Raspberry Pi - Servo Motor
This tutorial instructs you how to use Raspberry Pi to control servo motor. In detail, we will learn:
- How to connect servo motor to Raspberry Pi
- How to program for Raspberry Pi to rotate a servo motor
- How to control the speed of a servo motor using Raspberry Pi
Hardware Preparation
1 | × | Raspberry Pi 4 Model B | |
1 | × | Servo Motor | |
1 | × | Jumper Wires | |
1 | × | (Optional) Screw Terminal Adapter for Raspberry Pi | |
1 | × | (Optional) Power Adapter for Raspberry Pi 4B | |
1 | × | (Optional) Plastic Case for Raspberry Pi 4B |
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Overview of Servo Motor
A servo motor is a component capable of rotating its shaft within a specific range of motion, typically between 0 and 180 degrees. It is commonly used to control the angular position of an object.
The Servo Motor Pinout
The servo motor has three pins:
- VCC pin (usually red) which needs to be connected to VCC (5V)
- GND pin (typically black or brown) which needs to be connected to GND (0V)
- Signal pin (normally yellow or orange) which receives the PWM control signal from a Raspberry Pi's pin.
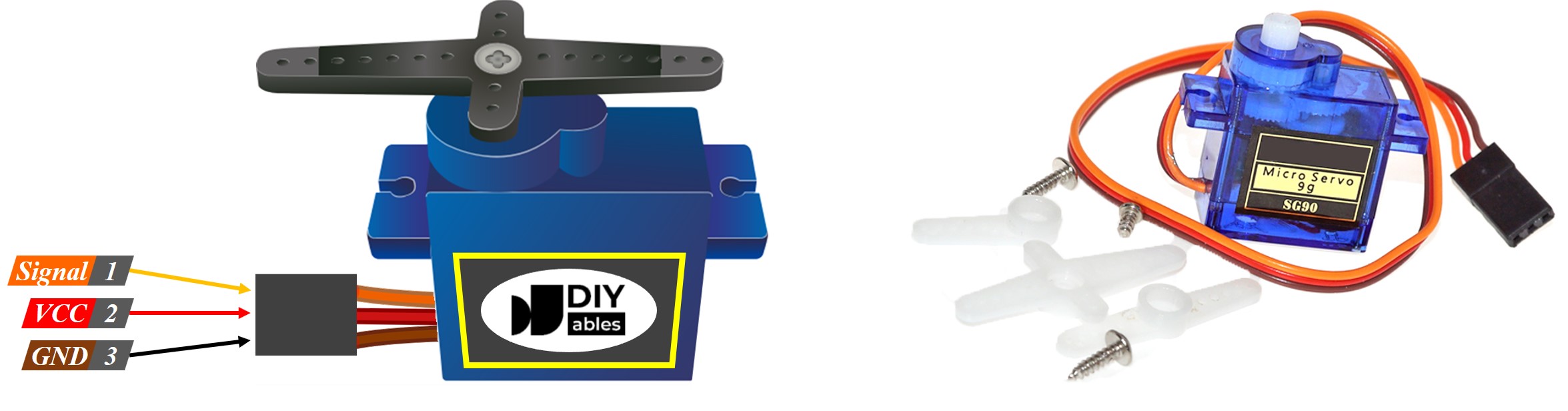
Raspberry Pi - Servo Motor
Some of the pins on a Raspberry Pi can be programmed to produce a PWM signal. We can control a servo motor by connecting its signal pin to one of the Raspberry Pi's pins and programming it to generate a PWM on that pin.
Thanks to the Raspberry Pi Servo library, controlling a servo motor is made easy. We don't need to understand the workings of the servo motor or how to generate a PWM signal. All we need to do is learn how to use the library.
Wiring Diagram
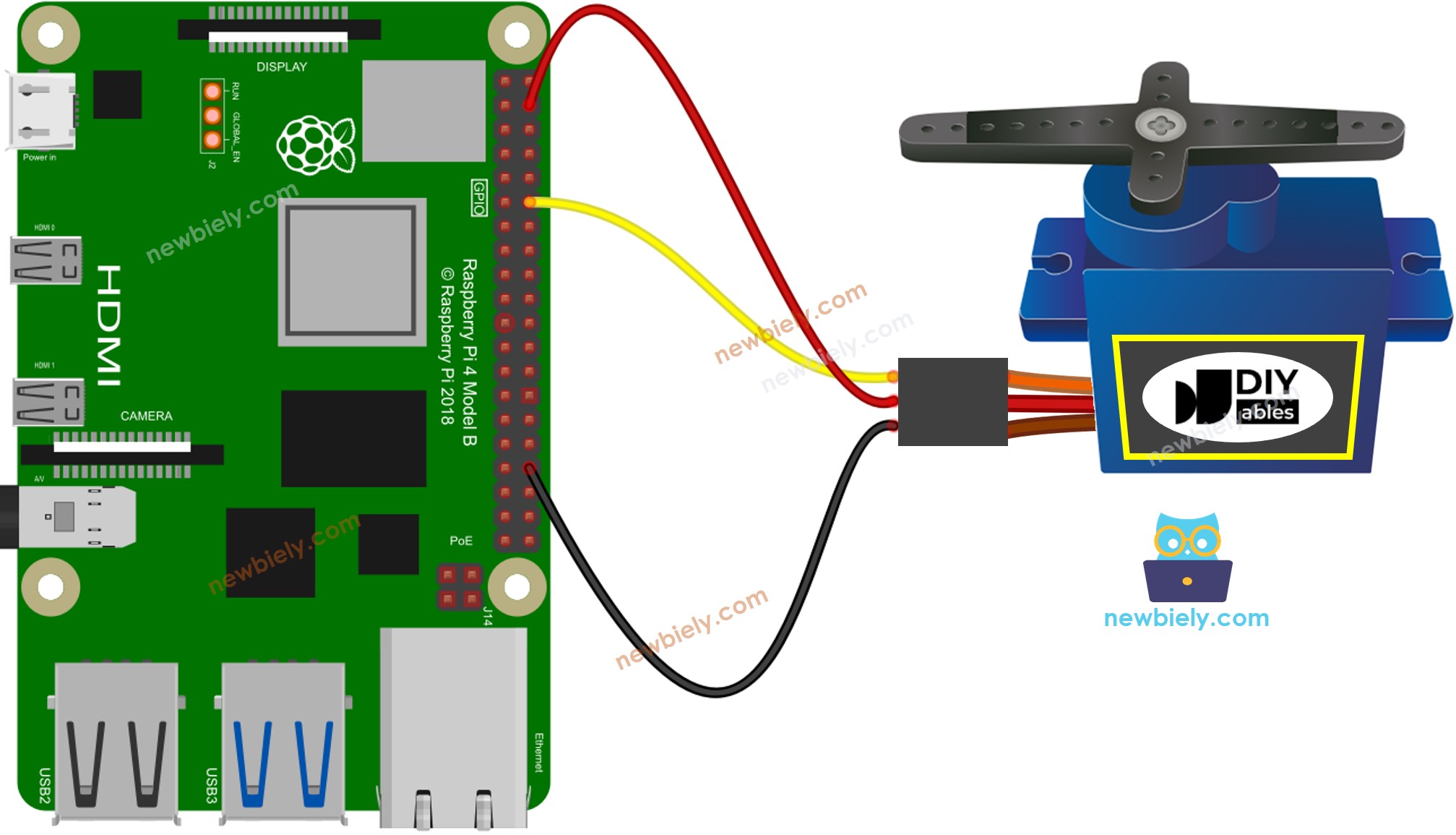
This image is created using Fritzing. Click to enlarge image
For the purpose of keeping things simple, the wiring diagram above is used for testing and learning, and is suitable for a servo motor with low torque. We strongly suggest that an external power supply be used for the servo motor. The wiring diagram below shows how to connect the servo motor to an external power source.
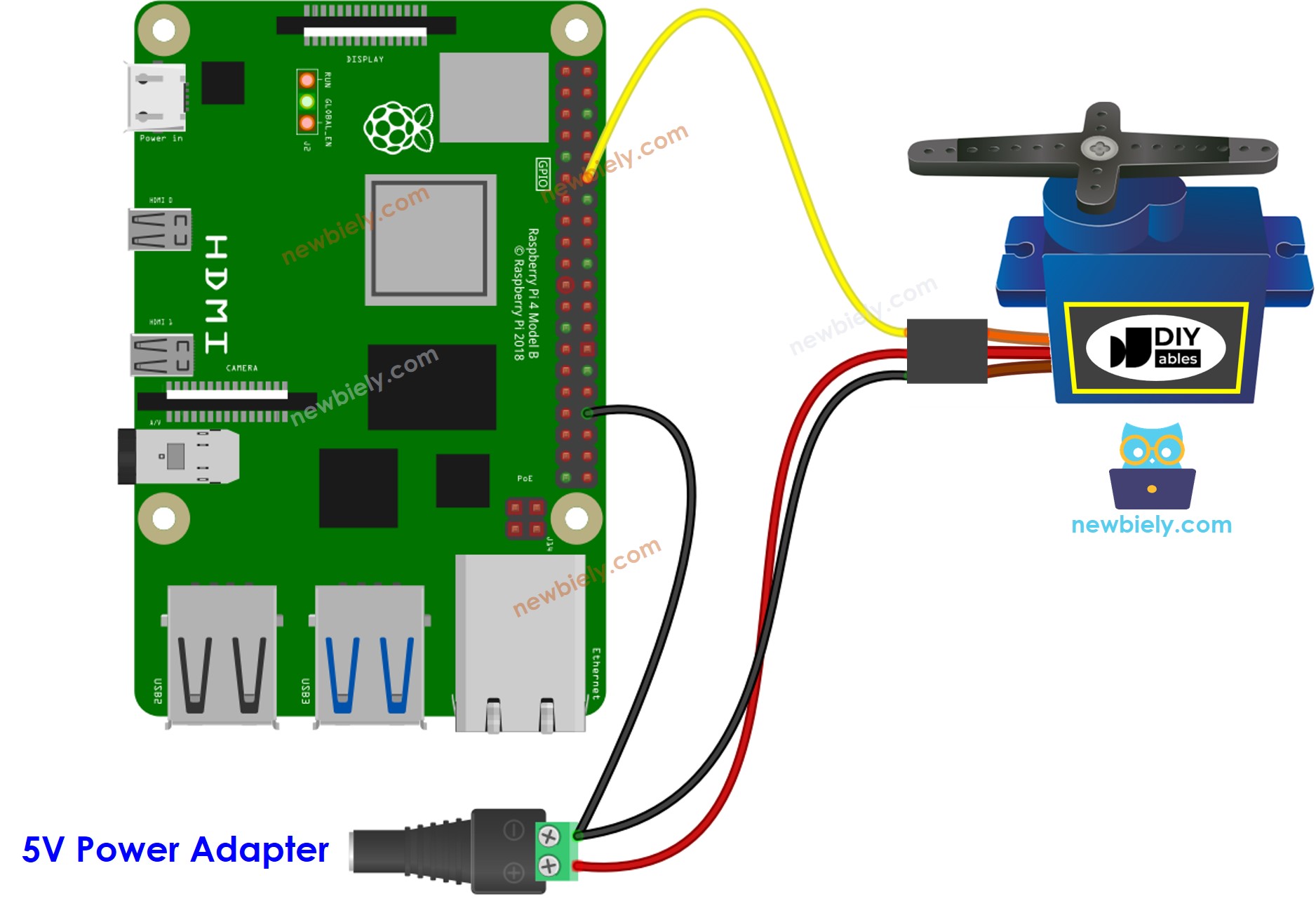
This image is created using Fritzing. Click to enlarge image
Raspberry Pi Code
Detailed Instructions
- Make sure you have Raspbian or any other Raspberry Pi compatible operating system installed on your Pi.
- Make sure your Raspberry Pi is connected to the same local network as your PC.
- Make sure your Raspberry Pi is connected to the internet if you need to install some libraries.
- If this is the first time you use Raspberry Pi, See how to set up the Raspberry Pi
- Connect your PC to the Raspberry Pi via SSH using the built-in SSH client on Linux and macOS or PuTTY on Windows. See to how connect your PC to Raspberry Pi via SSH.
- Make sure you have the RPi.GPIO library installed. If not, install it using the following command:
- Create a Python script file servo_motor.py and add the following code:
- Save the file and run the Python script by executing the following command in the terminal:
The script runs in an infinite loop continuously until you press Ctrl + C in the terminal.
- Check out the outcome: The servo motor rotates at a slow speed in both the clockwise and counter-clockwise directions, up to 180°.
Code Explanation
Check out the line-by-line explanation contained in the comments of the source code!