Raspberry Pi - Button - Servo Motor
This tutorial instructs you how to control a servo motor using an Raspberry Pi and a button. Here's how it works:
- When you press the button, the servo motor will rotate 90 degrees.
- When you press the button again, the motor will return to its original position of 0 degrees.
The same procedure is repeated infinitely.
Hardware Preparation
1 | × | Raspberry Pi 4 Model B | |
1 | × | Push Button | |
1 | × | (Optional) Panel-mount Push Button | |
1 | × | Servo Motor | |
1 | × | Breadboard | |
1 | × | Jumper Wires | |
1 | × | (Optional) Screw Terminal Adapter for Raspberry Pi |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you. We appreciate your support.
Wiring Diagram
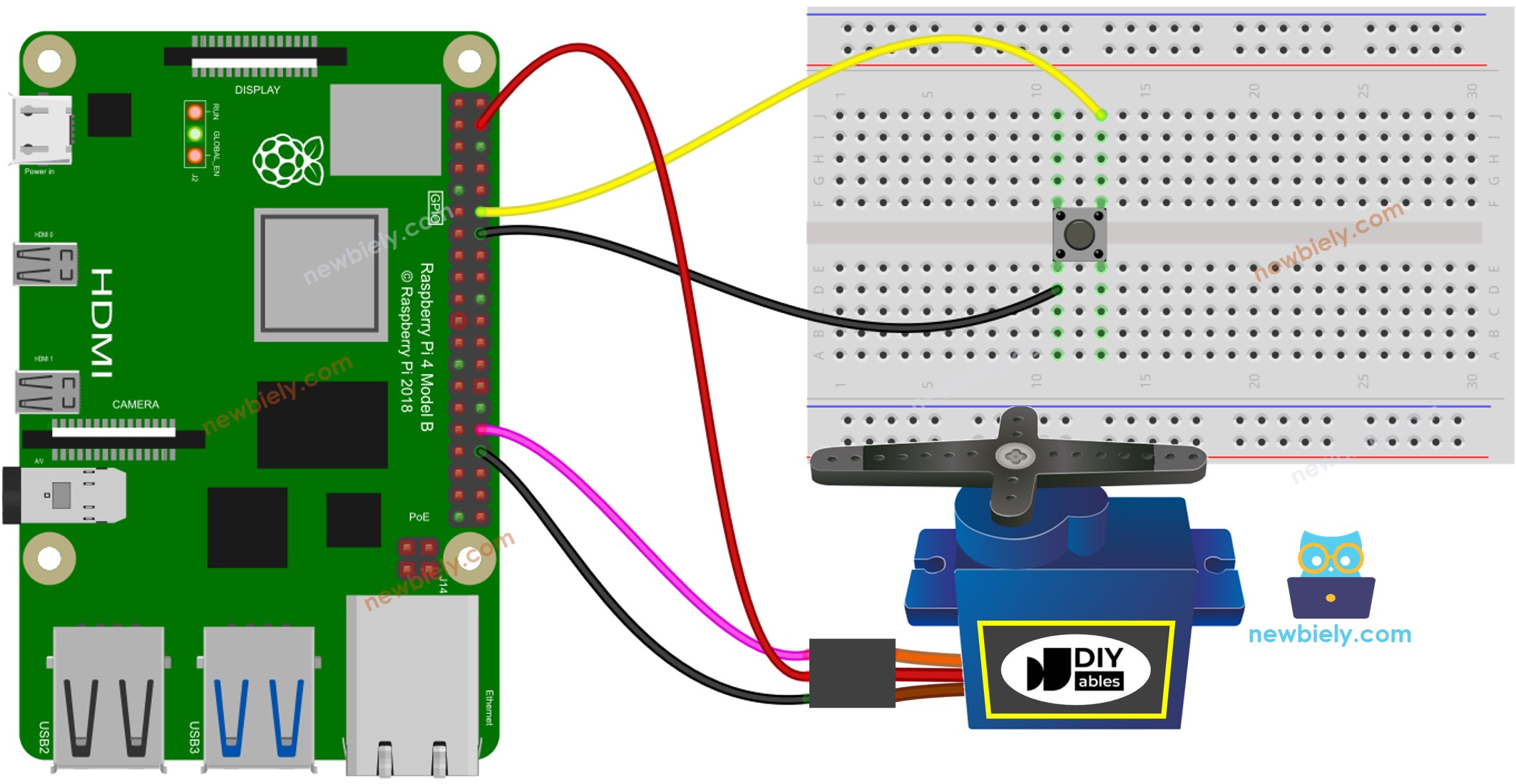
This image is created using Fritzing. Click to enlarge image
Please note that the wiring diagram shown above is only suitable for a servo motor with low torque. In case the motor vibrates instead of rotating, an external power source must be utilized to provide more power for the servo motor. The below demonstrates the wiring diagram with an external power source for servo motor.
TO BE ADD IMAGE
Please do not forget to connect GND of the external power to GND of Arduino Raspberry Pi.