Raspberry Pi - LED RGB
This tutorial instructs you how to use Raspberry Pi to control RGB LED. In detail, we will learn:
- How RGB LED works.
- How to connect an RGB LED to a Raspberry Pi.
- How to program the Raspberry Pi to control the color of the RGB LED.
Hardware Preparation
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of RGB LED
The RGB LED is capable of producing any color by combining the three primary colors: red, green, and blue. It is made up of three distinct LEDs ( red, green, and blue) but all contained in a single housing.
The RGB LED Pinout
An RGB LED has four pins:
- Common (Cathode-) pin which must be connected to GND (0V)
- R (red) pin to control red
- G (green) pin to control green
- B (blue) pin to control blue
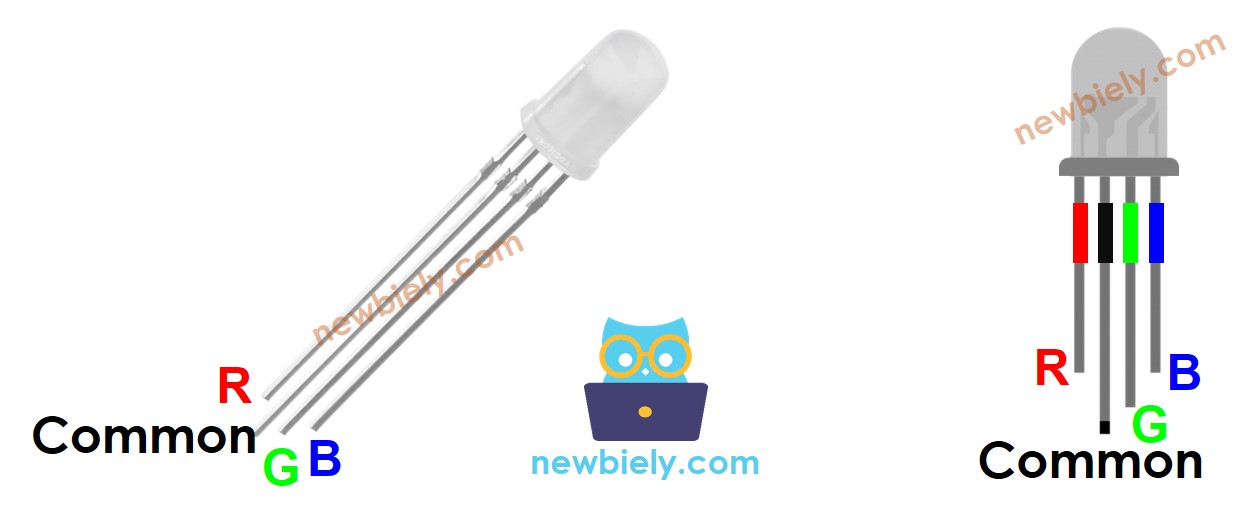
If we wanna hook up an RGB LED to an Raspberry Pi, we gotta use current-limiting resistors. It can be kinda tough to wire up, but don't worry. We can use this RGB LED module, that already has resistors built-in.
RGB LED Module also includes four pins:
- Common (Cathode-) pin: needs to be connected to GND (0V)
- R (red): pin is used to control red
- G (green): pin is used to control green
- B (blue): pin is used to control blue
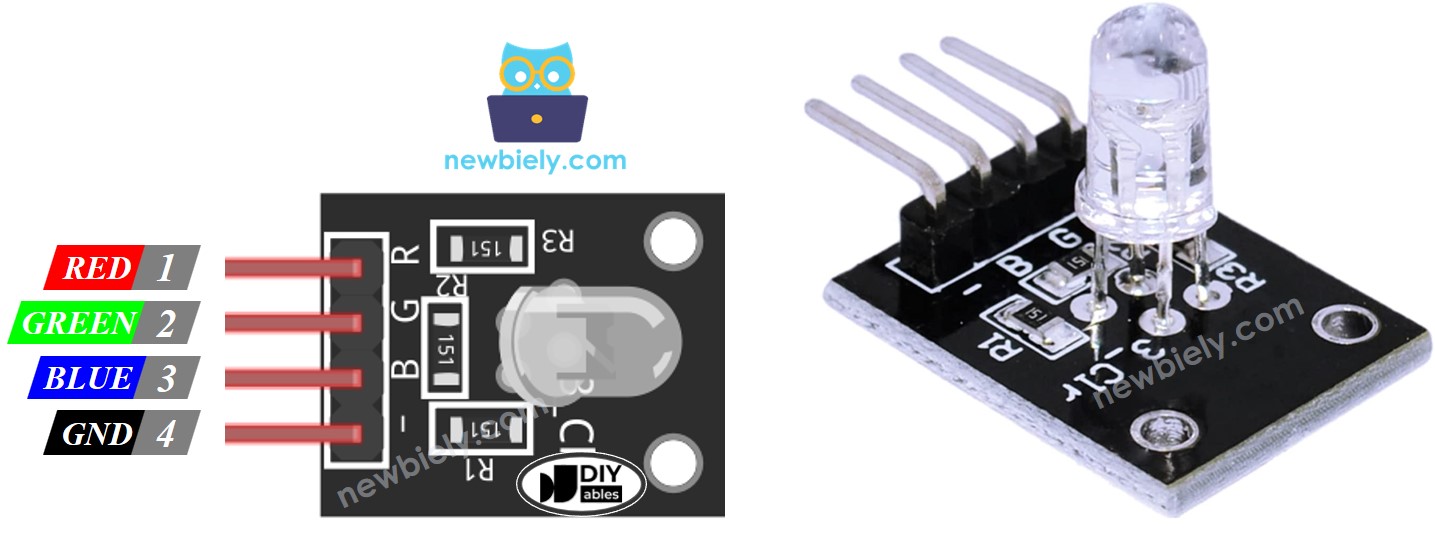
※ NOTE THAT:
The common type of pin for a RGB LED can vary; it can be either a cathode or anode. This tutorial will use a common cathode pin.
How it works
In the realm of physics, three color values - Red (R), Green (G) and Blue (B) - are combined to form a color. Each of these values has a range from 0 to 255. The combination of three values produces a total of 256 x 256 x 256 colors.
We can make RGB LED displays any color we desire by program Raspberry Pi to generate PWM signals (with duty cycle ranging from 0 to 255) to the R, G, and B pins. The duty cycle of PWM signals sent to the R, G and B pins are in proportion to the Red (R), Green (G) and Blue (B) color values respectively.
Wiring Diagram
- Wiring diagram between Raspberry Pi and RGB LED
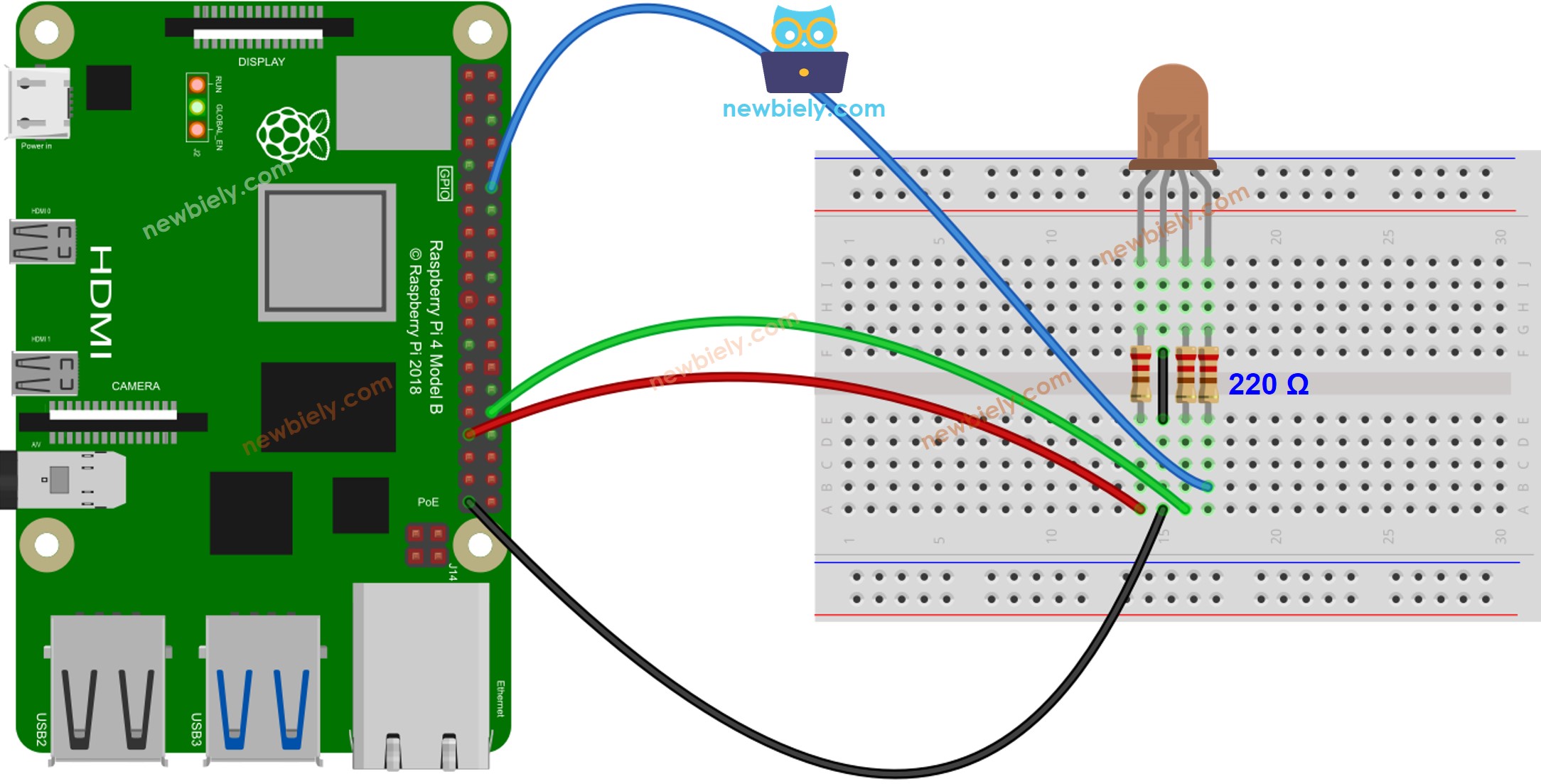
This image is created using Fritzing. Click to enlarge image
- Wiring diagram between Raspberry Pi and RGB LED module
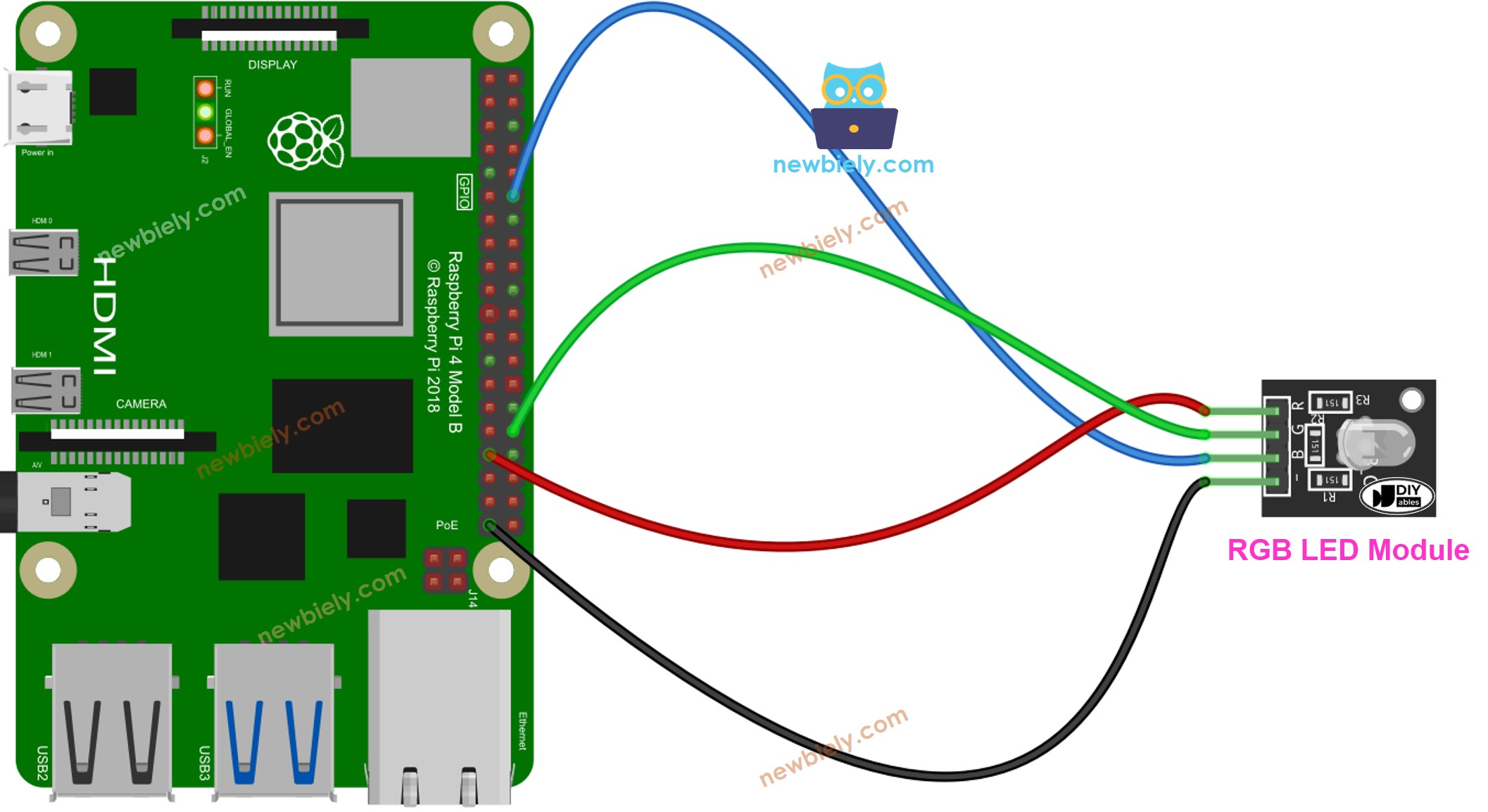
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
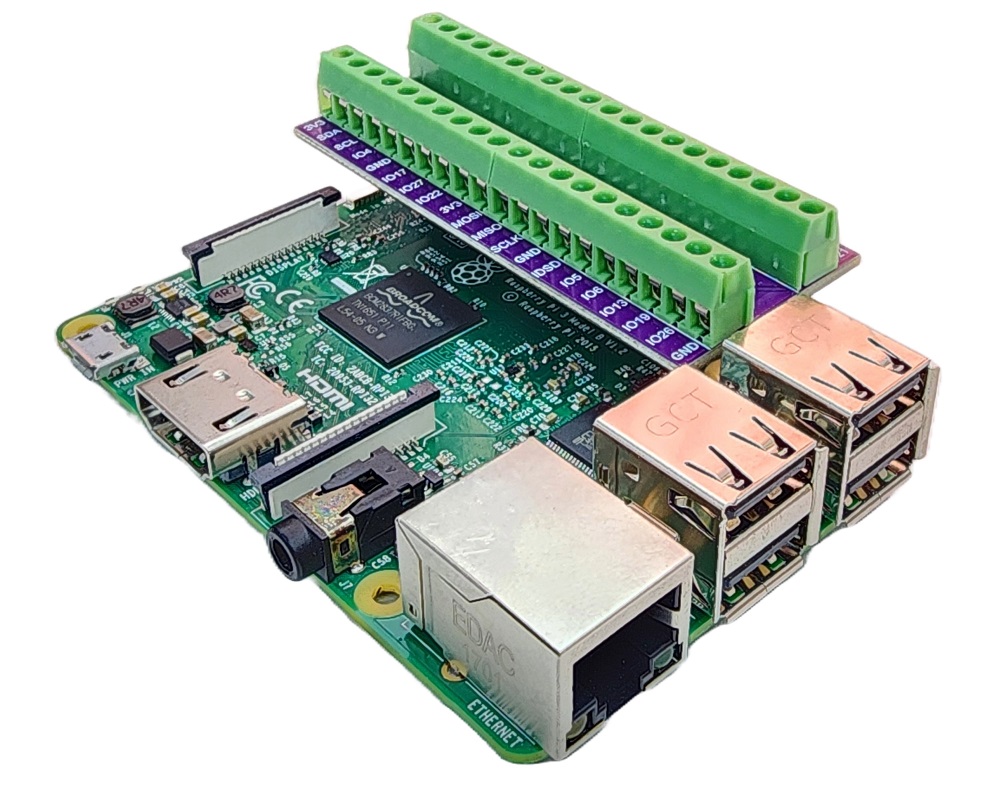
How To Control RGB LED
Let's learn how to control the GRB LED to any color, for example #00979D step-by-step:
- First, determine which color you want to display and get its color code. Tips:
- You can use the color picker to select the color code you want
- If you want to use a color from an image, you can use the online Colors From Image tool
- Then, convert the color code to R, G, B values using the tool from w3school. Make sure to take note of these values. In this case: R = 0, G = 151, B = 157
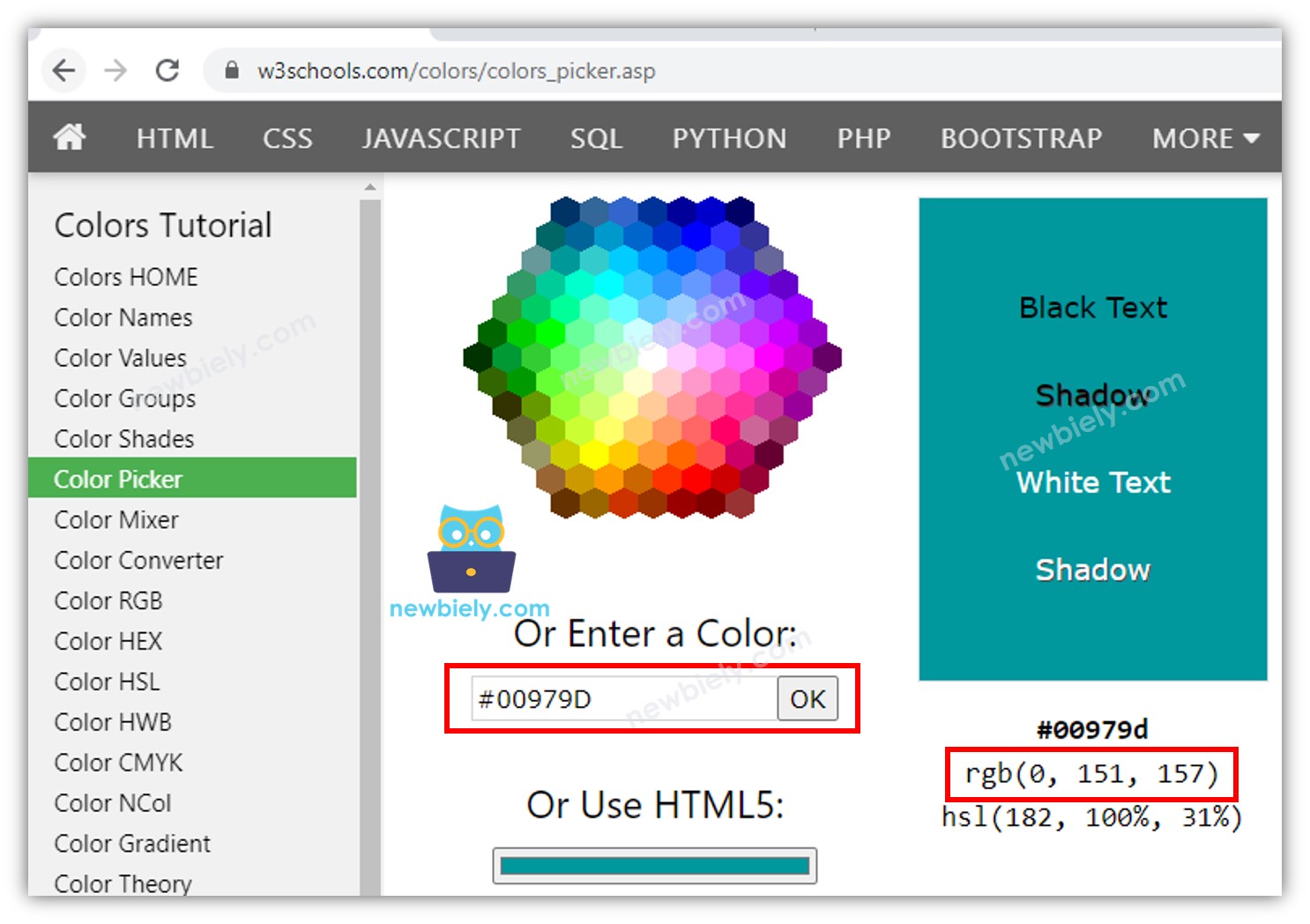
- Specify the Raspberry Pi pins that are connected to the R, G, and B pins. For example:
- Set the Raspberry Pi pins to output mode:
- Setup the Raspberry Pi pins to output PWM signal with frequency of 1000Hz:
- Control the LED to light up the color #00979D, which corresponds to R = 0, G = 151, B = 157.
Raspberry Pi - RGB LED Example Code
The Raspberry Pi code below changes the color of the LED in a sequence of the following shades:
- #00C9CC (Red = 0, Green = 201, Blue = 204)
- #F7788A (Red = 247, Green = 120, Blue = 138)
- #34A853 (Red = 52, Green = 168, Blue = 83)
When using many colors, we could shorten the Raspberry Pi code by creating a function:
Addtional Knowledge
For a RGB LED with a common Anode, you need to:
- Connect the common pin to 3.3V of a Raspberry Pi.
- Utilize the analogWrite() function and set the R, G and B values to 255 - R, 255 - G, and 255 - B, respectively.
An RGB LED Strip is made up of a sequence of RCB LEDs connected together. LED strips can be divided into addressable and non-addressable varieties. We will be making tutorials for each type of LED strip.
※ NOTE THAT:
Do not use a single resistor in the common pin of an RGB LED instead of three resistors in the other pins.
It is true that the three LEDs in a single RGB package are in parallel. In theory, it is ok to use a single resistor in the common pin. However, in reality, this is not recommended. This is because the real world LEDs do not have the same characteristics. The three LEDs in the RGB package are NOT identical ⇒ The resistors of the LEDs are different ⇒ The current is not evenly distributed to each LED ⇒ The brightness is not uniform and this could lead to the destruction of one LED, and eventually the other LEDs.