Raspberry Pi - Solenoid Lock
The Solenoid Lock, also referred to as the Electric Strike Lock, can be used to secure/release cabinets, drawers, and doors. This tutorial instructs you how to manipulate the solenoid lock using Raspberry Pi.
An alternative to the Solenoid Lock is the Electromagnetic Lock. For more information, please refer to the Raspberry Pi - Electromagnetic Lock tutorial.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Overview of Solenoid Lock
The Solenoid Lock Pinout
The Solenoid Lock has two wires:
- The Positive (+) wire (red) should be connected to the 12V of a DC power supply
- The Negative (-) wire (black) should be connected to the GND of a DC power supply
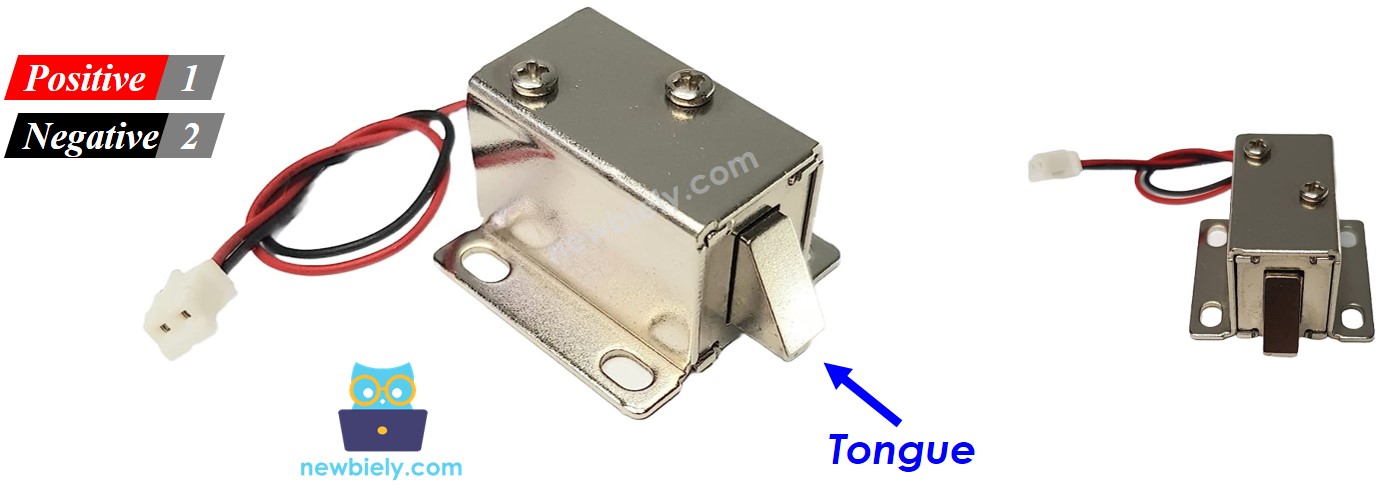
How It Works
- When the Solenoid Lock is powered, the lock tongue is extended, thus locking the door.
- When the Solenoid Lock is NOT powered, the lock tongue is retracted, thus unlocking the door.
※ NOTE THAT:
The solenoid lock typically requires 12V, 24V or 48V for operation. Therefore, it CANNOT be connected directly to a Raspberry Pi pin. A relay must be used to connect the solenoid lock to a Raspberry Pi pin.
If we connect the solenoid lock to the power source via a relay (in the normally open mode):
- When the relay is in an open state, the door will be unlocked.
- When the relay is in a closed state, the door will be locked.
We can connect the relay to Raspberry Pi and program Raspberry Pi to control the solenoid lock via relay. To find out more about relays, please refer to the Raspberry Pi - Relay tutorial.
Wiring Diagram
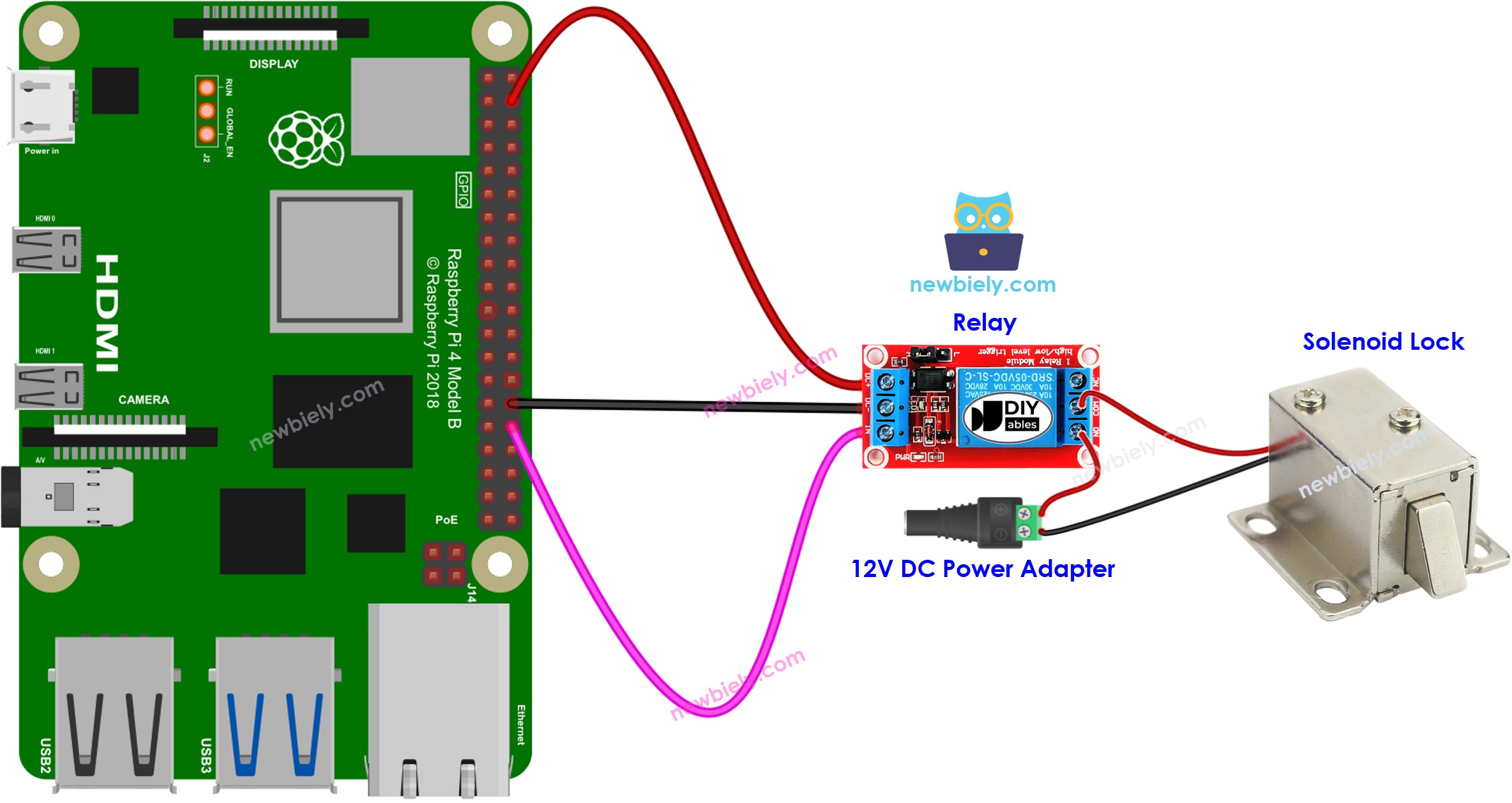
This image is created using Fritzing. Click to enlarge image
Raspberry Pi Code
The code below will cause the door to be locked and unlocked every 2 seconds.
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Create a Python script file solenoid_lock.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
- Check out the state of the lock tongue.
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.