Raspberry Pi - RFID - Relay
This tutorial instructs you how to use a Raspberry Pi and an RFID/NFC RC522 reader control a relay. You can also take this tutorial further and use the relay to control door lock, light bulb, motors, actuators, and so on.
Hardware Preparation
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some of these links are for products from our own brand, DIYables .
Overview of RFID/NFC RC522 Module and Relay
If you are unfamiliar with RFID/NFC RC522 Module and relay (pinout, how it works, how to program ...), the following tutorials can help you gain knowledge:
How It Works
- Some RFID/NFC tags have their UIDs pre-defined in the Raspberry Pi code.
- When a user taps an RFID/NFC tag on the RFID/NFC reader, the reader reads the UID from the tag.
- The Raspberry Pi then receives the UID from the reader and compares it with the pre-defined UIDs.
- If the UID matches one of the pre-defined UIDs, the Raspberry Pi will activate the relay.
Wiring Diagram
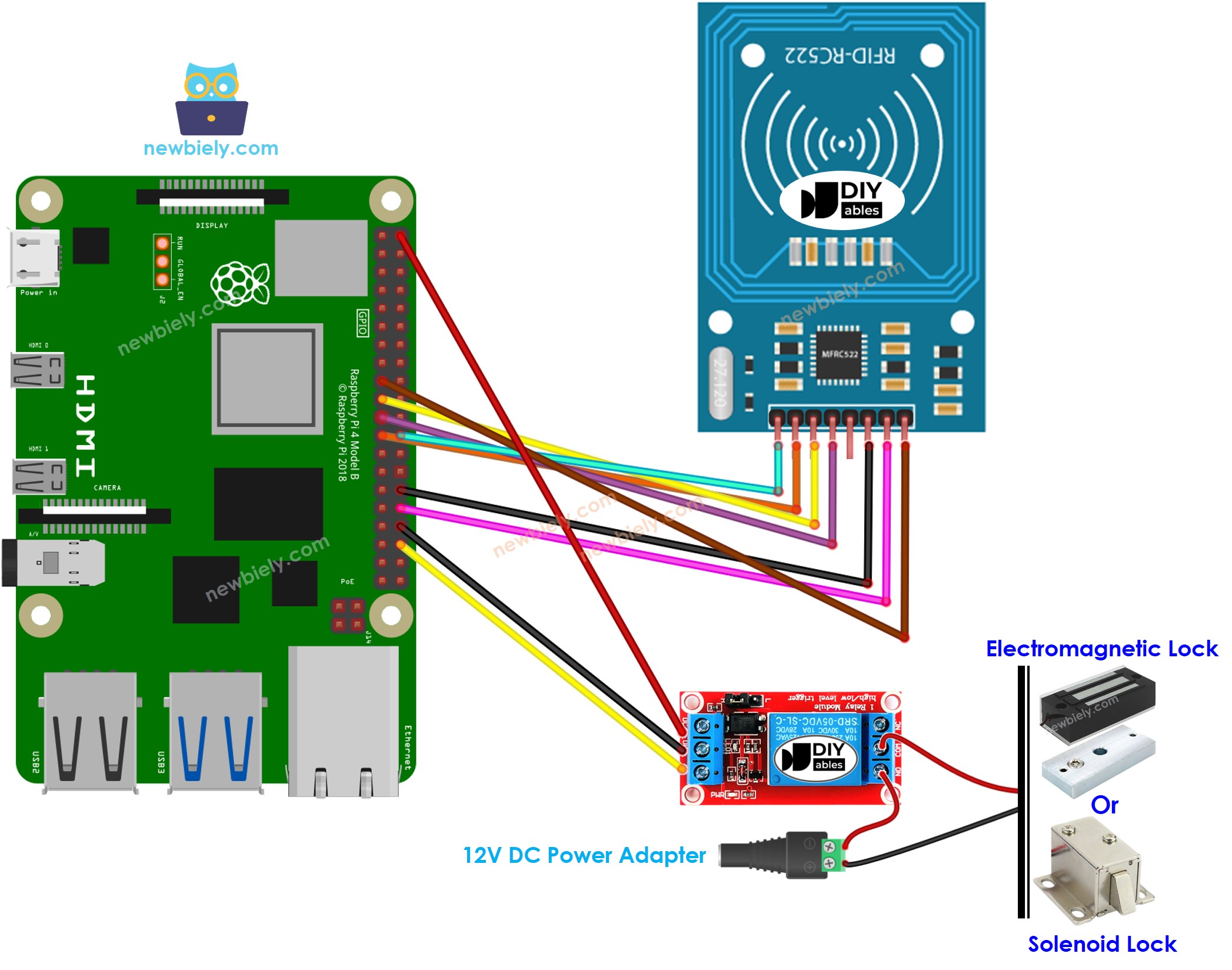
This image is created using Fritzing. Click to enlarge image
To simplify and organize your wiring setup, we recommend using a Screw Terminal Block Shield for Raspberry Pi. This shield ensures more secure and manageable connections, as shown below:
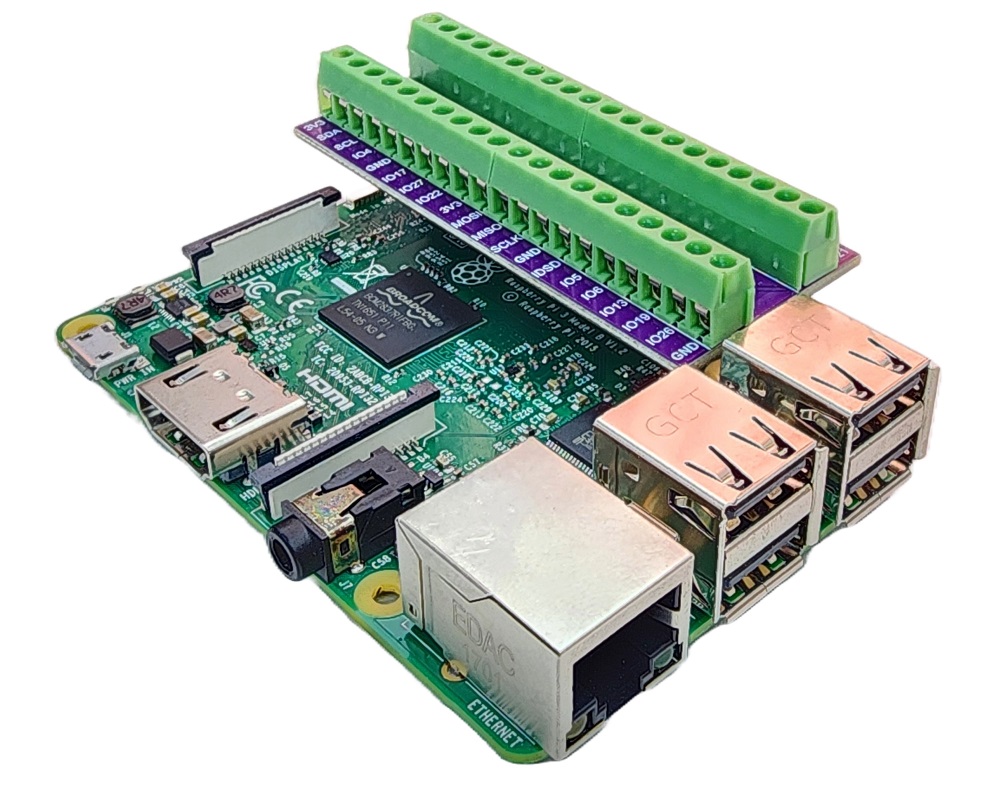
※ NOTE THAT:
The arrangement of pins may differ depending on the manufacturer. ALWAYS utilize the labels printed on the module. The image above displays the pinout of the modules from DIYables maker.
Raspberry Pi Code - Single RFID/NFC Tag
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Enable the SPI interface on Raspberry Pi by following the instruction on Raspberry Pi - how to enable SPI inteface
- Make sure you have the spidev library installed. If not, install it using the following command:
- Make sure you have the mfrc522 library installed. If not, install it using the following command:
- Create a Python script file rfid_relay.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
In order to identify the UID of an RFID/NFC tag:
- Run the above code and tap the tag on the RFID-RC522 module.
- The UID will be displayed on the Serial Monitor.
After obtaining the UID:
- Alter line 18 of the code to reflect the UID, for example changing byte authorizedUID[4] = {0xFF, 0xFF, 0xFF, 0xFF}; to byte authorizedUID[4] = {0x3A, 0xC9, 0x6A, 0xCB};
- Upload the code to the Raspberry Pi
- Place an RFID/NFC tag on the RFID-RC522 module
- Check out the output on the Serial Monitor
- Tap a different RFID/NFC tag onto the RFID-RC522 module.
- Check the output on the Serial Monitor.
※ NOTE THAT:
- To facilitate testing, the active time has been set to two seconds; however, it should be increased for practical use or demonstration.
- Installation of the MFRC522 library is necessary. For more information, please refer to the Raspberry Pi - RFID/NFC RC522 tutorial.