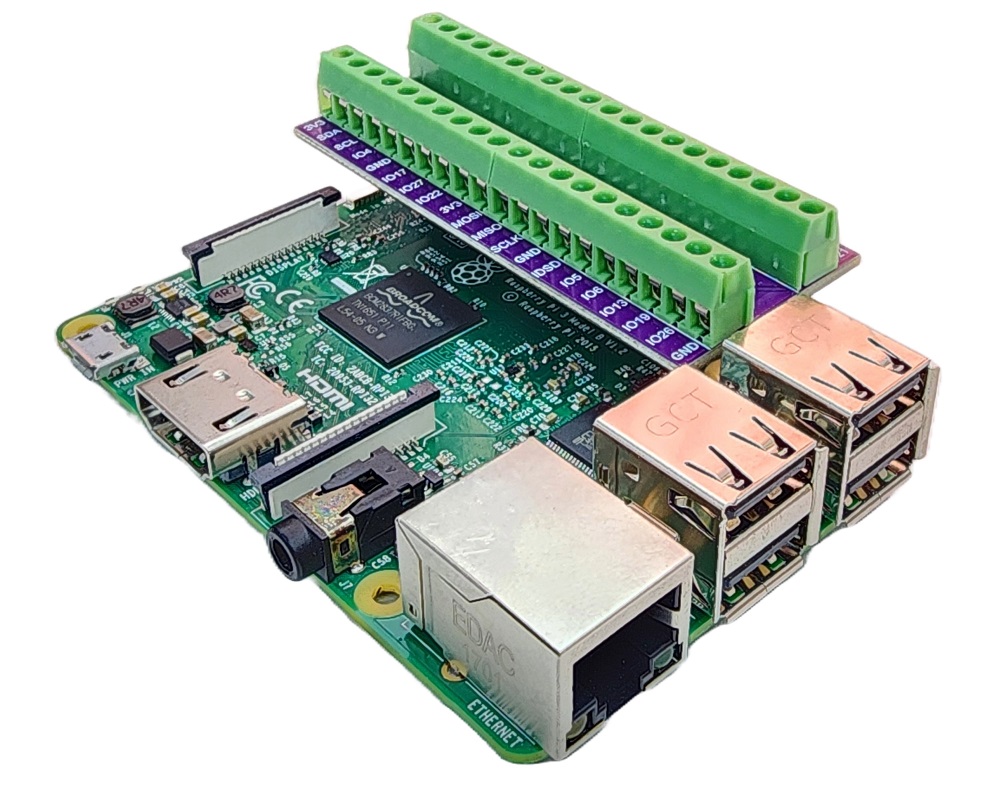
It is not necessary to pay attention to the color of the wires on the stepper motor. All that needs to be done is to connect the male connector on the 28BYJ-48 stepper motor to the female connector on the ULN2003 driver.
How To Program to control a stepper motor
There are three ways to regulate a stepper motor:
- Full-step
- Half-step
- Micro-step
For basic applications, the full-step method can be used. The details of the three methods will be discussed in the final section of this tutorial.
Raspberry Pi Code
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Create a Python script file stepper_uln2003.py and add the following code:
- Save the file and run the Python script by executing the following command in the Terminal:
- Check out the motor rotating.
- It should rotate one revolution in a clockwise direction, followed by two revolutions in an anti-clockwise direction, and then two revolutions in a clockwise direction.
By changing the value of delay variable in the code, you can change the speed of the stepper motor.
The script runs in an infinite loop continuously until you press Ctrl + C in the Terminal.
Additional Knowledge
Stepper motor vibrates while moving
Do not be concerned if the stepper motor shakes while in motion. This is a trait of the stepper motor. We can diminish the vibration by utilizing the micro-stepping control technique.
Additionally, due to its feature, if managed correctly, the stepper motor can create musical notes like a musical instrument. An example of this can be found here on Hackster.io.
Method of controlling stepper motors
- Full-step: The unit of movement is one step, which is equivalent to the value of degree indicated in the stepper motor's datasheet or manual.
- Half-step: Each full step is divided into two smaller steps. The unit of movement is half of the full step. This method allows the motor to move with double resolution.
- Micro-step: Each full step is divided into many smaller steps. The unit of movement is a fraction of the full step. The fraction can be 1/4, 1/8, 1/16, 1/32 or even more. This method allows the motor to move with higher resolution and smoother motion at low speeds. The larger the dividend, the higher the resolution and smoother the motion.
If the motor's datasheet specifies 1.8 degree/step:
- Full-step: The motor can move in increments of 1.8 degrees per step, resulting in 200 steps per revolution.
- Half-step: The motor can move in increments of 0.9 degrees per step, resulting in 400 steps per revolution.
- Micro-step: The motor can move in increments of 0.45, 0.225, 1125, 0.05625 degrees per step, resulting in 800, 1600, 3200, 6400... steps per revolution.
The code above used the full-step control technique.
Resonance Issue
This is for the more experienced users. Beginners do not need to worry about it. It occurs in a range of speeds, where the step rate is equal to the motor's natural frequency. There could be a noticeable change in the sound the motor makes, as well as an increase in vibration. In actual applications, developers should take this into account.