Arduino Nano ESP32 - Button LED
This tutorial guides you through utilizing the Arduino Nano ESP32 and a button to control the LED. We will explore two distinct use-cases:
Use-Case 1 - Synchronization between the LED and button states, explained as follows:
Use-Case 2 - Toggling the LED state with each button press, detailed as follows:
If the Arduino Nano ESP32 detects a transition from a HIGH to a LOW state in the button (indicating a press), it toggles the LED ON if it's currently OFF, or OFF if it's currently ON.
Releasing the button has no impact on the LED state.
In Use-Case 2, it is crucial to debounce the button to ensure proper functionality. We will explore the significance of debouncing by comparing the LED behavior when using the Arduino Nano ESP32 code with and without button debouncing.
Or you can buy the following sensor kits:
Disclosure: Some of the links provided in this section are Amazon affiliate links. We may receive a commission for any purchases made through these links at no additional cost to you.
Additionally, some of these links are for products from our own brand,
DIYables .
If you are unfamiliar with LED and button (including pinout, operation, and programming), the following tutorials can help:
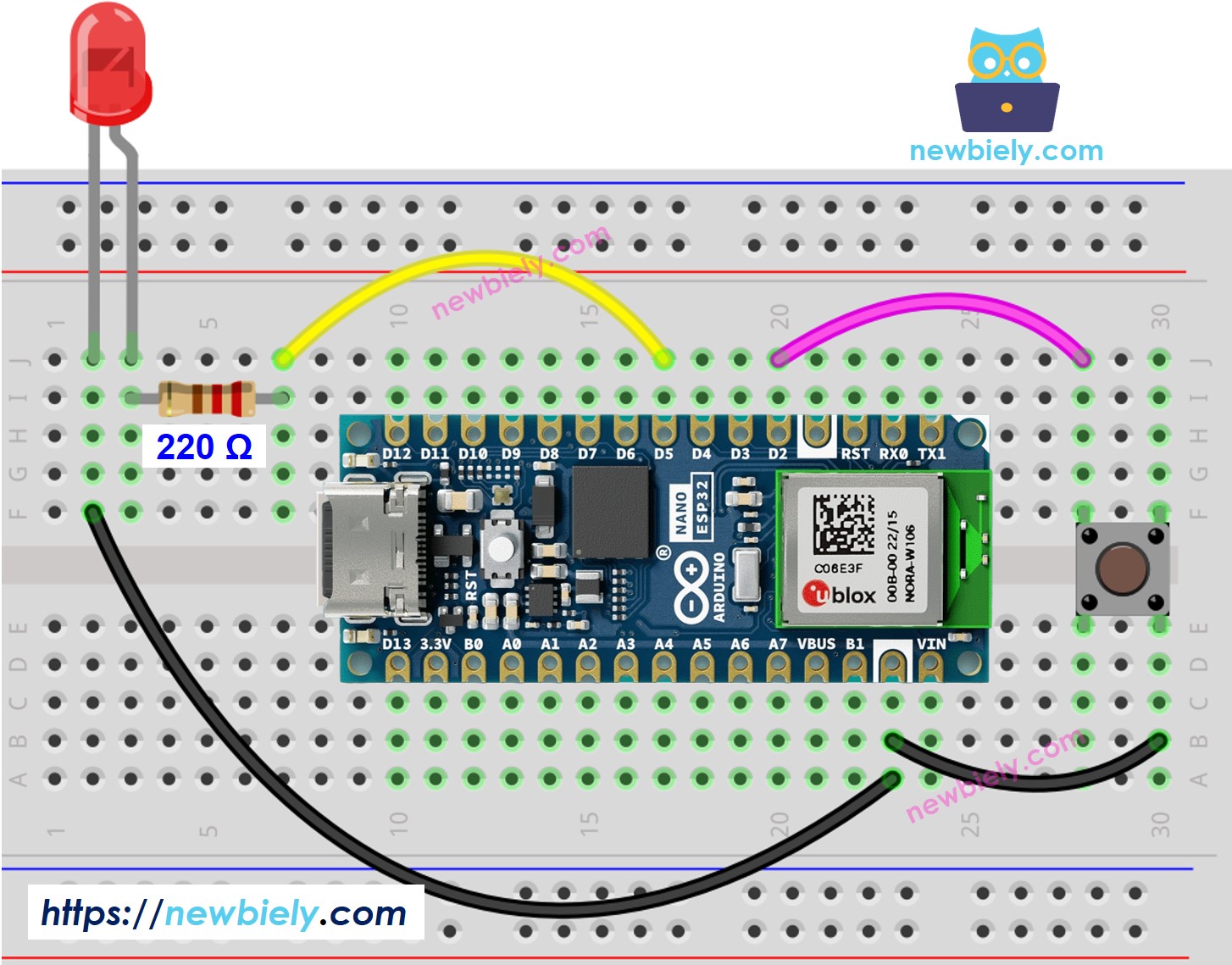
This image is created using Fritzing. Click to enlarge image
#define BUTTON_PIN D2
#define LED_PIN D5
int button_state = 0;
void setup() {
pinMode(LED_PIN, OUTPUT);
pinMode(BUTTON_PIN, INPUT_PULLUP);
}
void loop() {
button_state = digitalRead(BUTTON_PIN);
if(button_state == LOW)
digitalWrite(LED_PIN, HIGH);
else
digitalWrite(LED_PIN, LOW);
}
Wire the components as shown in the diagram.
Connect the Arduino Nano ESP32 board to your computer using a USB cable.
Open Arduino IDE on your computer.
Choose the Arduino Nano ESP32 board, and its respective COM port.
Copy the code and open it in the Arduino IDE.
Click the Upload button on the Arduino IDE to compile and upload the code to the Arduino Nano ESP32.
You will see that the LED state is in sync with the button state.
Check out the line-by-line explanation contained in the comments of the source code!
#define BUTTON_PIN D2
#define LED_PIN D5
int led_state = LOW;
int prev_button_state;
int button_state;
void setup() {
Serial.begin(9600);
pinMode(BUTTON_PIN, INPUT_PULLUP);
pinMode(LED_PIN, OUTPUT);
button_state = digitalRead(BUTTON_PIN);
}
void loop() {
prev_button_state = button_state;
button_state = digitalRead(BUTTON_PIN);
if(prev_button_state == HIGH && button_state == LOW) {
Serial.println("The button is pressed");
led_state = !led_state;
digitalWrite(LED_PIN, led_state);
}
}
You can locate the explanation in the comment lines of the Arduino Nano ESP32 code above.
In the code, the expression led_state = !led_state is equal to the following code:
if(led_state == LOW)
led_state = HIGH;
else
led_state = LOW;
Copy the code and open it in the Arduino IDE.
Upload the code to the Arduino Nano ESP32.
Press release and button several times.
Check out the change in the LED's state.
You may observe that the LED state is toggled every time the button is pressed. However, this behavior may not always be consistent. At times, the LED state may be rapidly toggled multiple times within a single button press, or it may not toggle at all(toggling twice in quick succession which can be difficult to see with the naked eye).
⇒ To solve this problem, we need to debounce for the button.
Debouncing a button can be challenging for beginners. Fortunately, the ezButton library makes it easy.
Why is debouncing necessary? See the Arduino Nano ESP32 - Button Debounce tutorial for more information.
#include <ezButton.h>
#define BUTTON_PIN D2
#define LED_PIN D5
ezButton button(BUTTON_PIN);
int led_state = LOW;
void setup() {
Serial.begin(9600);
pinMode(LED_PIN, OUTPUT);
button.setDebounceTime(50);
}
void loop() {
button.loop();
if(button.isPressed()) {
Serial.println("The button is pressed");
led_state = !led_state;
digitalWrite(LED_PIN, led_state);
}
}
Install the ezButton library. Refer to
How To for instructions.
Copy the code and open it with Arduino IDE.
Click the Upload button on Arduino IDE to upload the code to the Arduino Nano ESP32.
Press and release the button multiple times.
Check out the LED's state change.
You will see that the LED state is toggled exactly once each time the button is pressed.